How do I create, read, update, and delete (CRUD) documents in MongoDB?
This article details performing Create, Read, Update, and Delete (CRUD) operations in MongoDB. It covers using methods like insertOne(), find(), updateOne(), deleteOne(), and best practices for efficient operations including indexing, batch operatio
How to Perform CRUD Operations in MongoDB
This section details how to perform Create, Read, Update, and Delete (CRUD) operations in MongoDB. We'll use the example of a collection named "products" with documents containing _id
, name
, price
, and description
fields.
Creating Documents: To create a new document (insert), you use the insertOne()
method (for a single document) or insertMany()
(for multiple documents). Here's an example using the MongoDB shell:
db.products.insertOne( { name: "Laptop", price: 1200, description: "Powerful laptop" } ) db.products.insertMany( [ { name: "Tablet", price: 300, description: "Android tablet" }, { name: "Keyboard", price: 75, description: "Mechanical keyboard" } ] )
This code inserts one laptop document and then inserts multiple documents (tablet and keyboard) in a single operation. The _id
field is automatically generated if not provided. Driver-specific implementations will be similar, using the respective driver's insertOne()
or insertMany()
methods.
Reading Documents: Reading documents involves querying the collection using find()
. You can specify filters to retrieve specific documents. For example:
// Find all products db.products.find() // Find products with price less than 500 db.products.find( { price: { $lt: 500 } } ) // Find a single product by its ID db.products.findOne( { _id: ObjectId("...") } ) // Replace ... with the actual ObjectId
find()
returns a cursor, allowing you to iterate through the results. findOne()
returns a single document matching the query. Again, driver implementations will have equivalent methods.
Updating Documents: MongoDB provides several ways to update documents. updateOne()
updates a single document, while updateMany()
updates multiple documents. You use the $set
operator to modify fields.
// Update the price of a specific product db.products.updateOne( { name: "Laptop" }, { $set: { price: 1300 } } ) // Increase the price of all products by 10% db.products.updateMany( {}, { $inc: { price: { $multiply: [ 0.1, "$price" ] } } } )
The first example updates the price of a laptop. The second example uses the $inc
operator to increment the price of all products. More complex updates can be achieved using other update operators like $push
, $pull
, $addToSet
, etc.
Deleting Documents: deleteOne()
removes a single document, and deleteMany()
removes multiple documents.
// Delete a specific product db.products.deleteOne( { name: "Keyboard" } ) // Delete all products with price greater than 1000 db.products.deleteMany( { price: { $gt: 1000 } } )
These commands remove documents based on the provided criteria.
Best Practices for Efficient CRUD Operations in MongoDB
Efficient CRUD operations require careful consideration of several factors:
- Indexing: Create indexes on frequently queried fields to speed up
find()
operations. Indexes are similar to indices in relational databases. Choose appropriate index types (e.g., single-field, compound, geospatial) based on your queries. - Batch Operations: Use
insertMany()
,updateMany()
, anddeleteMany()
to perform operations on multiple documents in a single batch, significantly improving performance compared to individual operations. - Data Modeling: Design your data model carefully to minimize the number of queries needed for common operations. Proper schema design can dramatically improve query performance.
- Query Optimization: Avoid using
$where
clauses as they can be slow. Instead, use operators like$gt
,$lt
,$in
, etc., which leverage indexes effectively. Use appropriate projection ({ _id: 0, name: 1, price: 1 }
) to retrieve only the necessary fields, reducing data transfer. - Connection Pooling: Use connection pooling to reuse database connections, reducing the overhead of establishing new connections for each operation. Most drivers offer built-in connection pooling capabilities.
Handling Errors During CRUD Operations
Error handling is crucial for robust MongoDB applications. Drivers provide mechanisms to catch and handle exceptions during CRUD operations.
- Try-Catch Blocks: Wrap your CRUD operations within
try-catch
blocks to handle potential errors like network issues, invalid data, or database errors. - Error Codes: Examine the error codes returned by the database to determine the cause of the error and implement appropriate logic to handle it gracefully. MongoDB provides detailed error code documentation.
- Logging: Log errors for debugging and monitoring purposes. Include relevant information such as the error message, stack trace, and the operation that failed.
- Retry Mechanism: Implement a retry mechanism for transient errors (e.g., network timeouts). Retry a failed operation after a short delay to improve resilience.
Performing CRUD Operations with Different Drivers
The specific methods for performing CRUD operations vary slightly depending on the driver used. Here's a brief overview for Node.js and Python:
Node.js (using the mongodb
driver):
const { MongoClient } = require('mongodb'); // ... connection code ... const client = new MongoClient(uri); async function run() { try { await client.connect(); const db = client.db('mydatabase'); const collection = db.collection('products'); // ... CRUD operations using collection.insertOne(), collection.find(), etc. ... } finally { await client.close(); } } run().catch(console.dir);
Python (using the pymongo
driver):
import pymongo # ... connection code ... client = pymongo.MongoClient(uri) db = client['mydatabase'] collection = db['products'] # ... CRUD operations using collection.insert_one(), collection.find(), etc. ... client.close()
Both examples demonstrate the basic structure. Consult the documentation for your specific driver for detailed information on the available methods and options. Remember to replace placeholders like uri
with your actual connection string.
The above is the detailed content of How do I create, read, update, and delete (CRUD) documents in MongoDB?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

The core strategies of MongoDB performance tuning include: 1) creating and using indexes, 2) optimizing queries, and 3) adjusting hardware configuration. Through these methods, the read and write performance of the database can be significantly improved, response time, and throughput can be improved, thereby optimizing the user experience.
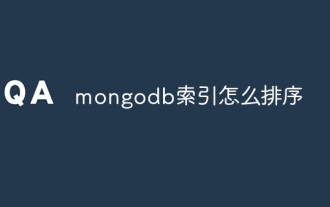
Sorting index is a type of MongoDB index that allows sorting documents in a collection by specific fields. Creating a sort index allows you to quickly sort query results without additional sorting operations. Advantages include quick sorting, override queries, and on-demand sorting. The syntax is db.collection.createIndex({ field: <sort order> }), where <sort order> is 1 (ascending order) or -1 (descending order). You can also create multi-field sorting indexes that sort multiple fields.

The main tools for connecting to MongoDB are: 1. MongoDB Shell, suitable for quickly viewing data and performing simple operations; 2. Programming language drivers (such as PyMongo, MongoDB Java Driver, MongoDB Node.js Driver), suitable for application development, but you need to master the usage methods; 3. GUI tools (such as Robo 3T, Compass) provide a graphical interface for beginners and quick data viewing. When selecting tools, you need to consider application scenarios and technology stacks, and pay attention to connection string configuration, permission management and performance optimization, such as using connection pools and indexes.

MongoDB is more suitable for processing unstructured data and rapid iteration, while Oracle is more suitable for scenarios that require strict data consistency and complex queries. 1.MongoDB's document model is flexible and suitable for handling complex data structures. 2. Oracle's relationship model is strict to ensure data consistency and complex query performance.
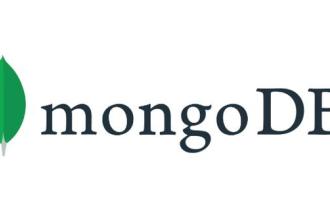
Choosing MongoDB or relational database depends on application requirements. 1. Relational databases (such as MySQL) are suitable for applications that require high data integrity and consistency and fixed data structures, such as banking systems; 2. NoSQL databases such as MongoDB are suitable for processing massive, unstructured or semi-structured data and have low requirements for data consistency, such as social media platforms. The final choice needs to weigh the pros and cons and decide based on the actual situation. There is no perfect database, only the most suitable database.
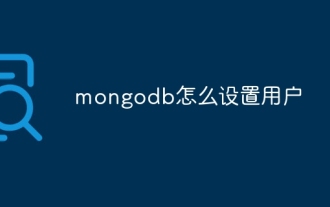
To set up a MongoDB user, follow these steps: 1. Connect to the server and create an administrator user. 2. Create a database to grant users access. 3. Use the createUser command to create a user and specify their role and database access rights. 4. Use the getUsers command to check the created user. 5. Optionally set other permissions or grant users permissions to a specific collection.
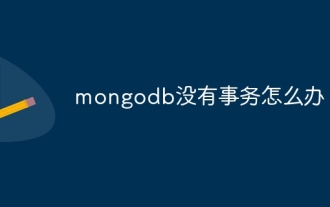
MongoDB lacks transaction mechanisms, which makes it unable to guarantee the atomicity, consistency, isolation and durability of database operations. Alternative solutions include verification and locking mechanisms, distributed transaction coordinators, and transaction engines. When choosing an alternative solution, its complexity, performance, and data consistency requirements should be considered.
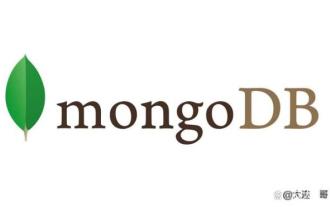
This article explains the advanced MongoDB query skills, the core of which lies in mastering query operators. 1. Use $and, $or, and $not combination conditions; 2. Use $gt, $lt, $gte, and $lte for numerical comparison; 3. $regex is used for regular expression matching; 4. $in and $nin match array elements; 5. $exists determine whether the field exists; 6. $elemMatch query nested documents; 7. Aggregation Pipeline is used for more powerful data processing. Only by proficiently using these operators and techniques and paying attention to index design and performance optimization can you conduct MongoDB data queries efficiently.
