How to perform remote calling and module expansion in PHP?
PHP is a scripting language widely used in Web development. It has a huge community and rich resources, and can easily realize the development of dynamic Web pages and data interaction. However, in actual development, it may be necessary to remotely call other services or extend the PHP module to achieve more functions and performance optimization. This article will introduce how to make remote calls and module extensions in PHP.
1. PHP remote calling
In actual development, we may need to make remote calls to other services, such as calling the API of other Web services, calling remote databases, etc. At this time we can use the curl extension or stream extension provided by PHP to implement remote calls.
- curl extension
curl is a powerful open source tool that can be used to interact with file transfer protocols and supports HTTP/HTTPS/FTP and other protocols. PHP provides the curl extension, which allows us to easily implement remote calls to other services through PHP code. The following is a simple curl call example:
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'http://example.com/api'); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); print_r($response);
In the above code example, a curl session is first initialized through the curl_init() function, and then the URL to be requested, whether a return value is required, etc. are set through the curl_setopt() function. parameters, and finally execute the request through the curl_exec() function and close the curl session with the curl_close() function. Print the returned results through the print_r() function.
- stream extension
The stream extension provides a set of PHP functions that allow us to operate various streams in a unified way, including files, network connections, etc. You can use the stream_context_create() function to create a context, and then use it as the third parameter of the stream_socket_client() function to implement remote calls to other services. The following is a simple stream call example:
$ctx = stream_context_create([ 'http' => [ 'method' => 'POST', 'header' => 'Content-type: application/json', 'content' => json_encode(['name' => 'John', 'age' => 25]), ], ]); $fp = stream_socket_client('http://example.com/api', $err, $errstr, 30, STREAM_CLIENT_CONNECT, $ctx); $response = stream_get_contents($fp); fclose($fp); print_r($response);
In the above code example, a context is first created through the stream_context_create() function, in which the request method, request header, request body and other parameters are set. Then establish a network connection to the specified service through the stream_socket_client() function, and pass in the context as the third parameter. Finally, obtain the response content through the stream_get_contents() function and close the network connection.
2. PHP module extension
A PHP module is a set of functions or classes that can extend the functions of PHP and may bring improvements in performance, security, etc. The following are some commonly used and easily extensible PHP modules.
- Redis
Redis is an open source, high-performance, key-value data storage system that can be used for caching and data storage. PHP provides a redis extension that makes it easy to interact with Redis using PHP. The following is a simple redis operation example:
$redis = new Redis(); $redis->connect('127.0.0.1', 6379); $redis->set('name', 'Bob'); echo $redis->get('name');
In the above code example, a Redis object is first created through the new keyword and connected to the Redis server through the connect() function. Then set a key-value pair through the set() function, and then obtain the corresponding value through the get() function.
- PDO
PDO (PHP Data Objects) is a data access abstraction layer provided by PHP that can be used to interact with various databases. PDO provides a unified set of interfaces, supports functions such as preprocessing and precompiled statements, and has good security and code reusability. The following is a simple example of PDO connection to MySQL:
$dbh = new PDO('mysql:host=localhost;dbname=test', 'username', 'password'); $stmt = $dbh->prepare('SELECT * FROM users WHERE id = ?'); $stmt->bindParam(1, $id); $stmt->execute(); $result = $stmt->fetchAll(PDO::FETCH_ASSOC); print_r($result);
In the above code example, a PDO object is first created through the new keyword and the MySQL connection parameters are passed in. Then create a prepared statement through the prepare() function, using placeholders? , indicating that a parameter will be passed in later using the bindParam() function. Finally, the precompiled statement is executed through the execute() function, and the query results are obtained through the fetchAll() function.
3. Conclusion
This article introduces how to perform remote calls and module extensions in PHP, including using curl and stream extensions to implement remote calls, and using redis and PDO extensions for data storage and access. The above examples are only the simplest usage, and the specific implementation can be flexibly adjusted according to actual needs. I hope this article can help PHP developers better cope with various development tasks.
The above is the detailed content of How to perform remote calling and module expansion in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
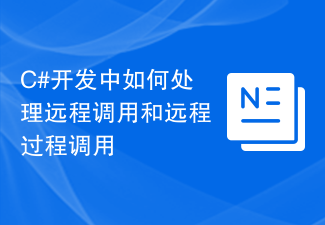
How to handle remote calls and remote procedure calls in C# development requires specific code examples. Introduction: With the rapid development of cloud computing and distributed systems, remote calls and remote procedure calls (RemoteProcedureCall, RPC for short) are becoming more and more important in software development. The more important it is. As a powerful programming language, C# also provides some powerful tools and frameworks for handling remote calls and RPC. This article will give some practical code examples on how to handle remote calls and RPC. 1. Remote calling
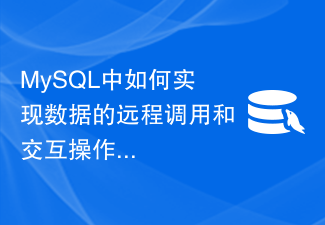
MySQL is a relational database management system that is widely used in various software development and data management scenarios. One of its important features is that it can realize remote calling and interactive operations of data. This article will introduce how to implement this function in MySQL and provide corresponding code examples. MySQL provides a feature called MySQL remote connections that allows data exchange between different machines. In order to achieve remote connection, we need to perform the following steps: Configuring the MySQL server First, we need to ensure
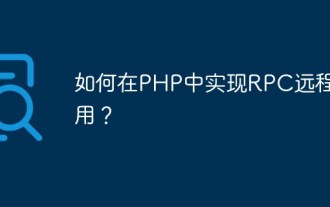
With the rapid development of the Internet and the widespread application of cloud computing technology, distributed systems and microservice architectures are becoming more and more common. In this context, remote procedure call (RPC) has become a common technical means. RPC can enable different services to be called remotely on the network, thereby realizing interconnection operations between different services and improving code reusability and scalability. As a widely used Web development language, PHP is also commonly used in the development of various distributed systems. So, how to implement RPC remote debugging in PHP?
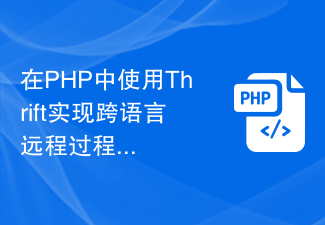
As applications become more complex and distributed, cross-language remote procedure calls (RPC) and communication become increasingly important. In software development, RPC refers to the technology that enables different programs or processes to communicate with each other over the network. Thrift is a simple and easy-to-use RPC framework that can help us quickly develop efficient cross-language RPC services. Thrift was developed by Facebook and is an efficient remote service invocation protocol. It supports multiple languages, including PHP, Java, Python
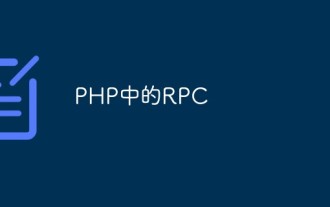
In recent years, with the rapid development of Internet technology, distributed systems have gradually become an indispensable part of the Internet application field. RPC technology in distributed systems is one of the important means to achieve communication between different processes and machines. Among them, RPC technology in PHP has gradually become one of the most widely used technologies among major Internet companies. RPC (RemoteProcedureCall) refers to remote procedure call, which is implemented through remote call on different processes or different machines.
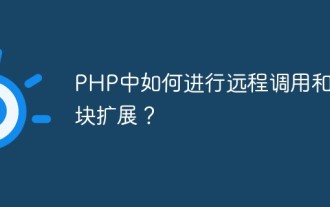
PHP is a scripting language widely used in Web development. It has a large community and rich resources, and can easily realize the development of dynamic Web pages and data interaction. However, in actual development, it may be necessary to remotely call other services or extend the PHP module to achieve more functions and performance optimization. This article will introduce how to make remote calls and module extensions in PHP. 1. PHP remote calling In actual development, we may need to make remote calls to other services, such as calling the API of other Web services, calling remote
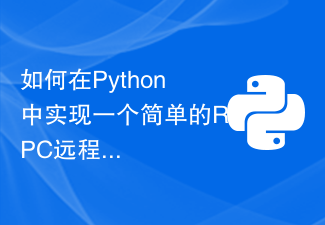
How to implement a simple RPC remote procedure call framework in Python. In distributed systems, a common communication mechanism is to implement function calls between different processes through RPC (RemoteProcedureCall, remote procedure call). RPC allows developers to call remote functions just like calling local functions, making distributed system development more convenient. This article will introduce how to use Python to implement a simple RPC framework and provide detailed code examples. 1. Define RPC

PHP is a widely used programming language mainly used for writing web applications. In web applications, PHP usually calls remote API interfaces, accesses remote databases, etc., so remote operations and data transmission are required. This article will introduce in detail the relevant knowledge of remote operations and data transmission in PHP, including common methods such as curl, fsockopen, file_get_contents, etc. 1. Use curl for remote operations. Curl is a very commonly used method in PHP for remote operations.
