What is bytecode, and how does it relate to Java's platform independence?
Bytecode in Java is the intermediate representation that enables platform independence. 1) Java code is compiled into bytecode stored in .class files. 2) The JVM interprets or compiles this bytecode into machine code at runtime, allowing the same bytecode to run on any device with a JVM, thus fulfilling Java's "write once, run anywhere" promise.
Bytecode is the intermediate representation of your code that sits between the high-level source code you write and the machine-specific binary code that the computer's processor understands. In the context of Java, bytecode is what the Java compiler produces when it translates your .java
files into .class
files. This bytecode is platform-independent, meaning it can run on any device that has a Java Virtual Machine (JVM) installed, regardless of the underlying operating system or hardware architecture.
Let's dive deeper into this fascinating world of bytecode and explore how it underpins Java's famous "write once, run anywhere" promise.
When I first started learning Java, the concept of bytecode was a bit of a mystery. I remember thinking, "Why can't the compiler just produce machine code directly?" The answer lies in the beauty of abstraction and portability. By compiling to bytecode, Java achieves a level of platform independence that few other languages can match.
Here's how it works: when you write a Java program, you compile it into bytecode using the javac
compiler. This bytecode is stored in .class
files, which contain instructions that are not specific to any particular hardware or operating system. Instead, these instructions are designed to be executed by the JVM.
The JVM acts as an interpreter or just-in-time (JIT) compiler, translating the bytecode into machine code that the host system can understand. This process happens at runtime, allowing the same bytecode to run on different platforms without modification.
Now, let's look at some code to illustrate this concept. Here's a simple Java class that we'll compile to bytecode:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, Bytecode!"); } }
When we compile this class, the javac
compiler will produce a HelloWorld.class
file containing bytecode. If we were to disassemble this bytecode (using a tool like javap
), we'd see something like this:
Compiled from "HelloWorld.java" public class HelloWorld { public HelloWorld(); Code: 0: aload_0 1: invokespecial #1 // Method java/lang/Object."<init>":()V 4: return public static void main(java.lang.String[]); Code: 0: getstatic #2 // Field java/lang/System.out:Ljava/io/PrintStream; 3: ldc #3 // String Hello, Bytecode! 5: invokevirtual #4 // Method java/io/PrintStream.println:(Ljava/lang/String;)V 8: return }
This bytecode is what the JVM will execute. It's not tied to any specific platform, which is why you can take this HelloWorld.class
file and run it on a Windows machine, a Mac, or even a Linux server, as long as they have a JVM installed.
The beauty of this approach is not just in its portability but also in its security and performance benefits. The JVM can perform various runtime checks and optimizations that wouldn't be possible if the code were compiled directly to machine code. For instance, the JVM can implement memory management and garbage collection, which helps prevent common programming errors like memory leaks.
However, there are some trade-offs to consider. The additional layer of abstraction introduced by bytecode and the JVM can lead to slightly slower execution compared to native code. But for most applications, the benefits of platform independence and the robust ecosystem of Java far outweigh these minor performance costs.
In my experience, working with bytecode has been both enlightening and challenging. When debugging issues, it's sometimes necessary to dive into the bytecode to understand what's happening at a lower level. Tools like javap
and profilers can be invaluable in these situations, helping you see how your high-level code translates into the bytecode that the JVM executes.
To wrap up, bytecode is the key to Java's platform independence. It allows developers to write code once and run it anywhere, thanks to the universal nature of the JVM. While there are some performance considerations, the advantages of this approach are clear, making Java a powerful choice for cross-platform development.
So, the next time you're writing Java code, remember that you're not just writing for your current machine—you're writing for the world, thanks to the magic of bytecode.
The above is the detailed content of What is bytecode, and how does it relate to Java's platform independence?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










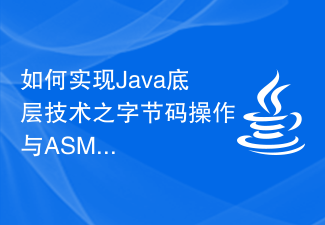
How to implement bytecode operations and ASM framework of Java's underlying technology Introduction: Java, as a high-level programming language, often does not need to pay attention to the underlying details for developers. However, in some special scenarios, we may need to have an in-depth understanding of Java's underlying technologies, such as bytecode operations. This article will introduce how to implement Java bytecode operations through the ASM framework and provide specific code examples. 1. What is bytecode operation? During the compilation process of Java, the source code will be compiled into bytecode and then used by the JVM
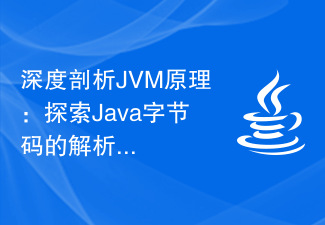
In-depth analysis of JVM principles: Exploring the parsing and execution of Java bytecode Introduction: JVM (Java Virtual Machine) is the core engine for Java program execution and is responsible for parsing and executing Java bytecode. A deep understanding of JVM principles is crucial for developers. It not only helps us optimize code performance, but also helps us solve some common problems. This article will provide an in-depth analysis of how the JVM works and illustrate it with specific code examples. 1. Composition of JVM JVM consists of three core components: class loader
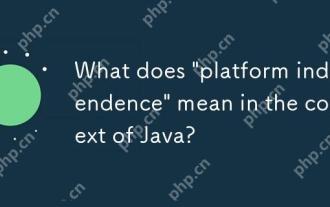
Java's platform independence means that the code written can run on any platform with JVM installed without modification. 1) Java source code is compiled into bytecode, 2) Bytecode is interpreted and executed by the JVM, 3) The JVM provides memory management and garbage collection functions to ensure that the program runs on different operating systems.
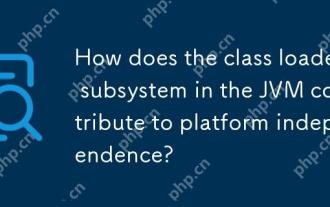
The class loader ensures the consistency and compatibility of Java programs on different platforms through unified class file format, dynamic loading, parent delegation model and platform-independent bytecode, and achieves platform independence.
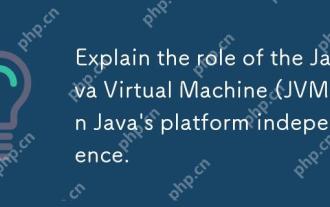
JVM enables Java to run across platforms. 1) JVM loads, validates and executes bytecode. 2) JVM's work includes class loading, bytecode verification, interpretation execution and memory management. 3) JVM supports advanced features such as dynamic class loading and reflection.
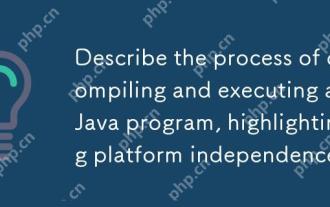
The compilation and execution of Java programs achieve platform independence through bytecode and JVM. 1) Write Java source code and compile it into bytecode. 2) Use JVM to execute bytecode on any platform to ensure the code runs across platforms.
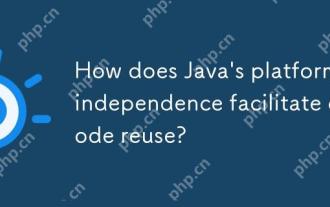
Java'splatformindependencefacilitatescodereusebyallowingbytecodetorunonanyplatformwithaJVM.1)Developerscanwritecodeonceforconsistentbehavioracrossplatforms.2)Maintenanceisreducedascodedoesn'tneedrewriting.3)Librariesandframeworkscanbesharedacrossproj
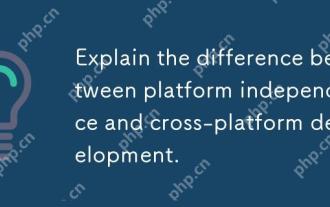
Platformindependenceallowsprogramstorunonanyplatformwithoutmodification,whilecross-platformdevelopmentrequiressomeplatform-specificadjustments.Platformindependence,exemplifiedbyJava,enablesuniversalexecutionbutmaycompromiseperformance.Cross-platformd
