How to use type traits in C?
type traits在C++中用于编译时类型检查和操作,提升代码的灵活性和类型安全性。1) 通过std::is_integral和std::is_floating_point等进行类型判断,实现高效的类型检查和输出。2) 使用std::is_trivially_copyable优化vector拷贝,根据类型选择不同的拷贝策略。3) 注意编译时决策、类型安全、性能优化和代码复杂性,合理使用type traits可以大大提升代码质量。
在C++中使用type traits可以大大提升代码的灵活性和类型安全性,这也是现代C++编程中的一个重要工具。让我们深入探讨一下如何使用它们,以及在实际应用中需要注意的点。
type traits在C++中主要用于编译时类型检查和操作。它允许我们根据类型进行决策,从而实现更高效、更安全的代码。让我们从一个简单的例子开始,来说明type traits的基本用法。
#include <type_traits> #include <iostream> template<typename T> void print_type_info(T value) { if constexpr (std::is_integral_v<T>) { std::cout << "Integral type: " << value << std::endl; } else if constexpr (std::is_floating_point_v<T>) { std::cout << "Floating point type: " << value << std::endl; } else { std::cout << "Other type: " << value << std::endl; } } int main() { print_type_info(42); // Integral type: 42 print_type_info(3.14); // Floating point type: 3.14 print_type_info("Hello"); // Other type: Hello return 0; }
在这个例子中,我们使用std::is_integral
和std::is_floating_point
来判断传入的类型是否为整数或浮点数,并根据类型进行不同的输出。这种方法不仅在运行时效率高,而且在编译时就能进行类型检查,减少了错误的可能性。
然而,type traits的真正威力在于它可以帮助我们编写更通用的模板代码。假设我们需要一个函数,该函数能够根据类型来决定是否需要使用某种优化策略。我们可以这样做:
#include <type_traits> #include <vector> #include <iostream> template<typename T> void optimize_vector(std::vector<T>& vec) { if constexpr (std::is_trivially_copyable_v<T>) { // 使用 memcpy 进行优化,因为类型是平凡拷贝的 T* data = vec.data(); std::memcpy(data, data, vec.size() * sizeof(T)); std::cout << "Optimized copy for trivially copyable type." << std::endl; } else { // 使用普通的拷贝 std::vector<T> temp = vec; vec = std::move(temp); std::cout << "Standard copy for non-trivially copyable type." << std::endl; } } int main() { std::vector<int> intVec = {1, 2, 3}; std::vector<std::string> stringVec = {"a", "b", "c"}; optimize_vector(intVec); // Optimized copy for trivially copyable type. optimize_vector(stringVec); // Standard copy for non-trivially copyable type. return 0; }
在这个例子中,我们使用std::is_trivially_copyable
来判断类型是否可以使用memcpy
进行优化。对于int
这样的平凡拷贝类型,我们可以使用memcpy
来提高性能,而对于std::string
这样的非平凡拷贝类型,我们则使用标准的拷贝方式。
使用type traits时需要注意的一些点:
编译时决策:type traits在编译时进行决策,这意味着你可以根据类型来优化代码的生成。但是,这也意味着错误会在编译时被发现,而不是运行时,因此在编写代码时需要确保类型检查的正确性。
类型安全:通过type traits,你可以确保代码在类型上是安全的。例如,你可以使用
std::enable_if
来限制模板函数的实例化,只允许特定类型的参数。性能优化:虽然type traits可以帮助你优化代码,但也要小心过度优化。有时候,编译器可能会比你更聪明,能够自动进行某些优化。
复杂性:使用type traits可能会增加代码的复杂性,尤其是在处理复杂的类型关系时。因此,在使用时需要权衡代码的可读性和性能。
最后,分享一个我曾经遇到的问题:在使用std::enable_if
时,如果条件判断不当,可能会导致一些奇怪的编译错误。这是因为std::enable_if
会影响模板的实例化,导致一些意想不到的结果。在这种情况下,仔细检查条件表达式和模板参数是非常重要的。
总的来说,type traits是C++中一个强大的工具,能够帮助你编写更灵活、更高效、更安全的代码。在使用时,结合实际需求和代码的可读性,合理运用type traits可以让你的代码更上一层楼。
The above is the detailed content of How to use type traits in C?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










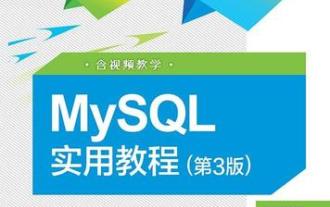
To safely and thoroughly uninstall MySQL and clean all residual files, follow the following steps: 1. Stop MySQL service; 2. Uninstall MySQL packages; 3. Clean configuration files and data directories; 4. Verify that the uninstallation is thorough.
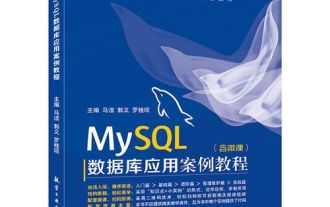
Efficient methods for batch inserting data in MySQL include: 1. Using INSERTINTO...VALUES syntax, 2. Using LOADDATAINFILE command, 3. Using transaction processing, 4. Adjust batch size, 5. Disable indexing, 6. Using INSERTIGNORE or INSERT...ONDUPLICATEKEYUPDATE, these methods can significantly improve database operation efficiency.
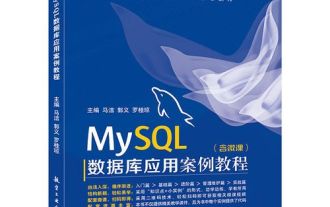
Methods for configuring character sets and collations in MySQL include: 1. Setting the character sets and collations at the server level: SETNAMES'utf8'; SETCHARACTERSETutf8; SETCOLLATION_CONNECTION='utf8_general_ci'; 2. Create a database that uses specific character sets and collations: CREATEDATABASEexample_dbCHARACTERSETutf8COLLATEutf8_general_ci; 3. Specify character sets and collations when creating a table: CREATETABLEexample_table(idINT
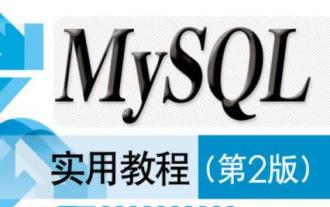
MySQL functions can be used for data processing and calculation. 1. Basic usage includes string processing, date calculation and mathematical operations. 2. Advanced usage involves combining multiple functions to implement complex operations. 3. Performance optimization requires avoiding the use of functions in the WHERE clause and using GROUPBY and temporary tables.
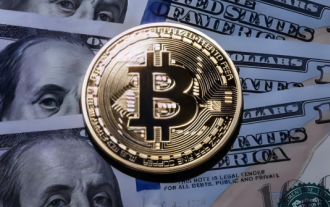
With the popularization and development of digital currency, more and more people are beginning to pay attention to and use digital currency apps. These applications provide users with a convenient way to manage and trade digital assets. So, what kind of software is a digital currency app? Let us have an in-depth understanding and take stock of the top ten digital currency apps in the world.
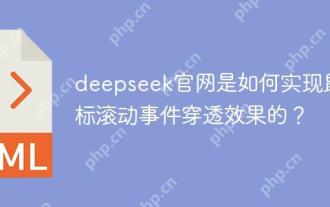
How to achieve the effect of mouse scrolling event penetration? When we browse the web, we often encounter some special interaction designs. For example, on deepseek official website, �...
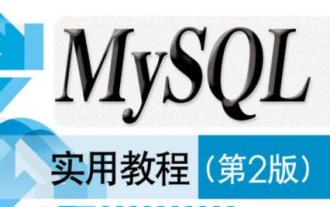
In MySQL, add fields using ALTERTABLEtable_nameADDCOLUMNnew_columnVARCHAR(255)AFTERexisting_column, delete fields using ALTERTABLEtable_nameDROPCOLUMNcolumn_to_drop. When adding fields, you need to specify a location to optimize query performance and data structure; before deleting fields, you need to confirm that the operation is irreversible; modifying table structure using online DDL, backup data, test environment, and low-load time periods is performance optimization and best practice.
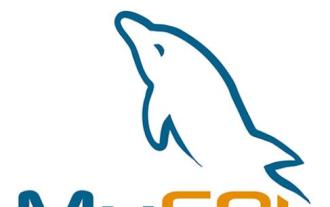
Subqueries can improve the efficiency of MySQL query. 1) Subquery simplifies complex query logic, such as filtering data and calculating aggregated values. 2) MySQL optimizer may convert subqueries to JOIN operations to improve performance. 3) Using EXISTS instead of IN can avoid multiple rows returning errors. 4) Optimization strategies include avoiding related subqueries, using EXISTS, index optimization, and avoiding subquery nesting.
