Write a program to print the binomial expansion series
The binomial expansion is a mathematical formula used to expand expressions of the form (a b)^n, where n is a positive integer and a and b can be any real or complex numbers. The expansion gives the coefficients of each term in the expansion.
A binomial expansion can be expressed as
$$\mathrm{(a b)^n= ^nC_0a^nb^0 ^nC_1a^{n-1}b^1 ^nCa^{n-2}b^2 ... ^nC_ra^{n-r }b^r ... ^nC_na^0b^n}$$
Where $\mathrm{^nC_r}$ is the binomial coefficient, given by the following formula
$\mathrm{^nC_r=\frac{n!}{r!\times(n−r)!}}$, where n! Represents the factorial of n
Expansion can be used to calculate all binomial terms using the above formula and substitute them into the expansion equation.
Problem Statement
Given three integers a, b and n. Find the terms of the binomial expansion of (a b)^n.
Example Example 1
Enter -
a = 1, b = 2, n = 3
Output -
[1, 6, 12, 8]
Explanation
is:Explanation
The binomial expansion (1 2)^3 is as follows
$\mathrm{(1 2)^3 = C(3,0)a^3b^0 C(3,1)a^2b^1 C(3,2)a^1b^2 C(3 ,3)a^0b^3}$
= 1*1*1 3*1*2 3*1*4 1*1*8
Therefore, [1, 6, 12, 8] are the terms of the binomial expansion.
Example Example 2
Enter -
a = 7, b = 2, n = 11
Output -
[2401, 2744, 1176, 224, 16]
Method 1: Recursive binomial expansion
Use the binomial expansion formula,
$$\mathrm{(a b)^n= ^nC_0a^nb^0 ^nC_1a^{n-1}b^1 ^nCa^{n-2}b^2 ... ^nC_ra^{n-r }b^r ... ^nC_na^0b^n}$$
We can find the value of each term by recursively calculating the binomial coefficients.
pseudocode
procedure binomialCoeff (n, r) if r == 0 or r == n ans = 1 else ans = binomialCoeff (n - 1, r - 1) + binomialCoeff (n - 1, r) end procedure procedure binomialTerms (a, b, n) Initialize vector: arr for r = 0 to n coeff = binomialCoeff(n, r) term = coeff + a^n-r + b^r add the term to arr ans = arr end procedure
Example: C implementation
In the following program, the binomialCoeff() function recursively calculates the value of the r-th binomial coefficient, while the binomialTerms() function calculates the value of the binomial term in the expansion.
#include <bits/stdc++.h> using namespace std; // Function for calculating binomial coefficients int binomialCoeff(int n, int r){ if (r == 0 || r == n) { return 1; } else { return binomialCoeff(n - 1, r - 1) + binomialCoeff(n - 1, r); } } // Function for calculating the binomial terms vector<int> binomialTerms(int a, int b, int n){ vector<int> ans; for (int r = 0; r <= n; r++) { // Calculate the rth binomial coefficients int coeff = binomialCoeff(n, r); // Calculate the rth binomial expansion term int term = coeff * pow(a, n - r) * pow(b, r); ans.push_back(term); } return ans; } int main(){ int a = 2, b = 3, n = 4; vector<int> res = binomialTerms(a, b, n); cout << "The binomial terms are : "; for (int i = 0; i < res.size(); i++) { cout << res[i] << " "; } return 0; }
Output
The binomial terms are : 16 96 216 216 81
Time complexity - O(2^n), where the time complexity of the binomialCoeff() function is O(2^n due to the recursive tree and 2^n nodes in binomialTerms() ) Since the nested loop calls binomialCoeff() n 1 times, the complexity of the function is O(n^2). So the overall complexity is O(2^n).
Space complexity - Due to the recursive call stack, the space complexity is O(n).
Method 2: Iterative Binomial Expansion
Use the binomial expansion formula,
$$\mathrm{(a b)^n= ^nC_0a^nb^0 ^nC_1a^{n-1}b^1 ^nCa^{n-2}b^2 ... ^nC_ra^{n-r }b^r ... ^nC_na^0b^n}$$
We can find the value of each term of this expansion by combining iteration and division.
We will create 2 functions where the first function calculates the binomial coefficient and the second function multiplies the powers of a and b to obtain the desired binomial term.
pseudocode
procedure binomialCoeff (n, r) res = 1 if r > n - r r = n - r end if for i = 0 to r-1 res = res * (n - i) res = res / (i + 1) ans = res end procedure procedure binomialTerms (a, b, n) Initialize vector: arr for r = 0 to n coeff = binomialCoeff(n, r) term = coeff + a^n-r + b^r add the term to arr ans = arr end procedure
Example: C implementation
In the following program, the binomialCoeff() function computes the r-th binomial coefficient, while the binomialTerms() function computes all terms of the binomial expansion given a, b, and n.
#include <bits/stdc++.h> using namespace std; // Function for calculating binomial coefficients int binomialCoeff(int n, int r){ int res = 1; if (r > n - r) { r = n - r; } for (int i = 0; i < r; i++) { res *= (n - i); res /= (i + 1); } return res; } // Function for calculating the binomial terms vector<int> binomialTerms(int a, int b, int n){ vector<int> ans; for (int r = 0; r <= n; r++){ // Calculate the rth binomial coefficients int coeff = binomialCoeff(n, r); // Calculate the rth binomial expansion term int term = coeff * pow(a, n - r) * pow(b, r); ans.push_back(term); } return ans; } int main(){ int a = 2, b = 3, n = 4; vector<int> res = binomialTerms(a, b, n); cout << "The binomial terms are : "; for (int i = 0; i < res.size(); i++){ cout << res[i] << " "; } return 0; }
Output
The binomial terms are : 16 96 216 216 81
Time complexity - O(n^2), where the time complexity of the binomialCoeff() function is O(r), where r is the smaller of r and n-r and binomialTerms() The function calls binomialCoeff() n 1 times due to the nested loop, and the complexity is O(n^2). So the overall complexity is O(n^2).
Space Complexity - Since the vector stores binomial terms, it is O(n).
in conclusion
In summary, to find the binomial terms of the binomial expansion, we can use one of the two methods mentioned above, with time complexity ranging from O(2^n) to O(n^2), where the iterative method is better than Recursive methods are more optimized.
The above is the detailed content of Write a program to print the binomial expansion series. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
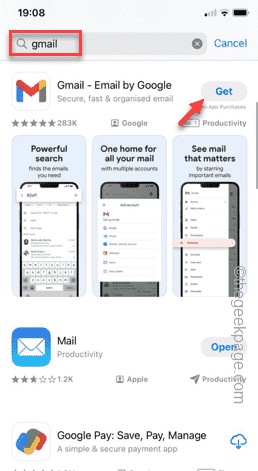
The default map on the iPhone is Maps, Apple's proprietary geolocation provider. Although the map is getting better, it doesn't work well outside the United States. It has nothing to offer compared to Google Maps. In this article, we discuss the feasible steps to use Google Maps to become the default map on your iPhone. How to Make Google Maps the Default Map in iPhone Setting Google Maps as the default map app on your phone is easier than you think. Follow the steps below – Prerequisite steps – You must have Gmail installed on your phone. Step 1 – Open the AppStore. Step 2 – Search for “Gmail”. Step 3 – Click next to Gmail app
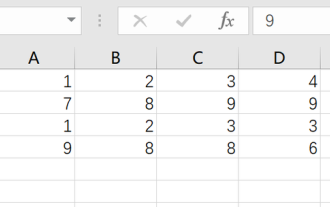
If when opening a file that needs to be printed, we will find that the table frame line has disappeared for some reason in the print preview. When encountering such a situation, we must deal with it in time. If this also appears in your print file If you have questions like this, then join the editor to learn the following course: What should I do if the frame line disappears when printing a table in Excel? 1. Open a file that needs to be printed, as shown in the figure below. 2. Select all required content areas, as shown in the figure below. 3. Right-click the mouse and select the "Format Cells" option, as shown in the figure below. 4. Click the “Border” option at the top of the window, as shown in the figure below. 5. Select the thin solid line pattern in the line style on the left, as shown in the figure below. 6. Select "Outer Border"
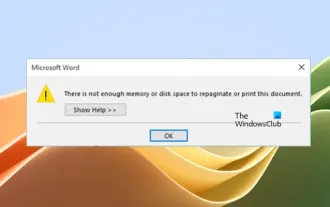
This article will introduce how to solve the problem of insufficient memory or disk space to repage or print the document in Microsoft Word. This error usually occurs when users try to print a Word document. If you encounter a similar error, please refer to the suggestions provided in this article to resolve it. Insufficient memory or disk space to repage or print this document Word error How to resolve the Microsoft Word printing error "There is not enough memory or disk space to repage or print the document." Update Microsoft Office Close memory-hogging applications Change your default printer Start Word in safe mode Rename the NorMal.dotm file Save the Word file as another
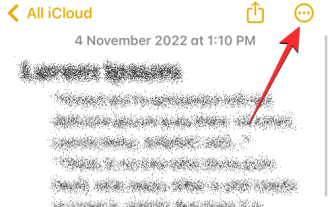
In this digital world, the need for printed pages has not disappeared. While you might think it's more convenient to save content on your computer and send it directly to the printer, you can do the same thing on your iPhone. With your iPhone's camera, you can take a photo or document, and you can also store the file directly for printing at any time. This way you can quickly and easily materialize the information you need and save it in a paper document. Whether at work or in daily life, iPhone provides you with a portable printing solution. The following post will help you understand everything you need to know if you wish to use your iPhone to print pages on a printer. Print from iPhone: Ask Apple
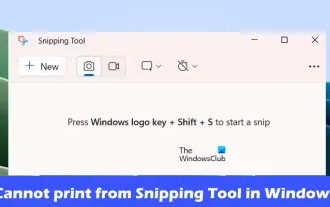
If you are unable to print using the Snipping Tool in Windows 11/10, it may be caused by corrupted system files or driver issues. This article will provide you with solutions to this problem. Can't print from Snipping Tool in Windows 11/10 If you can't print from Snipping Tool in Windows 11/10, use these fixes: Restart PC Printer Clear print queue Update printer and graphics driver Fix or reset Snipping Tool Run SFC and DISM Scan uses PowerShell commands to uninstall and reinstall Snipping Tool. let us start. 1] Restart your PC and printer Restarting your PC and printer helps eliminate temporary glitches
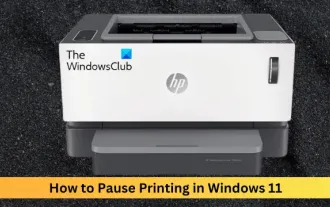
Printed a large file by mistake? Need to stop or pause printing to save ink and paper? There are many situations where you may need to pause an ongoing print job on your Windows 11 device. How to pause printing in Windows 11? In Windows 11, pausing printing will pause the print job, but it will not cancel the print task. This provides users with more flexible control. There are three ways to do this: Pause printing using the taskbar Pausing printing using Windows Settings Printing using the control panel Now, let’s look at these in detail. 1] Print using taskbar Right-click the print queue notification on the taskbar. Click to open all active printer options. Here, right-click on the print job and select Pause All
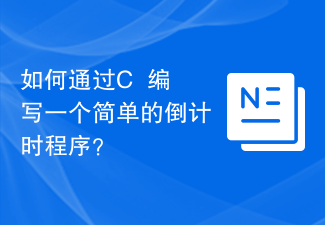
C++ is a widely used programming language that is very convenient and practical in writing countdown programs. Countdown program is a common application that can provide us with very precise time calculation and countdown functions. This article will introduce how to use C++ to write a simple countdown program. The key to implementing a countdown program is to use a timer to calculate the passage of time. In C++, we can use the functions in the time.h header file to implement the timer function. The following is the code for a simple countdown program
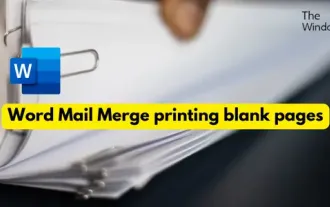
If you find that blank pages appear when printing a mail merge document using Word, this article will help you. Mail merge is a convenient feature that allows you to easily create personalized documents and send them to multiple recipients. In Microsoft Word, the mail merge feature is highly regarded because it helps users save time manually copying the same content for each recipient. In order to print the mail merge document, you can go to the Mailings tab. But some Word users have reported that when trying to print a mail merge document, the printer prints a blank page or doesn't print at all. This may be due to incorrect formatting or printer settings. Try checking the document and printer settings and make sure to preview the document before printing to ensure the content is correct. if
