How to handle validation and submission of form data in Vue development
How to handle the verification and submission of form data in Vue development
In Vue development, the verification and submission of form data is a common requirement. This article will introduce how to use Vue to handle the validation and submission of form data, and provide some specific code examples.
- Verification of form data
In Vue, two-way binding of form data can be achieved through the v-model directive. In this way, form data can be obtained and updated in real time for easy verification.
When verifying form data, you can use calculated attributes to monitor changes in form data, and determine whether the form data is legal through some conditional judgments.
For example, the following is a simple form component that contains a username and password input box, and a login button:
<template> <div> <input type="text" v-model="username" placeholder="用户名" /> <input type="password" v-model="password" placeholder="密码" /> <button @click="login">登录</button> </div> </template> <script> export default { data() { return { username: '', password: '', }; }, computed: { isValidForm() { return this.username !== '' && this.password !== ''; }, }, methods: { login() { if (this.isValidForm) { // 表单数据验证通过,可以进行登录操作 // ... } else { // 表单数据验证未通过,给出相应提示 // ... } }, }, }; </script>
In the above code, through the calculated property isValidForm
Monitor changes in form data and determine whether the form data is legal. When neither username
nor password
is empty, the form data is considered valid. In the click event processing function login
of the login button, perform corresponding operations based on the legality of the form data.
Of course, this is just a simple example. In actual development, more complex verification rules may be required and more detailed verification tips may be given. According to specific needs, you can use Vue's instructions, methods and plug-ins in combination to achieve more comprehensive and flexible form data validation.
- Submission of form data
Generally, submitting form data to the back-end server is accomplished by sending an HTTP request. In Vue, you can use the built-in axios
library or other third-party libraries to send HTTP requests.
The following is an example that demonstrates how to use the axios library to send a POST request to submit form data to the server:
import axios from 'axios'; export default { // ... methods: { login() { if (this.isValidForm) { const formData = { username: this.username, password: this.password, }; axios.post('/api/login', formData) .then(response => { // 请求成功处理逻辑 }) .catch(error => { // 请求错误处理逻辑 }); } else { // 表单数据验证未通过,给出相应提示 // ... } }, }, };
In the above code, the form data is first saved into a formData object, and then Use the post method of axios to send a POST request, and pass formData as the request body parameter to the backend server. By calling the then method and the catch method, you can handle the success and failure of the request respectively.
It should be noted that in actual development, it may be necessary to set the request header information, process response data, etc. according to the interface requirements of the back-end server.
To sum up, this article introduces how to handle the verification and submission of form data in Vue development. By rationally using Vue's features and related libraries, you can flexibly and efficiently handle the verification and submission of form data, improving development efficiency and user experience.
The above is the detailed content of How to handle validation and submission of form data in Vue development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
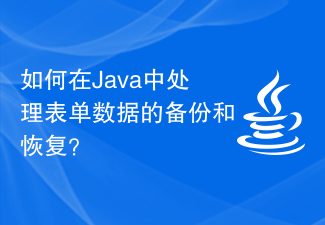
How to handle backup and restore of form data in Java? With the continuous development of technology, using forms for data interaction has become a common practice in web development. During the development process, we may encounter situations where we need to back up and restore form data. This article will introduce how to handle the backup and recovery of form data in Java and provide relevant code examples. Backing up form data During the process of processing form data, we need to back up the form data to a temporary file or database for future restoration. Below is one
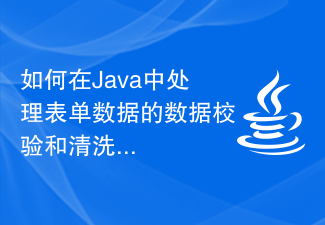
How to handle data validation and cleaning of form data in Java? With the development of web applications, forms have become the main way for users to interact with servers. However, due to the uncertainty of user input data, we need to verify and clean the form data to ensure the validity and security of the data. This article will introduce how to handle data verification and cleaning of form data in Java, and give corresponding code examples. First, we need to use the regular expressions provided by Java (RegularExpres
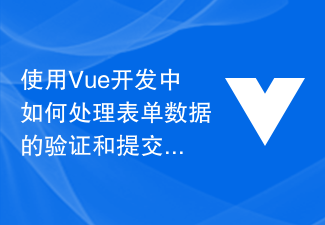
How to handle the verification and submission of form data in Vue development. In Vue development, the verification and submission of form data is a common requirement. This article will introduce how to use Vue to handle the validation and submission of form data, and provide some specific code examples. Verification of form data In Vue, two-way binding of form data can be achieved through the v-model directive. In this way, form data can be obtained and updated in real time for easy verification. When performing form data validation, you can use calculated properties to monitor form data
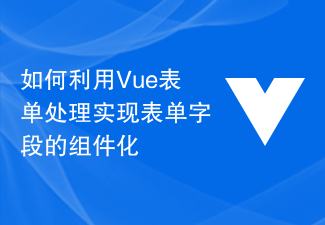
How to use Vue form processing to implement componentization of form fields. In recent years, front-end development technology has developed rapidly. Vue.js, as a lightweight, efficient, and flexible front-end framework, is widely used in front-end development. Vue.js provides a component-based idea that allows us to divide the page into multiple independent and reusable components. In actual development, the form is a component that we often encounter. How to componentize the processing of form fields is a problem that needs to be thought about and solved. In Vue, you can pass
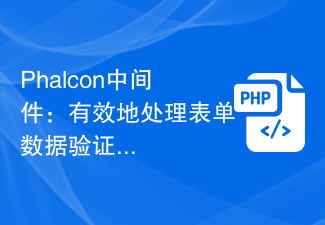
Phalcon middleware: effectively handle form data validation and filtering As web applications become increasingly complex, form data validation and filtering are becoming more and more important. Phalcon middleware provides a simple and efficient way to handle these tasks. Phalcon is an open source web development framework known for its extremely fast performance and efficient scalability. The middleware function is an important feature in the Phalcon framework, which allows developers to process data before or after the request is routed to the controller.
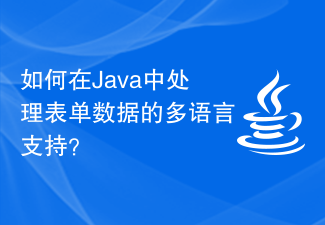
How to handle multi-language support for form data in Java? In today's globalized world, multi-language support is one of the important considerations when developing applications. When working with form data in a web application, it is crucial to ensure that input in different languages can be handled correctly. Java, as a widely used programming language, provides multi-language support for multiple methods to process form data. This article will introduce several common methods and provide corresponding code examples. Using Java's internationalization (i18n) mechanism provided by Java
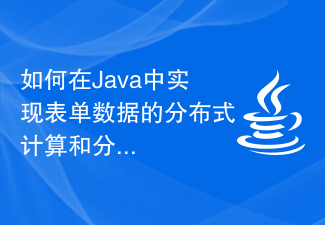
How to implement distributed computing and distributed processing of form data in Java? With the rapid development of the Internet and the increase in the amount of information, the demand for big data calculation and processing is also increasing. Distributed computing and distributed processing have become an effective means to solve large-scale computing and processing problems. In Java, we can use some open source frameworks to implement distributed computing and distributed processing of form data. This article will introduce an implementation method based on Apache Hadoop and Spring Boot. Apac
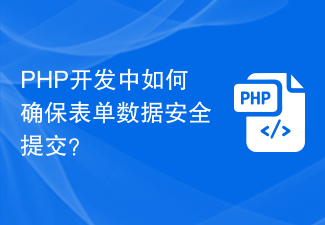
With the popularity of the Internet, the development of web applications has become more and more important. Processing user-submitted form data is particularly important for web applications because it involves user privacy and data security. Especially in PHP development, handling the safe submission of form data is an aspect that must be paid attention to. First of all, in order to ensure the safe submission of form data, the HTTPS protocol should be used to transmit the data. The HTTPS protocol transmits data through encryption, which can prevent data from being stolen or tampered with. You can install it on the website
