How to write a simple image recognition program using C++?
How to write a simple image recognition program using C?
In the development of modern science and technology, image recognition technology plays an increasingly important role. Whether it is face recognition, object detection or autonomous driving, image recognition plays a key role. This article will introduce how to use C to write a simple image recognition program to help readers understand the basic principles and implementation process of image recognition.
First, we need to install and configure OpenCV (open source computer vision library). OpenCV is a widely used computer vision library for processing image and video data. It provides a rich set of functions and tools for tasks such as image processing, feature extraction, and machine learning.
After installing OpenCV, we can start writing image recognition programs. Here is a simple example for recognizing faces in images:
#include <opencv2/opencv.hpp> int main() { cv::CascadeClassifier cascade; cascade.load("haarcascade_frontalface_default.xml"); cv::VideoCapture video(0); cv::Mat frame; while (true) { video >> frame; std::vector<cv::Rect> faces; cv::Mat gray_frame; cv::cvtColor(frame, gray_frame, cv::COLOR_BGR2GRAY); cv::equalizeHist(gray_frame, gray_frame); cascade.detectMultiScale(gray_frame, faces, 1.1, 3, 0, cv::Size(30, 30)); for (const auto& face : faces) { cv::rectangle(frame, face, cv::Scalar(0, 255, 0), 2); } cv::imshow("Face Recognition", frame); if (cv::waitKey(30) >= 0) { break; } } return 0; }
In this example, we first load a pre-trained face recognition model (haarcascade_frontalface_default.xml). Then, we open the camera and obtain a frame of image by calling the cv::VideoCapture class. Next, we convert each frame of image into a grayscale image and perform histogram equalization. This step can enhance the contrast of the image and help extract features in the image. Then, we use the detectMultiScale function of the cv::CascadeClassifier class to identify the face in the image, and mark the recognition result on the image with a rectangular box. Finally, we use the cv::imshow function to display the recognition results. By calling the cv::waitKey function, we can wait for the user to press any key on the keyboard to exit the program after each frame of image display.
This is just a simple image recognition example that shows how to use OpenCV and C to implement basic image recognition functions. Readers can further extend the program according to their own needs, such as calling different pre-trained models to detect other objects, or combining other image processing techniques to improve the accuracy of recognition.
To sum up, image recognition is a very meaningful technical field and has a wide range of applications in various industries. Through learning and practice, we can use C and OpenCV to write image recognition programs and provide powerful functional support for our projects. It is hoped that readers can have a certain understanding of the implementation and application of image recognition through the introduction and sample programs of this article, and can further learn and apply related technologies in depth.
The above is the detailed content of How to write a simple image recognition program using C++?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
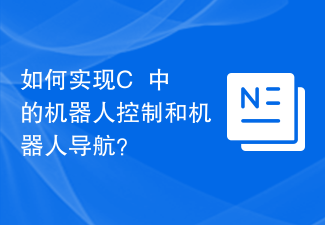
How to implement robot control and robot navigation in C++? Robot control and navigation are very important parts of robotics technology. In the C++ programming language, we can use various libraries and frameworks to implement robot control and navigation. This article will introduce how to use C++ to write code examples for controlling robots and implementing navigation functions. 1. Robot control In C++, we can use serial communication or network communication to realize robot control. The following is a sample code that uses serial communication to control robot movement: inclu
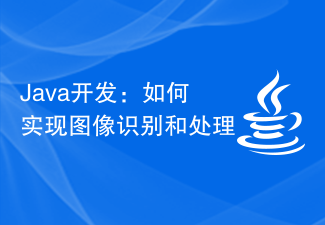
Java Development: A Practical Guide to Image Recognition and Processing Abstract: With the rapid development of computer vision and artificial intelligence, image recognition and processing play an important role in various fields. This article will introduce how to use Java language to implement image recognition and processing, and provide specific code examples. 1. Basic principles of image recognition Image recognition refers to the use of computer technology to analyze and understand images to identify objects, features or content in the image. Before performing image recognition, we need to understand some basic image processing techniques, as shown in the figure
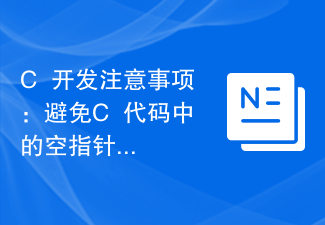
In C++ development, null pointer exception is a common error, which often occurs when the pointer is not initialized or is continued to be used after being released. Null pointer exceptions not only cause program crashes, but may also cause security vulnerabilities, so special attention is required. This article will explain how to avoid null pointer exceptions in C++ code. Initializing pointer variables Pointers in C++ must be initialized before use. If not initialized, the pointer will point to a random memory address, which may cause a Null Pointer Exception. To initialize a pointer, point it to an
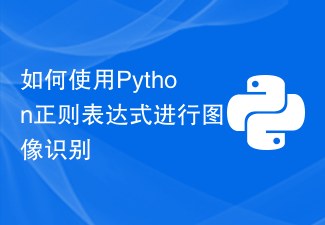
In computer science, image recognition has always been an important field. Using image recognition, we can let the computer recognize and analyze the content in the image and process it. Python is a very popular programming language that can be used in many fields, including image recognition. This article will introduce how to use Python regular expressions for image recognition. Regular expressions are a text pattern matching tool used to find text that matches a specific pattern. Python has a built-in "re" module for regular expressions
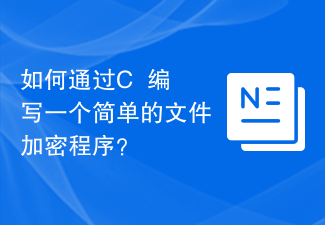
How to write a simple file encryption program in C++? Introduction: With the development of the Internet and the popularity of smart devices, the importance of protecting personal data and sensitive information has become increasingly important. In order to ensure the security of files, it is often necessary to encrypt them. This article will introduce how to use C++ to write a simple file encryption program to protect your files from unauthorized access. Requirements analysis: Before starting to write a file encryption program, we need to clarify the basic functions and requirements of the program. In this simple program we will use symmetry
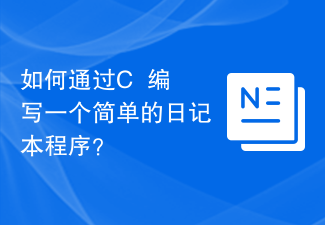
How to write a simple diary program in C++? Journals are a tool for many people to record their lives, thoughts, and feelings. By writing a simple diary program, you can record and manage personal diaries more conveniently and efficiently. In this article, we will introduce how to write a simple diary program using C++ language. First, we need to determine the basic functionality of the diary program. A simple diary program should have the following functions: Add diary: users can enter their own diary content and save it to a file. View date
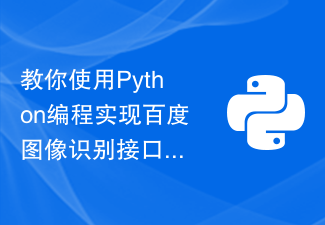
Teach you to use Python programming to implement the docking of Baidu's image recognition interface and realize the image recognition function. In the field of computer vision, image recognition technology is a very important technology. Baidu provides a powerful image recognition interface through which we can easily implement image classification, labeling, face recognition and other functions. This article will teach you how to use the Python programming language to realize the image recognition function by connecting to the Baidu image recognition interface. First, we need to create an application on Baidu Developer Platform and obtain
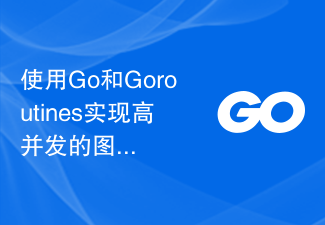
Using Go and Goroutines to implement a highly concurrent image recognition system Introduction: In today's digital world, image recognition has become an important technology. Through image recognition, we can convert information such as objects, faces, scenes, etc. in images into digital data. However, for recognition of large-scale image data, speed often becomes a challenge. In order to solve this problem, this article will introduce how to use Go language and Goroutines to implement a high-concurrency image recognition system. Background: Go language
