The logical process of Java development object-oriented programming (OOAD)
Java is widely used in object-oriented programming (OOAD). OOAD is an idea or method that establishes a connection between the real world and computer programs by abstracting real-world things into objects. This idea can improve developer efficiency, reduce code redundancy, and make programs easier to maintain and expand. This article will introduce the logical process of object-oriented programming in Java development.
- Identify the problem
OOAD first needs to identify the problem, that is, the goals and requirements of the problem. To complete this process, developers need to develop a deep understanding of their users and target audience in order to determine the problem that needs to be solved and the end goal they wish to achieve.
- Identify Objects
Once the problem is identified, developers can begin to identify the objects in the problem. In Java, an object can be viewed as a wrapper type with properties and methods. For example, in an employee management system, objects can be concepts such as employees and departments.
- Definition class
Definition class is the core part of OOAD. A class is a template that describes the properties and methods of an object. In Java, developers define a class through the keyword "class". For example:
class Employee {
//Attribute definition
String name;
int age;
double salary;
//Method definition
void work() {
System.out.println(name + " is working.");
}
}
In this example, "Employee" is a class with three attributes: name (a name of string type), age (an integer) type age) and salary (a double precision floating point type salary). In addition, it has a method called "work", which outputs the employee's name and indicates that the employee is working.
- Building relationships
Building relationships is a crucial step, which describes the connections between different classes. There are three types of relationships in Java: inheritance, implementation and aggregation. Inheritance is a relationship between subclasses and superclasses that describes how one class extends the capabilities of another class through inheritance. For example:
class Manager extends Employee {
// Attribute definition
String department;
// Method definition
void manage() {
System.out.println(name + " is managing " + department);
}
}
In this example, the Manager class inherits all properties and methods of the Employee class, and adds an attribute named department, which represents the department managed by the manager. The Manager class also has a method called "manage", which will output the name of the manager and the department he manages.
Implementation is a connection between an interface and a class. It describes how a class implements a given set of methods to meet the needs of an interface. For example:
interface Account {
// Method definition
double getBalance();
void deposit(double amount);
}
class SavingsAccount implements Account {
// Property definition
double balance;
// Method definition
public double getBalance() {
return balance;
}
public void deposit(double amount) {
balance += amount;
}
}
In this example, Account is an interface that defines two methods - "getBalance" and "deposit". The SavingsAccount class implements all methods of the Account interface and has a double-precision floating-point property called balance.
Aggregation is a connection between objects, which represents a whole composed of many parts. For example:
class Department {
// Attribute definition
String name;
List
// Method definition
void addEmployee(Employee employee) {
employees.add(employee);
}
}
In this example, the Department class has a property called "employees", which consists of many Employee objects. It also has a method called "addEmployee" which is used to add an Employee object to the employees list.
- Writing the Code
Once the classes and relationships are determined, it’s time to start writing the code. Java code should be written in an object-oriented manner, especially when using OOAD. When writing OOAD code, it's a good practice to write methods or functions that are reusable and short.
- Debugging and Testing
Finally, debugging and testing are critical parts of ensuring your code functions correctly. In Java applications, you can use a debugger to trace code and find errors. In addition, there are various testing frameworks and methods in Java that can help developers write test cases and test code.
In general, the logical process of object-oriented programming in Java development includes a series of steps such as identifying problems, identifying objects, defining classes, establishing relationships, writing code, debugging and testing. Understanding these steps can help developers write better Java code and improve code readability, maintainability, and scalability.
The above is the detailed content of The logical process of Java development object-oriented programming (OOAD). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
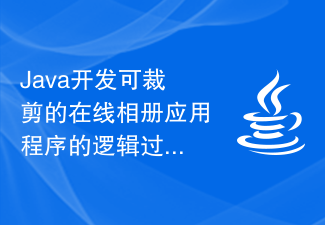
The logical process of developing a croppable online photo album application in Java In the digital age, mobile phone cameras have become the main device for more and more people to record their lives and commemorate precious moments. As the number of photos continues to increase, an easy-to-use photo album management tool has become an urgent need. This article will introduce how to develop a croppable online photo album application using Java. 1. Requirements analysis Before starting development, a needs analysis needs to be performed. Based on user needs, we can determine that this online photo album application needs to have the following functions: Users can
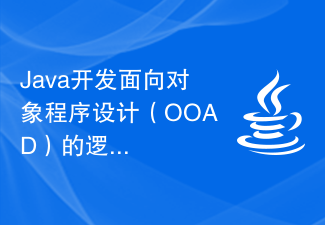
Java is widely used in object-oriented programming (OOAD). OOAD is an idea or method that establishes a connection between the real world and computer programs by abstracting real-world things into objects. This idea can improve developer efficiency, reduce code redundancy, and make programs easier to maintain and expand. This article will introduce the logical process of object-oriented programming in Java development. Identifying the problem OOAD first needs to identify the problem, that is, the goals and requirements of the problem. To complete this process, developers
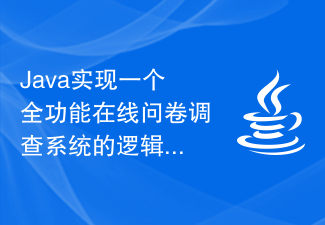
With the development of the Internet, more and more companies, schools and organizations need to conduct questionnaire surveys to collect user feedback, understand market demand, surveyor satisfaction, etc. In this case, a full-featured online questionnaire survey system can be implemented through Java programming to make the questionnaire survey more convenient and efficient. This article will introduce the logical process of implementing an online questionnaire system in Java. System Requirements Analysis Before developing an online questionnaire system, we need to conduct system requirements analysis to clarify the functions and characteristics of the system. First, this system requires
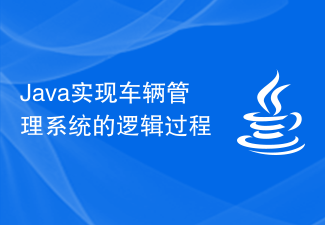
Java is a programming language widely used in the field of software development. Its simplicity, ease of learning, and cross-platform characteristics make it the first choice of many program developers. This article will introduce how to use Java language to implement the logical process of the vehicle management system. 1. Requirements analysis First, we need to clarify the requirements of the vehicle management system so that we can better grasp the logical relationships and processes of the entire system in subsequent design and development. Based on the common vehicle management systems on the market, we analyze the needs of vehicle management systems as follows: 1. Vehicle information maintenance
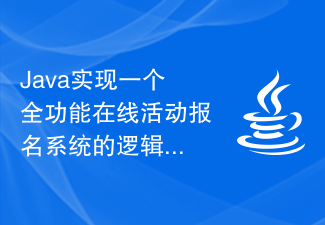
The logical process of implementing a full-featured online event registration system in Java. With the continuous development of society, people's lifestyles are also constantly changing. Some new forms of activities, such as online activities and online learning, are becoming more and more popular among people. For these activities, a fully functional online registration system is very necessary. As an efficient and safe programming language, Java can achieve this purpose very well. In this article, we will introduce the logical process of implementing a full-featured online event registration system in Java. 1. Demand points
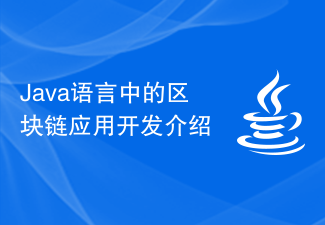
As blockchain technology becomes increasingly mature and widely used, more and more developers are beginning to pay attention to the development of blockchain. As one of the most widely used programming languages, Java language has gradually become an important choice for developing blockchain applications. This article will introduce in detail the content related to blockchain application development in Java language, including implementation principles, development frameworks and tools, to help developers better understand and use Java for blockchain development. 1. Principles of blockchain technology Before understanding the development of blockchain applications in Java language, we need to first understand
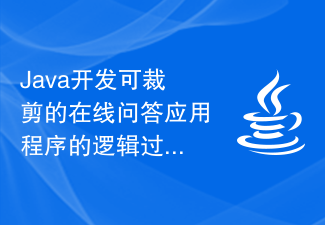
The logical process of developing a tailorable online question and answer application in Java. With the rapid development of the Internet, people's need to obtain information is becoming more and more urgent. The emergence of online question and answer applications meets users' needs for knowledge learning and problem solving. Therefore, it is of great practical significance to develop a tailorable online question and answer application. The following will introduce the logical process of developing a tailorable online question and answer application in Java. 1. Requirements analysis Before starting development, we need to conduct an in-depth analysis of the application's functions. First, ask
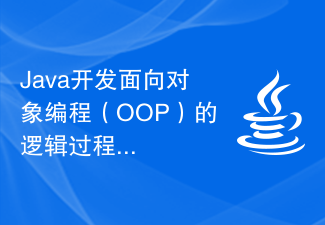
Java is an object-oriented programming language (Object-Oriented Programming, OOP), and the object-oriented programming idea is a software development method, the core of which is object-based programming. Object-oriented programming can help programmers better organize and manage code, and improve code reusability, maintainability and scalability. In this article, we will help readers better understand OOP ideas and the Java language by introducing the logical process of object-oriented programming in Java development.
