How to add name to setup in Vue3
#What is the use of name in Vue3?
1. You need to define the name when recursing the component
2. You can cache the component with keep-alive include exclude
3. You can do this when Vue reports an error or is debugging See the component's name
Vue3 definition name
1. Automatically generate
<script setup></script>
As long as script
Turning on the setup
syntax sugar mode single file component will automatically generate the corresponding name
option based on the file name, such as Tree.vue
Then his name
It is automatically generated by Tree
. There is a drawback to this. If you want to modify name
, you need to modify the component name. If there is a place import
, the component needs to be modified together.
2. Open a script to define the name
Advantages: You can define the name at will. Disadvantages: Two scripts appearing in a single file component will make people confused.
<template> <div></div> </template> <script lang="ts" setup> import {ref,reactive } from 'vue' </script> <script lang='ts'> export default { name:"XXX" } </script> <style lang="less" scoped> </style>
3. Use the third-party plug-in unplugin-vue-define-options
Installation methodnpm i unplugin-vue-define-options -D
vite
Use
// vite.config.ts import DefineOptions from 'unplugin-vue-define-options/vite' import Vue from '@vitejs/plugin-vue' export default defineConfig({ plugins: [Vue(), DefineOptions()], })
to configure tsconfig.json
// tsconfig.json { "compilerOptions": { // ... "types": ["unplugin-vue-define-options/macros-global" /* ... */] } }
Usage method by compiling macro defineOptions
Add name
and inheritAttrs
<script setup lang="ts"> defineOptions({ name: 'Foo', inheritAttrs: false, }) </script>
4. Personal thoughts: Isn’t it good to define the name directly in the script?
<template> <div></div> </template> <script name="xiaoman" lang="ts" setup> import {ref,reactive } from 'vue' </script> <style lang="less" scoped> </style>
This matter was also discussed intensely in the Vue community, and You Da also responded
You Da thinks this idea is good, but has some concerns. When we use components, we need to define name
and inheritAttrs
. The situation is very rare. Most component library developers may often use these two things, but for 90% of applications For components, it's really a different set of trade-offs, and dealing with props is going to be cumbersome, and coupled with the complexity of the implementation, I'm not sure it's worth it.
But there is already a plug-in that implements this function unplugin-vue-setup-extend-plus
Let’s copy a simple version. The principle is to add a script, but Developers cannot see this script
Vue3 setup supports name plug-in implementation ideas, learn from the above plug-in
import type { Plugin } from 'vite' //@vue/compiler-sfc 这个插件是处理我们单文件组件的代码解析 import { compileScript, parse } from '@vue/compiler-sfc' export default function setupName(): Plugin { return { name: 'vite:plugin:vue:name', //一个 Vite 插件可以额外指定一个 `enforce` 属性 //(类似于 webpack 加载器)来调整它的应用顺序。`enforce` 的值可以是`pre` 或 `post` //加载顺序为 //Alias //带有 `enforce: 'pre'` 的用户插件 //Vite 核心插件 //没有 enforce 值的用户插件 //Vite 构建用的插件 //带有 `enforce: 'post'` 的用户插件 //Vite 后置构建插件(最小化,manifest,报告) enforce: "pre", //transform code参数就是我们写的代码比如vue代码 id就是路径例如/src/xx/xx.vue transform(code, id) { //只处理vue结尾的文件 if (/.vue$/.test(id)) { let { descriptor } = parse(code) //通过compileScript 处理script 返回result //attrs: { name: 'xm', lang: 'ts', setup: true }, //lang: 'ts', //setup: true, const result = compileScript(descriptor, { id }) //attrs 此时就是一个对象 const name = result.attrs.name const lang = result.attrs.lang const inheritAttrs = result.attrs.inheritAttrs //写入script const template = ` <script ${lang ? `lang=${lang}` : ''}> export default { ${name ? `name:"${name}",` : ''} ${inheritAttrs ? `inheritAttrs: ${inheritAttrs !== 'false'},` : ''} } </script> `; //最后拼接上这段代码 也就是我们加的script这一段 返回code code += template; // console.log(code) } return code } } }
, and then introduce the plug-in we wrote in vite config ts
Tree.vue child component
<template> <div v-for="item in data"> {{ item.name }} <xm v-if="item?.children?.length" :data='item?.children'></xm> </div> </template> <script name='xm' lang="ts" setup> import { ref, reactive } from 'vue' defineProps<{ data: any[] }>() </script>
App.vue parent component
<template> <TreeVue :data="data"></TreeVue> </template> <script lang="ts" setup> import TreeVue from './components/Tree.vue'; const data = [ { name: "1", children: [ { name: "1-1", children: [ { name: "1-1-1" } ] } ] } ] </script>
The component was successfully recursed
The above is the detailed content of How to add name to setup in Vue3. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
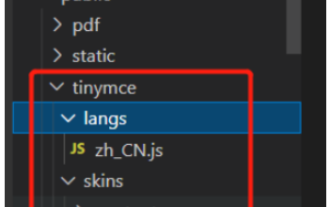
tinymce is a fully functional rich text editor plug-in, but introducing tinymce into vue is not as smooth as other Vue rich text plug-ins. tinymce itself is not suitable for Vue, and @tinymce/tinymce-vue needs to be introduced, and It is a foreign rich text plug-in and has not passed the Chinese version. You need to download the translation package from its official website (you may need to bypass the firewall). 1. Install related dependencies npminstalltinymce-Snpminstall@tinymce/tinymce-vue-S2. Download the Chinese package 3. Introduce the skin and Chinese package. Create a new tinymce folder in the project public folder and download the
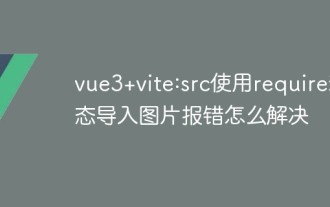
vue3+vite:src uses require to dynamically import images and error reports and solutions. vue3+vite dynamically imports multiple images. If vue3 is using typescript development, require will introduce image errors. requireisnotdefined cannot be used like vue2 such as imgUrl:require(' .../assets/test.png') is imported because typescript does not support require, so import is used. Here is how to solve it: use awaitimport
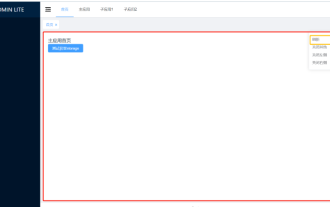
To achieve partial refresh of the page, we only need to implement the re-rendering of the local component (dom). In Vue, the easiest way to achieve this effect is to use the v-if directive. In Vue2, in addition to using the v-if instruction to re-render the local dom, we can also create a new blank component. When we need to refresh the local page, jump to this blank component page, and then jump back in the beforeRouteEnter guard in the blank component. original page. As shown in the figure below, how to click the refresh button in Vue3.X to reload the DOM within the red box and display the corresponding loading status. Since the guard in the component in the scriptsetup syntax in Vue3.X only has o
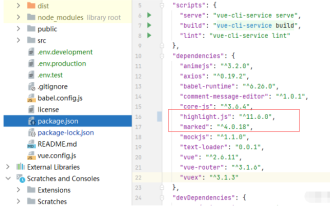
Vue implements the blog front-end and needs to implement markdown parsing. If there is code, it needs to implement code highlighting. There are many markdown parsing libraries for Vue, such as markdown-it, vue-markdown-loader, marked, vue-markdown, etc. These libraries are all very similar. Marked is used here, and highlight.js is used as the code highlighting library. The specific implementation steps are as follows: 1. Install dependent libraries. Open the command window under the vue project and enter the following command npminstallmarked-save//marked to convert markdown into htmlnpmins
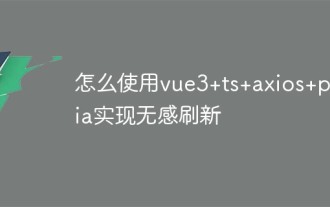
vue3+ts+axios+pinia realizes senseless refresh 1. First download aiXos and pinianpmipinia in the project--savenpminstallaxios--save2. Encapsulate axios request-----Download js-cookienpmiJS-cookie-s//Introduce aixosimporttype{AxiosRequestConfig ,AxiosResponse}from"axios";importaxiosfrom'axios';import{ElMess
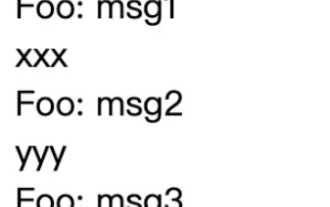
Preface Whether it is vue or react, when we encounter multiple repeated codes, we will think about how to reuse these codes instead of filling a file with a bunch of redundant codes. In fact, both vue and react can achieve reuse by extracting components, but if you encounter some small code fragments and you don’t want to extract another file, in comparison, react can be used in the same Declare the corresponding widget in the file, or implement it through renderfunction, such as: constDemo:FC=({msg})=>{returndemomsgis{msg}}constApp:FC=()=>{return(
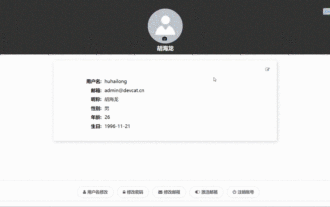
The final effect is to install the VueCropper component yarnaddvue-cropper@next. The above installation value is for Vue3. If it is Vue2 or you want to use other methods to reference, please visit its official npm address: official tutorial. It is also very simple to reference and use it in a component. You only need to introduce the corresponding component and its style file. I do not reference it globally here, but only introduce import{userInfoByRequest}from'../js/api' in my component file. import{VueCropper}from'vue-cropper&
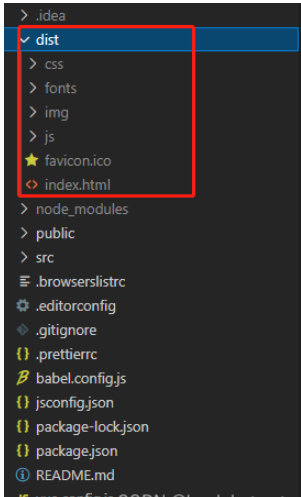
After the vue3 project is packaged and published to the server, the access page displays blank 1. The publicPath in the vue.config.js file is processed as follows: const{defineConfig}=require('@vue/cli-service') module.exports=defineConfig({publicPath :process.env.NODE_ENV==='production'?'./':'/&
