Can you concatenate lists using a loop in Python?
Yes, you can concatenate lists using a loop in Python. 1) Use separate loops for each list to append items to a result list. 2) Use a nested loop to iterate over multiple lists for a more concise approach. 3) Apply logic during concatenation, like filtering even numbers, for added flexibility. However, loops may be less efficient for large lists, where using the operator or extend method is more Pythonic and faster.
Yes, you can definitely concatenate lists using a loop in Python. Let's dive into this fascinating topic and explore how we can achieve this in a way that's both effective and showcases some cool Python tricks.
When I first started programming in Python, concatenating lists seemed like a simple task, but as I delved deeper, I realized there were multiple ways to do it, each with its own charm and use cases. Using a loop to concatenate lists is particularly interesting because it gives you fine-grained control over the process, allowing you to perform additional operations or checks as you go.
Let's start with a basic example of how you might concatenate two lists using a loop:
list1 = [1, 2, 3] list2 = [4, 5, 6] result = [] for item in list1: result.append(item) for item in list2: result.append(item) print(result) # Output: [1, 2, 3, 4, 5, 6]
This approach is straightforward but has its limitations. It's not the most Pythonic way to concatenate lists, but it's a great starting point for understanding how loops can be used in list manipulation.
If you're like me and love to explore different coding styles, you might want to try a more concise version using a single loop:
list1 = [1, 2, 3] list2 = [4, 5, 6] result = [] for lst in (list1, list2): for item in lst: result.append(item) print(result) # Output: [1, 2, 3, 4, 5, 6]
This method is not only more compact but also demonstrates a neat way to iterate over multiple lists. It's particularly useful if you need to concatenate more than two lists or if you want to apply some logic during the concatenation process.
Now, let's talk about the pros and cons of using a loop for list concatenation.
On the plus side, using a loop gives you the flexibility to modify or filter the items as you concatenate them. For instance, if you only want to include even numbers from list2
, you can easily do so:
list1 = [1, 2, 3] list2 = [4, 5, 6] result = [] for item in list1: result.append(item) for item in list2: if item % 2 == 0: result.append(item) print(result) # Output: [1, 2, 3, 4, 6]
However, using a loop can be less efficient than other methods, especially for large lists. Python provides more efficient ways to concatenate lists, such as the
operator or the extend
method:
list1 = [1, 2, 3] list2 = [4, 5, 6] # Using the operator result = list1 list2 print(result) # Output: [1, 2, 3, 4, 5, 6] # Using the extend method result = [] result.extend(list1) result.extend(list2) print(result) # Output: [1, 2, 3, 4, 5, 6]
These methods are generally faster and more Pythonic. However, if you need to perform operations on the items during concatenation, a loop might still be your best bet.
One thing to watch out for when using loops for concatenation is the potential for creating a new list in memory with each iteration. This can be a performance bottleneck if you're dealing with large datasets. In such cases, consider using generators or list comprehensions to be more memory-efficient.
Here's an example using a generator to concatenate lists in a memory-efficient way:
list1 = [1, 2, 3] list2 = [4, 5, 6] def concatenate_lists(*lists): for lst in lists: yield from lst result = list(concatenate_lists(list1, list2)) print(result) # Output: [1, 2, 3, 4, 5, 6]
This approach allows you to lazily concatenate the lists, which can be particularly useful if you're dealing with large datasets or if you need to process the concatenated list in chunks.
In conclusion, concatenating lists using a loop in Python is a versatile technique that offers a lot of control over the process. While it might not be the most efficient method for all cases, it's invaluable when you need to perform operations during concatenation. As you explore Python, don't shy away from experimenting with different methods to find what works best for your specific use case. Remember, the beauty of programming lies in the journey of discovery and optimization!
The above is the detailed content of Can you concatenate lists using a loop in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










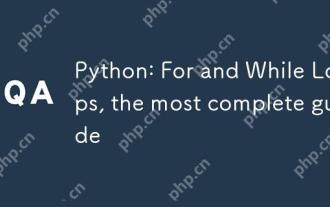
In Python, a for loop is used to traverse iterable objects, and a while loop is used to perform operations repeatedly when the condition is satisfied. 1) For loop example: traverse the list and print the elements. 2) While loop example: guess the number game until you guess it right. Mastering cycle principles and optimization techniques can improve code efficiency and reliability.

How to use while loop in Python In Python programming, loop is one of the very important concepts. Loops help us repeatedly execute a piece of code until a specified condition is met. Among them, the while loop is one of the most widely used loop structures. By using a while loop, we can implement more complex logic by executing it repeatedly depending on whether the condition is true or false. The basic syntax format for using while loop is as follows: while condition: loop body where the condition is

There are many methods to connect two lists in Python: 1. Use operators, which are simple but inefficient in large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use the = operator, which is both efficient and readable; 4. Use itertools.chain function, which is memory efficient but requires additional import; 5. Use list parsing, which is elegant but may be too complex. The selection method should be based on the code context and requirements.
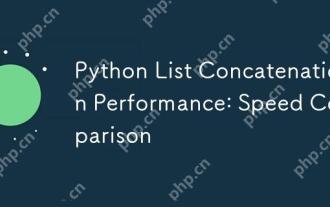
ThefastestmethodforlistconcatenationinPythondependsonlistsize:1)Forsmalllists,the operatorisefficient.2)Forlargerlists,list.extend()orlistcomprehensionisfaster,withextend()beingmorememory-efficientbymodifyinglistsin-place.
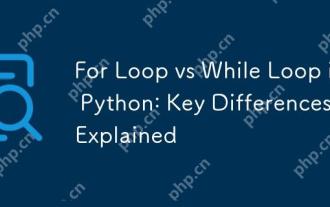
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf

Useforloopswhenthenumberofiterationsisknowninadvance,andwhileloopswheniterationsdependonacondition.1)Forloopsareidealforsequenceslikelistsorranges.2)Whileloopssuitscenarioswheretheloopcontinuesuntilaspecificconditionismet,usefulforuserinputsoralgorit
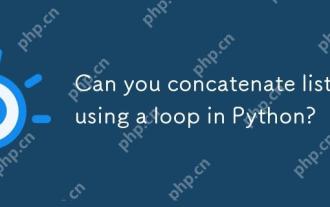
Yes,youcanconcatenatelistsusingaloopinPython.1)Useseparateloopsforeachlisttoappenditemstoaresultlist.2)Useanestedlooptoiterateovermultiplelistsforamoreconciseapproach.3)Applylogicduringconcatenation,likefilteringevennumbers,foraddedflexibility.Howeve
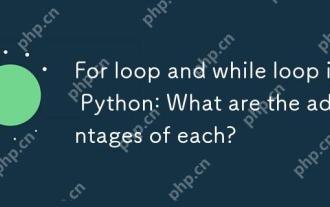
Forloopsareadvantageousforknowniterationsandsequences,offeringsimplicityandreadability;whileloopsareidealfordynamicconditionsandunknowniterations,providingcontrolovertermination.1)Forloopsareperfectforiteratingoverlists,tuples,orstrings,directlyacces
