SpringBoot scheduling tasks and common task expressions
This article mainly introduces SpringBoot scheduling tasks and common task expressions. 1. First, you need to annotate *applicatin.java with @EnableScheduling to detect whether there are scheduled tasks. 2. The @Scheduled annotation is used to mark this method as a scheduled task method. Spring will automatically scan this annotation and start the scheduling task.
package com.david.translate.quartz; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.scheduling.annotation.Scheduled; import org.springframework.stereotype.Component; import com.david.translate.service.SysUserService; @Component public class TimeQuartz { @Autowired private SysUserService userService; /** * 一分钟执行一次 */ @Scheduled(cron="0 0/1 * * * ?") public void executeFileDownLoadTask() { System.out.println(">>>>>>>>>>>>>>>>>>>任务执行 "+userService.findAll().size()); } }
The time configuration of scheduling tasks uses cron expressions. I recommend a website that generates expressions online. If you don’t know how to write or are unwilling to write it yourself, , can be generated directly using this website:
http://cron.qqe2.com/
The screenshot is as follows:
Some commonly used task expression examples:
0 * * * * ? Trigger once every 1 minute
0 0 * * * ? Trigger once every 1 hour every day
0 0 10 * * ? Triggered once every day at 10 o'clock
0 * 14 * * ? Triggered every 1 minute from 2 pm to 2:59 pm every day
0 30 9 1 * ? At 9:30 am on the 1st of every month
0 15 10 15 * ? Triggered at 10:15 am on the 15th of every month
/5 * * * ? Executed every 5 seconds
0 /1 * * ? Executed every 1 minute Once
0 0 5-15 * * ? Trigger on the hour from 5 to 15 o'clock every day
0 0/3 * * * ? Trigger once every three minutes
0 0-5 14 * * ? Every afternoon Trigger every 1 minute from 2pm to 2:05pm
0 0/5 14 * * ? Trigger every 5 minutes from 2pm to 2:55pm every day
0 0/5 14,18 * * ? Trigger every 5 minutes between 2pm and 2:55pm and every 5 minutes between 6pm and 6:55pm
0 0/30 9-17 * * ? Every half hour during 9 to 5 working hours
0 0 10,14,16 * * ? Every day at 10 am, 2 pm, 4 pm
0 0 12 ? * WED means every Wednesday at 12 noon
0 0 17 ? * TUES, THUR, SAT every Tuesday, Thursday, and Saturday at 5 pm
0 10,44 14 ? 3 WED Triggered every Wednesday in March at 2:10 and 2:44 pm
0 15 10 ? * MON -FRI triggers at 10:15 am from Monday to Friday
0 0 23 L * ? Execute once at 23:00 on the last day of each month
0 15 10 L * ? 10:00 am on the last day of each month 15 Trigger
0 15 10 ? * 6L Triggered at 10:15 am on the last Friday of each month
0 15 10 * * ? 2005 Triggered at 10:15 am every day in 2005
0 15 10 ? * 6L 2002-2005 Triggered at 10:15 am on the last Friday of each month from 2002 to 2005
0 15 10 ? * 6#3 Triggered at 10:15 am on the third Friday of each month
The above content is SpringBoot scheduling tasks and common task expressions. I hope it can help everyone.
Related recommendations:
Detailed explanation of the causes of Session timeout problem in SpringBoot
Introductory graphic tutorial about SpringBoot in Java
In-depth analysis of how springboot configures multiple redis connections
The above is the detailed content of SpringBoot scheduling tasks and common task expressions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










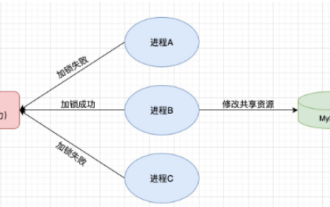
1. Redis implements distributed lock principle and why distributed locks are needed. Before talking about distributed locks, it is necessary to explain why distributed locks are needed. The opposite of distributed locks is stand-alone locks. When we write multi-threaded programs, we avoid data problems caused by operating a shared variable at the same time. We usually use a lock to mutually exclude the shared variables to ensure the correctness of the shared variables. Its scope of use is in the same process. If there are multiple processes that need to operate a shared resource at the same time, how can they be mutually exclusive? Today's business applications are usually microservice architecture, which also means that one application will deploy multiple processes. If multiple processes need to modify the same row of records in MySQL, in order to avoid dirty data caused by out-of-order operations, distribution needs to be introduced at this time. The style is locked. Want to achieve points
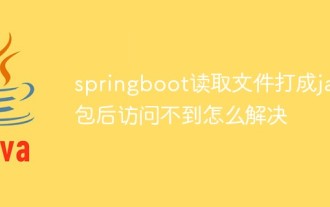
Springboot reads the file, but cannot access the latest development after packaging it into a jar package. There is a situation where springboot cannot read the file after packaging it into a jar package. The reason is that after packaging, the virtual path of the file is invalid and can only be accessed through the stream. Read. The file is under resources publicvoidtest(){Listnames=newArrayList();InputStreamReaderread=null;try{ClassPathResourceresource=newClassPathResource("name.txt");Input
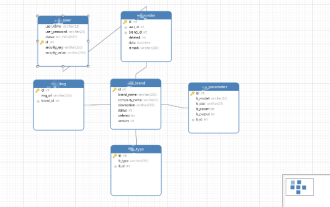
When Springboot+Mybatis-plus does not use SQL statements to perform multi-table adding operations, the problems I encountered are decomposed by simulating thinking in the test environment: Create a BrandDTO object with parameters to simulate passing parameters to the background. We all know that it is extremely difficult to perform multi-table operations in Mybatis-plus. If you do not use tools such as Mybatis-plus-join, you can only configure the corresponding Mapper.xml file and configure The smelly and long ResultMap, and then write the corresponding sql statement. Although this method seems cumbersome, it is highly flexible and allows us to
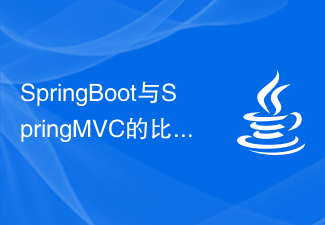
SpringBoot and SpringMVC are both commonly used frameworks in Java development, but there are some obvious differences between them. This article will explore the features and uses of these two frameworks and compare their differences. First, let's learn about SpringBoot. SpringBoot was developed by the Pivotal team to simplify the creation and deployment of applications based on the Spring framework. It provides a fast, lightweight way to build stand-alone, executable
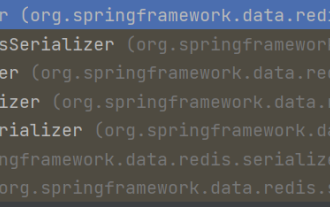
1. Customize RedisTemplate1.1, RedisAPI default serialization mechanism. The API-based Redis cache implementation uses the RedisTemplate template for data caching operations. Here, open the RedisTemplate class and view the source code information of the class. publicclassRedisTemplateextendsRedisAccessorimplementsRedisOperations, BeanClassLoaderAware{//Declare key, Various serialization methods of value, the initial value is empty @NullableprivateRedisSe
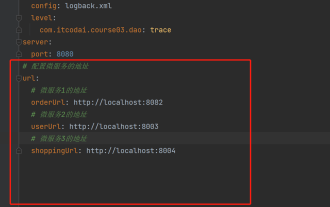
In projects, some configuration information is often needed. This information may have different configurations in the test environment and the production environment, and may need to be modified later based on actual business conditions. We cannot hard-code these configurations in the code. It is best to write them in the configuration file. For example, you can write this information in the application.yml file. So, how to get or use this address in the code? There are 2 methods. Method 1: We can get the value corresponding to the key in the configuration file (application.yml) through the ${key} annotated with @Value. This method is suitable for situations where there are relatively few microservices. Method 2: In actual projects, When business is complicated, logic
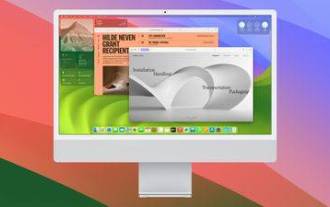
By default, macOSSonoma hides all active windows when you click on your desktop wallpaper. This is convenient if you tend to have a bunch of files on your desktop that you need to access. However, if you find this behavior maddening, there is a way to turn it off. Apple's latest macOS Sonoma Mac operating system has a new option called "Click the wallpaper to show the desktop." Enabled by default, this option can be particularly useful if you tend to have multiple windows open and want to access files or folders on your desktop without having to minimize or move the windows. When you enable the feature and click on the desktop wallpaper, all open windows are temporarily swept aside, allowing direct access to the desktop. Once done, you can again

This article will write a detailed example to talk about the actual development of dubbo+nacos+Spring Boot. This article will not cover too much theoretical knowledge, but will write the simplest example to illustrate how dubbo can be integrated with nacos to quickly build a development environment.
