Visual Studio: Code Compilation, Testing, and Deployment
In Visual Studio, the steps for compiling, testing, and deploying the code are as follows: 1. Compiling: Use Visual Studio's compiler options to convert source code into executable files, supporting multiple languages such as C#, C, and Python. 2. Testing: Use built-in frameworks such as MSTest and NUnit to perform unit testing to improve code quality and reliability. 3. Deployment: Transfer applications from the development environment to the production environment through web deployment, Azure deployment, etc. to ensure security and performance.
introduction
Today we are going to talk about Visual Studio, the leader in the developer tool, focusing on the three key links of code compilation, testing and deployment. As an experienced driver with rich programming experience, I know the importance of these links to the success of the project. With this article, you will learn how to efficiently compile, test, and deploy code in Visual Studio, and master some unknown tips and best practices.
Review of basic knowledge
Visual Studio is an integrated development environment (IDE) developed by Microsoft that supports multiple programming languages and platforms. What makes it powerful is that it is not only a code editor, but also integrates compilers, debuggers and project management tools. Familiar with these basic functions is the prerequisite for efficient use of Visual Studio.
In terms of compilation, Visual Studio provides a variety of compiler options, supporting multiple languages from C#, C to Python, and more. During the testing process, Visual Studio integrates a powerful unit testing framework and code coverage analysis tool. In terms of deployment, it supports a variety of deployment strategies from on-premises to the cloud.
Core concept or function analysis
Code Compilation
In Visual Studio, code compilation is the process of converting source code into executable files. This process not only turns the code into a language that the machine can understand, but also includes steps such as error checking and optimization.
// Simple compilation example using System; class Program { static void Main() { Console.WriteLine("Hello, World!"); } }
The above code shows a simple C# program that will generate an executable file after compilation. Visual Studio provides a wealth of compilation options that can be optimized for different needs, such as debug mode and release mode.
Code Testing
Testing is a critical step in ensuring code quality. Visual Studio has built-in testing frameworks such as MSTest and NUnit, allowing developers to write and run unit tests.
// Unit Testing Example using Microsoft.VisualStudio.TestTools.UnitTesting; namespace UnitTestProject1 { [TestClass] public class UnitTest1 { [TestMethod] public void TestMethod1() { Assert.AreEqual(2, 1 1); } } }
Unit testing can help you detect problems in your code early and improve the reliability and maintainability of your code.
Code deployment
Deployment is the process of transferring applications from development environment to production environment. Visual Studio supports a variety of deployment methods, including web deployment, Azure deployment, etc.
// Web deployment example using Microsoft.Web.Deployment; class Program { static void Main() { string siteName = "MyWebSite"; string server = "localhost"; DeploymentBaseOptions deployOptions = new DeploymentBaseOptions(); deployOptions.ComputerName = server; deployOptions.SiteName = siteName; DeploymentChangeSummary summary = DeploymentManager.SyncTo(deployOptions, @"C:\MyWebApp"); Console.WriteLine($"Deployment completed with {summary.Errors} errors and {summary.Warnings} warnings."); } }
The deployment process requires security, performance and other factors to be considered. Visual Studio provides a variety of tools and options to help you complete this process.
Example of usage
Compile and debug
In Visual Studio, compilation and debugging are closely combined. You can set breakpoints, step through the code, view variable values, etc. These functions greatly improve debugging efficiency.
// Debugging example using System; class Program { static void Main() { int x = 5; // Set breakpoint here int y = 10; int result = Add(x, y); Console.WriteLine($"The result is {result}"); } static int Add(int a, int b) { return ab; } }
Automated testing
Automated testing can greatly improve testing efficiency. Visual Studio supports a variety of automation testing tools, such as Selenium, for automated testing of web applications.
// Selenium automation test example using OpenQA.Selenium; using OpenQA.Selenium.Chrome; class Program { static void Main() { IWebDriver driver = new ChromeDriver(); driver.Navigate().GoToUrl("https://www.example.com"); IWebElement element = driver.FindElement(By.Id("myId")); element.Click(); driver.Quit(); } }
Continuous integration and deployment
Continuous integration (CI) and continuous deployment (CD) are at the heart of modern software development. Visual Studio integrates with Azure DevOps to enable automated build, test, and deployment.
// Azure DevOps example using Microsoft.TeamFoundation.Build.WebApi; using Microsoft.VisualStudio.Services.Common; using Microsoft.VisualStudio.Services.WebApi; class Program { static void Main() { VssConnection connection = new VssConnection(new Uri("https://dev.azure.com/yourOrganization"), new VssBasicCredential(string.Empty, "yourPAT")); BuildHttpClient buildClient = connection.GetClient<BuildHttpClient>(); // Trigger the build Build build = buildClient.QueueBuildAsync(new Build { Definition = new BuildDefinitionReference { Id = 1 } }).Result; Console.WriteLine($"Build queued with ID: {build.Id}"); } }
Performance optimization and best practices
In practical applications, how to optimize code is a problem that every developer needs to consider. Visual Studio provides a variety of performance analysis tools, such as performance profilers, to help you find bottlenecks in your code.
// Performance analysis example using System.Diagnostics; class Program { static void Main() { Stopwatch stopwatch = Stopwatch.StartNew(); for (int i = 0; i < 1000000; i ) { // Your code logic} stopwatch.Stop(); Console.WriteLine($"Time elapsed: {stopwatch.ElapsedMilliseconds} ms"); } }
In terms of best practice, it is important to keep the code readable and maintainable. Using meaningful variable names, writing clear comments, and following code style guides are all keys to improving code quality.
Pros and cons and pitfalls
- Compilation Optimization : While Visual Studio provides a wealth of compilation options, over-optimization can make the code difficult to debug. A balance needs to be found between optimization and debugging.
- Test coverage : Although unit testing is very important, excessive pursuit of test coverage may lead to the swelling of test code and reduce development efficiency. The key is to test the critical path and boundary conditions.
- Deployment Security : During the deployment process, security is a common pitfall point. Ensuring that sensitive information is not leaked, using a secure transmission protocol, etc. are all aspects that need to be paid attention to.
Through this article, I hope you can gain a deeper understanding of the powerful capabilities of Visual Studio in code compilation, testing, and deployment. Whether you are a new developer or an experienced veteran, these knowledge and skills can help you easily in your project.
The above is the detailed content of Visual Studio: Code Compilation, Testing, and Deployment. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
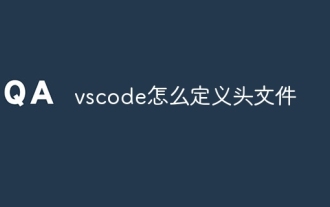
How to define header files using Visual Studio Code? Create a header file and declare symbols in the header file using the .h or .hpp suffix name (such as classes, functions, variables) Compile the program using the #include directive to include the header file in the source file. The header file will be included and the declared symbols are available.

VSCode is a lightweight code editor suitable for multiple languages and extensions; VisualStudio is a powerful IDE mainly used for .NET development. 1.VSCode is based on Electron, supports cross-platform, and uses the Monaco editor. 2. VisualStudio uses Microsoft's independent technology stack to integrate debugging and compiler. 3.VSCode is suitable for simple tasks, and VisualStudio is suitable for large projects.

Code editors that can run on Windows 7 include Notepad, SublimeText, and Atom. 1.Notepad: lightweight, fast startup, suitable for old systems. 2.SublimeText: Powerful and payable. 3.Atom: It is highly customizable, but it starts slowly.

Free versions of VisualStudio include VisualStudioCommunity and VisualStudioCode. 1. VisualStudioCommunity is suitable for individual developers, open source projects and small teams. It is powerful and suitable for individual projects and learning programming. 2. VisualStudioCode is a lightweight code editor that supports multiple programming languages and extensions. It has a fast startup speed and low resource usage, making it suitable for developers who need flexibility and scalability.
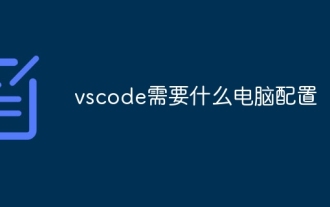
VS Code system requirements: Operating system: Windows 10 and above, macOS 10.12 and above, Linux distribution processor: minimum 1.6 GHz, recommended 2.0 GHz and above memory: minimum 512 MB, recommended 4 GB and above storage space: minimum 250 MB, recommended 1 GB and above other requirements: stable network connection, Xorg/Wayland (Linux)

Windows versions supported by VisualStudio include Windows 10, Windows 11, Windows 7, and Windows 8.1. 1) It is recommended to use Windows 10 or Windows 11 for the latest features and best support. 2) Ensure that the hardware configuration is sufficient, especially when developing large-scale projects. 3) VisualStudio2022 supports Windows 11 more optimized, providing better performance and user experience.
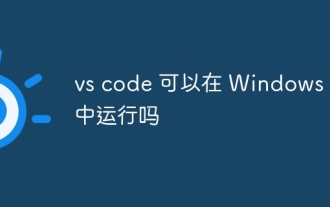
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
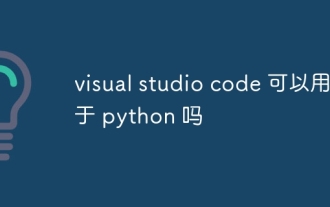
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
