How to safely obtain shared data in Goroutine?
In Go, the shared data of goroutine can be safely obtained through the following methods: 1. Mutex (Mutex): allows one goroutine to have exclusive access to shared data; 2. Channel (Channel): coordinates access to shared data and acts as a goroutine 3. Atomic Operation: read and modify shared variables atomically to ensure data consistency.
#How to safely obtain shared data in goroutine in Go?
In concurrent programming, coordinating access to shared data is crucial. The Go language provides several ways to achieve this, including mutexes, channels, and atomic operations.
Mutex lock
Mutex lock (Mutex) is used to allow one goroutine to access shared data at a time. To create a mutex, use the sync.Mutex
type. The following is an example of how to use a mutex:
package main import ( "fmt" "sync" ) // 共享变量 var counter int func main() { var mu sync.Mutex // 创建 10 个 goroutine,每个 goroutine 增加 counter for i := 0; i < 10; i++ { go func() { mu.Lock() defer mu.Unlock() counter++ fmt.Printf("goroutine %d: %d\n", i, counter) }() } }
Channel
Channels can be used to pass data between goroutines and can also be used to coordinate access to shared data . To create a channel, you can use the make(chan)
function. Here is an example of how to use channels:
package main import ( "fmt" "sync" ) // 共享变量 var counter int func main() { ch := make(chan struct{}) // 创建 10 个 goroutine,每个 goroutine 增加 counter for i := 0; i < 10; i++ { go func() { defer close(ch) for { select { case <-ch: return default: counter++ fmt.Printf("goroutine %d: %d\n", i, counter) } } }() } // 等待所有 goroutine 完成 for i := 0; i < 10; i++ { <-ch } }
Atomic operations
Atomic operations can be used to atomically read and modify the value of a shared variable. The Go language provides the sync/atomic
package to support atomic operations. Here is an example of how to use atomic operations:
package main import ( "fmt" "sync/atomic" ) // 共享变量 var counter int func main() { // 使用 AddInt64 增加 counter for i := 0; i < 10; i++ { go func() { atomic.AddInt64(&counter, 1) fmt.Printf("goroutine %d: %d\n", i, counter) }() } }
Among these methods, which method to choose depends on the specific scenario and the required level of security assurance.
The above is the detailed content of How to safely obtain shared data in Goroutine?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
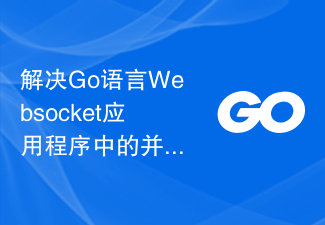
WebSocket is a modern network communication protocol that can achieve high real-time two-way communication. The Go language inherently supports concurrency, so it performs very well in Websocket applications. However, concurrency also brings some problems. In Websocket applications, this is mainly reflected in concurrency security. In this article, we will explain and demonstrate how to solve concurrency security issues in Go Websocket applications. Problem background is in Websocket application
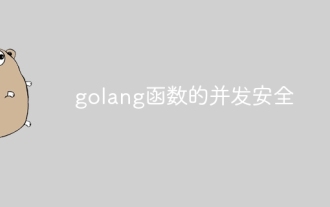
The concurrency safety of Go functions means that the function can still operate correctly when called concurrently, avoiding damage caused by multiple goroutines accessing data at the same time. Concurrency-safe functions can use methods such as locks, channels, or atomic variables. Locks allow goroutines to have exclusive access to critical sections, channels provide a secure communication mechanism, and atomic variables provide concurrent secure access to specific variables. In actual cases, channels are used to implement concurrency safety functions to ensure that multiple goroutines access shared resources in the correct order.
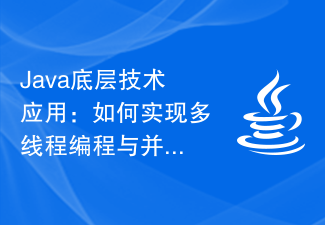
"Java Underlying Technology Application: How to Implement Multi-Threaded Programming and Concurrency Security" In today's software development field, multi-thread programming and concurrency security are very important topics. Especially in Java development, we often need to deal with multi-thread concurrency. However, achieving multi-threaded programming and concurrency safety is not an easy task. This article will introduce the application of Java's underlying technology and explore how to use specific code examples to achieve multi-threaded programming and concurrency safety. First, let’s understand multi-threaded programming in Java
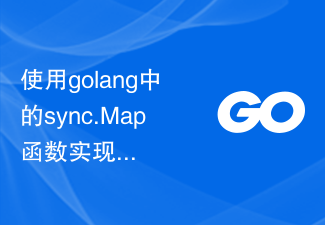
Title: Using the sync.Map function in golang to implement concurrent and safe mapping Introduction: In concurrent programming, multiple goroutines read and write the same data structure at the same time, which will cause data competition and inconsistency problems. In order to solve this problem, the Go language provides the Map type in the sync package, which is a concurrency-safe mapping that can safely perform read and write operations in multiple goroutines. This article will introduce how to use the sync.Map function to implement concurrent and safe mapping, and
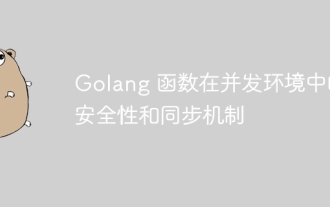
Function safety: goroutine safety: safe to call in concurrent goroutines. Non-goroutine safe: access shared state or depend on a specific goroutine. Synchronization mechanism: Mutex: protects concurrent access to shared resources. RWMutex: Allows concurrent reading and only one write. Cond: Wait for specific conditions to be met. WaitGroup: Wait for a group of goroutines to complete. Practical case: Concurrency counter uses Mutex to protect shared state and ensure correctness under concurrency.
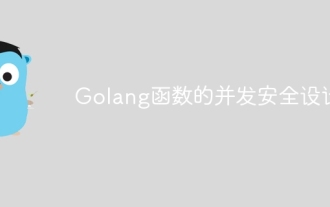
Concurrency safety of Golang functions is crucial. According to the type of shared resources accessed, concurrency-safe functions can be divided into immutable functions and variable functions. Variable functions need to use appropriate synchronization mechanisms, such as mutex locks, read-write locks, and atomic values, to ensure concurrency safety. The practical case demonstrates the use of mutex locks to implement concurrent safe variable functions. Other considerations include avoiding global variables, using pipes to pass data, and testing for concurrency.
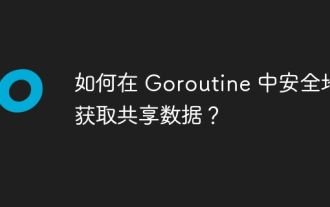
In Go, the shared data of goroutines can be safely obtained through the following methods: 1. Mutex (Mutex): allows one goroutine to have exclusive access to shared data; 2. Channel (Channel): coordinates access to shared data and serves as data between goroutines Transfer mechanism; 3. Atomic Operation: Read and modify shared variables atomically to ensure data consistency.
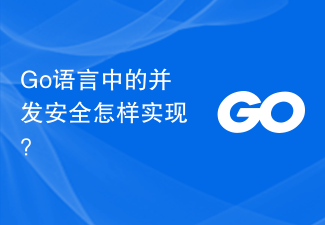
With the continuous development of computer technology, we must shift from single thread to multi-thread for program processing. Compared with the traditional concurrency processing model, the powerful concurrency processing mechanism of Go language has attracted the attention of many developers. The Go language provides a lightweight implementation mechanism that makes authentic concurrent code easier to write. However, it is inevitable that a multi-threaded environment will bring many race conditions (RaceCondition). When multiple threads try to read and write the same shared resource at the same time, due to the uncertainty in the order of execution
