


How to use React and WebSocket to implement real-time communication functions
How to use React and WebSocket to implement real-time communication functions
Overview:
In modern web applications, real-time communication has become very important. Many applications need to be able to obtain and update data in real time in order to display the latest information to users in a timely manner. In this article, we will introduce how to use React and WebSocket to implement real-time communication capabilities. We will demonstrate how to build a real-time chat application using WebSocket and provide specific code examples.
What is WebSocket?
WebSocket is a modern network communication protocol that provides a simple and efficient method for two-way communication between clients and servers. The WebSocket protocol allows long connections between clients and servers and can push these updates to the client in real time as data is updated. WebSockets are more efficient than the traditional HTTP request-response model and enable push notifications and instant communications in real-time applications.
Create a simple chat application using React:
First, we need to create a React application. We can use the Create React App tool to create a new React application. Run the following command in the terminal to create a new React application:
npx create-react-app realtime-chat-app
Once created, we can go into the folder and launch our application:
cd realtime-chat-app npm start
Now we have You have a basic React application. We will add WebSocket on this basis to realize real-time communication function.
Implementing WebSocket communication function:
First, we need to install the websocket library. Run the following command in the terminal to install the websocket library:
npm install --save websocket
In the React component, we can use the useState hook to manage our chat message list. We will also use the useEffect hook to initialize the WebSocket connection and handle received messages. The following is a sample code for a simple chat box component:
import React, { useState, useEffect } from "react"; import WebSocket from "websocket"; const ChatBox = () => { const [messages, setMessages] = useState([]); const [inputValue, setInputValue] = useState(""); let ws; useEffect(() => { ws = new WebSocket("ws://localhost:8000/ws"); // WebSocket服务器地址 ws.onopen = () => { console.log("WebSocket连接已建立"); }; ws.onmessage = (event) => { const message = JSON.parse(event.data); setMessages((messages) => [...messages, message]); }; return () => { ws.close(); }; }, []); const sendMessage = () => { ws.send(JSON.stringify({ content: inputValue })); setInputValue(""); }; return ( <div> <div> {messages.map((message, index) => ( <p key={index}>{message.content}</p> ))} </div> <input type="text" value={inputValue} onChange={(event) => setInputValue(event.target.value)} /> <button onClick={sendMessage}>发送</button> </div> ); }; export default ChatBox;
In the above code, we use useState to manage the chat message list (messages) and the value of the input box (inputValue). We also declare a WebSocket connection (ws) and initialize it when the component loads. When new messages are received, we use setMessages to update the message list. When the component is about to be unloaded, we close the WebSocket connection.
In the render function, we will render the message list and an input box. When the user clicks the send button, we will send the text content in the input box to the WebSocket server.
Start the WebSocket server:
In order for our WebSocket application to work properly, we also need to start a WebSocket server. In this example, we will use Node.js and the ws library to create a simple WebSocket server. Run the following command in the terminal to install the ws library:
npm install --save ws
Then, we can create a file called server.js and use the following code to create the WebSocket server:
const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8000 }); wss.on('connection', (ws) => { ws.on('message', (message) => { // 处理接收到的消息 wss.clients.forEach((client) => { if (client.readyState === WebSocket.OPEN) { client.send(message); } }); }); });
Now we The WebSocket server can be started by running the following command:
node server.js
Finally, we just need to add the ChatBox component to App.js and run our React application. When we enter a message in the input box and click the send button, the message will be transmitted to the server via WebSocket and returned to all connected clients in real time.
Summary:
In this article, we introduced how to use React and WebSocket to implement real-time communication functions. We use useState and useEffect hooks to manage and update the state of React components. We also create a WebSocket connection and update the UI when new messages are received. Finally, we created a simple WebSocket server to handle and forward messages. I hope this article helped you understand how to implement real-time communication functionality in React applications.
The above is the detailed content of How to use React and WebSocket to implement real-time communication functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
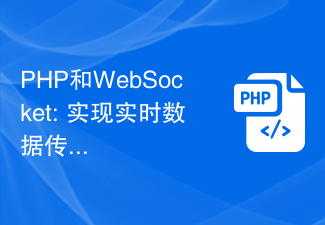
PHP and WebSocket: Best Practice Methods for Real-Time Data Transfer Introduction: In web application development, real-time data transfer is a very important technical requirement. The traditional HTTP protocol is a request-response model protocol and cannot effectively achieve real-time data transmission. In order to meet the needs of real-time data transmission, the WebSocket protocol came into being. WebSocket is a full-duplex communication protocol that provides a way to communicate full-duplex over a single TCP connection. Compared to H
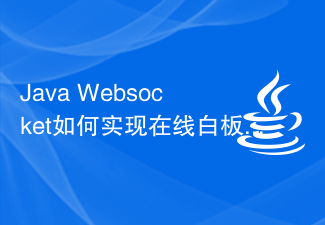
How does JavaWebsocket implement online whiteboard function? In the modern Internet era, people are paying more and more attention to the experience of real-time collaboration and interaction. Online whiteboard is a function implemented based on Websocket. It enables multiple users to collaborate in real-time to edit the same drawing board and complete operations such as drawing and annotation. It provides a convenient solution for online education, remote meetings, team collaboration and other scenarios. 1. Technical background WebSocket is a new protocol provided by HTML5. It implements
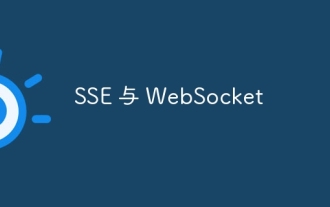
In this article, we will compare Server Sent Events (SSE) and WebSockets, both of which are reliable methods for delivering data. We will analyze them in eight aspects, including communication direction, underlying protocol, security, ease of use, performance, message structure, ease of use, and testing tools. A comparison of these aspects is summarized as follows: Category Server Sent Event (SSE) WebSocket Communication Direction Unidirectional Bidirectional Underlying Protocol HTTP WebSocket Protocol Security Same as HTTP Existing security vulnerabilities Ease of use Setup Simple setup Complex performance Fast message sending speed Affected by message processing and connection management Message structure Plain text or binary Ease of use Widely available Helpful for WebSocket integration
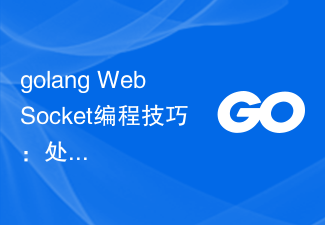
Golang is a powerful programming language, and its use in WebSocket programming is increasingly valued by developers. WebSocket is a TCP-based protocol that allows two-way communication between client and server. In this article, we will introduce how to use Golang to write an efficient WebSocket server that handles multiple concurrent connections at the same time. Before introducing the techniques, let's first learn what WebSocket is. Introduction to WebSocketWeb
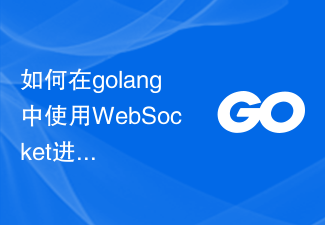
How to use WebSocket for file transfer in golang WebSocket is a network protocol that supports two-way communication and can establish a persistent connection between the browser and the server. In golang, we can use the third-party library gorilla/websocket to implement WebSocket functionality. This article will introduce how to use golang and gorilla/websocket libraries for file transfer. First, we need to install gorilla
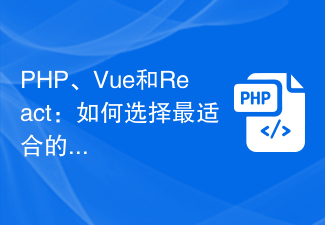
PHP, Vue and React: How to choose the most suitable front-end framework? With the continuous development of Internet technology, front-end frameworks play a vital role in Web development. PHP, Vue and React are three representative front-end frameworks, each with its own unique characteristics and advantages. When choosing which front-end framework to use, developers need to make an informed decision based on project needs, team skills, and personal preferences. This article will compare the characteristics and uses of the three front-end frameworks PHP, Vue and React.
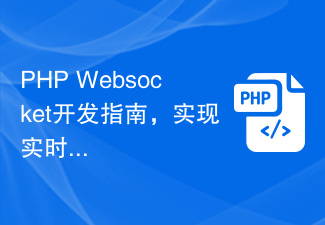
PHP Websocket Development Guide: Implementing Real-time Translation Function Introduction: With the development of the Internet, real-time communication is becoming more and more important in various application scenarios. As an emerging communication protocol, Websocket provides good support for real-time communication. This article will take you through a detailed understanding of how to use PHP to develop Websocket applications, and combine the real-time translation function to demonstrate its specific application. 1. What is the Websocket protocol? The Websocket protocol is a
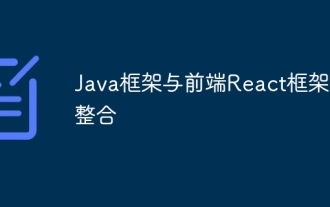
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.
