


C++ concurrent programming: How to perform thread-safe design of concurrent data structures?
Thread-safe concurrent data structure design: Implementation method: atomic type and mutex lock atomic type: ensure that multiple accesses are indivisible and ensure data consistency. Mutex lock: restricts access to shared data by one thread at a time to prevent concurrent data corruption. Example: Thread-Safe Queue demonstrates a thread-safe data structure implemented using a mutex lock.
C Concurrent Programming: Thread-safe concurrent data structure design
Understanding thread safety
Thread safety means that the data structure can be used by multiple Threads access concurrently without data corruption or program crashes. In C concurrent programming, achieving thread safety is crucial.
Atomic types and mutex locks
Atomic types:
Atomic types ensure that multiple accesses to the underlying data are indivisible to guarantee consistency . For example, std::atomic<int></int>
.
Mutex lock:
Mutex lock allows one thread to access shared data at a time, thereby preventing data corruption caused by concurrent access. Use std::mutex
.
Example: Thread-Safe Queue
The following is a simple thread-safe queue implemented using a mutex:
#include <iostream> #include <mutex> #include <queue> class ThreadSafeQueue { private: std::queue<int> data; std::mutex mtx; public: void push(int value) { std::lock_guard<std::mutex> lock(mtx); data.push(value); } int pop() { std::lock_guard<std::mutex> lock(mtx); if (data.empty()) throw std::runtime_error("Queue is empty"); int value = data.front(); data.pop(); return value; } bool empty() { std::lock_guard<std::mutex> lock(mtx); return data.empty(); } }; int main() { ThreadSafeQueue queue; std::thread t1([&queue] { for (int i = 0; i < 1000; ++i) { std::lock_guard<std::mutex> lock(queue.mtx); queue.push(i); } }); std::thread t2([&queue] { while (!queue.empty()) { std::lock_guard<std::mutex> lock(queue.mtx); std::cout << "Thread 2 popped: " << queue.pop() << std::endl; } }); t1.join(); t2.join(); return 0; }
In this example:
-
std::mutex
Used to protect concurrent access to queue data. -
std::lock_guard
Used to lock the mutex when entering the critical part of the queue and unlock it when leaving. - Multiple threads can safely push and pop data to the queue concurrently.
Conclusion
Implementing thread-safe concurrent data structures is a crucial aspect of concurrent programming in C. By using mechanisms such as atomic types and mutex locks, we can ensure data consistency and prevent data corruption or program crashes caused by concurrent access.
The above is the detailed content of C++ concurrent programming: How to perform thread-safe design of concurrent data structures?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
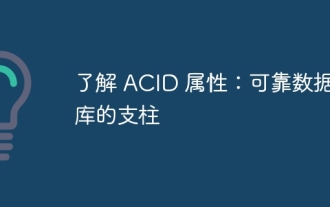
Detailed explanation of database ACID attributes ACID attributes are a set of rules to ensure the reliability and consistency of database transactions. They define how database systems handle transactions, and ensure data integrity and accuracy even in case of system crashes, power interruptions, or multiple users concurrent access. ACID Attribute Overview Atomicity: A transaction is regarded as an indivisible unit. Any part fails, the entire transaction is rolled back, and the database does not retain any changes. For example, if a bank transfer is deducted from one account but not increased to another, the entire operation is revoked. begintransaction; updateaccountssetbalance=balance-100wh
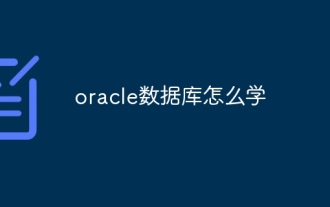
There are no shortcuts to learning Oracle databases. You need to understand database concepts, master SQL skills, and continuously improve through practice. First of all, we need to understand the storage and management mechanism of the database, master the basic concepts such as tables, rows, and columns, and constraints such as primary keys and foreign keys. Then, through practice, install the Oracle database, start practicing with simple SELECT statements, and gradually master various SQL statements and syntax. After that, you can learn advanced features such as PL/SQL, optimize SQL statements, and design an efficient database architecture to improve database efficiency and security.
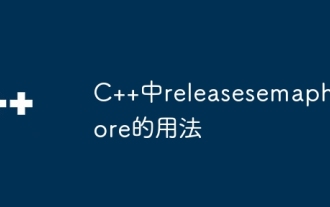
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
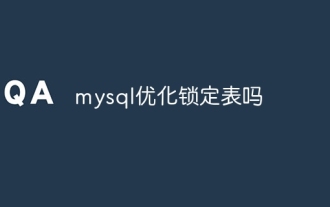
MySQL uses shared locks and exclusive locks to manage concurrency, providing three lock types: table locks, row locks and page locks. Row locks can improve concurrency, and use the FOR UPDATE statement to add exclusive locks to rows. Pessimistic locks assume conflicts, and optimistic locks judge the data through the version number. Common lock table problems manifest as slow querying, use the SHOW PROCESSLIST command to view the queries held by the lock. Optimization measures include selecting appropriate indexes, reducing transaction scope, batch operations, and optimizing SQL statements.
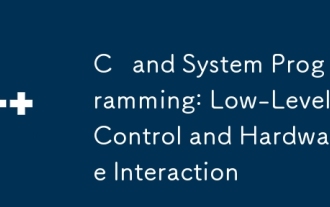
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
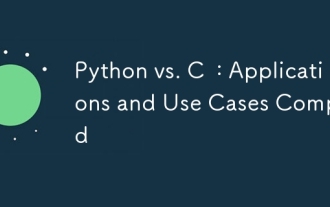
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
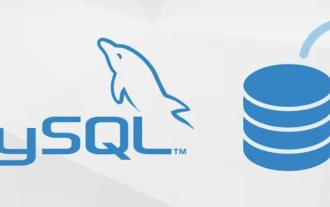
MySQL download prompts a disk write error. The solution is as follows: 1. Check whether the disk space is insufficient, clean up the space or replace a larger disk; 2. Use disk detection tools (such as chkdsk or fsck) to check and fix disk errors, and replace the hard disk if necessary; 3. Check the target directory permissions to ensure that the user account has write permissions; 4. Change the download tool or network environment, and use the download manager to restore interrupted download; 5. Temporarily close the anti-virus software or firewall, and re-enable it after the download is completed. By systematically troubleshooting these aspects, the problem can be solved.
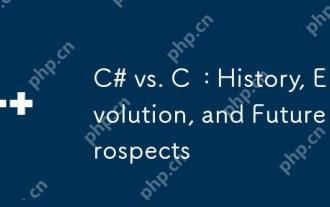
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
