Common errors and solutions in Java language
With the development and popularity of Java language, more and more people are beginning to learn and use Java language. However, in the process of learning and applying the Java language, we often encounter some errors and problems. This article will introduce common errors and solutions in the Java language to help readers use the Java language more smoothly.
1. The variable is not initialized
In the Java language, if the declared variable is not initialized, it cannot be used directly. Otherwise, the program will prompt an error that the variable is not initialized. This problem can be solved by initializing the variables.
For example, in the following code:
public class Test { public static void main(String[] args) { int x; System.out.println(x); } }
The program will prompt the error "Variable x is not initialized". To solve this problem, you need to initialize the variable mistake. This problem can be avoided by determining the range of the array index.
For example, in the following code:
public class Test { public static void main(String[] args) { int x = 0; System.out.println(x); } }
The program will prompt an "array out of bounds" error. To solve this problem, you can add a judgment statement to judge whether the index exceeds the range of the array:
public class Test { public static void main(String[] args) { int[] arr = new int[3]; System.out.println(arr[3]); } }
3. Null pointer exception
In Java language, if you try to access an empty object Properties or calling a method of a null object will prompt a Null Pointer Exception error. This problem can be avoided by checking whether the object is empty.
For example, in the following code:
public class Test { public static void main(String[] args) { int[] arr = new int[3]; if (arr.length > 3) { System.out.println(arr[3]); } } }
The program will prompt a "Null Pointer Exception" error. To solve this problem, you can add a judgment statement to judge whether the object is empty:
public class Test { public static void main(String[] args) { String str = null; System.out.println(str.length()); } }
4. Type conversion error
In the Java language, if incompatible type conversion is performed, it will Prompt type conversion error. This problem can be solved by using type conversion operators.
For example, in the following code:
public class Test { public static void main(String[] args) { String str = null; if(str != null){ System.out.println(str.length()); } } }
The program will prompt an "incompatible type conversion" error. To solve this problem, you can use the type conversion operator to convert the int type into the byte type:
public class Test { public static void main(String[] args) { int x = 1; byte b = (byte)x; } }
5. Infinite loop
In the Java language, if an infinite loop occurs when writing a program, it will This causes the program to fail to execute normally, causing resource waste or program crashes. This problem can be avoided by writing correct loop conditions and control statements.
For example, in the following code:
public class Test { public static void main(String[] args) { int x = 1; byte b = (byte)x; } }
The program will enter an infinite loop. To solve this problem, the loop conditions need to be adjusted:
public class Test { public static void main(String[] args) { while(true){ System.out.println("死循环"); } } }
This article introduces common errors and solutions in the Java language, including uninitialized variables, array out-of-bounds, null pointer exceptions, type conversion errors, and infinite loops wait. By studying this article, readers can run programs more smoothly and avoid errors and problems when using the Java language.
The above is the detailed content of Common errors and solutions in Java language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










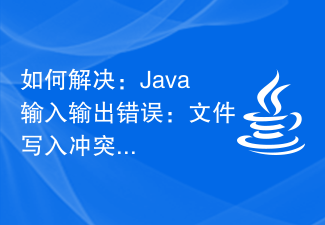
How to solve: Java input and output error: file write conflict In Java programming, reading and writing files are common operations. However, a file write conflict error occurs when multiple threads or processes try to write to the same file at the same time. This may result in data loss or corruption, so it is very important to resolve the file write conflict issue. Several methods to resolve Java input and output error file writing conflicts will be introduced below, along with code examples. Using the file locking mechanism Using the file locking mechanism ensures that at the same time
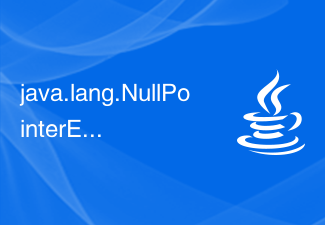
In Java programming, you may encounter java.lang.NullPointerException (hereinafter referred to as NPE) exception. This exception is usually thrown when you try to use a null object or access a null reference, and it indicates that an unexpected null value was found in the code. There are many ways to solve NPE. This article will introduce several common solutions. The most common reason to check for a null reference is to access a null object or null reference.
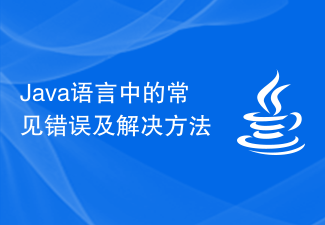
With the development and popularity of Java language, more and more people are beginning to learn and use Java language. However, in the process of learning and applying the Java language, we often encounter some errors and problems. This article will introduce common errors and solutions in the Java language to help readers use the Java language more smoothly. 1. The variable is not initialized. In the Java language, if the declared variable is not initialized, it cannot be used directly. Otherwise, the program will prompt an error that the variable is not initialized. This question can be answered by giving

WhenyouattempttostoreavalueofthewrongdatatypeinaPythonarray,you'llencounteraTypeError.Thisisduetothearraymodule'sstricttypeenforcement,whichrequiresallelementstobeofthesametypeasspecifiedbythetypecode.Forperformancereasons,arraysaremoreefficientthanl
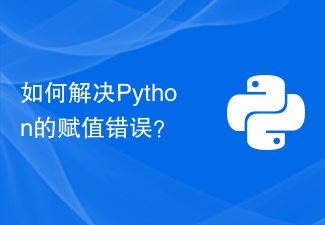
Python is a simple and easy-to-learn high-level programming language, and its flexibility and scalability are loved by programmers. But when writing Python code, we often encounter some assignment errors. These errors may cause our program to fail or even fail to compile. This article will discuss how to solve assignment errors in Python and help you write better Python code. Variables and Assignment in Python In Python, we use variables to store values. A variable is a dynamic entity that can store different types of
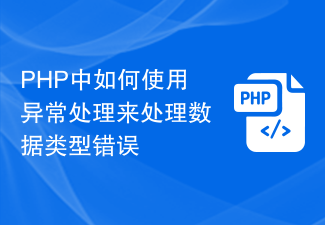
PHP is a commonly used server-side scripting language used to develop dynamic websites and web applications. When performing data processing, data type errors are often encountered. In order to handle these errors, PHP provides an exception handling mechanism. This article will share how to use exception handling to handle data type errors, with code examples. Exception handling is a mechanism for handling errors while a program is running. When an error occurs, an exception can be thrown to notify the code that an abnormal condition has occurred during execution. In PHP, exceptions are thrown via the slang
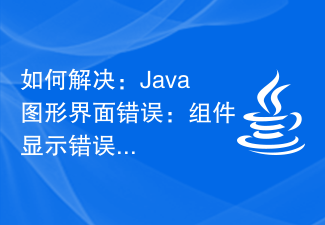
How to solve: Java graphical interface error: Component display error Introduction: Java, as a powerful programming language, is widely popular in graphical interface development. However, in the daily development process, we sometimes encounter the problem of component display errors. This article describes some common error conditions and provides solutions and code examples. Error situation 1: The component is not displayed. Sometimes, we add a component in the code, but after running the program, we find that the component is not displayed. This may be caused by the component not being added to the parent container.
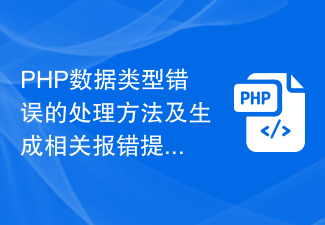
How to handle PHP data type errors and generate related error prompts In PHP programming, we often encounter data type errors, such as calculating strings as integers, or using arrays as strings. These errors may cause the program to fail or produce incorrect results. Therefore, we need to detect and handle data type errors in time. This article will introduce how to handle data type errors in PHP and provide relevant code examples. 1. Data type detection function PHP provides a series of functions to determine the type of variables
