


Discuss situations where writing platform-specific code in Java might be necessary.
Reasons for writing platform-specific code in Java include access to specific operating system features, interacting with specific hardware, and optimizing performance. 1) Use JNA or JNI to access the Windows registry; 2) Interact with Linux-specific hardware drivers through JNI; 3) Use Metal to optimize gaming performance on macOS through JNI. Nevertheless, writing platform-specific code can affect the portability of the code, increase complexity, and potentially pose performance overhead and security risks.
When it comes to Java, we often celebrate its "write once, run anywhere" philosophy. But let's dive into the gritty reality where writing platform-specific code becomes not just a choice, but a necessary.
Let's start with the obvious question: why would you ever want to write platform-specific code in Java? Isn't the whole point to avoid such headaches? Well, here's the deal: real-world applications often demand features that are tightly coupled with the underlying operating system or hardware. Let's explore some scenarios where you might find yourself rolling up your sleepes to write code that's tailored to a specific platform.
Imagine you're developing a desktop application that needs to interact directly with the Windows Registry. Java's standard libraries don't provide direct access to this feature. You might find yourself reaching for JNA (Java Native Access) or even JNI (Java Native Interface) to bridge the gap. Here's a quick example using JNA:
import com.sun.jna.Native; import com.sun.jna.Pointer; import com.sun.jna.platform.win32.Advapi32; import com.sun.jna.platform.win32.WinReg; import com.sun.jna.ptr.IntByReference; <p>public class WindowsRegistryExample { public static void main(String[] args) { Advapi32 advapi32 = Native.load("advapi32", Advapi32.class); WinReg.HKEYByReference phkResult = new WinReg.HKEYByReference(); IntByReference lpcbData = new IntByReference();</p><pre class='brush:php;toolbar:false;'> advapi32.RegOpenKeyEx(WinReg.HKEY_LOCAL_MACHINE, "SOFTWARE\\Microsoft\\Windows\\CurrentVersion", 0, WinReg.KEY_READ, phkResult); Pointer data = new Pointer(); advapi32.RegQueryValueEx(phkResult.getValue(), "ProgramFilesDir", 0, null, data, lpcbData); String programFilesDir = data.getString(0); System.out.println("Program Files Directory: " programFilesDir); advapi32.RegCloseKey(phkResult.getValue()); }
}
This code snippet demonstrates how to access the Windows Registry, a feature you won't find in Java's standard libraries. It's a bit of a hack, but sometimes you gotta do what you gotta do.
Another scenario where platform-specific code comes into play is when you're dealing with hardware that's only available on certain platforms. Suppose you're building an application that needs to interface with a Linux-specific hardware driver. You might need to write native code using JNI to communicate with that driver. Here's a rough outline of how you might approach this:
public class LinuxHardwareExample { public native void initializeDriver(); public native void readFromDevice(); public native void writeToDevice(String data); <pre class='brush:php;toolbar:false;'>static { System.loadLibrary("LinuxHardwareDriver"); } public static void main(String[] args) { LinuxHardwareExample example = new LinuxHardwareExample(); example.initializeDriver(); example.readFromDevice(); example.writeToDevice("Hello, Linux!"); }
}
In this case, you'd need to write a native library ( LinuxHardwareDriver
) in C or C to interact with the Linux driver, and then use JNI to call those native methods from Java.
Now, let's talk about performance. Sometimes, you need to squeeze every last drop of performance out of your application, and Java's virtual machine might not be up to the task for certain operations. This is where you might turn to platform-specific optimizations. For instance, if you're working on a high-performance game on macOS, you might want to use Metal, Apple's graphics API. Java doesn't have built-in support for Metal, so you'd need to write native code to leverage it.
import org.lwjgl.system.macosx.LibC; <p>public class MetalExample { public native void initializeMetal(); public native void renderFrame();</p><pre class='brush:php;toolbar:false;'> static { System.loadLibrary("MetalRenderer"); } public static void main(String[] args) { MetalExample example = new MetalExample(); example.initializeMetal(); example.renderFrame(); }
}
Again, you'd need to write a native library ( MetalRenderer
) to interface with Metal and call it from Java using JNI.
While writing platform-specific code can be a powerful tool, it's not without its drawbacks. Here are some pitfalls to watch out for:
Portability : The biggest downside is that your code becomes less portable. If you're targeting multiple platforms, you'll need to maintain multiple codebases or use conditional compilation, which can quickly become a maintenance nightmare.
Complexity : Using JNI or JNA adds complexity to your project. You'll need to deal with memory management, thread safety, and other low-level details that Java usually abstracts away.
Performance Overhead : While native code can be faster, the overhead of switching between Java and native code can sometimes negate those gains. It's cruel to profile your application to ensure that the performance benefits are worth the added complexity.
Security Risks : Native code can introduce security vulnerabilities, especially if you're not careful with memory management or if you're dealing with user input.
In conclusion, while Java's cross-platform nature is one of its greatest strengths, there are times when writing platform-specific code is necessary. Whether you're accessing the Windows Registry, interfacing with Linux hardware, or optimizing performance on macOS, these scenarios require a deep dive into the native world. Just be sure to weigh the benefits against the potential pitfalls and always keep maintainability and security in mind.
The above is the detailed content of Discuss situations where writing platform-specific code in Java might be necessary.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










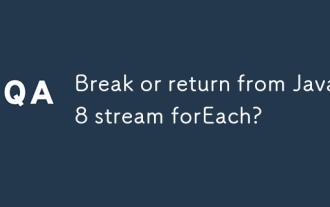
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
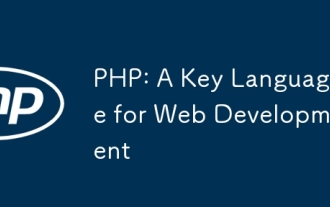
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
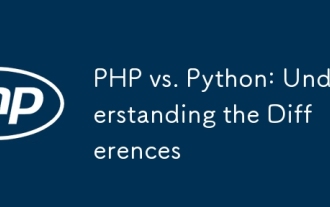
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
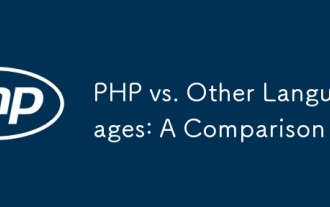
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
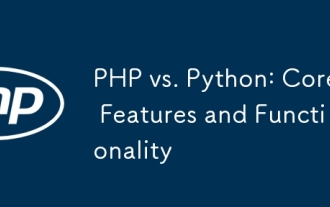
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
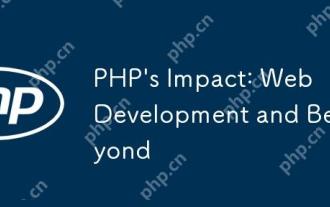
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
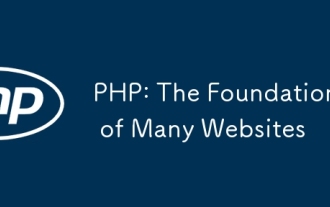
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
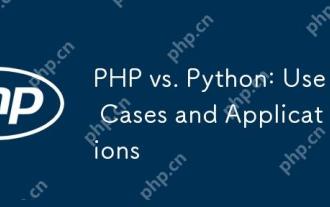
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.
