Use PHP and XML to parse and process data
Use PHP and XML to parse and process data
With the continuous development of the Internet, data processing has become more and more important. As a standard data format, XML is widely used in data exchange and storage. In PHP, we can use the built-in XML extension to parse and process XML data.
PHP provides DOM extension and SimpleXML extension to process XML data. DOM extension is a standard XML processing method that parses and manipulates XML data by building a model tree of the entire XML document; while SimpleXML extension is a simpler XML processing method that can directly access XML elements and attributes.
Next, let’s go through some code examples to understand how to use PHP and XML to parse and process data.
- Use DOM extension to parse XML data
// 加载XML文件 $xml = new DOMDocument(); $xml->load('data.xml'); // 获取根节点 $root = $xml->documentElement; // 遍历子节点 $children = $root->childNodes; foreach ($children as $child) { // 判断节点类型为元素节点 if ($child instanceof DOMElement) { // 获取节点名称 $nodeName = $child->nodeName; echo "节点名称:$nodeName "; // 获取节点属性 $attributes = $child->attributes; foreach ($attributes as $attr) { $attrName = $attr->nodeName; $attrValue = $attr->nodeValue; echo "属性名称:$attrName,属性值:$attrValue "; } // 获取节点文本内容 $nodeValue = $child->nodeValue; echo "节点文本内容:$nodeValue "; echo "-------------------------------- "; } }
- Use SimpleXML extension to parse XML data
// 加载XML文件 $xml = simplexml_load_file('data.xml'); // 遍历子节点 foreach($xml->children() as $child) { // 获取节点名称 $nodeName = $child->getName(); echo "节点名称:$nodeName "; // 获取节点属性 $attributes = $child->attributes(); foreach ($attributes as $attrName => $attrValue) { echo "属性名称:$attrName,属性值:$attrValue "; } // 获取节点文本内容 $nodeValue = $child->__toString(); echo "节点文本内容:$nodeValue "; echo "-------------------------------- "; }
The above is using PHP and XML Basic methods to parse and process data. Whether using DOM extension or SimpleXML extension, we can easily parse and process XML data to achieve rich functions. Hope this article is helpful to you!
The above is the detailed content of Use PHP and XML to parse and process data. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
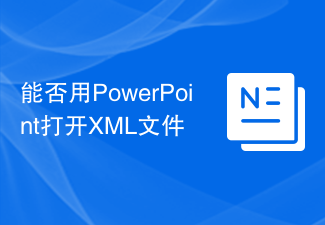
Can XML files be opened with PPT? XML, Extensible Markup Language (Extensible Markup Language), is a universal markup language that is widely used in data exchange and data storage. Compared with HTML, XML is more flexible and can define its own tags and data structures, making the storage and exchange of data more convenient and unified. PPT, or PowerPoint, is a software developed by Microsoft for creating presentations. It provides a comprehensive way of
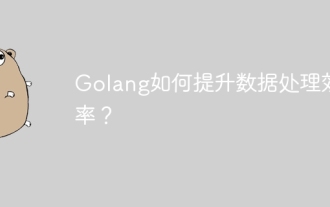
Golang improves data processing efficiency through concurrency, efficient memory management, native data structures and rich third-party libraries. Specific advantages include: Parallel processing: Coroutines support the execution of multiple tasks at the same time. Efficient memory management: The garbage collection mechanism automatically manages memory. Efficient data structures: Data structures such as slices, maps, and channels quickly access and process data. Third-party libraries: covering various data processing libraries such as fasthttp and x/text.
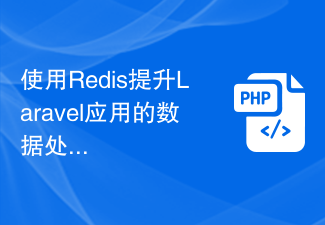
Use Redis to improve the data processing efficiency of Laravel applications. With the continuous development of Internet applications, data processing efficiency has become one of the focuses of developers. When developing applications based on the Laravel framework, we can use Redis to improve data processing efficiency and achieve fast access and caching of data. This article will introduce how to use Redis for data processing in Laravel applications and provide specific code examples. 1. Introduction to Redis Redis is a high-performance memory data
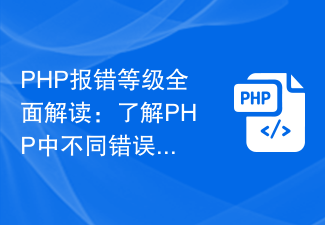
Comprehensive interpretation of PHP error levels: To understand the meaning of different error levels in PHP, specific code examples are required. During the PHP programming process, various errors are often encountered. It is very important for developers to understand the levels of these errors and what they mean. PHP provides seven different error reporting levels, each with its own specific meaning and impact. In this article, we will provide a comprehensive explanation of PHP error levels and provide specific code examples to help readers better understand these errors. E_ERROR(1
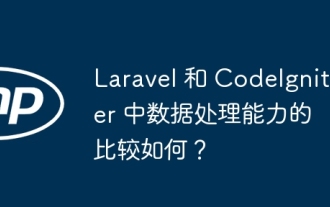
Compare the data processing capabilities of Laravel and CodeIgniter: ORM: Laravel uses EloquentORM, which provides class-object relational mapping, while CodeIgniter uses ActiveRecord to represent the database model as a subclass of PHP classes. Query builder: Laravel has a flexible chained query API, while CodeIgniter’s query builder is simpler and array-based. Data validation: Laravel provides a Validator class that supports custom validation rules, while CodeIgniter has less built-in validation functions and requires manual coding of custom rules. Practical case: User registration example shows Lar
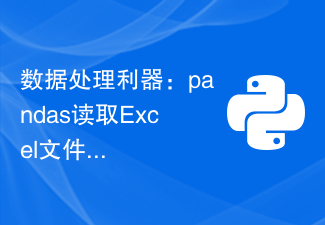
With the increasing popularity of data processing, more and more people are paying attention to how to use data efficiently and make the data work for themselves. In daily data processing, Excel tables are undoubtedly the most common data format. However, when a large amount of data needs to be processed, manually operating Excel will obviously become very time-consuming and laborious. Therefore, this article will introduce an efficient data processing tool - pandas, and how to use this tool to quickly read Excel files and perform data processing. 1. Introduction to pandas pandas
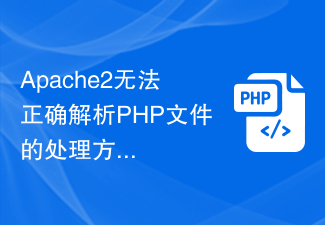
Due to space limitations, the following is a brief article: Apache2 is a commonly used web server software, and PHP is a widely used server-side scripting language. In the process of building a website, sometimes you encounter the problem that Apache2 cannot correctly parse the PHP file, causing the PHP code to fail to execute. This problem is usually caused by Apache2 not configuring the PHP module correctly, or the PHP module being incompatible with the version of Apache2. There are generally two ways to solve this problem, one is
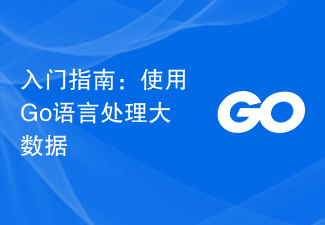
As an open source programming language, Go language has gradually received widespread attention and use in recent years. It is favored by programmers for its simplicity, efficiency, and powerful concurrent processing capabilities. In the field of big data processing, the Go language also has strong potential. It can be used to process massive data, optimize performance, and can be well integrated with various big data processing tools and frameworks. In this article, we will introduce some basic concepts and techniques of big data processing in Go language, and show how to use Go language through specific code examples.
