


Building a stable and reliable caching system: Sharing experience in the design and implementation of Java caching mechanism
Building a reliable caching system: Sharing of design and practical experience of Java caching mechanism
Introduction:
In most applications, data caching is an improvement A common approach to system performance. Caching reduces access to the underlying data source, significantly improving application response time. In Java, we can implement the caching mechanism in a variety of ways. This article will introduce some common caching design patterns and practical experiences, and provide specific code examples.
1. Cache design pattern:
- Memory-based cache
Memory-based cache is the most common cache design pattern. It stores data in memory for quick retrieval when the application needs it, typically using a HashMap or ConcurrentHashMap. Here is a simple memory-based cache example:
import java.util.HashMap; import java.util.Map; import java.util.concurrent.TimeUnit; public class InMemoryCache<T> { private final Map<String, CacheEntry<T>> cache; private final long expirationTime; private static class CacheEntry<T> { private final T value; private final long createTime; CacheEntry(T value) { this.value = value; this.createTime = System.currentTimeMillis(); } boolean isExpired(long expirationTime) { return System.currentTimeMillis() - createTime > expirationTime; } } public InMemoryCache(long expirationTime) { this.cache = new HashMap<>(); this.expirationTime = expirationTime; } public void put(String key, T value) { cache.put(key, new CacheEntry<>(value)); } public T get(String key) { CacheEntry<T> entry = cache.get(key); if (entry != null && !entry.isExpired(expirationTime)) { return entry.value; } else { cache.remove(key); return null; } } public static void main(String[] args) { InMemoryCache<String> cache = new InMemoryCache<>(TimeUnit.MINUTES.toMillis(30)); cache.put("key1", "value1"); String value = cache.get("key1"); System.out.println(value); } }
- Disk-based cache
Disk-based cache stores data in disk files so that it can be cached when the application needs it Read. This cache design pattern works well for larger data sets, but is slower to read than a memory-based cache. The following is a simple disk-based cache example:
import java.io.*; import java.util.HashMap; import java.util.Map; public class DiskCache<T> { private final Map<String, File> cache; public DiskCache() { this.cache = new HashMap<>(); } public void put(String key, T value) { try { File file = new File("cache/" + key + ".bin"); ObjectOutputStream outputStream = new ObjectOutputStream(new FileOutputStream(file)); outputStream.writeObject(value); outputStream.close(); cache.put(key, file); } catch (IOException e) { e.printStackTrace(); } } public T get(String key) { File file = cache.get(key); if (file != null && file.exists()) { try { ObjectInputStream inputStream = new ObjectInputStream(new FileInputStream(file)); T value = (T) inputStream.readObject(); inputStream.close(); return value; } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } } cache.remove(key); return null; } public static void main(String[] args) { DiskCache<String> cache = new DiskCache<>(); cache.put("key1", "value1"); String value = cache.get("key1"); System.out.println(value); } }
2. Caching practical experience:
- Selection of caching strategy
When selecting a caching strategy, you need to Consider the size of the cache, the lifecycle of the data, and the application's access patterns to the data. For frequently accessed and small-capacity data, you can choose memory-based caching; for larger-capacity data sets, you can use disk-based caching. - Cache cleaning and expiration processing
In order to prevent cached data from expiring, cache cleaning and expiration processing need to be performed regularly. You can set an expiration time based on the size and capacity of the cache, or use an elimination strategy (such as least recently used) for data cleaning. - Distributed processing of cache
In a distributed system, the consistency of cached data needs to be considered when multiple nodes share cached data. You can use a distributed cache system (such as Redis) to implement distributed processing of cache and ensure data consistency.
3. Conclusion:
By properly designing and using the caching mechanism, the performance and response speed of the application can be significantly improved. When building a reliable cache system, choose an appropriate cache strategy, perform cache cleaning and expiration regularly, and consider the consistency of distributed caches. This article provides specific code examples of memory- and disk-based caching design patterns, hoping to help readers build reliable caching systems.
References:
- Javatpoint. (2019). Java Cache. https://www.javatpoint.com/java-cache
- Baeldung. (2021) ). Spring Caching with Redis. https://www.baeldung.com/spring-data-redis-cache
The above is the detailed content of Building a stable and reliable caching system: Sharing experience in the design and implementation of Java caching mechanism. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
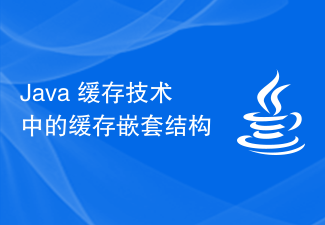
With the rapid development of the Internet and the explosive growth of the number of users, how to improve the performance of websites or applications has become a problem that every developer needs to pay attention to. Among them, caching technology is widely used to improve the response speed of the system and reduce the burden of background operations such as databases. The cache nesting structure in Java cache technology can more effectively improve cache efficiency. A cache is a temporary way of storing data, typically in memory, to avoid the need to access a database or other data source for each request. Simply put, the cache is in memory
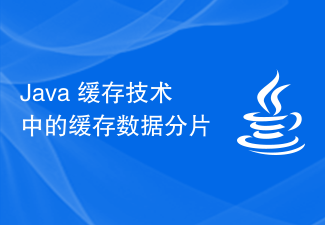
Cache data sharding in Java cache technology With the rapid development of the Internet and the arrival of the big data era, the rapid growth of data volume has brought huge challenges to our data storage and processing. In order to solve this problem, caching technology came into being. Caching refers to storing data in faster storage devices in order to speed up data access and read and write operations. In Java caching technology, caching data sharding is a common technical means. What is cached data sharding? In high-concurrency scenarios, cache often becomes a bottleneck. At this time, we can pass
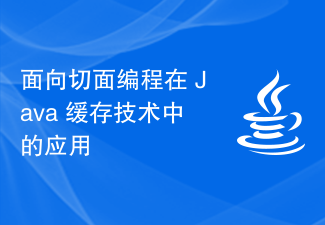
With the continuous development of Internet applications, the requirements for system performance are becoming higher and higher, especially in the field of data caching. Java caching technology has become one of the core technologies for many Internet applications due to its advantages such as high performance, high availability and high scalability. However, as the cache scale continues to expand and the cache logic becomes more complex, it is inevitable to encounter some problems, such as the consistency of cache data and the improvement of cache hit rate. Aspect-oriented programming (AOP) technology can effectively solve these problems by enhancing the process of caching logic.
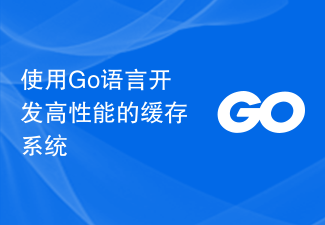
The Go language (also known as Golang) has always been known for its efficient concurrency and excellent performance, so it is very suitable for developing high-performance caching systems. This article will first introduce why Go language was chosen to develop a cache system, and then discuss in detail how to use the features and advantages of Go language to design and implement a high-performance cache system. Why choose Go language? Go language has the following characteristics, making it an ideal choice for developing high-performance caching systems: Concurrency performance: Go language’s built-in goroutine and ch
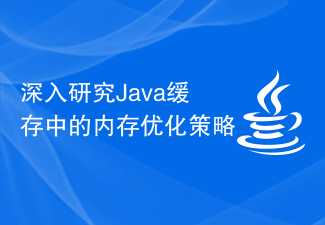
Efficiently Utilize Memory Resources: Exploring Memory Management Strategies in Java Cache Mechanism Overview: During the development process, optimizing memory usage is an important part of improving application performance. As a high-level programming language, Java provides a flexible memory management mechanism, of which caching is a commonly used technical means. This article will introduce the memory management strategy of Java caching mechanism and provide some specific code examples. 1. What is cache? Caching is a technology that temporarily stores calculation results in memory. It stores the calculation results in memory in advance
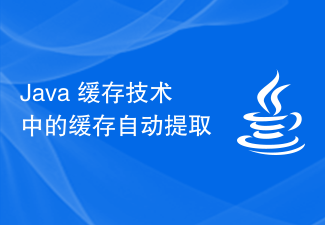
With the continuous development of computer technology, data processing has become more and more important. In the process of processing data, caching technology has always been a popular solution. The automatic cache extraction technology provides great convenience for a large number of applications. Cache automatic retrieval in Java cache technology is a technology that automatically determines whether the cache should be updated based on the cache hit rate. It automatically extracts and updates the content in the cache library by monitoring and counting cache hit rates. This technology uses Java
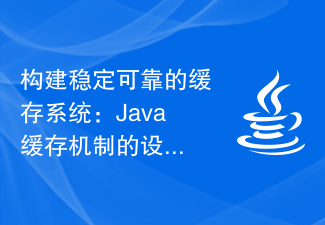
Building a reliable caching system: Design and practical experience sharing of Java caching mechanism Introduction: In most applications, data caching is a common method to improve system performance. Caching reduces access to the underlying data source, significantly improving application response time. In Java, we can implement the caching mechanism in a variety of ways. This article will introduce some common caching design patterns and practical experiences, and provide specific code examples. 1. Cache design pattern: Memory-based cache Memory-based cache is the most common
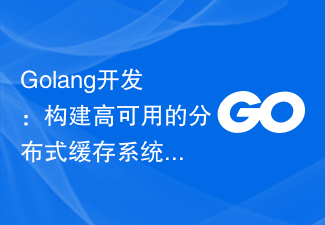
Golang development: Building a highly available distributed cache system Introduction: With the continuous development of the Internet, distributed cache systems play an important role in large-scale applications. In high-concurrency environments, traditional database storage methods often cannot meet application performance requirements. Therefore, distributed cache systems have become one of the solutions to achieve high efficiency and scalability. This article will introduce how to use Golang to develop a highly available distributed cache system, and provide specific code examples for readers' reference and learning. 1. Distributed cache
