


How to implement user authentication function in Yii framework
Method to implement user authentication function in Yii framework
Yii is a powerful PHP framework that provides developers with a series of tools and components to simplify the development process. One of the important functions is user authentication, which determines whether the user is legally logged into the system. This article will introduce how to implement user authentication function in Yii framework and provide code examples.
- Create user authentication model
First, we need to create a user authentication model to handle user login verification logic. In the Yii framework, we can use the yiiwebUser class to implement user authentication functions. The following is an example User model code:
namespace appmodels; use yiidbActiveRecord; use yiiwebIdentityInterface; class User extends ActiveRecord implements IdentityInterface { // 用户认证相关的属性和方法 /** * @inheritdoc */ public static function tableName() { return 'user'; // 数据库中存储用户信息的表名 } /** * @inheritdoc */ public static function findIdentity($id) { return static::findOne($id); // 根据用户ID查找用户信息 } /** * @inheritdoc */ public static function findIdentityByAccessToken($token, $type = null) { // 根据用户Token查找用户信息 throw new NotSupportedException('"findIdentityByAccessToken" is not implemented.'); } /** * @inheritdoc */ public function getId() { return $this->id; // 返回用户ID } /** * @inheritdoc */ public function getAuthKey() { return $this->auth_key; // 返回用户认证密钥 } /** * @inheritdoc */ public function validateAuthKey($authKey) { return $this->auth_key === $authKey; // 验证用户认证密钥是否有效 } /** * 根据用户名查找用户信息 * @param $username * @return static */ public static function findByUsername($username) { return static::findOne(['username' => $username]); } /** * 验证用户密码 * @param $password * @return bool */ public function validatePassword($password) { return Yii::$app->security->validatePassword($password, $this->password_hash); } }
In the above code, we implemented the IdentityInterface interface and overridden the related methods. These methods are mainly used to find user information and verify user identity based on user ID, authentication key and other information.
- Configure the user authentication component
Next, we need to configure the user authentication component so that the Yii framework can automatically authenticate when the user logs in. In the Yii framework, user authentication components are defined through configuration files. Open the config/web.php file and add the following code:
'components' => [ // ... 'user' => [ 'identityClass' => 'appmodelsUser', 'enableAutoLogin' => true, ], // ... ],
In the above code, we set 'identityClass' to the User class we just created, and 'enableAutoLogin' is set to true to enable the automatic login function.
- Complete the user login function
Now, we can use the user authentication function provided by Yii in the controller or view. The following is a code example of a simple user login function:
namespace appcontrollers; use Yii; use yiiwebController; use appmodelsLoginForm; class UserController extends Controller { // ... public function actionLogin() { $model = new LoginForm(); if ($model->load(Yii::$app->request->post()) && $model->login()) { // 登录成功跳转到首页 return $this->goHome(); } else { // 显示登录表单 return $this->render('login', [ 'model' => $model, ]); } } // ... }
In the above code, we created an actionLogin method in the UserController controller to handle the user's login request. By calling the load method, we can load the login form data submitted by the user into the LoginForm model. Then, perform user login authentication by calling the login method. If the login is successful, we redirect the user to the home page; if the login fails, we display the login form view.
- Create a login form model
Finally, we need to create a login form model to handle the validation and processing of user login form data. The following is a code example of a simple LoginForm model:
namespace appmodels; use Yii; use yiiaseModel; class LoginForm extends Model { public $username; public $password; public $rememberMe = true; private $_user = false; /** * @return array the validation rules. */ public function rules() { return [ [['username', 'password'], 'required'], ['rememberMe', 'boolean'], ['password', 'validatePassword'], ]; } /** * Validates the password. * This method serves as the inline validation for password. * * @param string $attribute the attribute currently being validated * @param array $params the additional name-value pairs given in the rule */ public function validatePassword($attribute, $params) { if (!$this->hasErrors()) { $user = $this->getUser(); if (!$user || !$user->validatePassword($this->password)) { $this->addError($attribute, 'Incorrect username or password.'); } } } /** * Logs in a user using the provided username and password. * @return bool whether the user is logged in successfully */ public function login() { if ($this->validate()) { return Yii::$app->user->login($this->getUser(), $this->rememberMe ? 3600*24*30 : 0); } else { return false; } } /** * Finds user by [[username]] * * @return User|null */ protected function getUser() { if ($this->_user === false) { $this->_user = User::findByUsername($this->username); } return $this->_user; } }
In the above code, we create a LoginForm model and define some properties and methods. The rules method defines the verification rules of the login form, the validatePassword method is used to verify the password entered by the user, the login method is used for logical processing of user login, and the getUser method is used to find user information based on the user name.
So far, we have successfully implemented the user authentication function in the Yii framework. Users can log in by accessing the actionLogin method in UserController. After successful login, they will jump to the specified page.
Summary
This article introduces the method of implementing user authentication function in Yii framework. By creating a user authentication model, configuring user authentication components, implementing the login function and creating a login form model, we can easily implement the user authentication function in the Yii framework. Of course, the user authentication function can also be further expanded and customized in combination with RBAC permission control and other functions to meet specific business needs.
The above is the detailed content of How to implement user authentication function in Yii framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
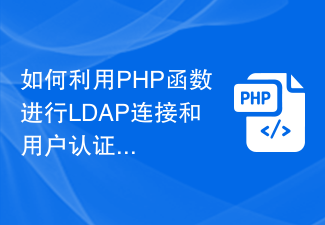
How to use PHP functions for LDAP connection and user authentication? LDAP (Lightweight Directory Access Protocol) is a protocol for accessing and maintaining distributed directory information. In web applications, LDAP is often used for user authentication and authorization. PHP provides a series of functions to implement LDAP connection and user authentication. Let's take a look at how to use these functions. Connecting to the LDAP server To connect to the LDAP server, we can use the ldap_connect function. The following is a connection to the LDAP server
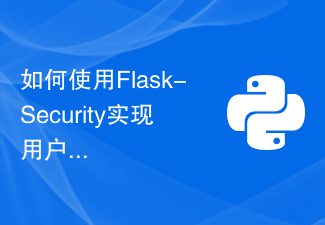
How to use Flask-Security to implement user authentication and authorization Introduction: In modern web applications, user authentication and authorization are essential functions. To simplify this process, Flask-Security is a very useful extension that provides a series of tools and functions to make user authentication and authorization simple and convenient. This article will introduce how to use Flask-Security to implement user authentication and authorization. 1. Install the Flask-Security extension: at the beginning
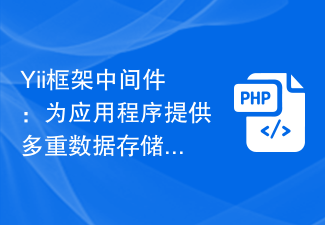
Yii framework middleware: providing multiple data storage support for applications Introduction Middleware (middleware) is an important concept in the Yii framework, which provides multiple data storage support for applications. Middleware acts like a filter, inserting custom code between an application's requests and responses. Through middleware, we can process, verify, filter requests, and then pass the processed results to the next middleware or final handler. Middleware in the Yii framework is very easy to use
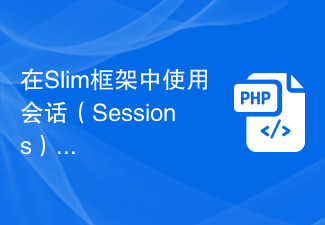
Method of using sessions (Sessions) for user authentication in the Slim framework In web applications, user authentication is an important function, which ensures that only authorized users can access restricted resources. Sessions are a commonly used authentication method that ensures that users remain authenticated throughout the session by storing user identity and status information. The Slim framework provides convenient tools and middleware to handle sessions and user authentication. Below we will introduce how to use sessions in the Slim framework
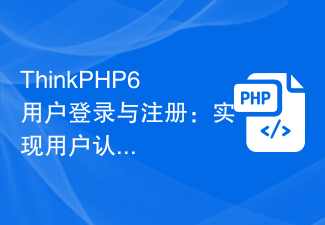
ThinkPHP6 user login and registration: implementing user authentication function Introduction: User login and registration is one of the common requirements of most web applications. In ThinkPHP6, user login and registration operations can be easily realized by using the built-in user authentication function. This article will introduce how to implement user authentication function in ThinkPHP6, and attach code examples. 1. Introduction to user authentication function User authentication refers to the process of verifying user identity. In web applications, user authentication usually involves user login
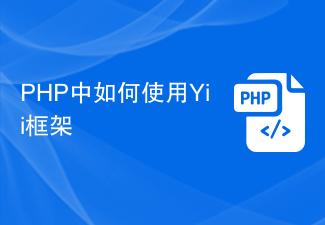
With the rapid development of web applications, modern web development has become an important skill. Many frameworks and tools are available for developing efficient web applications, among which the Yii framework is a very popular framework. Yii is a high-performance, component-based PHP framework that uses the latest design patterns and technologies, provides powerful tools and components, and is ideal for building complex web applications. In this article, we will discuss how to use Yii framework to build web applications. Install Yii framework first,
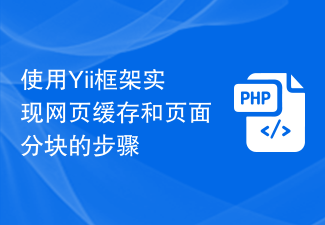
Steps to implement web page caching and page chunking using the Yii framework Introduction: During the web development process, in order to improve the performance and user experience of the website, it is often necessary to cache and chunk the page. The Yii framework provides powerful caching and layout functions, which can help developers quickly implement web page caching and page chunking. This article will introduce how to use the Yii framework to implement web page caching and page chunking. 1. Turn on web page caching. In the Yii framework, web page caching can be turned on through the configuration file. Open the main configuration file co
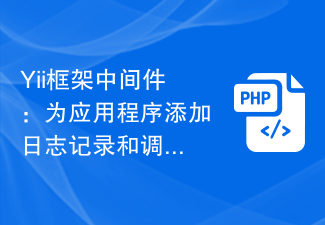
Yii framework middleware: Add logging and debugging capabilities to applications [Introduction] When developing web applications, we usually need to add some additional features to improve the performance and stability of the application. The Yii framework provides the concept of middleware that enables us to perform some additional tasks before and after the application handles the request. This article will introduce how to use the middleware function of the Yii framework to implement logging and debugging functions. [What is middleware] Middleware refers to the processing of requests and responses before and after the application processes the request.
