


What is serialization in PHP and what are potential security risks?
Serialization in PHP is a process of converting objects or data structures into strings, which are mainly implemented through serialize() and unserialize() functions. Serialization is used to save object state for delivery between different requests or systems. Potential security risks include object injection attacks and information leakage. Avoiding methods include: 1. Limit deserialized classes and use the second parameter of the unserialize() function; 2. Verify the data source to ensure it comes from a trusted source; 3. Consider using more secure data formats such as JSON.
introduction
Today we will talk about serialization in PHP. This topic is not only the basic skills that PHP developers must master, but also the key to understanding data storage and transmission. Through this article, you will not only learn about the basic concepts and implementation methods of serialization, but also explore its potential security risks and how to avoid them.
After you read this article, you will be able to handle serialization issues in PHP with confidence and be able to identify and prevent serialization-related security vulnerabilities.
Review of basic knowledge
In PHP, serialization is the process of converting an object or data structure into a string that can be stored or transmitted over the network. When using this data, it can be converted back to the original data structure by deserialization.
Serialization is mainly implemented in PHP through serialize()
and unserialize()
functions. They are built-in functions in PHP that provide the ability to convert complex data types into strings and recover data from strings.
Core concept or function analysis
Definition and function of serialization
Serialization is mainly used in PHP to save the state of an object so as to pass objects between different requests or between different systems. Its advantage is the ability to store and transmit complex data structures in a simple way.
For example, suppose you have an object containing user information that you can serialize and store in a database or transfer to another system via an API.
$user = (object) ['name' => 'John Doe', 'age' => 30]; $serializedUser = serialize($user); echo $serializedUser; // Output the serialized string
How it works
When you call the serialize()
function, PHP will iterate through all elements in the object or array and convert them into a special format string. This string contains the object's class name, attributes, and their values.
The deserialization process is to parse the string back to the original data structure. PHP rebuilds objects or arrays based on the information in the string.
It should be noted that the serialization and deserialization process may involve some performance overhead, especially when dealing with large data structures. In addition, deserialization requires ensuring the integrity and security of the data, as malicious data can lead to security vulnerabilities.
Example of usage
Basic usage
Serialization and deserialization are the most common uses, and here is a simple example:
// Serialize $data = ['name' => 'Alice', 'age' => 25]; $serializedData = serialize($data); echo $serializedData; // Output the serialized string// Deserialize $unserializedData = unserialize($serializedData); print_r($unserializedData); // Output the deserialized array
The function of each line is very clear: serialize()
converts the array into a string, unserialize()
converts the string back to the array.
Advanced Usage
In some cases, you may need to serialize the object and want to be able to call a specific method to restore the state of the object when deserializing. At this time, you can use __sleep()
and __wakeup()
magic methods.
class User { private $name; private $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } public function __sleep() { // Called before serialization, return the attribute that needs to be serialized return ['name', 'age']; } public function __wakeup() { // Call after deserialization to restore the state of the object echo "User object unserialized.\n"; } } $user = new User('Bob', 35); $serializedUser = serialize($user); echo $serializedUser; // Output the serialized string $unserializedUser = unserialize($serializedUser); // Output: User object unserialized.
This method is suitable for experienced developers because it involves the management of object life cycles and the use of magic methods.
Common Errors and Debugging Tips
Common errors in the process of serialization and deserialization include:
- Data Loss : If a serialized data structure contains non-serialized elements (such as resource types), these elements are lost during the serialization process.
- Security vulnerability : Malicious data may lead to code execution or information leakage.
Methods to debug these problems include:
- Use
var_dump()
orprint_r()
to view the serialized and deserialized data structures to ensure data integrity. - For security issues, make sure to deserialize only trusted data sources and use the second parameter of
unserialize()
function to limit the deserialized classes.
Performance optimization and best practices
In practical applications, it is very important to optimize the performance of serialization and deserialization. Here are some suggestions:
- Choose the right data format : PHP's serialization format may not be the most compact, if data needs to be transferred frequently, consider using JSON or other more compact formats.
- Avoid serializing large data structures : If possible, try to avoid serializing large data structures, as this increases performance overhead.
Comparing the performance differences between different methods, you can use PHP's microtime()
function to measure execution time. For example:
$data = range(1, 10000); $start = microtime(true); $serialized = serialize($data); $end = microtime(true); echo "Serialize time: " . ($end - $start) . " seconds\n"; $start = microtime(true); $json = json_encode($data); $end = microtime(true); echo "JSON encode time: " . ($end - $start) . " seconds\n";
This example shows the performance differences between serialization and JSON encoding, helping you choose a more suitable solution.
Potential security risks
Serialization has some potential security risks in PHP, mainly including:
- Object injection attack : Malicious users can execute arbitrary code during deserialization by constructing special serialized strings. This is because PHP allows automatic calls to objects' methods such as
__wakeup()
or__destruct()
when deserialized. - Information leakage : Serialized data may contain sensitive information, which may cause security issues if it is leaked.
How to avoid security risks
To avoid these security risks, the following measures can be taken:
- Restrict deserialized classes : Use the second parameter of
unserialize()
function to restrict classes that can be deserialized. For example:
$safeData = unserialize($serializedData, ["allowed_classes" => false]);
This prevents object injection attacks, as it only allows deserialization of scalar types and arrays.
- Verify data sources : Make sure to deserialize only data from trusted sources and avoid processing of user input data.
- Use alternatives : Consider using JSON or other safer data formats instead of PHP serialization, especially when processing user input data.
Through these methods, you can significantly reduce the security risks associated with serialization and ensure that your PHP applications are safer and more reliable.
I hope this article will be helpful for your understanding of serialization in PHP, and also remind you to pay attention to potential security risks. I wish you all the best on the PHP development journey!
The above is the detailed content of What is serialization in PHP and what are potential security risks?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
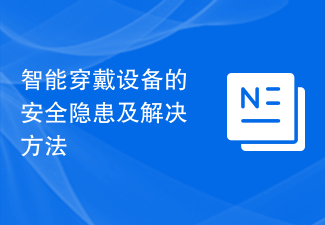
With the development of technology, the demand for smart wearable devices continues to rise. People now not only rely on watches to know the time, but also use smart watches or smart glasses to receive information, record exercise, detect health conditions, and more. However, these smart wearable devices also bring security risks. This article will discuss the safety hazards and solutions of smart wearable devices. 1. Security risks Data privacy leakage Smart wearable devices can collect a variety of personal data of users, such as physical health data, location data, social media activities, etc. However, these data may be

In the modern Internet architecture, Nginx, as an advanced web server and reverse proxy tool, is increasingly used in enterprise production environments. However, in actual use, administrators need to perform security downgrade operations on Nginx due to various reasons. Security downgrade means minimizing the security threats that the system exposes to the outside world while ensuring normal system functions. This article will explore the security risks and management best practices of using Nginx for secure downgrade. 1. Security Risks Using Nginx for Security
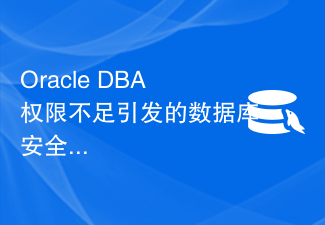
Database security risks caused by insufficient OracleDBA permissions With the rapid development of the Internet, databases, as an important information storage and management tool for enterprises, carry a large amount of sensitive data. In this process, the database administrator (DBA) plays a vital role and is responsible for ensuring the normal operation of the database and the security of the data. However, due to work requirements or management policies, the DBA's authority is sometimes restricted, which may cause database security risks. This article will introduce the possible consequences of insufficient DBA authority in Oracle database

Serialization in PHP is a process of converting objects or data structures into strings, which are mainly implemented through serialize() and unserialize() functions. Serialization is used to save object state for delivery between different requests or systems. Potential security risks include object injection attacks and information leakage. Avoiding methods include: 1. Limit deserialized classes and use the second parameter of the unserialize() function; 2. Verify the data source to ensure it comes from a trusted source; 3. Consider using more secure data formats such as JSON.
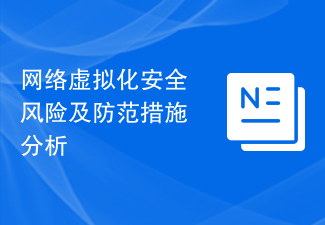
With the continuous development of information technology, virtualization technology has become one of the important supporting technologies for modern enterprise informatization. With the help of virtualization technology, enterprises can virtualize multiple physical hosts into one or more virtual hosts, thereby maximizing resource utilization, improving server usage efficiency, and reducing enterprise operating costs. At the same time, virtualization technology can also improve the business continuity and flexibility of enterprises by implementing functions such as isolation, dynamic migration, and snapshot backup of virtual machines. However, although virtualization technology brings many benefits, it also creates
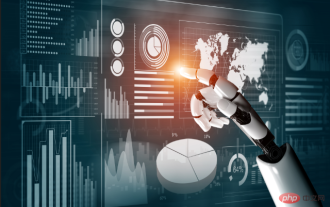
Since ChatGPT was officially launched in November 2022, millions of users have poured in crazily. Due to its excellent human-like language generation capabilities, programming software talent, and lightning-fast text analysis capabilities, ChatGPT has quickly become the tool of choice for developers, researchers, and everyday users. As with any disruptive technology, generative AI systems like ChatGPT have potential risks. In particular, major players in the technology industry, national intelligence agencies, and other government agencies have issued warnings about feeding sensitive information into artificial intelligence systems such as ChatGPT. Concerns about the security risks posed by ChatGPT stem from the possibility that information could ultimately be leaked into the public domain through ChatGPT, whether through security
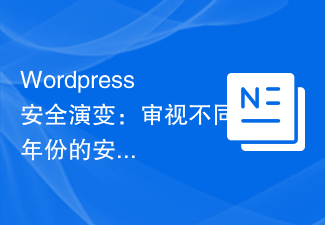
As one of the most popular content management systems in the world, WordPress’s security evolution has also attracted much attention over time. This article will examine WordPress security risks and protective measures in different years to help readers better understand the development process of WordPress security. When WordPress was first launched in 2003, security threats at the time focused on basic vulnerabilities and weak passwords. Although the first version of WordPress was relatively simple, security issues were not uncommon. website
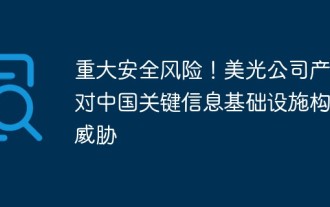
According to news on May 22, China’s Cybersecurity Review Office recently conducted a cybersecurity review of products sold in China by US storage solution provider Micron in accordance with laws and regulations. After review, it was found that Micron's products have serious cybersecurity issues, which may bring major security risks to China's critical information infrastructure supply chain and pose a threat to national security. Based on the necessary measures to safeguard national security, the Cybersecurity Review Office concluded in accordance with the law that the cyber security review would not be passed. According to relevant laws and regulations such as the Cybersecurity Law, operators of critical information infrastructure in China should stop purchasing Micron products. This cyber security review of Micron's products aims to prevent cyber security problems that may be caused by the products to ensure that the country
