


How to implement distributed computing and distributed processing of form data in Java?
How to implement distributed computing and distributed processing of form data in Java?
With the rapid development of the Internet and the increase in the amount of information, the demand for big data calculation and processing is also increasing. Distributed computing and distributed processing have become an effective means to solve large-scale computing and processing problems. In Java, we can use some open source frameworks to implement distributed computing and distributed processing of form data. This article will introduce an implementation method based on Apache Hadoop and Spring Boot.
- Introduction to Apache Hadoop:
Apache Hadoop is an open source, scalable distributed computing framework capable of processing large-scale data sets. It uses a distributed file system (HDFS) to store data and distributes computing through the MapReduce programming model. In Java, we can use the Hadoop MapReduce framework to write distributed computing tasks. - Introduction to Spring Boot:
Spring Boot is a framework for creating independent, production-level Spring applications that simplifies the configuration and deployment of Spring applications. In Java, we can use Spring Boot to build a scheduling and management system for distributed processing tasks.
The following will introduce the steps of how to use Apache Hadoop and Spring Boot to implement distributed computing and distributed processing of form data.
Step 1: Build a Hadoop cluster
First, we need to build a Hadoop cluster for distributed computing and processing. You can refer to Hadoop official documentation or online tutorials to build a cluster. Generally speaking, a Hadoop cluster requires at least three servers, one of which serves as the NameNode (master node) and the rest as DataNode (slave nodes). Ensure the cluster is working properly.
Step 2: Write MapReduce task
Create a Java project and import the Hadoop dependency library. Then write a MapReduce task to process the form data. Specific code examples are as follows:
import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.fs.Path; import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Job; import org.apache.hadoop.mapreduce.Mapper; import org.apache.hadoop.mapreduce.Reducer; import org.apache.hadoop.mapreduce.lib.input.FileInputFormat; import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat; import java.io.IOException; import java.util.StringTokenizer; public class WordCount { public static class TokenizerMapper extends Mapper<Object, Text, Text, IntWritable>{ private final static IntWritable one = new IntWritable(1); private Text word = new Text(); public void map(Object key, Text value, Context context ) throws IOException, InterruptedException { StringTokenizer itr = new StringTokenizer(value.toString()); while (itr.hasMoreTokens()) { word.set(itr.nextToken()); context.write(word, one); } } } public static class IntSumReducer extends Reducer<Text,IntWritable,Text,IntWritable> { private IntWritable result = new IntWritable(); public void reduce(Text key, Iterable<IntWritable> values, Context context ) throws IOException, InterruptedException { int sum = 0; for (IntWritable val : values) { sum += val.get(); } result.set(sum); context.write(key, result); } } public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); Job job = Job.getInstance(conf, "word count"); job.setJarByClass(WordCount.class); job.setMapperClass(TokenizerMapper.class); job.setCombinerClass(IntSumReducer.class); job.setReducerClass(IntSumReducer.class); job.setOutputKeyClass(Text.class); job.setOutputValueClass(IntWritable.class); FileInputFormat.addInputPath(job, new Path(args[0])); FileOutputFormat.setOutputPath(job, new Path(args[1])); System.exit(job.waitForCompletion(true) ? 0 : 1); } }
Step 3: Write a Spring Boot application
Next, we use Spring Boot to write an application for scheduling and managing distributed processing tasks. Create a new Spring Boot project and add Hadoop dependencies. Then write a scheduler and manager to submit and monitor distributed processing tasks, and process the results of the tasks. Specific code examples are as follows:
import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.fs.FileSystem; import org.apache.hadoop.fs.Path; import org.apache.hadoop.mapreduce.Job; import org.springframework.boot.CommandLineRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import java.io.IOException; @SpringBootApplication public class Application implements CommandLineRunner { // Hadoop配置文件路径 private static final String HADOOP_CONF_PATH = "/path/to/hadoop/conf"; // 输入文件路径 private static final String INPUT_PATH = "/path/to/input/file"; // 输出文件路径 private static final String OUTPUT_PATH = "/path/to/output/file"; public static void main(String[] args) { SpringApplication.run(Application.class, args); } @Override public void run(String... args) throws Exception { // 创建Hadoop配置对象 Configuration configuration = new Configuration(); configuration.addResource(new Path(HADOOP_CONF_PATH + "/core-site.xml")); configuration.addResource(new Path(HADOOP_CONF_PATH + "/hdfs-site.xml")); configuration.addResource(new Path(HADOOP_CONF_PATH + "/mapred-site.xml")); // 创建HDFS文件系统对象 FileSystem fs = FileSystem.get(configuration); // 创建Job对象 Job job = Job.getInstance(configuration, "WordCount"); // 设置任务的类路径 job.setJarByClass(Application.class); // 设置输入和输出文件路径 FileInputFormat.addInputPath(job, new Path(INPUT_PATH)); FileOutputFormat.setOutputPath(job, new Path(OUTPUT_PATH)); // 提交任务 job.waitForCompletion(true); // 处理任务的结果 if (job.isSuccessful()) { // 输出处理结果 System.out.println("Job completed successfully."); // 读取输出文件内容 // ... } else { // 输出处理失败信息 System.out.println("Job failed."); } } }
Step 4: Run the code
After properly configuring the related configuration files of Hadoop and Spring Boot, you can start the Spring Boot application and observe the execution of the task. If everything goes well, you should be able to see the execution results of the distributed computing tasks.
Through the above steps, we successfully implemented distributed computing and distributed processing of form data using Apache Hadoop and Spring Boot. The code can be adjusted and optimized according to actual needs to adapt to different application scenarios. Hope this article is helpful to you.
The above is the detailed content of How to implement distributed computing and distributed processing of form data in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
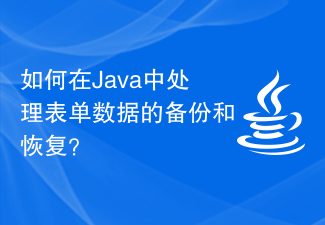
How to handle backup and restore of form data in Java? With the continuous development of technology, using forms for data interaction has become a common practice in web development. During the development process, we may encounter situations where we need to back up and restore form data. This article will introduce how to handle the backup and recovery of form data in Java and provide relevant code examples. Backing up form data During the process of processing form data, we need to back up the form data to a temporary file or database for future restoration. Below is one
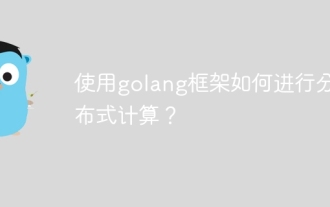
A step-by-step guide to implementing distributed computing with GoLang: Install a distributed computing framework (such as Celery or Luigi) Create a GoLang function that encapsulates task logic Define a task queue Submit a task to the queue Set up a task handler function
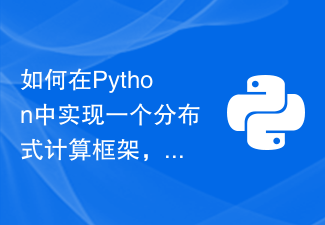
Title: Implementation of distributed computing framework and task scheduling and result collection mechanism in Python Abstract: Distributed computing is a method that effectively utilizes the resources of multiple computers to accelerate task processing. This article will introduce how to use Python to implement a simple distributed computing framework, including the mechanisms and strategies of task scheduling and result collection, and provide relevant code examples. Text: 1. Overview of distributed computing framework Distributed computing is a method that uses multiple computers to jointly process tasks to achieve the purpose of accelerating computing. In a distributed computing framework,
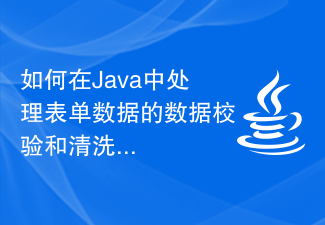
How to handle data validation and cleaning of form data in Java? With the development of web applications, forms have become the main way for users to interact with servers. However, due to the uncertainty of user input data, we need to verify and clean the form data to ensure the validity and security of the data. This article will introduce how to handle data verification and cleaning of form data in Java, and give corresponding code examples. First, we need to use the regular expressions provided by Java (RegularExpres
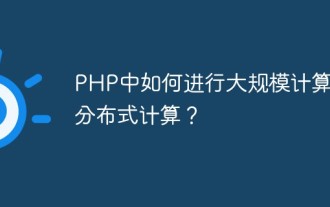
As the Internet continues to develop, web applications are becoming larger and larger and need to handle more data and more requests. In order to meet these needs, computing large-scale data and distributed computing have become an essential requirement. As an efficient, easy-to-use, and flexible language, PHP is also constantly developing and improving its own operating methods, and has gradually become an important tool for computing large-scale data and distributed computing. This article will introduce the concepts and implementation methods of large-scale computing and distributed computing in PHP. We will discuss how to use PHP
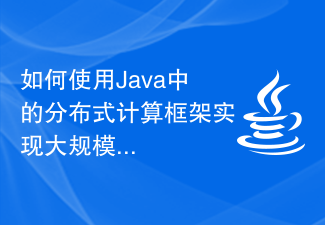
How to implement large-scale data processing using distributed computing framework in Java? Introduction: With the advent of the big data era, we need to process increasingly large amounts of data. Traditional single-machine computing can no longer meet this demand, so distributed computing has become an effective means to solve large-scale data processing problems. As a widely used programming language, Java provides a variety of distributed computing frameworks, such as Hadoop, Spark, etc. This article will introduce how to use the distributed computing framework in Java to achieve large-scale data processing
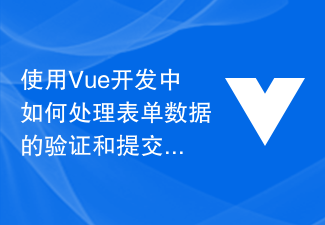
How to handle the verification and submission of form data in Vue development. In Vue development, the verification and submission of form data is a common requirement. This article will introduce how to use Vue to handle the validation and submission of form data, and provide some specific code examples. Verification of form data In Vue, two-way binding of form data can be achieved through the v-model directive. In this way, form data can be obtained and updated in real time for easy verification. When performing form data validation, you can use calculated properties to monitor form data
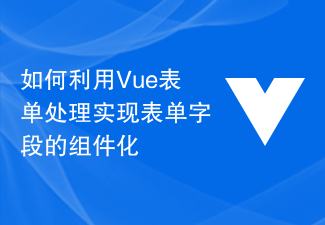
How to use Vue form processing to implement componentization of form fields. In recent years, front-end development technology has developed rapidly. Vue.js, as a lightweight, efficient, and flexible front-end framework, is widely used in front-end development. Vue.js provides a component-based idea that allows us to divide the page into multiple independent and reusable components. In actual development, the form is a component that we often encounter. How to componentize the processing of form fields is a problem that needs to be thought about and solved. In Vue, you can pass
