A comprehensive guide to parsing Maven local repository configuration
Detailed explanation of Maven local warehouse configuration, specific code examples are required
When using Maven to build a project, the configuration of the local warehouse is a very important part. This article will introduce in detail how to correctly configure the Maven local repository, with code examples.
The Maven local repository is a place used to save the dependent libraries required in the project. When Maven builds a project, it will automatically download the required dependency libraries from the remote warehouse based on the dependency configuration in the pom.xml file in the project and save them in the local warehouse. In this way, when the project is built again, Maven does not need to go to the remote warehouse to download the dependent libraries, but directly obtains them from the local warehouse.
To correctly configure the Maven local warehouse, you first need to find the settings.xml file in the conf folder in the Maven installation directory. By editing this file, you can modify the location of the local repository.
Find the following code block:
<!-- localRepository | The path to the local repository maven will use to store artifacts. | | Default: ${user.home}/.m2/repository --> <localRepository>${user.home}/.m2/repository</localRepository>
Replace ${user.home}/.m2/repository
with the custom local warehouse path. For example:
<!-- localRepository | The path to the local repository maven will use to store artifacts. | | Default: ${user.home}/.m2/repository --> <localRepository>/path/to/local/repository</localRepository>
After configuring the local warehouse path, you need to ensure that the path is valid in the system. You can check whether the local warehouse path is correct by executing the following command in the terminal:
mvn help:system
In the output result, look for the following content:
[INFO] Local Repository: /path/to/local/repository
If the path is displayed correctly, the configuration is successful.
In addition to configuring the local warehouse path in the settings.xml file, you can also specify the local warehouse path by adding the following <repositories>
element in the pom.xml file:
<repositories> <repository> <id>local-repo</id> <url>file:/path/to/local/repository</url> <releases> <enabled>true</enabled> </releases> <snapshots> <enabled>true</enabled> </snapshots> </repository> </repositories>
After this configuration, Maven will give priority to the local warehouse path specified in the pom.xml file, ignoring the configuration in the settings.xml file. When multiple people collaborate on a project, the local warehouse path can be specified uniformly to ensure that everyone is using the same local warehouse.
After configuring the path to the local warehouse, the next step is to install the dependent libraries into the local warehouse. There are two ways to install dependent libraries into the local warehouse:
- Use the Maven command
mvn install
, which will build the project and install the generated jar package into the local warehouse . - Manually copy the jar package of the dependent library to the corresponding location of the local warehouse.
The following is a code example showing how to manually install dependent libraries into a local warehouse:
mvn install:install-file -Dfile=/path/to/dependency.jar -DgroupId=com.example -DartifactId=dependency -Dversion=1.0 -Dpackaging=jar
Using this command will /path/to/dependency .jar
Copy to the com/example/dependency/1.0/
directory of the local warehouse.
By correctly configuring the Maven local repository and installing dependent libraries into the local repository, the project construction time and dependency conflict issues can be effectively reduced. At the same time, the unified local warehouse path also facilitates team collaboration and version control. I hope that the detailed introduction and code examples of this article can help readers correctly configure the Maven local warehouse and improve the efficiency and stability of project construction.
The above is the detailed content of A comprehensive guide to parsing Maven local repository configuration. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
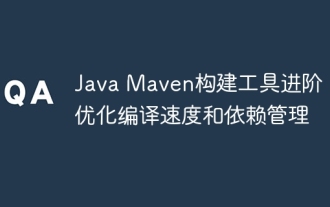
Optimize Maven build tools: Optimize compilation speed: Take advantage of parallel compilation and incremental compilation. Optimize dependencies: Analyze dependency trees and use BOM (bill of materials) to manage transitive dependencies. Practical case: illustrate optimizing compilation speed and dependency management through examples.
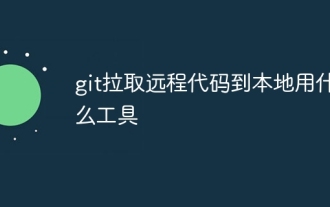
Specific steps for Git to pull remote code to the local warehouse: Open Git Bash or a terminal window. Navigate to the local repository directory where you want to pull the code. Run command: git pull
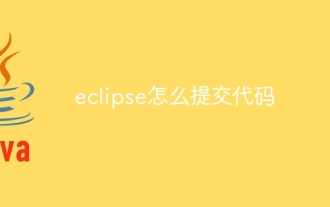
To commit code using Eclipse, follow these steps: Set up a version control system: Configure the Git path and initialize the remote repository. Create a Git repository: Select the project, right-click Shared Project and select Git. Add files to the staging area: Select the file in the "Git Staging" view and click the "+" button. Submit changes: Enter the information in the Submit message and click the Submit button. Push changes to the remote repository: Right-click the remote repository in the Git Repositories view and select Push.
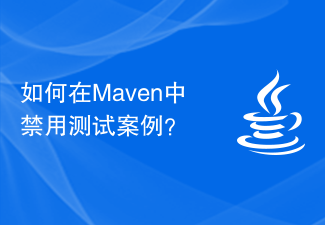
Maven is an open source project management tool that is commonly used for tasks such as construction, dependency management, and document release of Java projects. When using Maven for project build, sometimes we want to ignore the testing phase when executing commands such as mvnpackage, which will improve the build speed in some cases, especially when a prototype or test environment needs to be built quickly. This article will detail how to ignore the testing phase in Maven, with specific code examples. Why you should ignore testing During project development, it is often
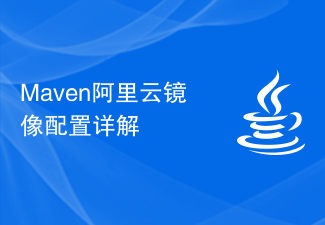
Detailed explanation of Maven Alibaba Cloud image configuration Maven is a Java project management tool. By configuring Maven, you can easily download dependent libraries and build projects. The Alibaba Cloud image can speed up Maven's download speed and improve project construction efficiency. This article will introduce in detail how to configure Alibaba Cloud mirroring and provide specific code examples. What is Alibaba Cloud Image? Alibaba Cloud Mirror is the Maven mirror service provided by Alibaba Cloud. By using Alibaba Cloud Mirror, you can greatly speed up the downloading of Maven dependency libraries. Alibaba Cloud Mirror
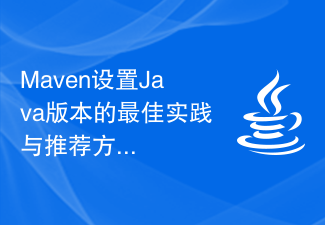
When using Maven to build a Java project, you often encounter situations where you need to set the Java version. Correctly setting the Java version can not only ensure that the project runs normally in different environments, but also avoid some compatibility issues and improve the stability and maintainability of the project. This article will introduce the best practices and recommended methods for setting Java versions in Maven, and provide specific code examples for reference. 1. Set the Java version in the pom.xml file. In the pom.xml file of the Maven project, you can
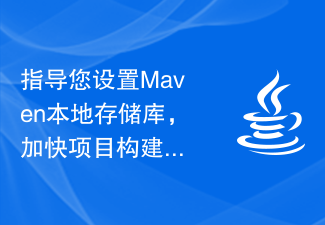
Teach you step by step how to configure Maven local warehouse: improve project construction speed Maven is a powerful project management tool that is widely used in Java development. It can help us manage project dependencies, build projects, and publish projects, etc. However, during the actual development process, we sometimes encounter the problem of slow project construction. One solution is to configure a local repository to improve project build speed. This article will teach you step by step how to configure the Maven local warehouse to make your project construction more efficient. Why do you need to configure a local warehouse?
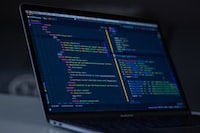
A version control system (VCS) is an indispensable tool in software development that allows developers to track and manage code changes. git is a popular and powerful VCS that is widely used in Java development. This guide will introduce the basic concepts and operations of Git, providing Java developers with the basics of version control. The basic concept of Git Repository: where code and version history are stored. Branch: An independent line of development in a code base that allows developers to make changes without affecting the main line of development. Commit: A change to the code in the code base. Rollback: Revert the code base to a previous commit. Merge: Merge changes from two or more branches into a single branch. Getting Started with Git 1. Install Git Download and download from the official website
