


Java Concurrent Collections Practical Guide: Easily Develop High Concurrency Systems
#The book "Java Concurrent Collections Practical Guide: Easily Developing High Concurrency Systems" recommended by php editor Xigua is a practical guide for Java developers to apply concurrent collections in actual projects. Through this book, readers can learn how to use the concurrent collection classes provided by Java to easily develop high-concurrency systems and improve the performance and stability of the system. This book is a rare practical guide for developers who want to understand concurrency programming in depth and improve the concurrency processing capabilities of the system.
Java Concurrent Collections are part of the Java Collections Framework and are designed for multi-threadedprogramming. They provide a series of threadsafe collection classes that can be used to store and manage shared data. The main advantages of Java concurrent collections include:
-
Thread safety: Java concurrent collections are carefully designed to ensure the consistency and integrity of data in a multi-threaded environment.
-
Efficiency: Java concurrent collections have been optimized to provide high-performance concurrent access performance.
-
Ease of use: Java concurrent collections provide an intuitive and simple api that is easy to use and understand.
2. Types of Java concurrent collections
Java concurrent collections mainly include the following types:
-
List: A collection used to store an ordered list of elements, supporting fast random access.
-
Set: A collection used to store unique elements, supporting fast search and deletion.
-
Map: Used to store a collection of key-value pairs, supporting efficient search for values based on keys.
-
Queue: A FIFO (first in first out) or LIFO (last in first out) queue for storing elements.
-
BlockingQueue: A blocking queue used to store elements, supporting thread-safe blocking enqueue and dequeue operations.
3. Usage of Java concurrent collections
The following are some examples to demonstrate the usage of Java concurrent collections:
- Use ConcurrentHashMap Store shared data
ConcurrentHashMap<String, Object> sharedDataMap = new ConcurrentHashMap<>(); // 线程 1 sharedDataMap.put("key1", "value1"); // 线程 2 Object value2 = sharedDataMap.get("key1");
- Use CopyOnWriteArrayList to implement thread-safe lists
CopyOnWriteArrayList<String> threadSafeList = new CopyOnWriteArrayList<>(); // 线程 1 threadSafeList.add("element1"); // 线程 2 String element1 = threadSafeList.get(0);
- Use BlockingQueue to implement the producer-consumer model
BlockingQueue<Integer> blockingQueue = new ArrayBlockingQueue<>(10); // 生产者线程 blockingQueue.put(1); // 消费者线程 Integer value = blockingQueue.take();
4. Frequently Asked Questions
- How to choose a suitable Java concurrent collection?
Choosing the appropriate Java concurrent collection depends on the specific needs of your application. In general, if you need a thread-safe list, use CopyOnWriteArrayList. If you need a thread-safe hash table, you can use ConcurrentHashMap. If you need a thread-safe queue, you can use BlockingQueue.
- Do Java concurrent collections affect performance?
Java concurrent collections are optimized to provide high-performance concurrent access performance. However, due to the presence of thread safety mechanisms, the performance of Java concurrent collections may be slightly lower than non-thread-safe collections.
- Do Java Concurrent Collections support all Java collection operations?
Java concurrent collections do not support all Java collection operations. For example, Java concurrent collections do not support the iterator remove operation. If you need to support all Java collection operations, you can use a non-blocking concurrent collection such as ConcurrentLinkedQueue or ConcurrentSkipListSet.
5. Summary
Java concurrent collections are a magic weapon for handling concurrent programming in the Java language. They provide efficient, safe, and easy-to-use collection classes to help developers easily build high-concurrency systems. Through Learning of this article, you should have mastered the characteristics, usage and some common examples of Java concurrent collections. Now you can confidently use Java concurrent collections in your projects and enjoy the convenience and high performance they bring.
The above is the detailed content of Java Concurrent Collections Practical Guide: Easily Develop High Concurrency Systems. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
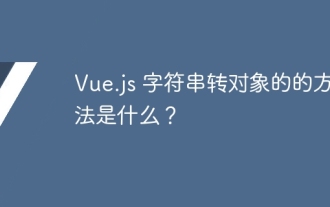
Using JSON.parse() string to object is the safest and most efficient: make sure that strings comply with JSON specifications and avoid common errors. Use try...catch to handle exceptions to improve code robustness. Avoid using the eval() method, which has security risks. For huge JSON strings, chunked parsing or asynchronous parsing can be considered for optimizing performance.
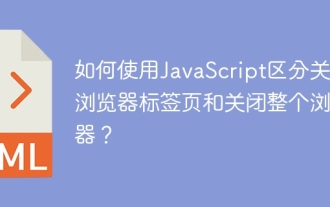
How to distinguish between closing tabs and closing entire browser using JavaScript on your browser? During the daily use of the browser, users may...
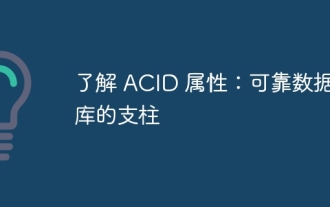
Detailed explanation of database ACID attributes ACID attributes are a set of rules to ensure the reliability and consistency of database transactions. They define how database systems handle transactions, and ensure data integrity and accuracy even in case of system crashes, power interruptions, or multiple users concurrent access. ACID Attribute Overview Atomicity: A transaction is regarded as an indivisible unit. Any part fails, the entire transaction is rolled back, and the database does not retain any changes. For example, if a bank transfer is deducted from one account but not increased to another, the entire operation is revoked. begintransaction; updateaccountssetbalance=balance-100wh
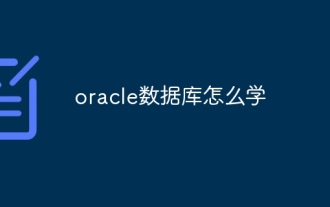
There are no shortcuts to learning Oracle databases. You need to understand database concepts, master SQL skills, and continuously improve through practice. First of all, we need to understand the storage and management mechanism of the database, master the basic concepts such as tables, rows, and columns, and constraints such as primary keys and foreign keys. Then, through practice, install the Oracle database, start practicing with simple SELECT statements, and gradually master various SQL statements and syntax. After that, you can learn advanced features such as PL/SQL, optimize SQL statements, and design an efficient database architecture to improve database efficiency and security.
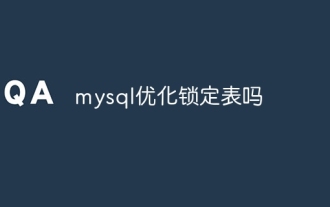
MySQL uses shared locks and exclusive locks to manage concurrency, providing three lock types: table locks, row locks and page locks. Row locks can improve concurrency, and use the FOR UPDATE statement to add exclusive locks to rows. Pessimistic locks assume conflicts, and optimistic locks judge the data through the version number. Common lock table problems manifest as slow querying, use the SHOW PROCESSLIST command to view the queries held by the lock. Optimization measures include selecting appropriate indexes, reducing transaction scope, batch operations, and optimizing SQL statements.

HadiDB: A lightweight, high-level scalable Python database HadiDB (hadidb) is a lightweight database written in Python, with a high level of scalability. Install HadiDB using pip installation: pipinstallhadidb User Management Create user: createuser() method to create a new user. The authentication() method authenticates the user's identity. fromhadidb.operationimportuseruser_obj=user("admin","admin")user_obj.
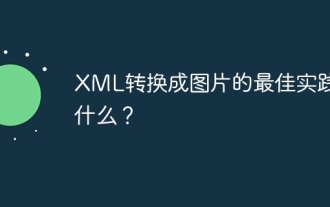
Converting XML into images can be achieved through the following steps: parse XML data and extract visual element information. Select the appropriate graphics library (such as Pillow in Python, JFreeChart in Java) to render the picture. Understand the XML structure and determine how the data is processed. Choose the right tools and methods based on the XML structure and image complexity. Consider using multithreaded or asynchronous programming to optimize performance while maintaining code readability and maintainability.
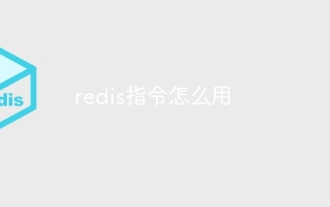
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).
