Python Best Practices: Writing Clean and Maintainable Code
Python's simplicity and readability make it a fantastic language for both beginners and experienced developers. However, writing clean, maintainable code requires more than just basic syntax knowledge. In this guide, we'll explore essential best practices that will elevate your Python code quality.
The Power of PEP 8
PEP 8 is Python's style guide, and following it consistently makes your code more readable and maintainable. Let's look at some key principles:
# Bad example def calculate_total(x,y,z): return x+y+z # Good example def calculate_total(price, tax, shipping): """Calculate the total cost including tax and shipping.""" return price + tax + shipping
Embrace Type Hints
Python 3's type hints improve code clarity and enable better tooling support:
from typing import List, Dict, Optional def process_user_data( user_id: int, settings: Dict[str, str], tags: Optional[List[str]] = None ) -> bool: """Process user data and return success status.""" if tags is None: tags = [] # Processing logic here return True
Context Managers for Resource Management
Using context managers with the with statement ensures proper resource cleanup:
# Bad approach file = open('data.txt', 'r') content = file.read() file.close() # Good approach with open('data.txt', 'r') as file: content = file.read() # File automatically closes after the block
Implement Clean Error Handling
Proper exception handling makes your code more robust:
def fetch_user_data(user_id: int) -> dict: try: # Attempt to fetch user data user = database.get_user(user_id) return user.to_dict() except DatabaseConnectionError as e: logger.error(f"Database connection failed: {e}") raise except UserNotFoundError: logger.warning(f"User {user_id} not found") return {}
Use List Comprehensions Wisely
List comprehensions can make your code more concise, but don't sacrifice readability:
# Simple and readable - good! squares = [x * x for x in range(10)] # Too complex - break it down # Bad example result = [x.strip().lower() for x in text.split(',') if x.strip() and not x.startswith('#')] # Better approach def process_item(item: str) -> str: return item.strip().lower() def is_valid_item(item: str) -> bool: item = item.strip() return bool(item) and not item.startswith('#') result = [process_item(x) for x in text.split(',') if is_valid_item(x)]
Dataclasses for Structured Data
Python 3.7 dataclasses reduce boilerplate for data containers:
from dataclasses import dataclass from datetime import datetime @dataclass class UserProfile: username: str email: str created_at: datetime = field(default_factory=datetime.now) is_active: bool = True def __post_init__(self): self.email = self.email.lower()
Testing is Non-Negotiable
Always write tests for your code using pytest:
import pytest from myapp.calculator import calculate_total def test_calculate_total_with_valid_inputs(): result = calculate_total(100, 10, 5) assert result == 115 def test_calculate_total_with_zero_values(): result = calculate_total(100, 0, 0) assert result == 100 def test_calculate_total_with_negative_values(): with pytest.raises(ValueError): calculate_total(100, -10, 5)
Conclusion
Writing clean Python code is an ongoing journey. These best practices will help you write more maintainable, readable, and robust code. Remember:
- Follow PEP 8 consistently
- Use type hints for better code clarity
- Implement proper error handling
- Write tests for your code
- Keep functions and classes focused and single-purpose
- Use modern Python features appropriately
What best practices do you follow in your Python projects? Share your thoughts and experiences in the comments below!
The above is the detailed content of Python Best Practices: Writing Clean and Maintainable Code. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
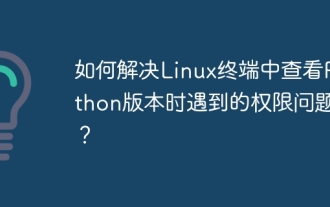
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
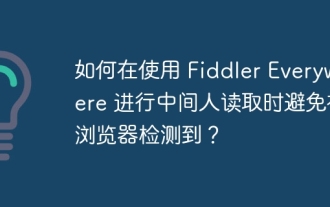
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
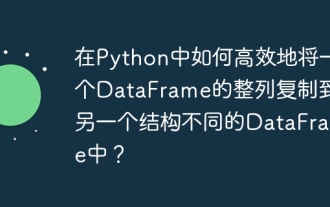
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
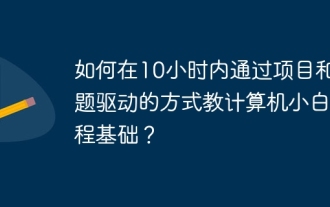
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
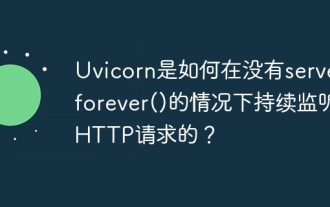
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
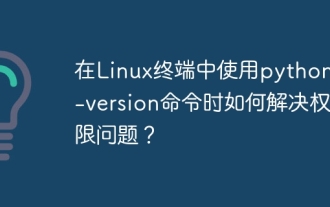
Using python in Linux terminal...
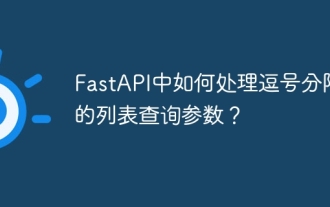
Fastapi ...
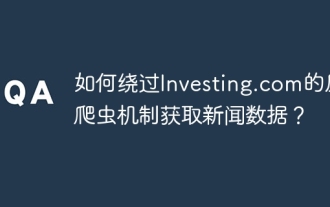
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
