What knowledge do you need to know to modify XML content
XML modification involves modifying its tree structure based on tags and attributes. Use tools such as ElementTree to implement operations, including adding, deleting, modifying, and finding nodes. When optimizing performance, you should avoid frequent searches and modifications, use XPath to locate nodes, organize structure reasonably, and pay attention to coding issues. After modification, use the XML verification tool to verify and develop good code habits to ensure accuracy and maintainability.
XML modification: It's not just adding, deleting, modifying and checking
Are you planning to modify the XML? Don't think that it can be done by simply adding, deleting, modifying and checking. This thing seems simple, but in fact it has hidden mystery. If you are not careful, you may fall into the pit and cannot get out of it for a long time. In this article, let’s take a look at the XML modifications to help you avoid detours. After reading it, you will not only master XML modification skills, but also have a deeper understanding of the underlying mechanism of XML to avoid those crazy bugs.
The essence of XML: Structured data
Don't rush to get started, we have to figure out what XML is. To put it bluntly, XML is a format used to store and transmit data. It uses tags to organize data and form a tree structure. Understanding this is crucial because XML modification is essentially about operating on this tree. You have to understand the hierarchical relationships and attributes of the labels in order to accurately modify the target data. Don't underestimate this tree structure. It determines how you modify it and also determines the efficiency of your code.
Tools and techniques you need to master
It’s not possible to have theory alone, we have to use tools to practice it. Python's xml.etree.ElementTree
module is a good choice. It provides a simple and easy-to-use API to facilitate various operations on XML. Of course, you can also use other languages and libraries, such as Java's DOM API or C#'s XmlDocument class. The principles are similar, but the syntax is slightly different. Remember, choosing the right tool can achieve twice the result with half the effort.
Core operation: the art of adding, deleting, modifying and checking
Now, let’s talk about the specific modification operations.
- Add nodes (new): This is like adding branches and leaves to the tree. You need to create a new node object first and then add it to the child node list of the target node. Don't forget to set the tags and properties of the node. It should be noted here that the location of adding nodes is very important, which directly affects the structure of XML and the meaning of data. If the added location is incorrect, it may cause data parsing errors.
- Delete nodes (delete): This is like pruning a branch. You need to find the target node and remove it from the parent node's child node list. When deleting nodes, be careful not to delete important data by mistake. It is recommended to back up before deletion, or to carefully check the scope of the deletion operation.
- Modify nodes (modify): This is like changing the color of leaves. You can modify the tags, properties, or text content of a node. When modifying, you must ensure the validity and integrity of the data. For example, when modifying attribute values, you must comply with the definition rules of the attribute.
- Finding nodes (query): It's like finding a specific tree in the woods. You need to find the target node based on the node's tag, attribute, or text content.
ElementTree
module provides convenient search methods such asfind()
andfindall()
. Efficient search methods can greatly improve the efficiency of your code.
Code Example (Python):
<code class="python">import xml.etree.ElementTree as ET tree = ET.parse('data.xml') root = tree.getroot() # 查找名为'book'的节点book = root.find('./book[@id="123"]') # 修改节点属性book.set('price', '29.99') # 添加新节点new_chapter = ET.SubElement(book, 'chapter') new_chapter.text = 'A New Chapter' # 删除节点(假设存在名为'old_chapter'的节点) old_chapter = book.find('old_chapter') if old_chapter is not None: book.remove(old_chapter) tree.write('modified_data.xml')</code>
Performance Optimization and Traps
Performance is a key issue when modifying large XML files. Try to avoid frequent node search and modification operations. You can consider using XPath expressions for efficient node positioning. In addition, rationally organizing XML structures can also improve efficiency. Remember, the modification of large XML files should be carried out in stages to avoid memory overflow. Also, XML file encoding issues are also easily overlooked. Be sure to pay attention to the character encoding settings to prevent garbled codes.
Experience:
Don't underestimate XML verification. After modification, be sure to use the XML verification tool to check to ensure that the modified XML file complies with the specifications. This can avoid a lot of unnecessary trouble. Also, develop good code habits and write clear and easy-to-understand code to facilitate maintenance by yourself and others. Finally, only by practicing and summarizing more can we truly master the essence of XML modification.
The above is the detailed content of What knowledge do you need to know to modify XML content. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
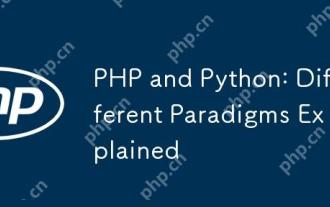
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
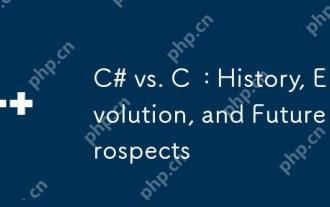
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
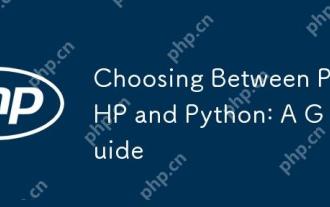
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
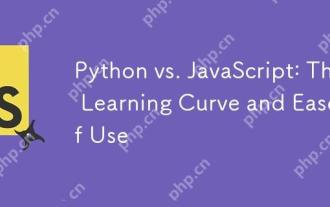
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
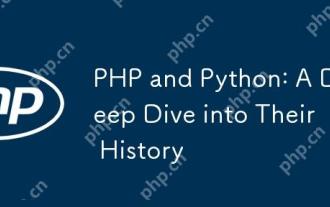
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
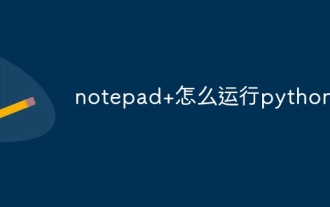
Running Python code in Notepad requires the Python executable and NppExec plug-in to be installed. After installing Python and adding PATH to it, configure the command "python" and the parameter "{CURRENT_DIRECTORY}{FILE_NAME}" in the NppExec plug-in to run Python code in Notepad through the shortcut key "F6".
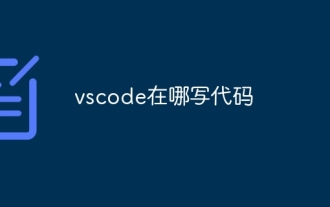
Writing code in Visual Studio Code (VSCode) is simple and easy to use. Just install VSCode, create a project, select a language, create a file, write code, save and run it. The advantages of VSCode include cross-platform, free and open source, powerful features, rich extensions, and lightweight and fast.
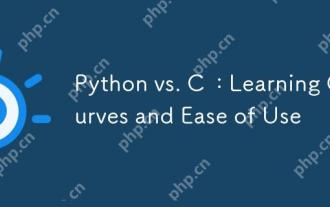
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
