Python vs. C : Learning Curves and Ease of Use
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2. C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
introduction
When it comes to the learning curve and ease of use of programming languages, Python and C are often compared together. Why? Because they represent two extremes in modern programming languages: Python is known for its simplicity and ease of learning, while C is known for its strength and complexity. Today, we will dive into the learning curve and ease of use of these two languages to help you better understand their respective strengths and challenges.
Review of basic knowledge
Both Python and C are very important programming languages, but they differ significantly in design philosophy and application fields. Created by Guido van Rossum in the late 1980s, Python was designed to be an easy-to-learn and use language that emphasizes the readability and simplicity of the code. C was developed by Bjarne Stroustrup in the early 1980s and is an extension of the C language, aiming to provide higher programming flexibility and performance.
Python's syntax is simple and close to natural language, which makes it ideal for beginners. Its dynamic typing system and automatic memory management allow developers to focus on logic rather than detail. C provides lower-level control, supporting advanced features such as object-oriented programming, generic programming and multiple inheritance, but this also means higher learning barriers and more complex code management.
Core concept or function analysis
Python's learning curve and ease of use
Python's learning curve is relatively flat, thanks to its concise syntax and rich standard library. Let's look at a simple Python code example:
# Calculate the sum of all numbers in a list = [1, 2, 3, 4, 5] total = sum(numbers) print(f"The sum of the numbers is: {total}")
This snippet demonstrates the simplicity and readability of Python. Python's dynamic typing system and automatic memory management allow developers to get started quickly and focus on solving problems rather than the language itself.
However, Python's ease of use also presents some challenges. For example, a dynamic type system, while convenient, can also lead to runtime errors, which requires developers to be more careful when writing code. Furthermore, the explanatory way Python executes can be sacrificed in performance, especially when dealing with large amounts of data or high-performance computing.
C's learning curve and ease of use
The learning curve of C is steeper, mainly because of its complex grammar and rich features. Let's look at a simple C code example:
#include <iostream> #include <vector> #include <numeric> int main() { std::vector<int> numbers = {1, 2, 3, 4, 5}; int total = std::accumulate(numbers.begin(), numbers.end(), 0); std::cout << "The sum of the numbers is: " << total << std::endl; return 0; }
This code snippet demonstrates the power and complexity of C. C provides fine-grained control of memory and performance, which makes it very popular in system programming and high-performance computing. However, this control also means that developers need to deal with more details, such as manual memory management and type safety, which increases the difficulty of learning and use.
The complexity of C also brings more optimization opportunities and flexibility, but it also means higher error risk and longer development cycles. Especially for beginners, understanding concepts such as pointers, memory management, and templates of C can be a huge challenge.
Example of usage
Basic usage of Python
The basic usage of Python is very intuitive, let's look at a simple file reading and processing example:
# Read and process a text file with open('example.txt', 'r') as file: content = file.read() words = content.split() print(f"Total words: {len(words)}")
This code snippet shows the simplicity of Python's file operations and string processing. Python's with
statements and built-in functions such as split
and len
allow developers to quickly complete common tasks.
Basic usage of C
The basic usage of C requires more code and more detailed control. Let's look at a similar file reading and processing example:
#include <iostream> #include <fstream> #include <string> #include <vector> #include <sstream> int main() { std::ifstream file("example.txt"); if (!file.is_open()) { std::cerr << "Unable to open file" << std::endl; return 1; } std::string content((std::isriseambuf_iterator<char>(file)), std::isriseambuf_iterator<char>()); std::istringstream iss(content); std::vector<std::string> words; std::string word; while (iss >> word) { words.push_back(word); } std::cout << "Total words: " << words.size() << std::endl; file.close(); return 0; }
This code snippet demonstrates the complexity of file operations and string processing in C. C requires manual management of file opening and closing, handling errors, and using more standard libraries to accomplish the same task.
Common Errors and Debugging Tips
Common errors in Python include indentation errors, type errors, and runtime errors. Debugging tips include debugging using pdb
modules, logging using print
statements, and using exception handling to catch and handle errors.
In C, common errors include memory leaks, pointer errors, and compilation errors. Debugging tips include using debuggers such as gdb
, logging with cout
statements, and using exception handling to catch and handle errors.
Performance optimization and best practices
Performance optimization of Python
Python's performance optimization mainly focuses on the following aspects:
- Use libraries such as
numpy
andpandas
for efficient data processing - Parallel calculations using
multiprocessing
orthreading
modules - Use tools such as
cython
ornumba
for code compilation and optimization
For example, using numpy
can significantly improve the performance of array operations:
import numpy as np # Use numpy for array operations arr = np.array([1, 2, 3, 4, 5]) total = np.sum(arr) print(f"The sum of the numbers is: {total}")
Performance optimization of C
C's performance optimization is more complex and diverse, including:
- Use containers such as
std::vector
andstd::array
for efficient data management - Use
std::algorithm
library for efficient algorithm implementation - Code optimization using compiler optimization options and manual inline functions
For example, using std::vector
and std::accumulate
can efficiently calculate the sum of an array:
#include <iostream> #include <vector> #include <numeric> int main() { std::vector<int> numbers = {1, 2, 3, 4, 5}; int total = std::accumulate(numbers.begin(), numbers.end(), 0); std::cout << "The sum of the numbers is: " << total << std::endl; return 0; }
Best Practices
In Python, best practices include:
- Writing highly readable code with PEP 8 style guide
- Manage dependencies using virtual environment
- Writing unit tests and integration tests
In C, best practices include:
- Write highly readable code with a consistent coding style
- Use smart pointers to manage memory to avoid memory leaks
- Write unit tests and integration tests, using frameworks such as
googletest
in conclusion
Python and C have their own advantages in learning curve and ease of use. Python is known for its simplicity and ease of learning, suitable for beginners and rapid prototyping; while C is known for its power and complexity, suitable for applications requiring high performance and low-level control. Which language you choose depends on your needs and goals, but no matter which one you choose, you need to constantly learn and practice in order to truly master its essence.
The above is the detailed content of Python vs. C : Learning Curves and Ease of Use. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
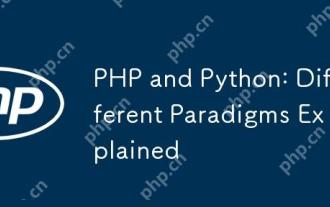
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
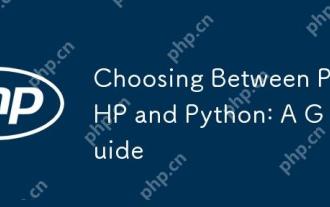
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
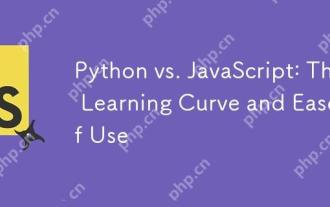
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
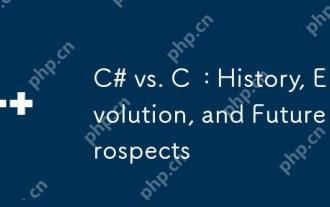
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
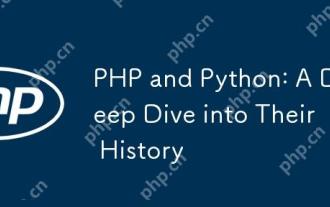
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
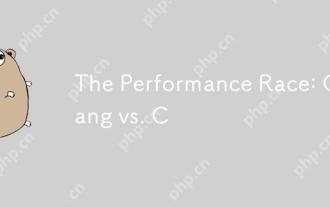
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
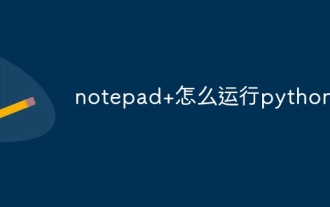
Running Python code in Notepad requires the Python executable and NppExec plug-in to be installed. After installing Python and adding PATH to it, configure the command "python" and the parameter "{CURRENT_DIRECTORY}{FILE_NAME}" in the NppExec plug-in to run Python code in Notepad through the shortcut key "F6".
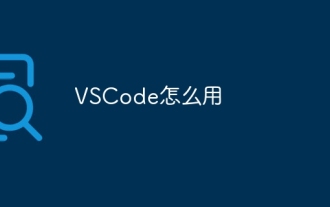
Visual Studio Code (VSCode) is a cross-platform, open source and free code editor developed by Microsoft. It is known for its lightweight, scalability and support for a wide range of programming languages. To install VSCode, please visit the official website to download and run the installer. When using VSCode, you can create new projects, edit code, debug code, navigate projects, expand VSCode, and manage settings. VSCode is available for Windows, macOS, and Linux, supports multiple programming languages and provides various extensions through Marketplace. Its advantages include lightweight, scalability, extensive language support, rich features and version
