


High-concurrency network programming: Use Go WaitGroup to implement concurrent servers
High-concurrency network programming: Using Go WaitGroup to implement concurrent servers
Foreword:
With the development of network applications, high-concurrency servers have become an indispensable part of the Internet field. A missing part. For servers, handling large numbers of concurrent requests is an important challenge. This article will introduce how to use WaitGroup of Go language to implement a high-concurrency server and provide specific code examples.
1. Introduction to Go language
Go language is an open source programming language developed by Google. It combines the performance advantages of statically typed languages and the development efficiency of dynamically typed languages. It is suitable for building high-concurrency programs. web application. The Go language has built-in support for concurrent programming, and concurrent operations can be easily implemented through the use of goroutines and channels.
2. Concurrent server architecture design
When designing a high-concurrency server, there are several key factors to consider:
- Asynchronous processing: The server should be able to process multiple servers at the same time Connection, if one connection performs slowly, it will not affect the processing of other connections.
- Resource allocation: The server must allocate resources reasonably to ensure that each connection can obtain sufficient resources.
- Data sharing: The server should be able to correctly handle shared data to prevent data competition and conflicts.
- Request queue: The server needs a request queue to buffer pending requests so that the server can process the requests at its own pace.
3. Use WaitGroup to implement high-concurrency server
In Go language, you can use WaitGroup in the sync package to implement high-concurrency operations. Simply put, WaitGroup allows us to wait for the completion of a group of concurrent operations. The following are the detailed steps to use WaitGroup to implement a high-concurrency server:
-
Introduce the required packages:
import ( "net" "log" "sync" )
Copy after login Define a request processing function:
func handleRequest(conn net.Conn, wg *sync.WaitGroup) { defer wg.Done() // 处理请求逻辑 }
Copy after loginDefine the server main function:
func main() { listener, err := net.Listen("tcp", ":8080") if err != nil { log.Fatal(err) } defer listener.Close() var wg sync.WaitGroup for { conn, err := listener.Accept() if err != nil { log.Fatal(err) } wg.Add(1) go handleRequest(conn, &wg) } wg.Wait() }
Copy after login
In the above code, we create a WaitGroup instancewg
, Use wg.Wait()
in the main function to wait for all goroutines processing requests to complete. In the handleRequest
function, we handle the request for each connection and use wg.Done()
after the function is completed to notify the WaitGroup that the goroutine has completed.
4. Summary
By using WaitGroup of Go language, we can easily implement a high-concurrency server. WaitGroup allows us to wait for the completion of a group of concurrent operations, giving us greater control over concurrency processing. I hope the sample code in this article will help you understand how to implement a high-concurrency server. At the same time, everyone is also encouraged to further learn the relevant knowledge of concurrent programming to cope with increasingly complex network application requirements.
The above is the detailed content of High-concurrency network programming: Use Go WaitGroup to implement concurrent servers. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
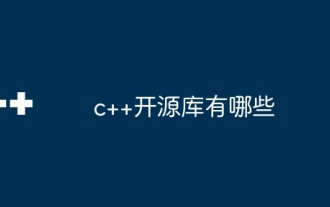
C++ provides a rich set of open source libraries covering the following functions: data structures and algorithms (Standard Template Library) multi-threading, regular expressions (Boost) linear algebra (Eigen) graphical user interface (Qt) computer vision (OpenCV) machine learning (TensorFlow) Encryption (OpenSSL) Data compression (zlib) Network programming (libcurl) Database management (sqlite3)

The C++ standard library provides functions to handle DNS queries in network programming: gethostbyname(): Find host information based on the host name. gethostbyaddr(): Find host information based on IP address. dns_lookup(): Asynchronously resolves DNS.

Commonly used protocols in Java network programming include: TCP/IP: used for reliable data transmission and connection management. HTTP: used for web data transmission. HTTPS: A secure version of HTTP that uses encryption to transmit data. UDP: For fast but unstable data transfer. JDBC: used to interact with relational databases.
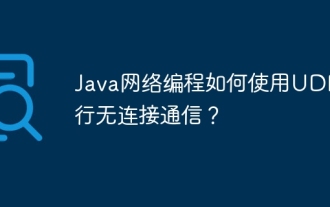
UDP (User Datagram Protocol) is a lightweight connectionless network protocol commonly used in time-sensitive applications. It allows applications to send and receive data without establishing a TCP connection. Sample Java code can be used to create a UDP server and client, with the server listening for incoming datagrams and responding, and the client sending messages and receiving responses. This code can be used to build real-world use cases such as chat applications or data collection systems.
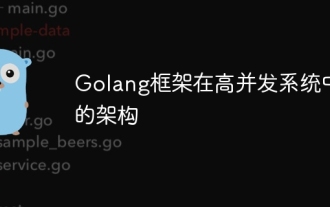
For high-concurrency systems, the Go framework provides architectural modes such as pipeline mode, Goroutine pool mode, and message queue mode. In practical cases, high-concurrency websites use Nginx proxy, Golang gateway, Goroutine pool and database to handle a large number of concurrent requests. The code example shows the implementation of a Goroutine pool for handling incoming requests. By choosing appropriate architectural patterns and implementations, the Go framework can build scalable and highly concurrent systems.
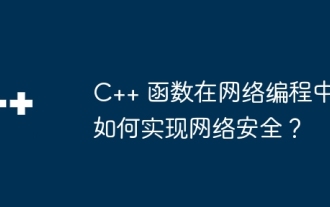
C++ functions can achieve network security in network programming. Methods include: 1. Using encryption algorithms (openssl) to encrypt communication; 2. Using digital signatures (cryptopp) to verify data integrity and sender identity; 3. Defending against cross-site scripting attacks ( htmlcxx) to filter and sanitize user input.
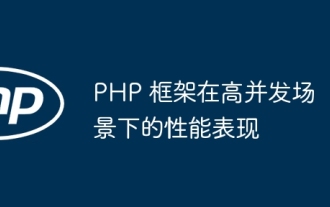
In high-concurrency scenarios, according to benchmark tests, the performance of the PHP framework is: Phalcon (RPS2200), Laravel (RPS1800), CodeIgniter (RPS2000), and Symfony (RPS1500). Actual cases show that the Phalcon framework achieved 3,000 orders per second during the Double Eleven event on the e-commerce website.
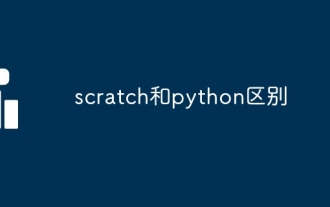
The differences between Scratch and Python are: Target Audience: Scratch is aimed at beginners and educational settings, while Python is aimed at intermediate to advanced programmers. Syntax: Scratch uses a drag-and-drop building block interface, while Python uses a text syntax. Features: Scratch focuses on ease of use and visual programming, while Python offers more advanced features and extensibility.
