


About the PHP framework Laravel plug-in Pagination method to implement custom paging
This article mainly introduces the relevant information about the PHP framework Laravel5.1 plug-in Pagination to implement custom paging. Friends who need it can refer to
Laravel’s paging is very convenient, and it is actually quite easy to expand. Let's take an example and extend the paginate() and simplePaginate() methods to implement our custom paging style, such as displaying "previous page" and "next page" instead of "《" and "》". Of course, once you master the expansion method, you can unscrupulously expand a paging you want, such as jumping to a certain page, displaying the total number of records in the paging, the currently displayed record range, and so on. . .
5.1 and 5.2 should be the same method. I am using the 5.2 version here. The documentation tells us that Paginator corresponds to the query builder and Eloquent's simplePaginate method, while LengthAwarePaginator is equivalent to the paginate method. Then let’s take a look at the source code to see how this paginate implements render(),
Illuminate/Pagination/LengthAwarePaginator.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
|
render() is an instance of Presenter, and the instantiated render method is called to realize paging display. If not, call render() in BootstrapThreePresenter to see what BootstrapThreePresenter does
Illuminate/Pagination/BootstrapThreePresenter.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
|
Here you can see that BootstrapThreePresenter implements the interface of PresenterContract. render() is the real implementation of paging display. The first parameter PaginatorContract in the construction method is actually a Paginator. Let’s continue to look at PresenterContract. That is, what methods are defined in the Presenter interface and need to be implemented
illuminate/contracts/Pagination/Presenter.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
|
The render and hasPages methods are defined and need to be implemented
Okay, now we are very clear. If we want to customize the paging display, we must write our own Presenter to implement the interface. render() and hasPages() in it will do the trick.
First of all, let's simply implement a paginate() to display "previous page" and "next page", with an example of pagination numbers in the middle.
Create a new file as follows (personal habit)
app/Foundations/Pagination/CustomerPresenter.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 |
|
It’s that simple, mainly the render() method. If you need to modify the paging style or add paging jumps in the project, just rewrite the html elements in each display method. It’s very easy. Flexible, this also needs to be fixed in the blade template. For example, our Paginator is called $users. The default paging display is like this:
{!! $users->render() !!}
Modify to our customized paging display:
{!! with(new \App\Foundations\Pagination\CustomerPresenter($categories))->render() !!}
Okay, now you should be able to see on the page that the paging links contain the style of "previous page" and "next page" plus numbers.
So what if you extend simplePaginate? It's actually very simple. You just need to inherit the CustomerPresenter just now and implement hasPages and render. As for why, you can just look at it the way I viewed the source code above. For example, we change it to "Previous article" "and"Next article"
New App\Foundations\Pagination\CustomerSimplePresenter.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
|
Pagination display:
{!! with(new \App\Foundations\Pagination\CustomerSimplePresenter($categories))->render() !!}
The method is this method. Just modify it and rewrite the corresponding method of displaying html elements according to your own needs.
The above is the entire content of this article. I hope it will be helpful to everyone's study. For more related content, please pay attention to the PHP Chinese website!
Related recommendations:
Pages and Form Validation in Laravel 4
How to solve the problem of Laravel's throttle middleware failure
The above is the detailed content of About the PHP framework Laravel plug-in Pagination method to implement custom paging. For more information, please follow other related articles on the PHP Chinese website!
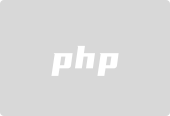
Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!
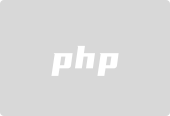
Hot Article
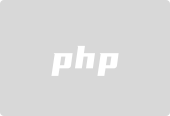
Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)
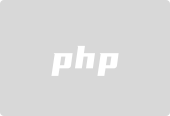
Hot Topics










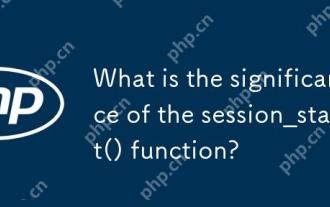
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.
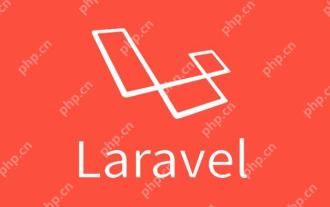
The main differences between Laravel and Yii are design concepts, functional characteristics and usage scenarios. 1.Laravel focuses on the simplicity and pleasure of development, and provides rich functions such as EloquentORM and Artisan tools, suitable for rapid development and beginners. 2.Yii emphasizes performance and efficiency, is suitable for high-load applications, and provides efficient ActiveRecord and cache systems, but has a steep learning curve.
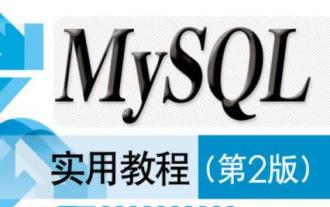
MySQL functions can be used for data processing and calculation. 1. Basic usage includes string processing, date calculation and mathematical operations. 2. Advanced usage involves combining multiple functions to implement complex operations. 3. Performance optimization requires avoiding the use of functions in the WHERE clause and using GROUPBY and temporary tables.
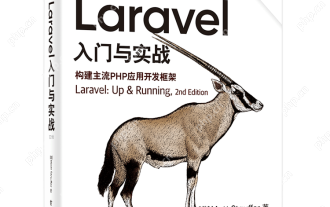
The essential Laravel extension packages for 2024 include: 1. LaravelDebugbar, used to monitor and debug code; 2. LaravelTelescope, providing detailed application monitoring; 3. LaravelHorizon, managing Redis queue tasks. These expansion packs can improve development efficiency and application performance.

Composer is a dependency management tool for PHP, and manages project dependencies through composer.json file. 1) parse composer.json to obtain dependency information; 2) parse dependencies to form a dependency tree; 3) download and install dependencies from Packagist to the vendor directory; 4) generate composer.lock file to lock the dependency version to ensure team consistency and project maintainability.
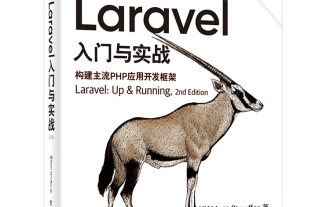
Integrating Sentry and Bugsnag in Laravel can improve application stability and performance. 1. Add SentrySDK in composer.json. 2. Add Sentry service provider in config/app.php. 3. Configure SentryDSN in the .env file. 4. Add Sentry error report in App\Exceptions\Handler.php. 5. Use Sentry to catch and report exceptions and add additional context information. 6. Add Bugsnag error report in App\Exceptions\Handler.php. 7. Use Bugsnag monitoring
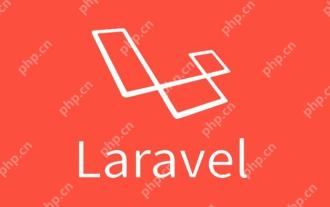
Building a live chat application in Laravel requires using WebSocket and Pusher. The specific steps include: 1) Configure Pusher information in the .env file; 2) Set the broadcasting driver in the broadcasting.php file to Pusher; 3) Subscribe to the Pusher channel and listen to events using LaravelEcho; 4) Send messages through Pusher API; 5) Implement private channel and user authentication; 6) Perform performance optimization and debugging.
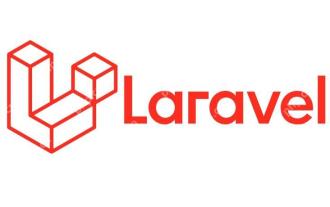
EagerLoading can solve N 1 query problems in Laravel. 1) Use the with method to preload relevant model data, such as User::with('posts')->get(). 2) For nested relationships, use with('posts.comments'). 3) Avoid overuse, selective loading, and use the load method as needed. Through these methods, the number of queries can be significantly reduced and the application performance can be improved.
