


Teach you how to use Python programming to realize the docking of Baidu image recognition interface and realize the image recognition function.
Teach you how to use Python programming to realize the docking of Baidu image recognition interface and realize the image recognition function
In the field of computer vision, image recognition technology is a very important one technology. Baidu provides a powerful image recognition interface through which we can easily implement image classification, labeling, face recognition and other functions. This article will teach you how to use the Python programming language to realize the image recognition function by connecting to the Baidu image recognition interface.
First, we need to create an application on the Baidu Developer Platform and obtain the API Key and Secret Key of the application. Only in this way can we use Baidu image recognition interface.
Next, we need to install the Python request library requests and the Python SDK library aip of Baidu image recognition. Enter the following commands on the command line to install these two libraries:
pip install requests pip install baidu-aip
After the installation is complete, we can import these two libraries into the Python code and start writing image recognition code.
First, we need to import the requests library and aip library, as well as some parameters required to call Baidu image recognition API:
import requests from aip import AipImageClassify # 设置百度图像识别API的参数 APP_ID = 'your_app_id' API_KEY = 'your_api_key' SECRET_KEY = 'your_secret_key' # 创建一个AipImageClassify的实例 client = AipImageClassify(APP_ID, API_KEY, SECRET_KEY)
In the above code, we use our own APP_ID, API_KEY and SECRET_KEY, these information need to be replaced according to the actual situation.
Next, we can write a function to realize the image recognition function by calling Baidu image recognition API:
def image_recognition(image_path): # 读取图像的二进制数据 with open(image_path, 'rb') as f: image_data = f.read() # 调用百度图像识别API对图像进行识别 result = client.advancedGeneral(image_data) # 输出识别结果 if 'error_code' in result: print('识别失败:', result['error_msg']) else: for item in result['result']: print('标签:', item['keyword']) print('可信度:', item['score'])
In the above code, we first read the binary data of the image , and calls the advancedGeneral
method of Baidu Image Recognition API, which can return the image recognition result. Finally, we output the label and credibility of the image by looping through the results.
Finally, we can call the function written above to implement the image recognition function:
if __name__ == '__main__': # 要识别的图像路径 image_path = 'path/to/your/image.jpg' # 调用图像识别函数 image_recognition(image_path)
In the above code, we first specify the image path to be recognized, and then call the image identification function. Just replace the image path to be recognized with your own image path.
Through the above steps, we can use the Python programming language to connect to the Baidu image recognition interface to realize the image recognition function. In this way, we can easily perform operations such as classifying images, labeling them, and identifying faces. I hope this article can help readers who are interested in image recognition.
The above is the detailed content of Teach you how to use Python programming to realize the docking of Baidu image recognition interface and realize the image recognition function.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
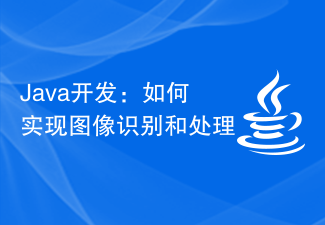
Java Development: A Practical Guide to Image Recognition and Processing Abstract: With the rapid development of computer vision and artificial intelligence, image recognition and processing play an important role in various fields. This article will introduce how to use Java language to implement image recognition and processing, and provide specific code examples. 1. Basic principles of image recognition Image recognition refers to the use of computer technology to analyze and understand images to identify objects, features or content in the image. Before performing image recognition, we need to understand some basic image processing techniques, as shown in the figure
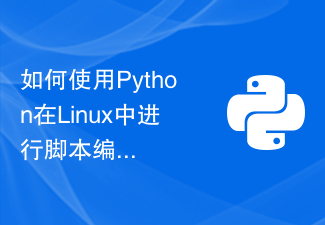
How to use Python to write and execute scripts in Linux In the Linux operating system, we can use Python to write and execute various scripts. Python is a concise and powerful programming language that provides a wealth of libraries and tools to make scripting easier and more efficient. Below we will introduce the basic steps of how to use Python for script writing and execution in Linux, and provide some specific code examples to help you better understand and use it. Install Python
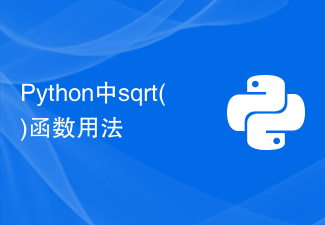
Usage and code examples of the sqrt() function in Python 1. Function and introduction of the sqrt() function In Python programming, the sqrt() function is a function in the math module, and its function is to calculate the square root of a number. The square root means that a number multiplied by itself equals the square of the number, that is, x*x=n, then x is the square root of n. The sqrt() function can be used in the program to calculate the square root. 2. How to use the sqrt() function in Python, sq
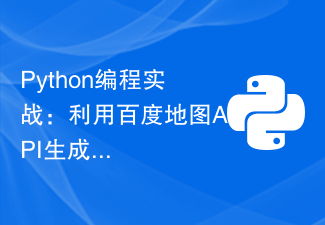
Python programming practice: How to use Baidu Map API to generate static map functions Introduction: In modern society, maps have become an indispensable part of people's lives. When working with maps, we often need to obtain a static map of a specific area for display on a web page, mobile app, or report. This article will introduce how to use the Python programming language and Baidu Map API to generate static maps, and provide relevant code examples. 1. Preparation work To realize the function of generating static maps using Baidu Map API, I
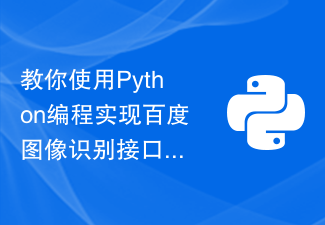
Teach you to use Python programming to implement the docking of Baidu's image recognition interface and realize the image recognition function. In the field of computer vision, image recognition technology is a very important technology. Baidu provides a powerful image recognition interface through which we can easily implement image classification, labeling, face recognition and other functions. This article will teach you how to use the Python programming language to realize the image recognition function by connecting to the Baidu image recognition interface. First, we need to create an application on Baidu Developer Platform and obtain
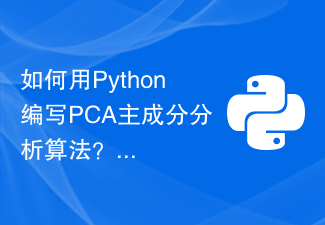
How to write PCA principal component analysis algorithm in Python? PCA (Principal Component Analysis) is a commonly used unsupervised learning algorithm used to reduce the dimensionality of data to better understand and analyze data. In this article, we will learn how to write the PCA principal component analysis algorithm using Python and provide specific code examples. The steps of PCA are as follows: Standardize the data: Zero the mean of each feature of the data and adjust the variance to the same range to ensure
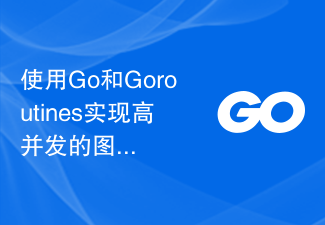
Using Go and Goroutines to implement a highly concurrent image recognition system Introduction: In today's digital world, image recognition has become an important technology. Through image recognition, we can convert information such as objects, faces, scenes, etc. in images into digital data. However, for recognition of large-scale image data, speed often becomes a challenge. In order to solve this problem, this article will introduce how to use Go language and Goroutines to implement a high-concurrency image recognition system. Background: Go language
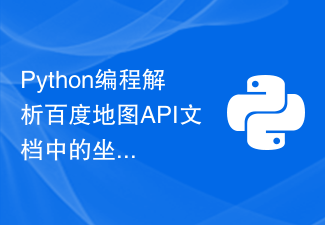
Python programming to analyze the coordinate conversion function in Baidu Map API document Introduction: With the rapid development of the Internet, the map positioning function has become an indispensable part of modern people's lives. As one of the most popular map services in China, Baidu Maps provides a series of APIs for developers to use. This article will use Python programming to analyze the coordinate conversion function in Baidu Map API documentation and give corresponding code examples. 1. Introduction In development, we sometimes involve coordinate conversion issues. Baidu Map AP
