


Explain the role of the Java Virtual Machine (JVM) in Java's platform independence.
JVM使Java实现跨平台运行。1)JVM加载、验证和执行字节码。2)JVM的工作包括类加载、字节码验证、解释执行和内存管理。3)JVM支持高级功能如动态类加载和反射。
引言
在编程世界中,Java以其"一次编写,到处运行"的口号闻名,这一切都要归功于Java虚拟机(JVM)。今天,我要带大家深入了解JVM在Java平台独立性中扮演的关键角色。通过这篇文章,你将不仅能理解JVM如何让Java代码在不同操作系统上运行,还能领略到这一技术背后的设计哲学和实现细节。我会结合自己的编程经验,分享一些实用的见解和可能遇到的挑战。
基础知识回顾
Java是一种高级编程语言,它的核心设计理念是跨平台性。为了实现这一目标,Java引入了JVM作为运行时环境。JVM是一个抽象的计算机器,它能够在任何支持它的操作系统上运行。这意味着无论你是使用Windows、Linux还是Mac OS,只要安装了JVM,你的Java代码就能无缝运行。
JVM的主要任务是将Java源代码编译成字节码,然后在运行时将字节码解释或编译成特定于主机的机器码。这样的设计让Java程序可以独立于硬件和操作系统。
核心概念或功能解析
JVM的定义与作用
JVM是Java平台独立性的基石。它负责加载、验证和执行字节码文件(.class文件)。JVM的作用可以简单总结为:
- 加载:将.class文件加载到内存中。
- 验证:确保加载的字节码是合法的且不会损害JVM的安全性。
- 执行:将字节码转换为机器码并执行。
下面是一个简单的示例,展示了JVM如何执行Java代码:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
当你运行这个程序时,JVM会将HelloWorld.class
文件加载并执行,输出"Hello, World!"。
JVM的工作原理
JVM的工作原理可以分为几个主要阶段:
- 类加载:JVM通过类加载器将.class文件加载到内存中。
- 字节码验证:确保字节码符合Java语言规范,防止恶意代码执行。
- 解释执行:JVM解释字节码,将其转换为机器码并执行。现代JVM通常采用即时编译(JIT)技术,将热点代码编译为机器码以提高性能。
- 内存管理:JVM负责管理内存,包括垃圾回收,确保程序的稳定运行。
JVM的设计使得Java程序可以在不同的硬件和操作系统上运行,但这也带来了一些挑战。例如,性能优化需要考虑不同JVM实现的差异,调试JVM相关的内存问题也需要一定的技巧。
使用示例
基本用法
在Java中,JVM的基本用法就是编写Java代码并编译成字节码,然后通过JVM运行。例如:
public class BasicExample { public static void main(String[] args) { System.out.println("Running on JVM!"); } }
这段代码会被编译成BasicExample.class
,然后通过JVM执行,输出"Running on JVM!"。
高级用法
JVM的强大之处在于它支持多种高级功能,如动态类加载和反射。以下是一个使用反射的示例:
import java.lang.reflect.Method; public class AdvancedExample { public static void main(String[] args) throws Exception { Class<?> clazz = Class.forName("java.lang.String"); Method method = clazz.getMethod("length"); String str = "Hello, JVM!"; Object result = method.invoke(str); System.out.println("String length: " + result); } }
这段代码动态加载String
类,并通过反射调用length
方法,展示了JVM的灵活性。
常见错误与调试技巧
在使用JVM时,可能会遇到一些常见的问题,如内存泄漏、类加载失败等。以下是一些调试技巧:
- 内存泄漏:使用JVM的内存分析工具,如VisualVM,找出内存泄漏的源头。
- 类加载问题:检查类路径是否正确,确保所有依赖的类都能够被正确加载。
- 性能问题:使用JVM的性能分析工具,如JProfiler,找出性能瓶颈并优化。
性能优化与最佳实践
在实际应用中,优化JVM的性能至关重要。以下是一些优化策略:
- JIT编译:利用JVM的即时编译技术,将热点代码编译为机器码,提高执行效率。
- 垃圾回收调优:根据应用特点调整垃圾回收策略,如选择CMS或G1垃圾收集器。
- 内存管理:合理设置JVM的堆大小和栈大小,避免频繁的垃圾回收。
在编程实践中,遵循以下最佳实践可以提高代码的可读性和维护性:
- 代码可读性:使用有意义的变量名和方法名,添加必要的注释,确保代码易于理解。
- 模块化设计:将代码分成多个小模块,方便维护和测试。
- 性能监控:定期使用JVM的监控工具检查应用的性能,及时发现和解决问题。
通过这篇文章,你应该对JVM在Java平台独立性中的角色有了更深入的理解。JVM不仅让Java程序能够跨平台运行,还提供了强大的性能优化和调试工具。在实际开发中,掌握JVM的使用和优化技巧将大大提升你的编程能力。
The above is the detailed content of Explain the role of the Java Virtual Machine (JVM) in Java's platform independence.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










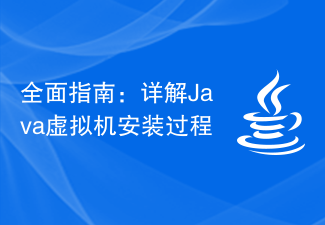
Essentials for Java development: Detailed explanation of Java virtual machine installation steps, specific code examples required. With the development of computer science and technology, the Java language has become one of the most widely used programming languages. It has the advantages of cross-platform and object-oriented, and has gradually become the preferred language for developers. Before using Java for development, you first need to install the Java Virtual Machine (JavaVirtualMachine, JVM). This article will explain in detail the installation steps of the Java virtual machine and provide specific code examples.
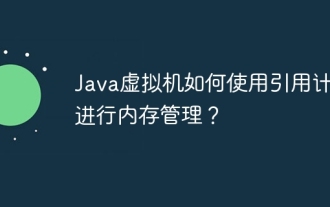
The Java virtual machine uses reference counting to manage memory usage. When the reference count of an object reaches 0, the JVM will perform garbage collection. The reference counting mechanism includes: each object has a counter that stores the number of references pointing to the object. When the object is created, the reference counter is set to 1. When an object is referenced, the reference counter is incremented. When the reference ends, the reference counter is decremented.
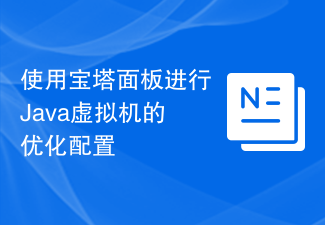
With the continuous development of the Internet, more and more applications and businesses require the use of programs developed in the Java language. For the running of Java programs, the performance of the Java Virtual Machine (JVM) is very important. Therefore, optimizing configuration is an important means to improve the performance of Java applications. Pagoda panel is a commonly used server control panel that can help users manage servers more conveniently. This article will introduce how to use the Pagoda panel to optimize the configuration of the Java virtual machine. Step one: Install Java virtual machine
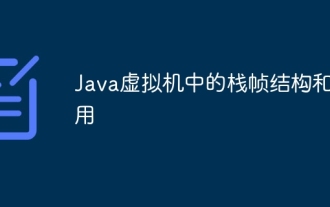
The stack frame is the basic data structure for executing methods in the Java Virtual Machine (JVM), and includes the following parts: Local variable table: stores the local variables of the method. Operand stack: stores operands and intermediate results. Frame data: Contains return address and current program counter. The functions of the stack frame include: storing local variables. Perform operand operations. Handle method calls. Assist with exception handling. Assisted garbage collection.
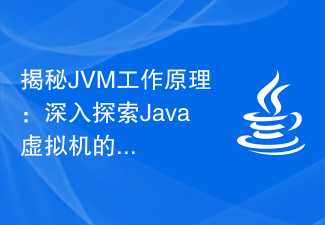
Detailed explanation of JVM principles: In-depth exploration of the working principle of the Java virtual machine requires specific code examples 1. Introduction With the rapid development and widespread application of the Java programming language, the Java Virtual Machine (JavaVirtualMachine, referred to as JVM) has also become indispensable in software development. a part of. As the running environment for Java programs, JVM can provide cross-platform features, allowing Java programs to run on different operating systems. In this article, we will delve into how the JVM works

Explore: The working principle and core functions of the Java Virtual Machine Introduction: The Java Virtual Machine (JavaVirtualMachine, JVM for short) is the core part of Java program running. It is responsible for compiling Java source code into executable bytecode and executing it. This article will delve into the working principles and core functions of the Java virtual machine, and use specific code examples to help readers better understand. 1. Working Principle of Java Virtual Machine 1.1 Class Loader (ClassLoader) J
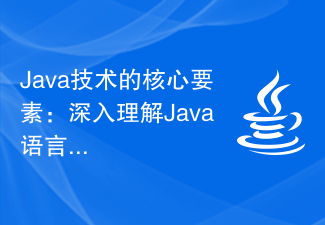
Java core technology stack: In-depth understanding of the Java language, Java virtual machine, and JavaSE library. With the continuous development of computer science and technology, the Java language has become one of the most popular programming languages in the world. As a cross-platform high-level programming language, Java is widely used in various fields, especially in enterprise-level application development and cloud computing. To become an excellent Java developer, you must be proficient in the Java core technology stack, namely Java language, Java virtual machine and Java
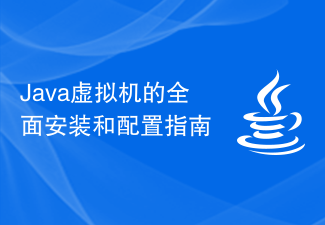
Starting from scratch: Detailed explanation of Java virtual machine installation and configuration [Introduction] Java is a cross-platform programming language, and its execution platform depends on the Java Virtual Machine (JavaVirtualMachine, JVM). By installing and configuring the Java virtual machine, you can run Java programs on different operating systems. This article will take you from scratch, detail how to install and configure a Java virtual machine, and provide some commonly used Java code examples. Let’s start learning! [Part 1: J
