How to Authenticate Users with Email in Django?
Django Authentication with Email
In Django, the default authentication mechanism utilizes usernames for login credentials. However, certain scenarios may necessitate authenticating users through their email addresses instead. To achieve this, creating a custom authentication backend is the recommended approach.
Custom Authentication Backend
The following Python code exemplifies a custom authentication backend that authenticates users based on their email addresses:
<code class="python">from django.contrib.auth import get_user_model from django.contrib.auth.backends import ModelBackend class EmailBackend(ModelBackend): def authenticate(self, request, username=None, password=None, **kwargs): UserModel = get_user_model() try: user = UserModel.objects.get(email=username) except UserModel.DoesNotExist: return None else: if user.check_password(password): return user return None</code>
Configuration
To utilize the custom authentication backend, add the following to your Django project's settings:
<code class="python">AUTHENTICATION_BACKENDS = ['path.to.auth.module.EmailBackend']</code>
Usage
With the custom authentication backend in place, you can authenticate users via email using the following steps:
<code class="python"># Get email and password from the request email = request.POST['email'] password = request.POST['password'] # Authenticate the user user = authenticate(username=email, password=password) # Log in the user if authentication was successful if user is not None: login(request, user)</code>
This approach allows for user authentication through their email addresses without the need for usernames.
The above is the detailed content of How to Authenticate Users with Email in Django?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
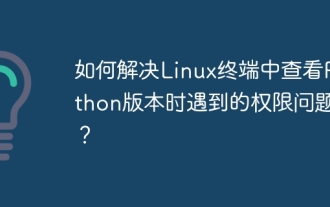
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
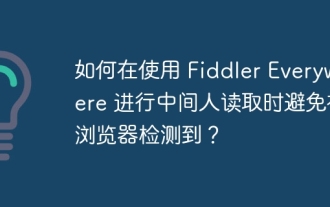
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
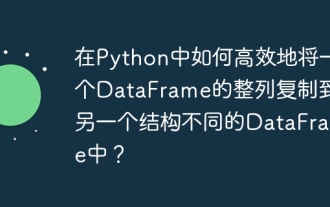
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
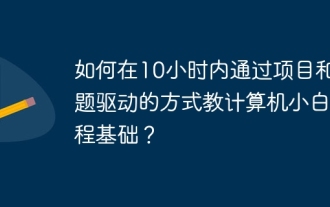
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
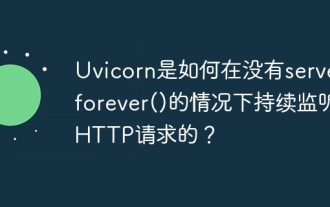
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
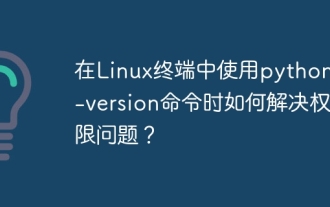
Using python in Linux terminal...
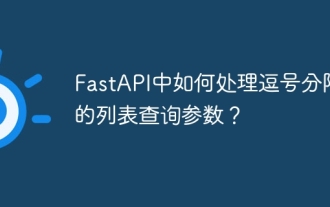
Fastapi ...
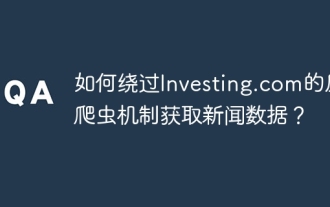
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
