How to solve the data overflow problem in C++ big data development?
How to solve the data overflow problem in C big data development?
In the process of C big data development, we often encounter the problem of data overflow. Data overflow means that when the value of data exceeds the range that its variable type can represent, it will lead to erroneous results or unpredictable program behavior. In order to solve this problem, we need to take some measures to ensure that the data does not overflow during the calculation process.
1. Select the appropriate data type
In C, the selection of data type is very important to avoid data overflow problems. Based on actual needs, we should choose appropriate data types to store and process data. If you are dealing with a large amount of integer data, you can choose to use the long long
or unsigned long long
type, which can represent a larger range of integers. If you are dealing with floating-point data, you can choose the double
or long double
type, which can represent higher-precision floating-point numbers.
The following is a sample code that demonstrates the use of appropriate data types to avoid data overflow problems:
#include <iostream> #include <limits> int main() { long long a = std::numeric_limits<long long>::max(); long long b = a + 1; std::cout << "a: " << a << std::endl; std::cout << "b: " << b << std::endl; return 0; }
Run the above code, the output result is:
a: 9223372036854775807 b: -9223372036854775808
From the output result It can be seen that when the value of a
is equal to std::numeric_limits<long long>::max()
, that is, 9223372036854775807
, for a
Performs an operation of adding 1, and the value of b
becomes -9223372036854775808
. This is because the maximum value of the long long
type will overflow after adding 1 and become the minimum value
.
2. Range Check
In addition to selecting the appropriate data type, range checking is also an important step to avoid data overflow problems. Before performing numerical calculations, we should first determine whether the input data is within a reasonable range to avoid calculation results exceeding the range of the data type.
The following is a sample code that demonstrates how to perform range checking:
#include <iostream> #include <limits> bool isAdditionSafe(long long a, long long b) { return a > 0 && b > std::numeric_limits<long long>::max() - a; } int main() { long long a, b; std::cout << "Enter a: "; std::cin >> a; std::cout << "Enter b: "; std::cin >> b; if (isAdditionSafe(a, b)) { std::cout << "Addition is safe." << std::endl; } else { std::cout << "Addition is not safe." << std::endl; } return 0; }
Run the above code to determine whether the addition of the input a and b values is safe. If the addition result exceeds the range of the long long
type, Addition is not safe.
is output, otherwise Addition is safe.
is output.
3. Overflow handling
If data overflow inevitably occurs, we can deal with the overflow problem in some ways. A common way to handle this is to use an exception handling mechanism. When an overflow occurs, an exception is thrown and handled accordingly. Another way is to perform appropriate truncation or rounding operations when overflow occurs to ensure the accuracy of the results.
The following is a sample code that demonstrates how to use the exception handling mechanism to handle overflow problems:
#include <iostream> #include <limits> long long safeAdd(long long a, long long b) { if (isAdditionSafe(a, b)) { throw std::overflow_error("Addition overflow"); } return a + b; } int main() { long long a, b; std::cout << "Enter a: "; std::cin >> a; std::cout << "Enter b: "; std::cin >> b; try { long long result = safeAdd(a, b); std::cout << "Addition is safe. Result: " << result << std::endl; } catch (const std::overflow_error& e) { std::cout << "Addition overflow occurred." << std::endl; } return 0; }
In the above code, when the added result exceeds long long
When the scope of the type is exceeded, an exception will be thrown. We can use the try-catch
statement to catch this exception and then handle it accordingly. If an overflow occurs, Addition overflow occurred.
will be output.
Summary:
In C big data development, it is very important to avoid data overflow problems. By selecting appropriate data types, performing range checks, and handling overflows appropriately, we can ensure that the data will not overflow during calculations and obtain correct results. In actual development, we should also optimize and improve according to specific circumstances to ensure the performance and stability of the program.
The above is the detailed content of How to solve the data overflow problem in C++ big data development?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
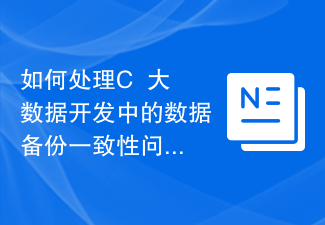
How to deal with the data backup consistency problem in C++ big data development? In C++ big data development, data backup is a very important part. In order to ensure the consistency of data backup, we need to take a series of measures to solve this problem. This article will discuss how to deal with data backup consistency issues in C++ big data development and provide corresponding code examples. Using transactions for data backup Transactions are a mechanism to ensure the consistency of data operations. In C++, we can use the transaction concept in the database to implement data backup.
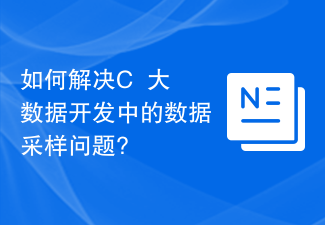
How to solve the data sampling problem in C++ big data development? In C++ big data development, the amount of data is often very large. In the process of processing these big data, a very common problem is how to sample the big data. Sampling is to select a part of sample data from a big data collection for analysis and processing, which can greatly reduce the amount of calculation and increase the processing speed. Below we will introduce several methods to solve the data sampling problem in C++ big data development, and attach code examples. 1. Simple random sampling Simple random sampling is the most common

How to solve the problem of uneven data distribution in C++ big data development? In the C++ big data development process, uneven data distribution is a common problem. When the distribution of data is uneven, it will lead to inefficient data processing or even failure to complete the task. Therefore, solving the problem of uneven data distribution is the key to improving big data processing capabilities. So, how to solve the problem of uneven data distribution in C++ big data development? Some solutions are provided below, along with code examples to help readers understand and practice. Data Sharding Algorithm Data Sharding Algorithm is
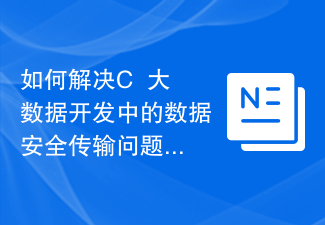
How to solve the problem of data security transmission in C++ big data development? With the rapid development of big data, data security transmission has become an issue that cannot be ignored during the development process. In C++ development, we can ensure the security of data during transmission through encryption algorithms and transmission protocols. This article will introduce how to solve the problem of data security transmission in C++ big data development and provide sample code. 1. Data encryption algorithm C++ provides a rich encryption algorithm library, such as OpenSSL, Crypto++, etc. These libraries can be used
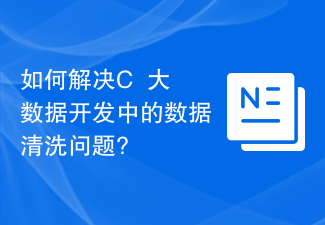
How to solve the data cleaning problem in C++ big data development? Introduction: In big data development, data cleaning is a very important step. Correct, complete, and structured data are the basis for algorithm analysis and model training. This article will introduce how to use C++ to solve data cleaning problems in big data development, and give specific implementation methods through code examples. 1. The concept of data cleaning Data cleaning refers to the preprocessing of original data to make it suitable for subsequent analysis and processing. Mainly includes the following aspects: Missing value processing: deleting or filling missing values
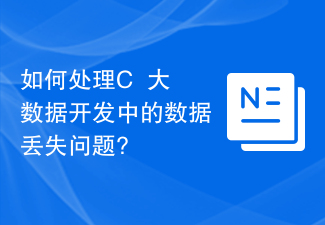
How to deal with the data loss problem in C++ big data development? With the advent of the big data era, more and more companies and developers are beginning to pay attention to big data development. As an efficient and widely used programming language, C++ has also begun to play an important role in big data processing. However, in C++ big data development, the problem of data loss often causes headaches. This article will introduce some common data loss problems and solutions, and provide relevant code examples. Sources of Data Loss Issues Data loss issues can arise from many sources, here are a few

How to solve the data overflow problem in C++ big data development? In the process of C++ big data development, we often encounter the problem of data overflow. Data overflow means that when the value of data exceeds the range that its variable type can represent, it will lead to erroneous results or unpredictable program behavior. In order to solve this problem, we need to take some measures to ensure that the data does not overflow during the calculation process. 1. Choose the appropriate data type In C++, the choice of data type is very important to avoid data overflow problems. According to actual needs, we should
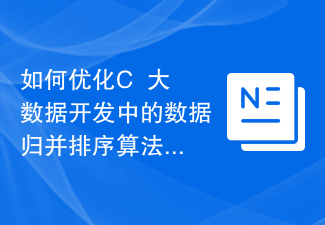
How to optimize the data merging and sorting algorithm in C++ big data development? Introduction: In big data development, data processing and sorting are very common requirements. The data merging and sorting algorithm is an effective sorting algorithm that splits the sorted data and then merges them two by two until the sorting is completed. However, in the case of large data volumes, traditional data merging and sorting algorithms are not very efficient and require a lot of time and computing resources. Therefore, in C++ big data development, how to optimize the data merging and sorting algorithm has become an important task. 1. Background
