


Controlling PHP output: caching and compressing dynamic pages_PHP Tutorial
mod_gzip is an Apache module whose function is to use Gzip to compress static html pages. Browsers that follow the IETF standard can accept gzip encoding (IE, Netscape, etc.). mod_gzip can increase the download time of a page by 4-5 times. I strongly recommend you use mod_gzip on your web server. However, we also had to build our own compression engine in PHP. In this article, I will introduce how to use PHP's output control function to greatly speed up page loading speed.
Introducing PHP’s output control function
The most satisfying thing about PHP4 is that you can let PHP cache all output generated by scripts, and the browser will not receive anything until you decide to send them out. In a script, you can use these functions to set headers and cookies, but this is only a small part of the powerful output functions.
void ob_start(void);
?> ;
Tells the PHP processor to redirect all output to an internal buffer. No output will be sent to the browser until ob_start is called.
string ob_get_contents(void);
?> ;
This function returns the "output buffer" as a string. You can call this function to send the accumulated output to the browser. (Only after turning off the buffering function!!)
int ob_get_length(void);
?> ;
Returns the length of the string in the cache.
void ob_end_clean(void);
?> ;
Clear the output cache and turn off the output cache. This function must be used before the content in the cache is output to the browser.
void 501([int flag])
Used to turn on/off the implicit flush action switch (default is off). If flush is turned on, every time print/echo or other output commands are called, the output content will be immediately sent to the browser.
Use output controls to compress PHP output
You must use the Zlib extension package compiled in PHP4 to compress the output. If necessary, you can view the installation instructions for the Zlib package in the PHP documentation.
First, initialize the output cache:
ob_start();
ob_implicit_flush(0) ;
?>
After that, use print, echo, or any other method you like to generate all output content, for example:
print("Hey this is a compressed output!" );
?>
After the page is generated, we retrieve the output content:
$contents = ob_get_contents();
ob_end_clean ();
?>
After that, it must be detected whether the browser supports compressed data. If supported, the browser will send an ACCEPT-ENCODEING HTTP header to the server. We only need to check if there is a "gzip,deflate" string in the $HTTP_ACCEPT_ENCODING variable.
if(ereg('gzip, deflate',$ HTTP_ACCEPT_ENCODING)) {
// Generate Gzip compressed content here
} else {
echo $contents;
}
?>
This method is simple to use and clearly structured. Let's see how to generate compressed output:
//Tell the browser that the data it will receive is gzip data
//Of course before that, you have checked whether they support gzip, x-gzip data format
//If x-gzip is supported, then the following header must be replaced by z-gzip
header("Content-Encoding: gzip");
//Display the header of the gzip file
//Only displayed once
echo "x1fx8bx08x00x00x00x00x00";
//Calculate the file size and CRC code
$Size = strlen($contents);
$Crc = crc32($contents);
//Compress data
$contents = gzcompress($contents, 9);
//We can’t just output it like this because the CRC code is confusing.
//If I use "echo $contents" here, the compressed data will be sent,
//but it will be incomplete. The last four bytes of the file are CRC check codes, but only three bytes were sent.
//The last byte is lost. I don't know if this bug is solved in version 4.0.2,
//But the best way to avoid errors is to add the correct CRC check code to the end of the compressed data.
//
//Strip the old CRC check code
$contents = substr($contents, 0, strlen($contents) - 4);
//Only display compressed data
echo $contents;
//Output CRC, and the size of the original data (bytes)
gzip_PrintFourChars($Crc);
gzip_PrintFourChars($Size);
function gzip_PrintFourChars($Val) {
for ($i = 0; $i <4; $i ++) {
echo chr($Val % 256);
$Val = floor($Val / 256);
}
}
?>
//Okay, you can also append more compressed data in this way.
For actual testing, all script codes are as follows:
ob_start();
ob_implicit_flush(0) ;
print("I'm compressed!n");
$contents = ob_get_contents();
ob_end_clean();
header("Content-Encoding: gzip");
echo "x1fx8bx08x00x00x00x00x00";
$Size = strlen($contents);
$Crc = crc32($contents);
$contents = gzcompress($contents, 9);
$contents = substr($contents, 0, strlen($contents) - 4);
echo $contents;
gzip_PrintFourChars($Crc);
gzip_PrintFourChars($Size);
function gzip_PrintFourChars($Val) {
for ($i = 0; $i <4; $i ++) {
echo chr($Val % 256);
$Val = floor($Val / 256);
}
}
?>
Cache PHP output
When PHP4 had not yet come out and I had to use PHP3, I was very interested in developing some caching mechanisms to reduce database loading and file system access. There is no particularly good method in PHP3, but with output caching, everything becomes much easier in PHP4.
Here’s a simple example:
//Construct a file name for the requested URI
$cached_file=md5($REQUEST_URI);
if((!file_exists("/cache/$cached_file"))||(!is_valid("/cache/$cached_file"))) {
//is_valid function to verify the cache, you can use this function Check whether the cache has expired or other specific conditions.
//Generate output if the file is not in the Cache or is not available
ob_start();
ob_implicit_flush(0);
//Output here...
$contents = ob_get_contents();
ob_end_clean();
$fil=fopen($cached_file,"w+");
fwrite($fil,$contents,$strlen($contents) );
fclose($fil);
}
/ If the requested file is in cache and available, then:
readfile($cached_file);
?>
This is a simple example, using output caching, you can build a complex content generation system, use different caching mechanisms for different chunks or programs, etc...
Conclusion
PHP output control functions are very useful for redirecting the output generated by scripts to the cache. Outputting cached data for gzip-enabled browsers can reduce load times. It can also be used as a caching mechanism to reduce access to data sources (databases or files), which is of great significance when using XML.
If we build an engine in PHP, cache data from data sources (xml documents and databases), and dynamically generate content in XML format (without appearance-presentation) we can get the output of these XML and use XSLT Convert to any format we want (html, wap, palm, pdf, etc.). This task can be accomplished nicely using PHP4's output caching and the Sablotron XSLT extension.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
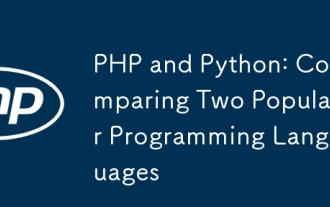
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
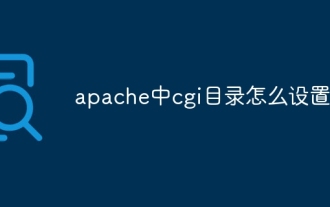
To set up a CGI directory in Apache, you need to perform the following steps: Create a CGI directory such as "cgi-bin", and grant Apache write permissions. Add the "ScriptAlias" directive block in the Apache configuration file to map the CGI directory to the "/cgi-bin" URL. Restart Apache.
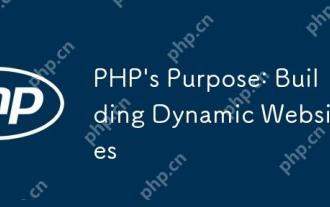
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.
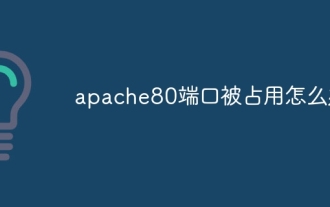
When the Apache 80 port is occupied, the solution is as follows: find out the process that occupies the port and close it. Check the firewall settings to make sure Apache is not blocked. If the above method does not work, please reconfigure Apache to use a different port. Restart the Apache service.
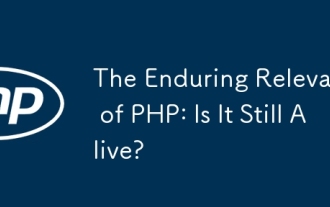
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
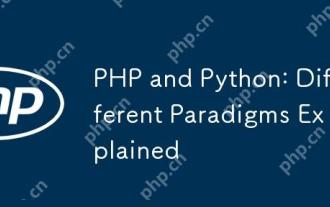
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
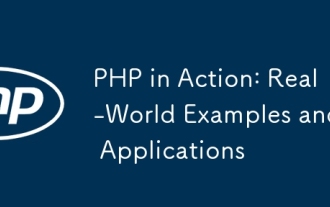
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
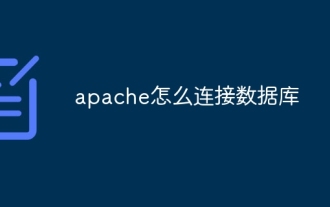
Apache connects to a database requires the following steps: Install the database driver. Configure the web.xml file to create a connection pool. Create a JDBC data source and specify the connection settings. Use the JDBC API to access the database from Java code, including getting connections, creating statements, binding parameters, executing queries or updates, and processing results.
