


For Loop vs While Loop: Python Syntax, Use Cases & Examples
For loops are used when the number of iterations is known, while while loops are used until a condition is met. 1) For loops are ideal for sequences like lists, using syntax like 'for fruit in fruits: print(fruit)'. 2) While loops are suitable for unknown iteration counts, e.g., 'while countdown > 0: print(countdown) countdown -= 1'. Both loops have specific use cases and can be combined for enhanced functionality.
When it comes to looping in Python, developers often find themselves choosing between for
loops and while
loops. So, what's the difference between these two? In essence, for
loops are typically used when you know the number of iterations in advance, while while
loops are better suited for scenarios where you need to continue looping until a certain condition is met. But there's more to it than just this basic distinction. Let's dive deeper into the syntax, use cases, and some personal insights on when and how to use each.
Let's start with the for
loop. This is my go-to when I'm dealing with sequences like lists, tuples, or even strings. The syntax is straightforward:
fruits = ['apple', 'banana', 'cherry'] for fruit in fruits: print(fruit)
What I love about for
loops is how they seamlessly integrate with Python's iterable objects. It's like the language was designed with this in mind, making your code cleaner and more readable. But here's a tip: if you ever find yourself needing the index along with the item, don't forget about enumerate
:
for index, fruit in enumerate(fruits): print(f'Fruit at index {index}: {fruit}')
Now, let's switch gears to while
loops. These are perfect for situations where you're not sure how many iterations you'll need. Here's a simple example where we're counting down:
countdown = 5 while countdown > 0: print(countdown) countdown -= 1 print("Liftoff!")
One thing I've learned the hard way is to be cautious with while
loops. They can easily lead to infinite loops if you're not careful with your conditions. Always make sure your condition will eventually become false, or have a way to break out of the loop.
In terms of use cases, I find for
loops incredibly versatile. They're my first choice for iterating over collections, and they work beautifully with Python's list comprehensions. Here's a quick example of using a for
loop to create a list of squares:
squares = [x**2 for x in range(10)] print(squares)
On the other hand, while
loops shine in scenarios where you need to keep looping until something specific happens. For instance, if you're writing a game loop or a server that needs to keep running until it's manually stopped, a while
loop is your friend.
Let's talk about performance for a moment. In most cases, the performance difference between for
and while
loops is negligible. However, if you're dealing with very large datasets, for
loops can be slightly more efficient because they're optimized for iterating over sequences. But don't let this sway you too much; readability and maintainability should always come first.
Here's a little trick I use sometimes: combining for
and while
loops. For example, if you need to iterate over a list but also want to break out of the loop under certain conditions, you can use a for
loop with a break
statement:
numbers = [1, 2, 3, 4, 5] for num in numbers: if num == 3: break print(num)
This approach gives you the best of both worlds: the simplicity of a for
loop with the flexibility to exit early.
In conclusion, both for
and while
loops have their place in Python programming. For
loops are my go-to for iterating over known sequences, while while
loops are essential for scenarios where you need to loop until a condition is met. Remember, the key to choosing between them is understanding your specific use case and prioritizing code readability. Happy coding!
The above is the detailed content of For Loop vs While Loop: Python Syntax, Use Cases & Examples. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










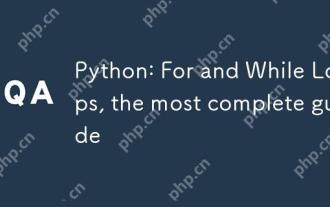
In Python, a for loop is used to traverse iterable objects, and a while loop is used to perform operations repeatedly when the condition is satisfied. 1) For loop example: traverse the list and print the elements. 2) While loop example: guess the number game until you guess it right. Mastering cycle principles and optimization techniques can improve code efficiency and reliability.
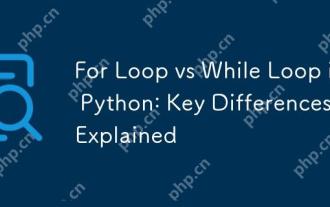
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf

How to use while loop in Python In Python programming, loop is one of the very important concepts. Loops help us repeatedly execute a piece of code until a specified condition is met. Among them, the while loop is one of the most widely used loop structures. By using a while loop, we can implement more complex logic by executing it repeatedly depending on whether the condition is true or false. The basic syntax format for using while loop is as follows: while condition: loop body where the condition is
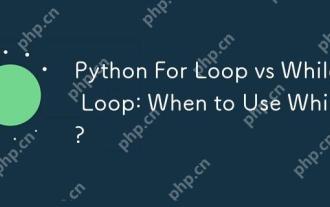
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.

Useforloopswhenthenumberofiterationsisknowninadvance,andwhileloopswheniterationsdependonacondition.1)Forloopsareidealforsequenceslikelistsorranges.2)Whileloopssuitscenarioswheretheloopcontinuesuntilaspecificconditionismet,usefulforuserinputsoralgorit
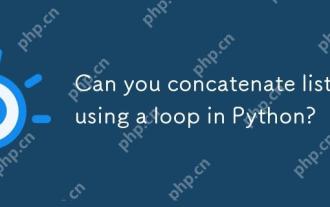
Yes,youcanconcatenatelistsusingaloopinPython.1)Useseparateloopsforeachlisttoappenditemstoaresultlist.2)Useanestedlooptoiterateovermultiplelistsforamoreconciseapproach.3)Applylogicduringconcatenation,likefilteringevennumbers,foraddedflexibility.Howeve
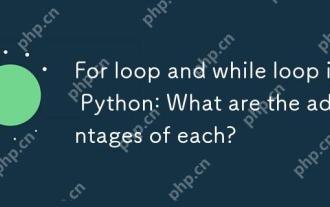
Forloopsareadvantageousforknowniterationsandsequences,offeringsimplicityandreadability;whileloopsareidealfordynamicconditionsandunknowniterations,providingcontrolovertermination.1)Forloopsareperfectforiteratingoverlists,tuples,orstrings,directlyacces

In Python, for loops are suitable for cases where the number of iterations is known, while loops are suitable for cases where the number of iterations is unknown and more control is required. 1) For loops are suitable for traversing sequences, such as lists, strings, etc., with concise and Pythonic code. 2) While loops are more appropriate when you need to control the loop according to conditions or wait for user input, but you need to pay attention to avoid infinite loops. 3) In terms of performance, the for loop is slightly faster, but the difference is usually not large. Choosing the right loop type can improve the efficiency and readability of your code.
