


The clever combination of Golang asynchronous processing and Go WaitGroup
The clever combination of Golang asynchronous processing and Go WaitGroup
Introduction:
In software development, asynchronous processing is a common technical means that can improve the program Performance and responsiveness. In the Go language, asynchronous processing coroutines can be managed more concisely by using WaitGroup. This article will introduce the basic concepts of Golang asynchronous processing and explain in detail how to cleverly combine Go WaitGroup to implement asynchronous processing.
1. The concept of asynchronous processing
Asynchronous processing means that during the execution of the program, there is no need to wait for an operation to be completed, but to continue executing the next piece of code. Common asynchronous processing methods include multi-threading, event-driven, callback functions, etc. In Go language, you can use goroutine to achieve concurrency to achieve asynchronous processing.
2. Goroutine in Golang
Goroutine is a lightweight thread in the Go language that can be created and destroyed at a very low cost. Concurrency can be used through goroutine to improve program performance and concurrency capabilities. To use goroutine, you only need to add the go keyword before the function or method. For example:
func main() { go myfunction() // 其他代码 } func myfunction() { // 异步处理的代码逻辑 }
In the above code, myfunction will be called as an independent goroutine and executed asynchronously.
3. WaitGroup in Golang
WaitGroup is a synchronization primitive in the Go language, which can be used to wait for the completion of the execution of a group of goroutines. The counter inside WaitGroup can be used to control whether all goroutines have been executed. You can use the Add method to increase the counter value, the Done method to decrease the counter value, and the Wait method to block and wait for the counter to return to zero.
When using WaitGroup, the general process is as follows:
- Create WaitGroup object
- When starting goroutine, use the Add method to increase the value of the counter
- After the goroutine execution is completed, use the Done method to reduce the counter value
- In the main goroutine, use the Wait method to block and wait for the counter to return to zero
- After all goroutine execution is completed, continue to execute subsequent code
The specific code is as follows:
import ( "fmt" "sync" ) var wg sync.WaitGroup func main() { for i := 0; i < 10; i++ { wg.Add(1) go myfunction(i) } wg.Wait() // 所有goroutine执行完成后,继续执行后续代码 fmt.Println("all goroutines completed") } func myfunction(id int) { defer wg.Done() // 异步处理的代码逻辑 fmt.Println("goroutine", id, "completed") }
In the above code, we created a loop containing 10 goroutines, and executed asynchronous processing code logic in each goroutine. After each goroutine is executed, the wg.Done() method is called to decrement the counter value. In the main goroutine, we use the wg.Wait() method to wait for the counter to reach zero.
By using WaitGroup, we can easily manage the concurrent execution of multiple goroutines and continue to execute subsequent code after all goroutines are executed.
4. Clever application of combining asynchronous processing and WaitGroup
When the number of tasks we need to process is unknown, we can combine asynchronous processing and WaitGroup to achieve more flexible concurrent processing. A sample code is given below:
import ( "fmt" "sync" "time" ) var wg sync.WaitGroup func main() { tasks := []string{"task1", "task2", "task3", "task4", "task5"} for _, task := range tasks { wg.Add(1) go processTask(task) } wg.Wait() fmt.Println("all tasks completed") } func processTask(task string) { defer wg.Done() // 模拟耗时的任务处理 time.Sleep(time.Second * 2) fmt.Println("task", task, "completed") }
In the above code, we represent the list of pending tasks through a string slice. In the loop, we use the Add method to increase the counter value and start a goroutine for each task to process. After the processing is completed, we call the Done method to decrease the counter value. Finally, use the Wait method to wait for the execution of all tasks to complete.
In this way, we can conveniently handle an unknown number of tasks and continue executing subsequent code after all tasks are completed.
Conclusion:
This article introduces the concept of asynchronous processing in Golang, and how to cleverly use Go WaitGroup to manage asynchronous processing goroutines. Through asynchronous processing, we can improve the performance and responsiveness of the program, and using WaitGroup can help us manage and control the concurrent execution of asynchronous processing more conveniently. By mastering this technique, you can effectively improve the development efficiency of Golang programs.
The above is the detailed content of The clever combination of Golang asynchronous processing and Go WaitGroup. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
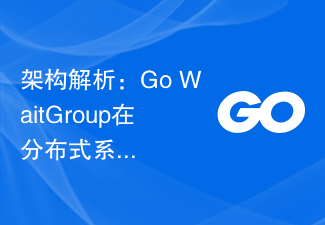
Architecture Analysis: Application of GoWaitGroup in Distributed Systems Introduction: In modern distributed systems, in order to improve the performance and throughput of the system, it is often necessary to use concurrent programming technology to handle a large number of tasks. As a powerful concurrent programming language, Go language is widely used in the development of distributed systems. Among them, WaitGroup is an important concurrency primitive provided by the Go language, which is used to wait for the completion of a group of concurrent tasks. This article will discuss GoWaitGr from the perspective of distributed systems

Golang Concurrent Programming: Using GoWaitGroup to Implement Task Scheduler Introduction In Golang, implementing concurrent programming can greatly improve the performance and efficiency of the program. The task scheduler is a very important part of concurrent programming. It can be used to schedule the execution sequence of concurrent tasks and the completion of synchronized tasks. This article will introduce how to use WaitGroup in Golang to implement a simple task scheduler and give specific code examples. Introduction to WaitGroupWaitGrou
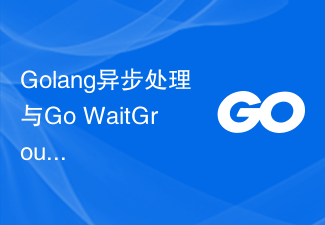
Introduction to the clever combination of Golang asynchronous processing and GoWaitGroup: In software development, asynchronous processing is a common technical means that can improve program performance and responsiveness. In the Go language, asynchronous processing coroutines can be managed more concisely by using WaitGroup. This article will introduce the basic concepts of Golang asynchronous processing and explain in detail how to cleverly combine GoWaitGroup to achieve asynchronous processing. 1. The concept of asynchronous processing Asynchronous processing refers to the process of program execution
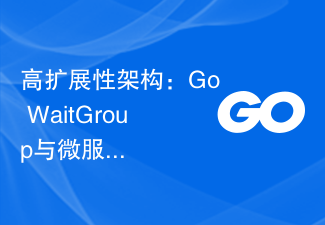
Highly scalable architecture: Seamless integration of GoWaitGroup and microservices In today's fast-paced Internet era, how to build a highly scalable architecture has become an important challenge for software developers. With the rise of microservice architecture, Go language, as an efficient and reliable programming language, is widely used to build high-performance distributed systems. The WaitGroup function in the Go language provides convenience for parallel processing. This article will focus on how to seamlessly connect GoWaitGroup with microservices to implement
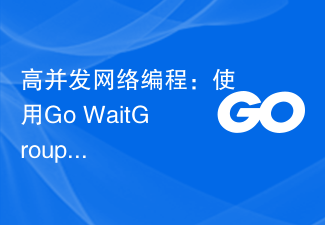
High-concurrency network programming: Using GoWaitGroup to implement concurrent servers Preface: With the development of network applications, high-concurrency servers have become an indispensable part of the Internet field. For servers, handling large numbers of concurrent requests is an important challenge. This article will introduce how to use WaitGroup of Go language to implement a high-concurrency server and provide specific code examples. 1. Introduction to Go language Go language is an open source programming language developed by Google. It combines the advantages of statically typed languages.
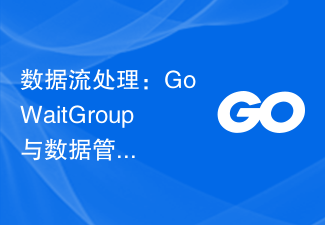
Data Flow Processing: Efficient Combination of GoWaitGroup and Data Pipeline Abstract: Data flow processing is a common task in modern computer application development. It involves processing large amounts of data and is required to be completed in the shortest possible time. As an efficient concurrent programming language, Go language provides some powerful tools to handle data flows. Among them, WaitGroup and data pipeline are two commonly used modules. This article will introduce how to use an efficient combination of WaitGroup and data pipeline to process data
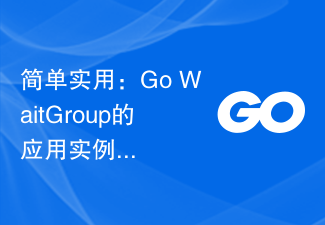
Simple and practical: Sharing application examples of GoWaitGroup Introduction: Go language is a concurrent programming language with many built-in tools and features for concurrent processing. One of them is sync.WaitGroup, which provides an elegant and simple way to wait for the completion of a group of concurrent tasks. This article will share a specific application example showing how to use WaitGroup to speed up the execution of concurrent tasks. What is WaitGroup? sync.WaitGroup is a Go language standard
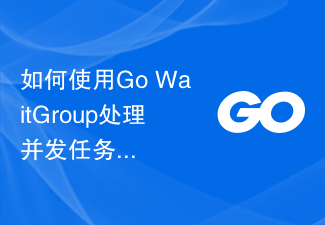
How to use GoWaitGroup to handle concurrent tasks In the Go language, we can handle concurrent tasks by using sync.WaitGroup. sync.WaitGroup can provide a concise and effective way to coordinate the execution of coroutines when handling concurrent tasks. sync.WaitGroup is a useful tool and the preferred method for handling concurrent tasks when we don't know how many coroutines need to wait. It allows us to ensure that before all tasks are completed,
