


How to use PHP to develop data visualization and chart display modules in CMS
With the development of the Internet, website construction and management have become more and more important, especially content management systems (CMS). CMS not only allows website administrators to easily manage the content of the website, but also allows website users to easily obtain the information they need. In CMS, data visualization and chart display are very important modules, they can help us better understand and display data. This article will introduce how to use PHP to develop data visualization and chart display modules in CMS.
1. Choose the appropriate chart library and framework
To develop the data visualization and chart display module in CMS, we first need to choose the appropriate chart library and framework. Currently, popular chart libraries on the market include Chart.js, Highcharts, D3.js, etc., while frameworks include Bootstrap, Semantic UI, etc.
Chart.js is an easy-to-use JavaScript chart library that provides multiple types of charts, including linear charts, bar charts, pie charts, etc. It supports responsive layout and can adapt to various screen sizes.
Highcharts is a powerful JavaScript chart library that provides multiple types of charts, including curve charts, bar charts, scatter charts, etc. It supports advanced features such as dynamically updating data and exporting charts.
D3.js is a data-driven JavaScript chart library that provides powerful data visualization capabilities and supports custom charts and animation effects.
Bootstrap is a popular CSS framework that makes it easy to create responsive layouts and beautiful interfaces.
Semantic UI is another CSS framework that provides a large number of UI components and layouts with a high degree of customizability.
We can choose the appropriate chart library and framework according to specific needs.
2. Write PHP code for data query and processing
Before developing the data visualization and chart display module in CMS, we need to first write PHP code to query and process data from the database. We can use database extensions like MySQLi or PDO in PHP to connect to the database and execute queries. The following is a simple PHP code example for connecting to a MySQL database and querying the number of students:
//连接MySQL数据库 $conn = mysqli_connect('localhost', 'username', 'password', 'database'); if (!$conn) { die("连接失败: " . mysqli_connect_error()); } //查询学生人数 $sql = "SELECT COUNT(*) AS student_count FROM students"; $result = mysqli_query($conn, $sql); if (!$result) { die("查询失败: " . mysqli_error($conn)); } //处理查询结果 $row = mysqli_fetch_assoc($result); $student_count = $row['student_count'];
In actual development, we need to write more complex data query and processing logic according to specific needs.
3. Use the chart library to generate charts
Once we obtain the required data, we can use the chart library to generate charts. Taking Chart.js as an example, we can use the following code to generate a histogram in the web page:
<canvas id="myChart"></canvas> <script src="https://cdn.jsdelivr.net/npm/chart.js"></script> <script> var ctx = document.getElementById('myChart').getContext('2d'); var myChart = new Chart(ctx, { type: 'bar', data: { labels: ['A', 'B', 'C', 'D', 'E'], datasets: [{ label: '学生人数', data: [12, 19, 3, 5, 2], backgroundColor: [ 'rgba(255, 99, 132, 0.2)', 'rgba(54, 162, 235, 0.2)', 'rgba(255, 206, 86, 0.2)', 'rgba(75, 192, 192, 0.2)', 'rgba(153, 102, 255, 0.2)' ], borderColor: [ 'rgba(255, 99, 132, 1)', 'rgba(54, 162, 235, 1)', 'rgba(255, 206, 86, 1)', 'rgba(75, 192, 192, 1)', 'rgba(153, 102, 255, 1)' ], borderWidth: 1 }] }, options: { scales: { yAxes: [{ ticks: { beginAtZero: true } }] } } }); </script>
This code uses the Chart.js library, adds a histogram to the web page, and sets the related Parameters, including data, labels, background color, border color, etc. We can adjust these parameters to generate different types and styles of charts based on specific needs.
4. Embed the chart into the CMS page
Finally, we need to embed the generated chart into the CMS page. We can create a page template containing charts in CMS and embed the PHP code that generates charts into the template to achieve data visualization and chart display.
<!DOCTYPE html> <html> <head> <title>学生人数统计</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> </head> <body> <h1>学生人数统计</h1> <canvas id="myChart"></canvas> <script src="https://cdn.jsdelivr.net/npm/chart.js"></script> <script> // PHP代码开始 <?php //查询学生人数 $conn = mysqli_connect('localhost', 'username', 'password', 'database'); $sql = "SELECT COUNT(*) AS student_count FROM students"; $result = mysqli_query($conn, $sql); $row = mysqli_fetch_assoc($result); $student_count = $row['student_count']; //生成柱状图 $data = array(12, 19, 3, 5, 2); $labels = array('A', 'B', 'C', 'D', 'E'); ?> // PHP代码结束 // JavaScript代码开始 var ctx = document.getElementById('myChart').getContext('2d'); var myChart = new Chart(ctx, { type: 'bar', data: { labels: <?php echo json_encode($labels); ?>, datasets: [{ label: '学生人数', data: <?php echo json_encode($data); ?>, backgroundColor: [ 'rgba(255, 99, 132, 0.2)', 'rgba(54, 162, 235, 0.2)', 'rgba(255, 206, 86, 0.2)', 'rgba(75, 192, 192, 0.2)', 'rgba(153, 102, 255, 0.2)' ], borderColor: [ 'rgba(255, 99, 132, 1)', 'rgba(54, 162, 235, 1)', 'rgba(255, 206, 86, 1)', 'rgba(75, 192, 192, 1)', 'rgba(153, 102, 255, 1)' ], borderWidth: 1 }] }, options: { scales: { yAxes: [{ ticks: { beginAtZero: true } }] } } }); // JavaScript代码结束 ?> ?> </script> </body> </html>
In this example, we use PHP's json_encode function to convert the data into JavaScript code and pass it to the Chart.js library to generate a histogram. In actual development, we can adjust PHP and JavaScript codes according to specific needs to achieve more complex data visualization and chart display.
Summary
This article introduces how to use PHP to develop data visualization and chart display modules in CMS. We need to first select the appropriate chart library and framework, then write PHP code for data query and processing, use the chart library to generate charts, and finally embed the charts into the CMS page. In actual development, we should choose appropriate tools and technologies based on specific needs to achieve efficient, reliable, and easy-to-use data visualization and chart display functions, and provide website users with a good user experience.
The above is the detailed content of How to use PHP to develop data visualization and chart display modules in CMS. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
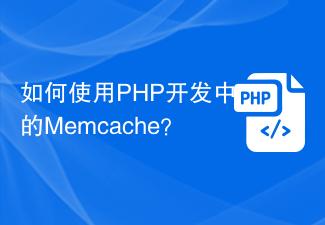
In web development, we often need to use caching technology to improve website performance and response speed. Memcache is a popular caching technology that can cache any data type and supports high concurrency and high availability. This article will introduce how to use Memcache in PHP development and provide specific code examples. 1. Install Memcache To use Memcache, we first need to install the Memcache extension on the server. In CentOS operating system, you can use the following command
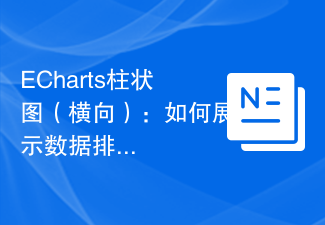
ECharts histogram (horizontal): How to display data rankings requires specific code examples. In data visualization, histogram is a commonly used chart type, which can visually display the size and relative relationship of data. ECharts is an excellent data visualization tool that provides developers with rich chart types and powerful configuration options. This article will introduce how to use the histogram (horizontal) in ECharts to display data rankings, and give specific code examples. First, we need to prepare a data containing ranking data

Graphviz is an open source toolkit that can be used to draw charts and graphs. It uses the DOT language to specify the chart structure. After installing Graphviz, you can use the DOT language to create charts, such as drawing knowledge graphs. After you generate your graph, you can use Graphviz's powerful features to visualize your data and improve its understandability.
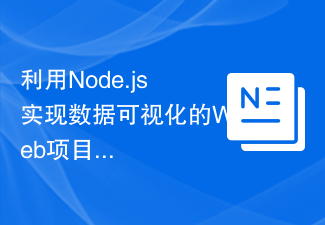
Web projects that use Node.js to implement data visualization require specific code examples. With the advent of the big data era, data visualization has become a very important way of displaying data. By converting data into charts, graphs, maps and other forms, it can visually display the trends, correlations and distribution of data, helping people better understand and analyze the data. As an efficient and flexible server-side JavaScript environment, Node.js can well implement data visualization web projects. in the text,
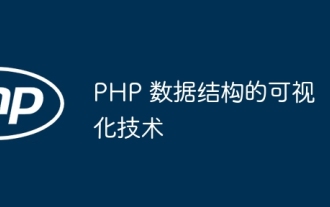
There are three main technologies for visualizing data structures in PHP: Graphviz: an open source tool that can create graphical representations such as charts, directed acyclic graphs, and decision trees. D3.js: JavaScript library for creating interactive, data-driven visualizations, generating HTML and data from PHP, and then visualizing it on the client side using D3.js. ASCIIFlow: A library for creating textual representation of data flow diagrams, suitable for visualization of processes and algorithms.
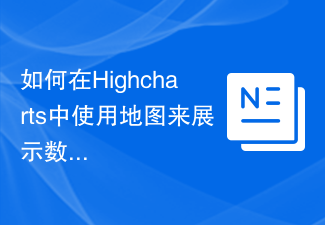
How to use maps to display data in Highcharts Introduction: In the field of data visualization, using maps to display data is a common and intuitive way. Highcharts is a powerful JavaScript charting library that provides rich functionality and flexible configuration options. This article will introduce how to use maps to display data in Highcharts and provide specific code examples. Introducing map data: When using a map, you first need to prepare map data. High
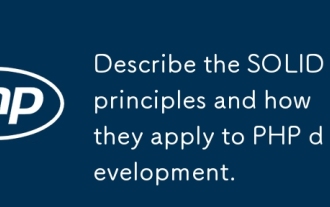
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
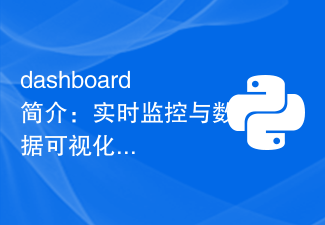
Introduction to Dashboard: A powerful tool for real-time monitoring and data visualization, specific code examples are required Dashboard is a common data visualization tool that allows people to quickly browse multiple indicators in one place. Dashboard can monitor the running status of anything in real time and provide accurate information and reports. Whether you're managing a business, tracking data for a project, tracking market trends, or processing machine learning data output, Dashboard can always be used to its advantage. D
