Rotation invariance problem in image recognition
The issue of rotation invariance in image recognition
Abstract: In image recognition tasks, the rotation invariance of images is an important issue. In order to solve this problem, this article introduces a method based on convolutional neural network (CNN) and gives specific code examples.
- Introduction
Image recognition is an important research direction in the field of computer vision. In many practical applications, the rotation invariance of images is a critical issue. For example, in face recognition, the same person's face should still be correctly recognized when rotated at different angles. Therefore, how to achieve rotation invariance of images becomes a challenge. - Related Work
In past research, various methods have been proposed to solve the problem of image rotation invariance. One of the common methods is to use Scale-Invariant Feature Transform (SIFT) to extract image features, and then achieve rotation invariance through feature matching. However, this method requires detecting and matching a large number of feature points in the image, and the computational complexity is high. - Methods based on convolutional neural networks
In recent years, with the development of deep learning, Convolutional Neural Network (CNN) has achieved great success in the field of image recognition. CNN can automatically learn the characteristics of images through multi-layer convolution and pooling operations. In order to achieve image rotation invariance, we can use the feature extraction capability of CNN and perform rotation invariance operations on the features. - Code Example
The following is a simple code example implemented in Python language, showing how to use CNN to achieve rotation invariance of images.
import numpy as np import tensorflow as tf # 构建CNN模型 model = tf.keras.Sequential([ tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(32, 32, 3)), tf.keras.layers.MaxPooling2D((2, 2)), tf.keras.layers.Conv2D(64, (3, 3), activation='relu'), tf.keras.layers.MaxPooling2D((2, 2)), tf.keras.layers.Flatten(), tf.keras.layers.Dense(64, activation='relu'), tf.keras.layers.Dropout(0.2), tf.keras.layers.Dense(10, activation='softmax') ]) # 加载训练数据 (x_train, y_train), (x_test, y_test) = tf.keras.datasets.cifar10.load_data() # 数据预处理 x_train = x_train / 255.0 x_test = x_test / 255.0 # 训练模型 model.compile(optimizer='adam', loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True), metrics=['accuracy']) model.fit(x_train, y_train, epochs=10) # 旋转测试图像 test_image = np.array([[0.5, 0.5, 0.5], [0.5, 0.5, 0.5], [0.5, 0.5, 0.5]]) rotated_image = tf.image.rot90(test_image) # 预测图像 predictions = model.predict(np.expand_dims(rotated_image, 0)) print(predictions)
- Conclusion
This article introduces the problem of rotation invariance in image recognition and gives a specific code example based on CNN. By using convolutional neural networks, we can achieve rotation invariance of images and improve the accuracy of image recognition. Future research can further explore more efficient and accurate methods on this basis.
References:
[1] Lowe, D. G. (2004). Distinctive image features from scale-invariant keypoints. International journal of computer vision, 60(2), 91-110.
[2] LeCun, Y., Bengio, Y., & Hinton, G. (2015). Deep learning. nature, 521(7553), 436-444.
Keywords: Image recognition; Rotation invariance; convolutional neural network; code example
The above is the detailed content of Rotation invariance problem in image recognition. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
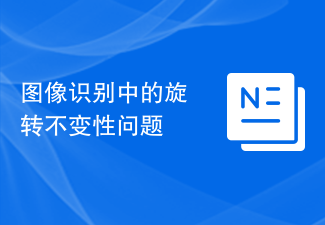
Summary of the issue of rotation invariance in image recognition: In image recognition tasks, the rotation invariance of images is an important issue. In order to solve this problem, this article introduces a method based on convolutional neural network (CNN) and gives specific code examples. Introduction Image recognition is an important research direction in the field of computer vision. In many practical applications, the rotation invariance of images is a critical issue. For example, in face recognition, the same person's face should still be correctly recognized when rotated at different angles. therefore,
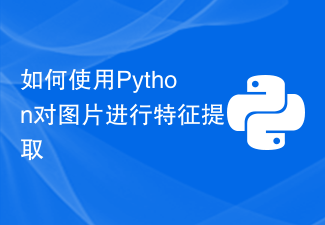
How to use Python to extract features from images In computer vision, feature extraction is an important process. By extracting the key features of an image, we can better understand the image and use these features to achieve various tasks, such as target detection, face recognition, etc. Python provides many powerful libraries that can help us perform feature extraction on images. This article will introduce how to use Python to extract features from images and provide corresponding code examples. Environment configuration First, we need to install Python
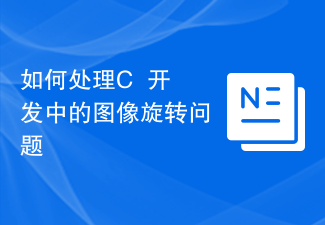
Image processing is one of the common tasks in C++ development. Image rotation is a common requirement in many applications, whether implementing image editing functions or image processing algorithms. This article will introduce how to deal with image rotation problems in C++. 1. Understand the principle of image rotation. Before processing image rotation, you first need to understand the principle of image rotation. Image rotation refers to rotating an image around a certain center point to generate a new image. Mathematically, image rotation can be achieved through matrix transformation, and the rotation matrix can be used to
![[Python NLTK] Text classification, easily solve text classification problems](https://img.php.cn/upload/article/000/465/014/170882739298555.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
Text classification is one of the natural language processing (NLP) tasks that aims to classify text into predefined categories. Text classification has many practical applications, such as email filtering, spam detection, sentiment analysis, and question answering systems, etc. The task of using the pythonNLTK library to complete text classification can be divided into the following steps: Data preprocessing: First, the data needs to be preprocessed, including removing punctuation marks, converting to lowercase, removing spaces, etc. Feature extraction: Next, features need to be extracted from the preprocessed text. Features can be words, phrases, or sentences. Model training: Then, the extracted features need to be used to train a classification model. Commonly used classification models include Naive Bayes, Support Vector Machines, and Decision Trees. Assessment: Final
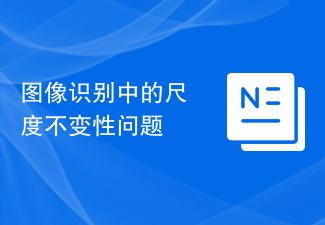
Scale invariance issues in image recognition, requiring specific code examples Abstract: In the field of image recognition, scale invariance has always been a key issue. This article will introduce the concept and significance of scale invariance, and provide some specific code examples to help readers better understand and apply scale invariance in image recognition. 1. Introduction In image recognition tasks, scale invariance is a very important issue. Scale invariance means that when an image is transformed at different scales, its recognition results should remain consistent. This is because in real

Latent feature learning problem in unsupervised learning, requires specific code examples In the field of machine learning, unsupervised learning refers to the automatic learning and discovery of useful structures and patterns in data without label or category information. In unsupervised learning, latent feature learning is an important problem, which aims to learn higher-level, more abstract feature representations from raw input data. The goal of latent feature learning is to discover the most discriminating features from raw data to facilitate subsequent classification, clustering or other machine learning tasks. it can help
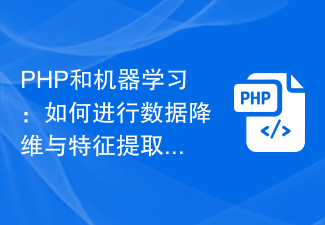
PHP and machine learning: How to perform data dimensionality reduction and feature extraction Introduction: Machine learning plays an increasingly important role in today's technological development. As the size of data continues to grow, processing and analyzing big data has become particularly critical. In machine learning, data dimensionality reduction and feature extraction are two very important tasks. They can help us reduce the dimensionality of the data set and extract key information for better model training and prediction. This article will introduce how to use PHP for data dimensionality reduction and feature extraction, and give corresponding code examples. 1. What
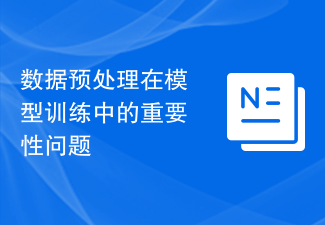
The importance of data preprocessing in model training and specific code examples Introduction: In the process of training machine learning and deep learning models, data preprocessing is a very important and essential link. The purpose of data preprocessing is to transform raw data into a form suitable for model training through a series of processing steps to improve the performance and accuracy of the model. This article aims to discuss the importance of data preprocessing in model training and give some commonly used data preprocessing code examples. 1. The importance of data preprocessing Data cleaning Data cleaning is the
