


How Can I Save and Load Custom Python Objects for Data Persistence?
Saving Python Objects for Data Persistence
Question:
I have created a custom Python object and would like to save it for later use. How can I achieve this for data persistence?
Saving Objects with the Pickle Module:
The Pickle module in Python's standard library provides a convenient way to save objects for data persistence. Consider the following example with a custom object:
import pickle class Company: def __init__(self, name, value): self.name = name self.value = value company1 = Company('banana', 40)
To save this object, we can use the following code:
with open('company_data.pkl', 'wb') as outp: pickle.dump(company1, outp, pickle.HIGHEST_PROTOCOL)
This will create a pickle file containing the serialized representation of the company1 object.
Reading Saved Objects:
Once saved, the object can be deserialized and retrieved later:
with open('company_data.pkl', 'rb') as inp: company1 = pickle.load(inp)
company1 now contains the original object, with its name and value attributes intact.
Additional Considerations:
- cPickle vs Pickle: cPickle, the C version of pickle, offers improved performance. If available, use import cPickle as pickle.
- Data Stream Formats (Protocols): Pickle supports different data stream formats. For highest compatibility, use pickle.HIGHEST_PROTOCOL (-1).
- Saving Multiple Objects: Pickle can store multiple objects in a single file. Use containers like lists or dictionaries to group them.
The above is the detailed content of How Can I Save and Load Custom Python Objects for Data Persistence?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
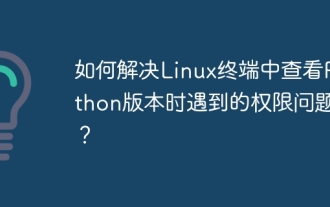
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
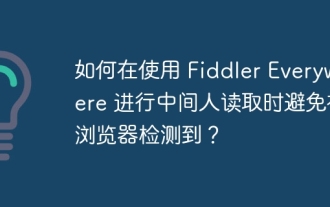
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
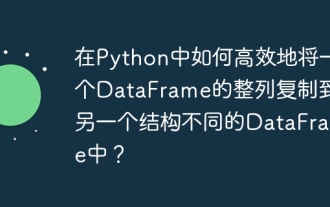
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
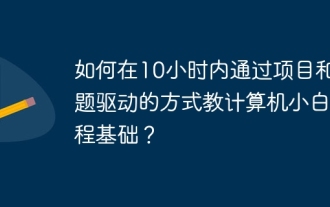
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
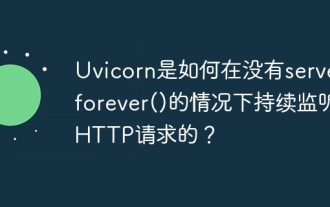
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
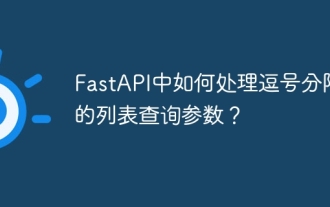
Fastapi ...
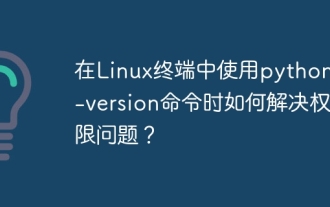
Using python in Linux terminal...
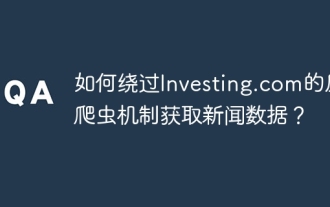
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
