


Detailed explanation of encapsulation of React Native vertical carousel component
This article mainly introduces the encapsulation of the React Native notification message vertical carousel component. It has certain reference value. Interested friends can refer to it. I hope it can help everyone.
The example in this article shares the encapsulation code of the React Native notification message vertical carousel component for your reference. The specific content is as follows
import React, {Component} from 'react' import { Text, View, Animated, Easing, StyleSheet, } from 'react-native' export default class ScrollVertical extends Component { static defaultProps = { enableAnimation: true, }; constructor(props) { super(props) let translateValue= new Animated.ValueXY({x: 0, y: 0}) translateValue.addListener(({x,y})=>{ // Log('value',x,y) }) this.state = { translateValue: translateValue, // 滚屏高度 scrollHeight: this.props.scrollHeight || 32, // 滚屏内容 kb_content: [], // Animated.View 滚动到的 y轴坐标 kb_tempValue: 0, // 最大偏移量 kb_contentOffsetY: 0, // 每一次滚动切换之前延迟的时间 delay: this.props.delay || 500, // 每一次滚动切换的持续时间 duration: this.props.duration || 500, enableAnimation: true, } } render() { return ( <View style={[styles.kbContainer, {height: this.state.scrollHeight}, this.props.kbContainer]}> { this.state.kb_content.length !== 0 ? <Animated.View style={[ {flexDirection: 'column'}, { transform: [ {translateY: this.state.translateValue.y} ] } ]}> {this.state.kb_content.map(this._createKbItem.bind(this))} </Animated.View> : null } </View> ) } componentWillReceiveProps(nextProps) { Log('componentWillReceiveProps', nextProps) this.setState({ enableAnimation: nextProps.enableAnimation?true:false }, () => { this.startAnimation(); } ) } componentDidMount() { Log('componentDidMount') let content = this.props.data || [] if (content.length !== 0) { let h = (content.length + 1) * this.state.scrollHeight this.setState({ kb_content: content.concat(content[0]), kb_contentOffsetY: h }) // 开始动画 // this._startAnimation() this.startAnimation(); } } _createKbItem(kbItem, index) { return ( <View key={index} style={[{justifyContent: 'center', height: this.state.scrollHeight}, this.props.scrollStyle]}> <Text style={[styles.kb_text_c, this.props.textStyle]}>{kbItem.content}</Text> </View> ) } startAnimation = () => { if (this.state.enableAnimation) { if(!this.animation){ this.animation = setTimeout(() => { this.animation=null; this._startAnimation(); }, this.state.delay); } } } componentWillUnmount() { if (this.animation) { clearTimeout(this.animation); } if(this.state.translateValue){ this.state.translateValue.removeAllListeners(); } } _startAnimation = () => { this.state.kb_tempValue -= this.state.scrollHeight; if (this.props.onChange) { let index = Math.abs(this.state.kb_tempValue) / (this.state.scrollHeight); this.props.onChange(index<this.state.kb_content.length-1?index:0); } Animated.sequence([ // Animated.delay(this.state.delay), Animated.timing( this.state.translateValue, { isInteraction: false, toValue: {x: 0, y: this.state.kb_tempValue}, duration: this.state.duration, // 动画持续的时间(单位是毫秒),默认为500 easing: Easing.linear } ), ]) .start(() => { // 无缝切换 // Log('end') if (this.state.kb_tempValue - this.state.scrollHeight === -this.state.kb_contentOffsetY) { // 快速拉回到初始状态 this.state.translateValue.setValue({x: 0, y: 0}); this.state.kb_tempValue = 0; } this.startAnimation(); }) } } const styles = StyleSheet.create({ kbContainer: { // 必须要有一个背景或者一个border,否则本身高度将不起作用 backgroundColor: 'transparent', overflow: 'hidden' }, kb_text_c: { fontSize: 18, color: '#181818', }
Use
import React, {Component} from 'react'; import { StyleSheet, View, TouchableOpacity, Alert, ScrollView, ART, TouchableHighlight, ListView, Dimensions, Text } from 'react-native'; import ScrollVertical from '../../app-widget/scroll-vertical' const dataArray = [ { title: '降价了', }, { title: '全场五折', }, { title: '打到骨折', } ] export default class extends React.Component { render() { let array = [{ content: '' }]; if (dataArray && dataArray.length > 0) { array = []; for (let item of dataArray) { array.push({ content: item.title}); } } return ( <View style={{ padding: Constant.sizeMarginDefault, paddingBottom: 0, backgroundColor: '#FFFFFF' }}> <TouchableOpacity onPress={() => { if (dataArray && dataArray.length > 0) { Log(dataArray[this.index].title) } }} style={{ flexDirection: 'row', backgroundColor: "#FFFFFF", alignItems: 'center', borderRadius: 8, paddingLeft: 5, paddingRight: 5 }}> <Text style={{ fontSize: Constant.scaleFontSize(14) }} fontWeight={'bold'}>公告</Text> <View style={{ marginLeft: 5, marginRight: 8, backgroundColor: '#b01638', borderRadius: 8, width: 22, alignItems: 'center', }}> <Text style={{ color: 'white', fontSize: Constant.fontSizeSmall }}>新</Text> </View> <View style={{ flexDirection: 'row', flex: 1 }}> <ScrollVertical onChange={(index => { this.index = index; })} enableAnimation={true} data={array} delay={2500} duration={1000} scrollHeight={34} scrollStyle={{ alignItems: 'flex-start' }} textStyle={{ color: Constant.colorTxtContent, fontSize: Constant.fontSizeSmall }} /> </View> <View style={{ height: 14, width: 1, backgroundColor: Constant.colorTxtContent }} /> <Text style={{ color: Constant.colorTxtContent, paddingLeft: Constant.sizeMarginDefault, fontSize: Constant.fontSizeSmall }}>查看</Text> </TouchableOpacity> </View> ); } };
Related recommendations:
React Native How to implement the address picker function
How to solve the cross-domain resource loading error in React Native
Detailed explanation of building a development environment for React Native
The above is the detailed content of Detailed explanation of encapsulation of React Native vertical carousel component. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
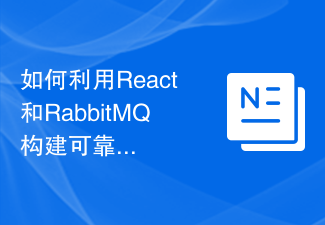
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
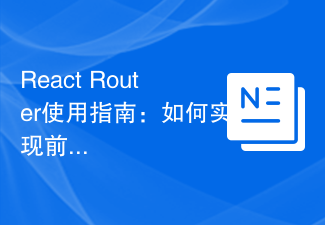
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
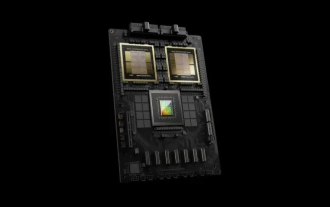
According to news from this site on April 17, TrendForce recently released a report, believing that demand for Nvidia's new Blackwell platform products is bullish, and is expected to drive TSMC's total CoWoS packaging production capacity to increase by more than 150% in 2024. NVIDIA Blackwell's new platform products include B-series GPUs and GB200 accelerator cards integrating NVIDIA's own GraceArm CPU. TrendForce confirms that the supply chain is currently very optimistic about GB200. It is estimated that shipments in 2025 are expected to exceed one million units, accounting for 40-50% of Nvidia's high-end GPUs. Nvidia plans to deliver products such as GB200 and B100 in the second half of the year, but upstream wafer packaging must further adopt more complex products.
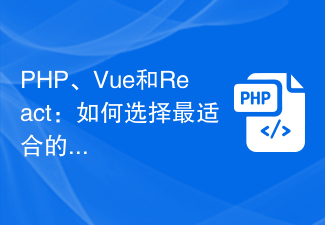
PHP, Vue and React: How to choose the most suitable front-end framework? With the continuous development of Internet technology, front-end frameworks play a vital role in Web development. PHP, Vue and React are three representative front-end frameworks, each with its own unique characteristics and advantages. When choosing which front-end framework to use, developers need to make an informed decision based on project needs, team skills, and personal preferences. This article will compare the characteristics and uses of the three front-end frameworks PHP, Vue and React.
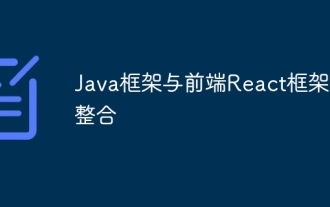
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.
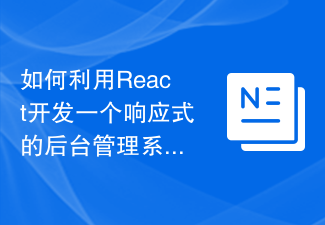
How to use React to develop a responsive backend management system. With the rapid development of the Internet, more and more companies and organizations need an efficient, flexible, and easy-to-manage backend management system to handle daily operations. As one of the most popular JavaScript libraries currently, React provides a concise, efficient and maintainable way to build user interfaces. This article will introduce how to use React to develop a responsive backend management system and give specific code examples. Create a React project first
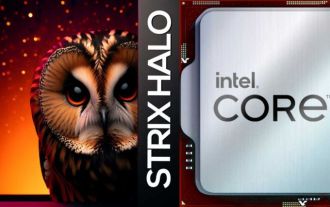
This website reported on July 9 that the AMD Zen5 architecture "Strix" series processors will have two packaging solutions. The smaller StrixPoint will use the FP8 package, while the StrixHalo will use the FP11 package. Source: videocardz source @Olrak29_ The latest revelation is that StrixHalo’s FP11 package size is 37.5mm*45mm (1687 square millimeters), which is the same as the LGA-1700 package size of Intel’s AlderLake and RaptorLake CPUs. AMD’s latest Phoenix APU uses an FP8 packaging solution with a size of 25*40mm, which means that StrixHalo’s F
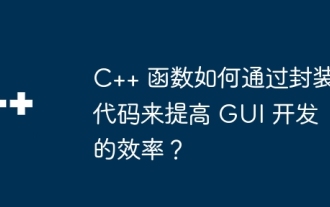
By encapsulating code, C++ functions can improve GUI development efficiency: Code encapsulation: Functions group code into independent units, making the code easier to understand and maintain. Reusability: Functions create common functionality that can be reused across applications, reducing duplication and errors. Concise code: Encapsulated code makes the main logic concise and easy to read and debug.
