


Analysis of application scenarios of Goroutines in Golang concurrent programming practice
Application scenario analysis of Goroutines in Golang concurrent programming practice
Introduction:
With the continuous improvement of computer performance, multi-core processors have become mainstream. In order to make full use of the advantages of multi-core processors, we Concurrent programming techniques need to be used to implement multi-threaded operations. In the Go language, Goroutines (coroutines) are a very powerful concurrent programming mechanism that can be used to achieve efficient concurrent operations. In this article, we will explore the application scenarios of Goroutines and give some sample codes.
Application Scenario 1: Concurrent Task Processing
Goroutines can easily implement concurrent task processing, especially when multiple user requests need to be processed at the same time or batch data processing needs to be performed, Goroutines can Greatly improve the system's response speed. The following is a simple sample code:
package main import ( "fmt" "time" ) func doTask(taskID int) { // 模拟任务的耗时操作 time.Sleep(time.Second) fmt.Println("Task", taskID, "is done") } func main() { for i := 0; i < 10; i++ { go doTask(i) } // 等待所有任务完成 time.Sleep(time.Second * 10) }
In the above sample code, we use a loop to start 10 Goroutines and call the doTask function to perform each task. Each task sleeps for 1 second to simulate processing time. Through the concurrent execution of Goroutines, all tasks can be executed simultaneously instead of sequentially. Finally, we use the time.Sleep function to wait for all tasks to complete to ensure that the program does not exit early.
Application Scenario 2: Concurrent Data Processing
Goroutines are also very suitable for concurrent data processing tasks, especially in the case of large amounts of data, which can effectively increase the processing speed. The following is a sample code:
package main import ( "fmt" "sync" ) func process(data int, wg *sync.WaitGroup) { defer wg.Done() // 模拟数据处理耗时 data++ fmt.Println("Processed data:", data) } func main() { var wg sync.WaitGroup for i := 0; i < 10; i++ { wg.Add(1) go process(i, &wg) } // 等待所有数据处理完成 wg.Wait() }
In the above sample code, we define a process function to process data. It will add 1 to each data and print out the processed results. In the main function, we use sync.WaitGroup to wait for all Goroutines to complete. Through parallel processing of Goroutines, all data processing can be performed at the same time, greatly improving the processing speed.
Application Scenario 3: Concurrent Network Communication
Goroutines are an ideal mechanism for handling network communication in the Go language. Especially in server programming, they can handle multiple client requests at the same time and improve the system's efficiency. Concurrency capabilities. The following is a simple sample code:
package main import ( "fmt" "net/http" ) func handleRequest(w http.ResponseWriter, r *http.Request) { // 处理HTTP请求 fmt.Fprintf(w, "Hello, World!") } func main() { http.HandleFunc("/", handleRequest) err := http.ListenAndServe(":8080", nil) if err != nil { fmt.Println("Server error:", err) } }
In the above sample code, we used the http package to create an HTTP server. By using Goroutines, we can process multiple requests simultaneously instead of processing them one by one in sequence every time a client request is received. This enables our server to handle multiple requests at the same time, improving the system's concurrent processing capabilities.
Summary:
Goroutines are one of the core mechanisms for implementing concurrent programming in the Go language. They can be used in various application scenarios, including concurrent task processing, concurrent data processing, and concurrent network communication. Through concurrent programming, we can make full use of the advantages of multi-core processors and improve the system's response speed and concurrent processing capabilities. In practical applications, we need to carefully design the number and scheduling methods of Goroutines to avoid problems such as resource competition and deadlock. I hope this article will help you understand the application scenarios of Goroutines, and I also hope it will encourage you to try concurrent programming techniques in practice.
The above is the detailed content of Analysis of application scenarios of Goroutines in Golang concurrent programming practice. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
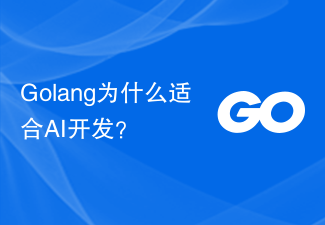
Why is Golang suitable for AI development? With the rapid development of artificial intelligence (AI) technology, more and more developers and researchers have begun to pay attention to the potential of using the Golang programming language in the field of AI. Golang (also known as Go) is an open source programming language developed by Google. It is loved by developers for its high performance, high concurrency and simplicity and ease of use. This article will explore why Golang is suitable for AI development and provide some sample code to demonstrate Golang's advantages in the AI field. High sex
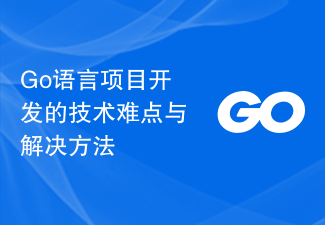
Technical Difficulties and Solutions in Go Language Project Development With the popularization of the Internet and the development of informatization, the development of software projects has received more and more attention. Among many programming languages, Go language has become the first choice of many developers because of its powerful performance, efficient concurrency capabilities and simple and easy-to-learn syntax. However, there are still some technical difficulties in the development of Go language projects. This article will explore these difficulties and provide corresponding solutions. 1. Concurrency control and race conditions The concurrency model of Go language is called "goroutine", which makes

Golang Development: Building a Distributed File Storage System In recent years, with the rapid development of cloud computing and big data, the demand for data storage has continued to increase. In order to cope with this trend, distributed file storage systems have become an important technical direction. This article will introduce how to build a distributed file storage system using the Golang programming language and provide specific code examples. 1. Design of distributed file storage system A distributed file storage system is a system that stores file data dispersedly on multiple machines. It divides the data into multiple blocks.
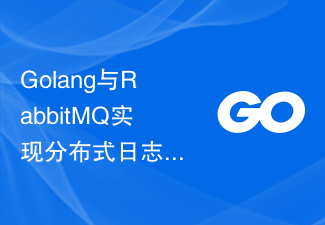
Details, techniques, and best practices for implementing distributed log collection and analysis with Golang and RabbitMQ. In recent years, with the popularity of microservice architecture and the complexity of large-scale systems, log collection and analysis have become more and more important. In a distributed system, the logs of each microservice are often scattered in different places. How to efficiently collect and analyze these logs becomes a challenge. This article will introduce the details, techniques, and best practices on how to use Golang and RabbitMQ to implement distributed log collection and analysis. Ra
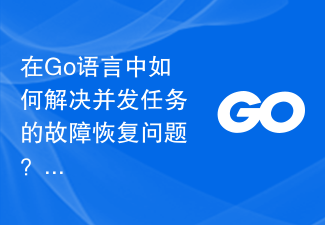
How to solve the problem of failure recovery of concurrent tasks in Go language? In modern software development, the use of concurrent processing can significantly improve the performance of the program. In the Go language, we can achieve efficient concurrent task processing by using goroutine and channels. However, concurrent tasks also bring some new challenges, such as handling failure recovery. This article will introduce some methods to solve the problem of concurrent task failure recovery in Go language and provide specific code examples. Error handling in concurrent tasks When processing concurrent tasks,
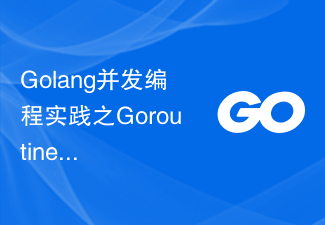
Introduction to the application scenario analysis of Goroutines in Golang concurrent programming practice: With the continuous improvement of computer performance, multi-core processors have become mainstream. In order to make full use of the advantages of multi-core processors, we need to use concurrent programming technology to implement multi-threaded operations. In the Go language, Goroutines (coroutines) are a very powerful concurrent programming mechanism that can be used to achieve efficient concurrent operations. In this article, we will explore the application scenarios of Goroutines and give some examples.
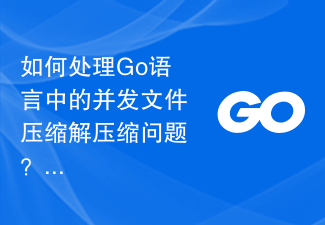
How to deal with concurrent file compression and decompression in Go language? File compression and decompression is one of the tasks frequently encountered in daily development. As file sizes increase, compression and decompression operations can become time-consuming, so concurrency becomes an important means of improving efficiency. In the Go language, you can use the features of goroutine and channel to implement concurrent processing of file compression and decompression operations. File compression First, let's take a look at how to implement file compression in the Go language. Go language standard
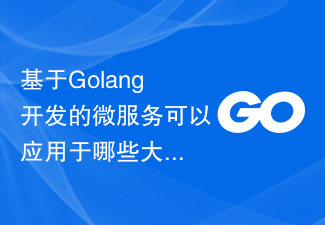
What large-scale systems can microservices developed based on Golang be applied to? Microservice architecture has become one of the popular development models today. It takes individual, independent services as its core and communicates with each other to build a large-scale system. As an efficient and reliable programming language, Golang has the characteristics of excellent concurrency performance, simplicity and ease of use, so it is very suitable for developing microservices. So, what large-scale systems can microservices developed based on Golang be applied to? Below I will look at it from different angles
