


How to perform identity authentication and authorization for Java function development
How to perform identity authentication and authorization for Java function development
In the modern Internet era, identity authentication and authorization are a very important part of software development. Whether it is a website, mobile application or other type of software, the user's identity needs to be authenticated to ensure that only legitimate users can access and use relevant functions. This article will introduce how to use Java to develop identity authentication and authorization functions, and attach code examples.
1. Identity Authentication
Identity authentication is the process of verifying the user's identity to ensure that the identity credentials (such as user name and password) provided by the user are correct. Common identity authentication methods include basic authentication, form authentication, and third-party authentication.
- Basic Authentication
Basic authentication is the simplest form of identity authentication. It base64 encodes the user's account and password and authenticates them stored on the server. Information is compared. The following is an example of using basic authentication:
import java.io.IOException; import java.nio.charset.StandardCharsets; import java.util.Base64; public class BasicAuthenticationExample { public boolean authenticate(String username, String password) { // 模拟从服务器端获取用户存储的账号和密码 String storedUsername = "admin"; String storedPassword = "password"; // 对用户提供的账号和密码进行Base64编码 String encodedUsername = Base64.getEncoder().encodeToString(username.getBytes(StandardCharsets.UTF_8)); String encodedPassword = Base64.getEncoder().encodeToString(password.getBytes(StandardCharsets.UTF_8)); // 比对用户提供的账号和密码与服务器端存储的认证信息 return encodedUsername.equals(Base64.getEncoder().encodeToString(storedUsername.getBytes(StandardCharsets.UTF_8))) && encodedPassword.equals(Base64.getEncoder().encodeToString(storedPassword.getBytes(StandardCharsets.UTF_8))); } public static void main(String[] args) throws IOException { BasicAuthenticationExample example = new BasicAuthenticationExample(); // 模拟用户提供的账号和密码 String username = "admin"; String password = "password"; boolean authenticated = example.authenticate(username, password); System.out.println("身份认证结果:" + authenticated); } }
- Form authentication
Form authentication means that after the user enters the account and password on the login page, the authentication information is entered in the form. The form is submitted to the server for validation. The following is an example of using form authentication:
import java.io.IOException; public class FormAuthenticationExample { public boolean authenticate(String username, String password) { // 模拟从服务器端获取用户存储的账号和密码 String storedUsername = "admin"; String storedPassword = "password"; // 比对用户提供的账号和密码与服务器端存储的认证信息 return username.equals(storedUsername) && password.equals(storedPassword); } public static void main(String[] args) throws IOException { FormAuthenticationExample example = new FormAuthenticationExample(); // 模拟用户提供的账号和密码 String username = "admin"; String password = "password"; boolean authenticated = example.authenticate(username, password); System.out.println("身份认证结果:" + authenticated); } }
- Third-party authentication
Third-party authentication refers to using a third-party platform (such as Google, Facebook, etc.) to verify users identity. Normally, users choose to use a third-party platform to log in in the application, and then pass the obtained authorization information to the server for verification. The following is an example of using Google third-party authentication:
(see official documentation for sample code)
2. Identity authorization
Identity authorization is performed on authenticated users The process of permission management controls the user's access to specific functions based on the user's identity and operation permissions. Common identity authorization methods include role authorization, resource-based authorization and RBAC model.
- Role Authorization
Role authorization refers to assigning users to different roles, and each role has different permissions. The following is an example of using role authorization:
(see official documentation for sample code)
- Resource-based authorization
Resource-based authorization refers to Control based on user access to specific resources. The following is an example of using resource-based authorization:
(see official documentation for sample code)
- RBAC model
RBAC (Role-Based Access Control) model is a common identity authorization model that clearly defines and manages the relationship between users, roles, and permissions. The following is an example of using the RBAC model for identity authorization:
(See official documentation for sample code)
Identity authentication and authorization are an essential part of software development. Through the introduction of this article, I believe readers can understand how to use Java to develop identity authentication and authorization functions, and be able to choose appropriate identity authentication and authorization methods according to specific needs. Code examples can help readers better understand and practice related functional development.
The above is the detailed content of How to perform identity authentication and authorization for Java function development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
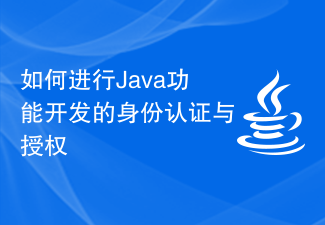
How to perform identity authentication and authorization for Java function development In the modern Internet era, identity authentication and authorization are a very important part of software development. Whether it is a website, mobile application or other type of software, the user's identity needs to be authenticated to ensure that only legitimate users can access and use relevant functions. This article will introduce how to use Java to develop identity authentication and authorization functions, and attach code examples. 1. Identity Authentication Identity authentication is the process of verifying the identity of a user to ensure that the identity credentials provided by the user (such as user name and
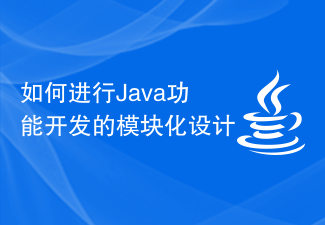
How to carry out modular design for Java function development Introduction: In the software development process, modular design is an important way of thinking. It divides a complex system into multiple independent modules, each with clear functions and responsibilities. In this article, we will discuss how to implement modular design for Java function development and give corresponding code examples. 1. Advantages of modular design Modular design has the following advantages: Improve code reusability: different modules can be reused in different projects, reducing the need for repeated development
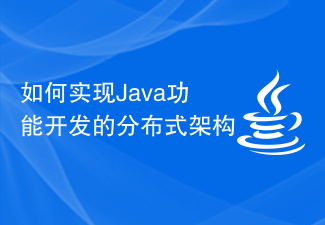
How to implement distributed architecture for Java function development. In today's era of rapid development of information technology, distributed architecture has become the first choice for major enterprises to develop systems. The distributed architecture improves the performance and scalability of the system by distributing different functional modules of the system to run on different servers. This article will introduce how to use Java to implement functional development of distributed architecture and provide corresponding code examples. 1. Build a distributed environment. Before starting function development, we first need to build a distributed environment. A distributed environment consists of multiple servers
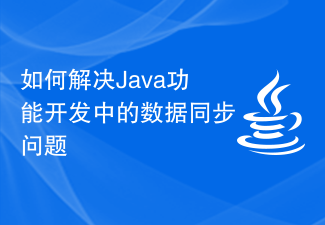
How to solve the data synchronization problem in Java function development. Data synchronization is a common problem in Java function development. When multiple threads access shared data at the same time, data inconsistency may occur. To solve this problem, we can use various synchronization mechanisms and technologies to ensure data consistency and correctness. 1. Use the synchronized keyword The synchronized keyword is the most basic synchronization mechanism in Java and can be used to modify methods or code blocks. its work
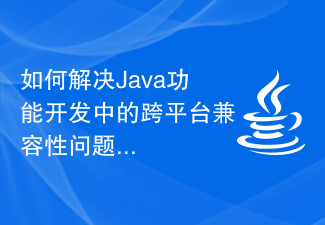
How to solve the cross-platform compatibility problem in Java function development. With the popularity of the Java language and the expansion of its application scope, a very important problem is often faced when developing Java programs, that is, the cross-platform compatibility problem. Since different operating systems have different implementations of Java virtual machines, various problems may occur when the same Java code is run on different platforms. This article describes some common cross-platform compatibility issues and provides corresponding solutions and code examples. 1. Encoding issues on different operating systems
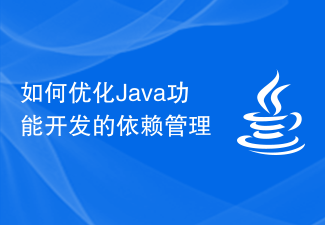
How to optimize dependency management for Java function development Introduction: In Java development, dependency management is an important aspect. Good dependency management can promote code maintainability and scalability, while also improving development efficiency. This article will introduce some methods to optimize dependency management in Java function development and provide code examples. 1. Use build tools Using build tools is the preferred method for managing dependencies. Currently, the more popular build tools include Maven and Gradle. Build tools can automatically download and manage project dependencies, and
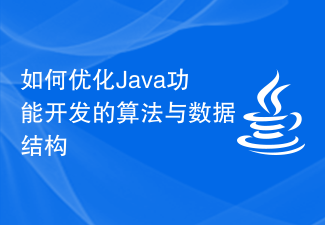
How to optimize algorithms and data structures for Java function development Introduction: In software development, algorithms and data structures are two important aspects. Their performance directly affects the running speed and resource consumption of the program. For Java developers, how to optimize algorithms and data structures is an issue that cannot be ignored. This article will introduce some common algorithm and data structure optimization techniques and illustrate them through code examples. 1. Select the appropriate data structure Choosing the appropriate data structure is the first step in optimizing the algorithm. Common data structures include arrays, linked lists,
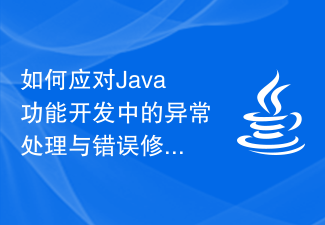
How to deal with exception handling and error repair in Java function development Summary: In the process of Java function development, exception handling and error repair are very important. This article will introduce how to effectively deal with exception handling and error repair in Java development, and explain in detail through code examples. The Importance of Exception Handling In Java development, exceptions are inevitable. When errors or exceptions occur during code execution, without appropriate handling, the program may crash or produce unpredictable results. The purpose of exception handling is
