


How Can I Ensure My Python Classes Are Truly Equal: A Guide to Equivalence Methods
Keeping Your Python Classes Equal: A Comprehensive Guide to Equivalence Methods
In Python, the eq and ne special methods provide a convenient way to define equivalence for custom classes. While the basic approach of comparing __dict__s is a viable option, it may face challenges with subclasses and interoperability with other types.
A More Robust Equivalence Handling
To address these limitations, consider a more comprehensive implementation:
class Number: def __init__(self, number): self.number = number def __eq__(self, other): if isinstance(other, Number): return self.number == other.number return NotImplemented def __ne__(self, other): x = self.__eq__(other) if x is not NotImplemented: return not x return NotImplemented def __hash__(self): return hash(tuple(sorted(self.__dict__.items()))) class SubNumber(Number): pass
This version includes:
- Subclass Handling: It ensures that equivalence is checked properly between the Number class and its subclasses.
- Non-commutative Correction: It ensures that equality comparisons are commutative, regardless of the operand order.
- Hash Overriding: By defining a custom hash method, it ensures that objects with the same value have the same hash value, which is crucial for set and dictionary operations.
Validation and Testing
To verify the robustness of this approach, here's a set of assertions:
n1 = Number(1) n2 = Number(1) n3 = SubNumber(1) n4 = SubNumber(4) assert n1 == n2 assert n2 == n1 assert not n1 != n2 assert not n2 != n1 assert n1 == n3 assert n3 == n1 assert not n1 != n3 assert not n3 != n1 assert not n1 == n4 assert not n4 == n1 assert n1 != n4 assert n4 != n1 assert len(set([n1, n2, n3])) == 1 assert len(set([n1, n2, n3, n4])) == 2
These assertions demonstrate the correct behavior of the equivalence methods and the consistency of hash values.
By embracing this more comprehensive approach, you can create Python classes with robust equivalence handling, ensuring reliable comparisons and accurate set and dictionary operations.
The above is the detailed content of How Can I Ensure My Python Classes Are Truly Equal: A Guide to Equivalence Methods. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
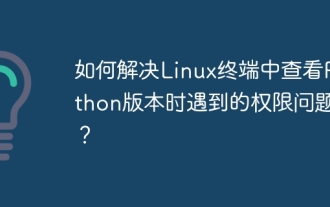
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
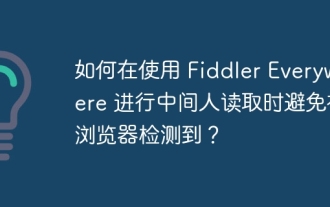
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
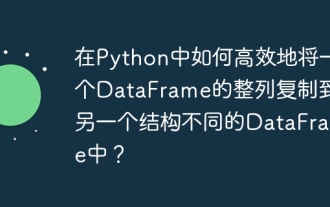
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
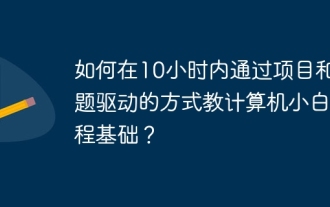
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
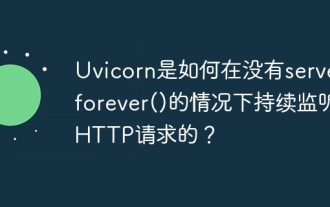
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
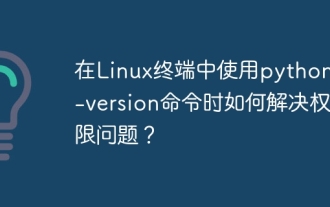
Using python in Linux terminal...
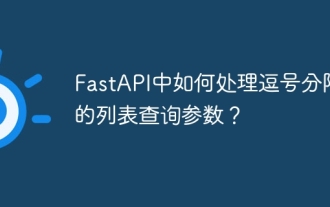
Fastapi ...
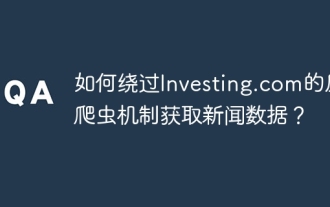
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
