


Generators in PHP7: How to efficiently process large amounts of data and speed up code execution?
Generators in PHP7: How to efficiently process large amounts of data and speed up code execution?
With the development of the Internet, the amount of data we face is getting larger and larger, and processing large amounts of data has become an important task for developers. Generator was introduced in PHP7, which provides us with a way to efficiently process large amounts of data and accelerate code execution.
A generator is a special iterator in PHP. Using a generator, you can generate values one by one in a loop without loading all the data into memory at once. This delayed generation of values can greatly save memory space and reduce the time of data acquisition and processing.
The basic syntax of the generator is as follows:
function generator_function() { // 生成值的逻辑 yield $value1; // 生成更多的值 yield $value2; // ... } $generator = generator_function();
Among them, the yield keyword is used to generate a value and return it to the caller. When a generator function is called, it does not immediately execute the code in the function body, but returns a generator object. Then, each time the next() method of the generator object is called, the generator function will run until the yield keyword is encountered and generate a value and return it to the caller.
Below we illustrate the usage of the generator through a practical example. Suppose we have an array holding 1 million numbers, we need to process these numbers one by one and perform corresponding operations.
function process_numbers($numbers) { foreach ($numbers as $number) { // 处理数字的逻辑 yield $result; } } $numbers = range(1, 1000000); $generator = process_numbers($numbers); foreach ($generator as $result) { echo $result . PHP_EOL; }
In the above code, we define a generator function process_numbers(), which receives an array holding 1 million numbers as a parameter. In the loop, we process each number and return the result through the yield keyword. Then, we traverse the generator object $generator through the foreach loop and output the results one by one.
By using generators, we can only process one number at a time and avoid loading all the data into memory. This not only saves memory space but also speeds up code execution.
In addition to the basic generator syntax, PHP7 also provides some additional features to make generators more powerful and controllable.
First of all, the generator function can receive parameters to customize the behavior of the generator according to actual needs. For example, we can limit the number of values produced by a generator by passing parameters to the generator function.
function limited_generator($limit) { for ($i = 1; $i <= $limit; $i++) { yield $i; } } $generator = limited_generator(100);
In the above code, we define a generator function limited_generator() that receives the parameter $limit. Through the for loop, we generate values from 1 to $limit in the generator function.
Secondly, the generator object can also be paused and resumed, which allows us to control and operate the generator during its execution. By calling the send() method of the generator object, we can send a value to the generator and receive this value via yield within the generator function.
function generator_with_send() { $value1 = yield; // 对$value1进行处理 $value2 = yield; // 对$value2进行处理 yield $result; } $generator = generator_with_send(); $generator->send($value1); $generator->send($value2); $result = $generator->current();
In the above code, we define a generator function generator_with_send(), which internally receives the value and processes it through yield. We can send a value to the generator when calling the send() method of the generator object.
Finally, the generator can also return the final result through the return keyword of the generator syntax, and end the running of the generator by throwing an exception inside the generator object.
function generator_with_return() { // 产生值的逻辑 return $result; } $generator = generator_with_return(); $result = $generator->current();
By using generators, we can efficiently process large amounts of data and speed up code execution. The lazy generation feature and iteration-based processing of the generator are undoubtedly powerful tools for processing large data sets. In actual development, we can flexibly use generators to optimize code performance according to specific needs and scenarios.
Although generators have significant advantages in processing large amounts of data and improving code execution speed, we must also pay attention to the rational use of generators. In some cases, our needs may be better met using other techniques or optimization algorithms. Therefore, we need to weigh the pros and cons of using generators in actual development and choose the most suitable solution.
The above is the detailed content of Generators in PHP7: How to efficiently process large amounts of data and speed up code execution?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
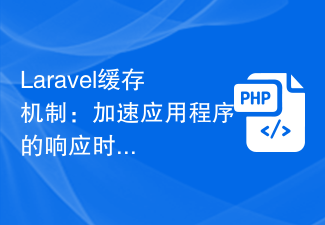
Laravel Caching Mechanism: Accelerate Application Response Time Introduction: In today's Internet era, fast application response time is crucial to user experience and business success. In order to improve the performance and responsiveness of the application, developers need to adopt some strategies. One of them is to use caching mechanism. As a popular PHP framework, Laravel provides a powerful caching mechanism that can help us speed up the response time of our applications. This article will introduce in detail the use of Laravel caching mechanism
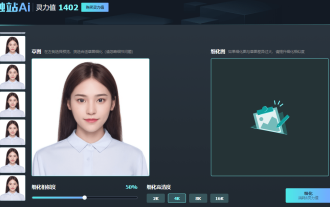
After actual testing, the AI ID photo generator performed well and its powerful functions are amazing. You really don’t need to worry about taking photos anymore! This sentence is rewritten as follows: Use Chuzhan AI software (the copyright and interpretation rights belong to Chuzhan AI and are only used to show the generated effect) sketch mode: Whether in daily work or business office situations, professional image is crucial . A beautiful ID photo can enhance one's professional image. ID photos generated through AI not only meet traditional photo standards, but can also restore a person's unique facial features. AI technology can intelligently identify various details such as facial contours, skin color, lighting, etc., and generate the most suitable ID photo. Whether it is appearance or temperament, it can be perfectly displayed and leave a deep first impression on people. AI generates ID photos with one click.
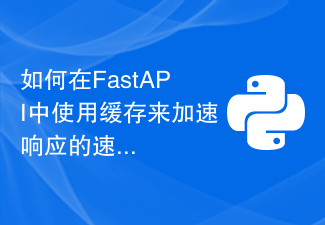
How to use caching in FastAPI to speed up responses Introduction: In modern web development, performance is an important concern. If our application cannot respond to customer requests quickly, it may lead to a decline in user experience or even user churn. Using cache is one of the common methods to improve the performance of web applications. In this article, we will explore how to use caching to speed up the response speed of the FastAPI framework and provide corresponding code examples. 1. What is cache? A cache is a cache that will be accessed frequently
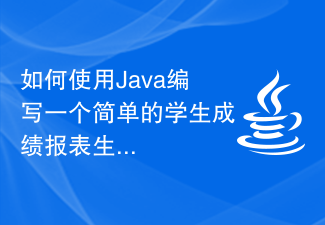
How to write a simple student performance report generator using Java? Student Performance Report Generator is a tool that helps teachers or educators quickly generate student performance reports. This article will introduce how to use Java to write a simple student performance report generator. First, we need to define the student object and student grade object. The student object contains basic information such as the student's name and student number, while the student score object contains information such as the student's subject scores and average grade. The following is the definition of a simple student object: public

How to use Numba to speed up numerical calculations of Python programs Introduction: Python is a very flexible and easy-to-use language when it comes to numerical calculations. However, since Python is an interpreted language, it runs relatively slowly, especially in intensive numerical computing tasks. In order to improve the performance of Python programs, we can use some optimization tools and libraries. One very powerful library is Numba, which can use just-in-time compilation without changing the structure of Python code.
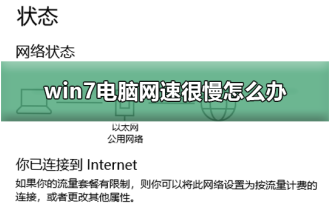
Many friends who use win7 system computers find that the Internet speed is extremely slow when using the computer. What is happening? It may be that there are certain restrictions on the network in your network settings. Today I will teach you how to remove network restrictions and make the network speed extremely fast. Just select the advanced settings and change the value to "20MHz/ 40MHzauto" is enough. Let’s take a look at the specific tutorials. Methods to improve the network speed of win7 computer 1. The editor takes the win7 system as an example to illustrate. Right-click the "Network" icon on the right side of the desktop taskbar and select "Network and Sharing Center" to open it. 2. Click "Change Adapter Settings" in the newly appeared interface, then right-click "Local Area Connection" and select "Properties" to open. 3. In the open "Local

How to turn on hardware acceleration With the development of technology, hardware acceleration has become one of the important means to improve computer performance. By using hardware acceleration, we can speed up the computer's running speed, improve graphics processing capabilities, and make the computer more efficient and stable. So, how to turn on hardware acceleration? This article will introduce it to you in detail. First, we need to clarify the concept of hardware acceleration. Hardware acceleration generally refers to the use of dedicated computer hardware for acceleration processing, rather than through software. Common hardware acceleration includes GPU (graphics processing unit) plus
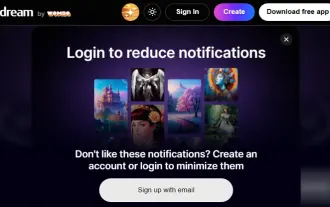
If you are eager to find the top free AI animation art generator, you can end your search. The world of anime art has been captivating audiences for decades with its unique character designs, captivating colors and captivating plots. However, creating anime art requires talent, skill, and a lot of time. However, with the continuous development of artificial intelligence (AI), you can now explore the world of animation art without having to delve into complex technologies with the help of the best free AI animation art generator. This will open up new possibilities for you to unleash your creativity. What is an AI anime art generator? The AI Animation Art Generator utilizes sophisticated algorithms and machine learning techniques to analyze an extensive database of animation works. Through these algorithms, the system learns and identifies different animation styles
