


How to choose the right Python web framework for your project?
How to choose the right Python web framework for your project?
In modern software development, choosing the right programming language and framework is a crucial step. As a powerful and popular programming language, Python has many excellent web frameworks to choose from. This article will introduce some common Python web frameworks and provide code examples to help you choose the right framework for your project.
- Django:
Django is a powerful and mature Python web framework. It provides rich features and out-of-the-box solutions for quickly building large and complex web applications. Here is a simple Django code example:
from django.urls import path from django.http import HttpResponse def hello(request): return HttpResponse("Hello, Django!") urlpatterns = [ path('hello/', hello), ]
- Flask:
Flask is a tiny and flexible Python web framework suitable for building small and fast web applications. It provides a simple and elegant interface that is easy to understand and get started. The following is a simple Flask code example:
from flask import Flask app = Flask(__name__) @app.route('/hello') def hello(): return "Hello, Flask!" if __name__ == '__main__': app.run()
- Bottle:
Bottle is a simple and lightweight Python web framework suitable for small projects and API development. It relies only on the Python standard library and requires no external dependencies. The following is a simple Bottle code example:
from bottle import route, run @route('/hello') def hello(): return "Hello, Bottle!" run(host='localhost', port=8000)
- Pyramid:
Pyramid is a general-purpose Python web framework that provides a large number of customizable features and a flexible architecture. It is suitable for medium to large projects and applications requiring a high degree of customization. Here is a simple Pyramid code example:
from wsgiref.simple_server import make_server from pyramid.config import Configurator from pyramid.response import Response def hello(request): return Response('Hello, Pyramid!') if __name__ == '__main__': config = Configurator() config.add_route('hello', '/hello') config.add_view(hello, route_name='hello') app = config.make_wsgi_app() server = make_server('localhost', 8000, app) server.serve_forever()
Choosing the right Python web framework depends on your project needs and personal preference. If you need to build a simple web application quickly, Flask and Bottle may be more suitable for you. If you need to handle complex business logic and large databases, Django and Pyramid are better choices.
The above is the detailed content of How to choose the right Python web framework for your project?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
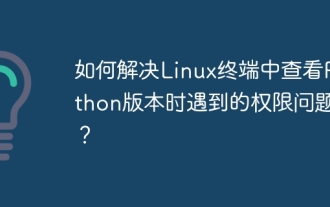
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
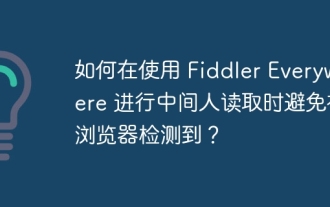
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
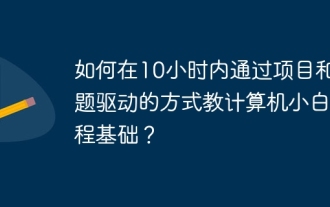
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
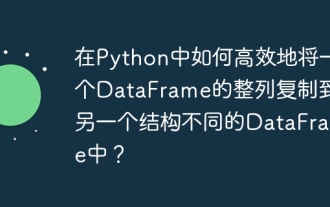
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
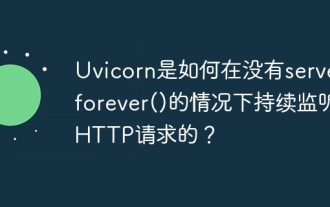
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
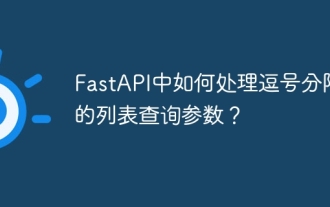
Fastapi ...
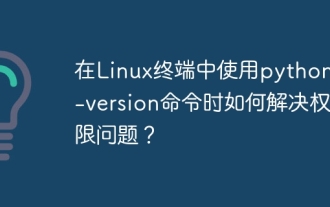
Using python in Linux terminal...
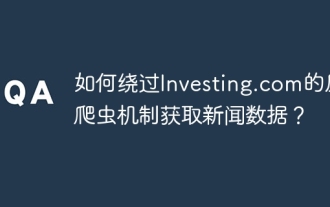
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
