An introductory tutorial on concurrent programming using Java
Java is one of the most popular programming languages today, and Java's concurrent programming is one of its most powerful features. Java's concurrent programming allows us to use multi-threads to process tasks concurrently and improve the running efficiency of the program. This article will introduce the basic knowledge and practical operations of Java's concurrent programming in detail, and help readers understand the basic concepts and coding methods of Java's concurrent programming.
1. The concept of concurrent programming
Concurrent programming refers to the ability of multiple threads to execute a piece of code at the same time. The number of threads in the program determines how many concurrencies the program has, so concurrent programming is multi-threaded programming. For Java, threads can be created and managed through Java Thread.
Thread is the smallest execution unit in Java. Each Java program has a main thread, and the thread is used to control the execution of the program. When you need to start other threads, you can call the Java Thread class to construct a new thread. In Java, the number of threads running simultaneously is limited. Multi-threaded programming can be used to maximize the use of modern computer hardware resources and improve the running efficiency of the program.
2. Methods to implement concurrent programming in Java
1. Use the Thread class to create and start threads
The Thread class in Java is the key class for creating and managing threads , threads are created in the program by inheriting the Thread class or implementing the Runnable interface. Example of using the Thread class to create and start a thread:
class MyClass extends Thread { public void run() { // 线程执行的代码 } } public class Main { public static void main(String[] args) { MyClass thread = new MyClass(); thread.start(); // 开始执行线程 } }
2. Implementing the Runnable interface
class MyClass implements Runnable { public void run() { // 线程执行的代码 } } public class Main { public static void main(String[] args) { Thread thread = new Thread(new MyClass()); thread.start(); // 开始执行线程 } }
3. Thread synchronization method
Thread synchronization refers to shared access by multiple threads Resource technology is a technology that ensures that they reasonably calculate and obtain shared resources in a certain order. Thread synchronization in Java can be ensured through the Synchronized keyword, which can modify methods and code blocks to ensure that only subsequent threads can access critical resources.
class MyClass { private int count; public synchronized void increase() { count++; } }
4. Thread waiting method
In Java, we can also use the wait() method to wait for the end of thread execution, and use the notify() and notifyAll() methods to notify the concurrent execution of the next Round tasks.
5. Thread interruption method
In Java, thread interruption can be achieved by calling the interrupt() method, and using the isInterrupted() method to determine whether it has been interrupted.
class MyClass extends Thread { private volatile boolean flag = true; public void run() { while (flag) { // 线程执行的代码 } } public void stopThread() { flag = false; interrupt(); // 中断线程 } }
3. Precautions for concurrent programming in Java
1. Thread performance issues
When creating multiple threads, you should pay attention to the number of threads. Too many threads may cause The CPU is overloaded, affecting system performance.
2. Deadlock problem
When multiple threads occupy some key resources at the same time, a deadlock problem may occur, causing the program to be unable to continue execution. Therefore, developers need to properly plan and manage resource sharing between threads.
3. Race condition problem
A race condition refers to a situation where an error occurs in the program state due to concurrent execution. When multiple threads access shared resources at the same time, their execution order may be interleaved, resulting in program execution errors. At this point, synchronization is required to avoid race condition issues.
4. Conclusion
This article introduces the basic concepts and operation methods of concurrent programming in Java, including thread creation, startup, synchronization, waiting and interruption, etc. When writing multi-threaded code, you need to pay attention to thread performance issues, deadlock issues, and race conditions. Through studying this article, I believe that readers can master the basic knowledge of concurrent programming and provide a solid foundation for the concurrency mechanism of Java programming.
The above is the detailed content of An introductory tutorial on concurrent programming using Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics











AI can help optimize the use of Composer. Specific methods include: 1. Dependency management optimization: AI analyzes dependencies, recommends the best version combination, and reduces conflicts. 2. Automated code generation: AI generates composer.json files that conform to best practices. 3. Improve code quality: AI detects potential problems, provides optimization suggestions, and improves code quality. These methods are implemented through machine learning and natural language processing technologies to help developers improve efficiency and code quality.

HTML5 brings five key improvements: 1. Semantic tags improve code clarity and SEO effects; 2. Multimedia support simplifies video and audio embedding; 3. Form enhancement simplifies verification; 4. Offline and local storage improves user experience; 5. Canvas and graphics functions enhance the visualization of web pages.
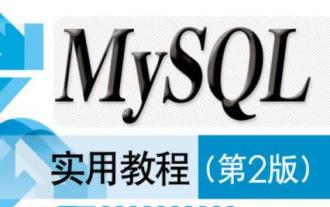
MySQL functions can be used for data processing and calculation. 1. Basic usage includes string processing, date calculation and mathematical operations. 2. Advanced usage involves combining multiple functions to implement complex operations. 3. Performance optimization requires avoiding the use of functions in the WHERE clause and using GROUPBY and temporary tables.
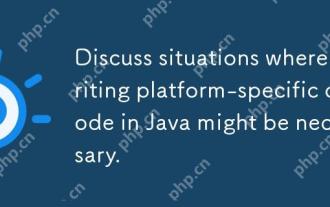
Reasons for writing platform-specific code in Java include access to specific operating system features, interacting with specific hardware, and optimizing performance. 1) Use JNA or JNI to access the Windows registry; 2) Interact with Linux-specific hardware drivers through JNI; 3) Use Metal to optimize gaming performance on macOS through JNI. Nevertheless, writing platform-specific code can affect the portability of the code, increase complexity, and potentially pose performance overhead and security risks.
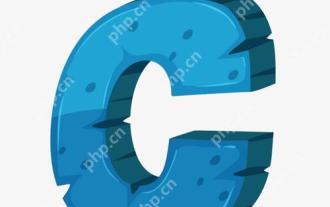
typetraits are used in C for compile-time type checking and operation, improving code flexibility and type safety. 1) Type judgment is performed through std::is_integral and std::is_floating_point to achieve efficient type checking and output. 2) Use std::is_trivially_copyable to optimize vector copy and select different copy strategies according to the type. 3) Pay attention to compile-time decision-making, type safety, performance optimization and code complexity. Reasonable use of typetraits can greatly improve code quality.
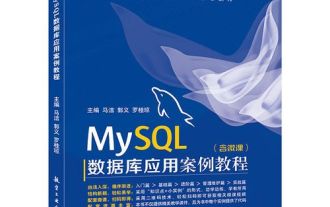
Methods for configuring character sets and collations in MySQL include: 1. Setting the character sets and collations at the server level: SETNAMES'utf8'; SETCHARACTERSETutf8; SETCOLLATION_CONNECTION='utf8_general_ci'; 2. Create a database that uses specific character sets and collations: CREATEDATABASEexample_dbCHARACTERSETutf8COLLATEutf8_general_ci; 3. Specify character sets and collations when creating a table: CREATETABLEexample_table(idINT
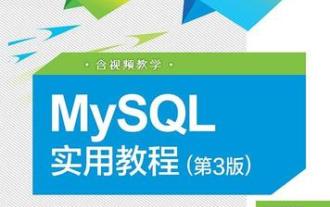
Renaming a database in MySQL requires indirect methods. The steps are as follows: 1. Create a new database; 2. Use mysqldump to export the old database; 3. Import the data into the new database; 4. Delete the old database.
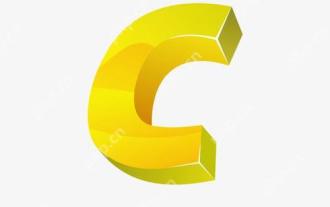
Implementing singleton pattern in C can ensure that there is only one instance of the class through static member variables and static member functions. The specific steps include: 1. Use a private constructor and delete the copy constructor and assignment operator to prevent external direct instantiation. 2. Provide a global access point through the static method getInstance to ensure that only one instance is created. 3. For thread safety, double check lock mode can be used. 4. Use smart pointers such as std::shared_ptr to avoid memory leakage. 5. For high-performance requirements, static local variables can be implemented. It should be noted that singleton pattern can lead to abuse of global state, and it is recommended to use it with caution and consider alternatives.
