


Essential JS basic knowledge—Systematic compilation of common interview questions
1.JS built-in types
are divided into basic data types and Object.
Basic data types include: null, undefined, string, boolean, number, symbol .
console.log(typeof null);//object console.log(typeof []);//object console.log(typeof {});//object
What should you do if you want to distinguish between null, array, and object?
console.log(Object.prototype.toString.call(null));//[object Null] console.log(Object.prototype.toString.call([]));//[object Array] console.log(Object.prototype.toString.call({}));//[object Object]
My simple understanding: toString (data); function: convert data into a string.
Recommended related articles:The most complete collection of js interview questions in 2020 (latest)
2. Type conversion
Type conversion: divided into explicit type conversion and implicit type conversion.
1.Number (data)
如果数据内容式纯粹的数字,才可以转化为数字,否则式NaN。
var str2="12px";//NaN var str2="1.2";//1.2 var str2="1.2.3";//NaN var str2=null;//0 console.log(Number(str2));
2.NaN
NaN的数据类型书Number。注意:NaN和任何东西都不相等,包括自己。
3.isNaN (data)
会先把数据用Number转化,转化完了之后在判断是不是NaN,如果是NaN则返回为true。否则返回为fasle。
console.log(isNaN(1));//false console.log(isNaN("123"));//false console.log(isNaN("abc"));//true
4.parseInt(data) and parseFloat(data)
parseInt(数据):把数据变成整数,舍去小数点,取整数。 parseFloat(数据):把数据转化为数字,可以是小数。 注意:这两个方法会从左往右开始,除去空格,找到第一位非0数字,开始进行转换,直到转换到不是数字的那位为止,或者,转换出合适的值为止。
console.log( parseInt( "1" ) );//1 console.log( parseInt( "1.9" ) );//1 console.log( parseInt( "20px" ) );//20 console.log( parseInt( " 25px" ) );//25 console.log( parseInt( " 0.0026px" ) );//0 console.log( parseFloat( " 0.0026px" ) );//0.0026
5.Stirng() and Boolean() can also be displayed Type conversion is not mentioned here
条件判断中,除了null,undefined,'',NaN,false,0都转化为false,其余都转化为true。
6. Implicit type conversion
只有当加法运算时,其中一方是字符串类型,就会把另一个也转为字符串类型。其他运算只要其中一方是数字,那么另一方就转为数字。并且加法运算会触发三种类型转换:将值转换为原始值,转换为数字,转换为字符串。
<script> console.log( "abc"-1 );//NaN console.log( NaN-1 );//NaN console.log( NaN+1 );//NaN console.log( NaN+"1" );//NaN1 //------------------------------------------ console.log( "3"-1 );//转成数字2 console.log( "345" - 0 );//转成数字345 console.log( "345" * 1 );//转成数字345 console.log( "345" / 1 );//转成数字345 console.log( "345px" - 0 );//NaN //------------------------------------------ console.log( 123 + "" );//转成字符串 "123" //------------------------------------------ console.log( !!234 );//转成boolean类型 true console.log( !!0 );//转成boolean类型 false console.log( !!{} );//转成boolean类型 true console.log( !!null );//转成boolean类型 false
3. Operators
== and = ==
==: 1.如果类型相同的话,比较内容 类型不相同,类型转化在比较 1)一个是undefined,一个是null,则相等。 2)数字和字符串的的话,将字符串转化为数字再进行比较。 ===: 1.类型相同比教内容,类型不同则返回fasle。
4.Prototype, prototype chain, new
1.new
1.新生成了一个对象 2.链接到原型 3.绑定this 4.返回新对象
2.prototype prototype
prototype 原型 当一个函数被申明的时候,该函数下默认有一个属性:prototype,该属性的值是一个对象 当我们去调用一个对象的属性或者方法的时候,如果该对象自身没有该属性或方法, 则会调用到该对象 的 构造函数的prototype的属性或方法 把通过构造函数构造出来的对象,共有的方法或者属性,放在prototype身上 __proto__ 当一个对象被创建的时候, 该对象会自动被添加上一个属性:__proto__, 他的值也是一个对象,并且该属性 就是 当前这个对象的构造函数的prototype 对象.__proto__ === 构造函数.prototype
3.Prototype chain
Function.prototype.a = "a"; Object.prototype.b = "b"; function Person(){} console.log(Person); //function Person() let p = new Person(); console.log(p); //Person {} 对象 console.log(p.a); //undefined console.log(p.b); //b
Analysis:
会一直通过__proto__向上查找,最后当查找到Object.prototype时找到,最后打印出b,向上查找过程中,得到的是Object.prototype,而不是Function.prototype,找不到a属性,所以结果为undefined,这就是原型链,通过__proto__向上进行查找,最终到null结束
//Function function Function(){} console.log(Function); //Function() console.log(Function.prototype.constructor); //Function() console.log(Function.prototype.__proto__); //Object.prototype console.log(Function.prototype.__proto__.__proto__); //NULL console.log(Function.prototype.__proto__.constructor); //Object() console.log(Function.prototype.__proto__ === Object.prototype); //true
Summary:
1.查找属性,如果本身没有,则会去__proto__中查找,也就是构造函数的显式原型中查找,如果构造函数中也没有该属性,因为构造函数也是对象,也有__proto__,那么会去它的显式原型中查找,一直到null,如果没有则返回undefined 2.p.__proto__.constructor == function Person(){} 3.p.___proto__.__proto__== Object.prototype 4.p.___proto__.__proto__.__proto__== Object.prototype.__proto__ == null 5.通过__proto__形成原型链而非protrotype
5.instanceof
检测对象原型是否在要检测对象的原型链上,返回值为true或者false 使用:object instanceof constructor
function Fn () {} var f = new Fn(); console.log( f instanceof Fn );//true console.log( f instanceof Object );//true
// //str是字面量生成的,是由JS内部的String构造函数new出来的。 // //但是str会立刻"压扁"自己,让自己不是对象。 // //所以str都不是对象了,自然instanceof String 的得到的值为fasle // //但str.indexOf(),str还是可以调用indexOf()方法的原因是,当它调用方法的时候,会重新将自己包装成对象。 // //使用结束后会重新"压扁"自己,让自己不是对象。 var str = "123"; console.log( str instanceof Array );//false console.log( str instanceof String);//false console.log( str instanceof Object);//false // var obj = {}; console.log( obj instanceof Array );//false console.log( obj instanceof Object);//true
6.hasOwnProperty
作用 用来判断某个对象是否含有 指定的 自身属性 语法 boolean object.hasOwnProperty(prop) 参数 object 要检测的对象 prop 要检测的属性名称。 注意:不会沿着原型链查找属性,只查找自身属性
function Fn(name,gender){ this.name = name; this.gender = gender; } Fn.prototype.kind = "human"; Fn.prototype.say = function(){ console.log(1); }; var f = new Fn("kimoo","男"); console.log( f.hasOwnProperty("name") ); //true console.log( f.hasOwnProperty("kind") ); //false console.log( f.hasOwnProperty("say") ); //false
8.call,bind,apply
## The functions of #call, bind, and apply are to modify this pointer.
Difference:
1.call:函数会立即执行,括号中的内容 第一个参数 就是 函数执行时候 ,内部的this指向。后面的参数就是函数内部的实参。
function foo (a,b) { console.log( this ); console.log( a,b ); } foo.call( document,2,3 );//运行结果:#document 2,3
2.apply:函数会立即执行,括号中的内容 第一个参数 就是 函数执行时候 ,内部的this指向。不过第二个参数接受数组。
function foo (a,b) { console.log( this ); console.log( a,b ); } foo.apply( document,[2,3] ); // 和call 相似 直接调用 , 不过第二个参数接受数组。运行结果:#document 2,3
3.bind:函数 不会 立刻执行,返回的是 修改了 this指向的新函数.第二个参数是参数列表。
function foo (a,b) { console.log( this ); console.log( a,b ); } var fn = foo.bind( document,2,3);// 函数 不会 立刻执行,返回的是 修改了 this指向的新函数 fn(); //运行结果: //#document //2 3
<script>
function Person(){
this.arr = [1,2,3];
this.age = 10;
}
Person.prototype.say = function(){
console.log( "我会说话" );
};
// 父类构造函数中的方法,使用借用构造函数的方式继承
function Coder(name,money) {
Person.call(this);
this.name = name;
this.money = money;
}
// 父类构造函数原型上的方法,使用原型链继承
Coder.prototype = new Person();
Coder.prototype.constructor = Coder; // 重新修改 constructor
Coder.prototype.say = function(){
console.log( "我是程序员,我叫" + this.name +",我一个月:"+ this.money );
}
var p = new Person();
console.log( p.age );
console.log( p.arr );
console.log( p.kind );
p.say();
var c1 = new Coder("a",10000);
console.log( c1.age );
c1.say();
c1.arr.push(4);
console.log( c1.arr );
console.log( c1.name );
console.log( c1.money );
var c2 = new Coder("b",30000);
c2.say();
console.log( c2.age );
console.log( c2.arr );
console.log( c2.name );
console.log( c2.money );
console.log(Coder.prototype.constructor);
</script>
Copy after login
10.Copy inheritance<script> function Person(){ this.arr = [1,2,3]; this.age = 10; } Person.prototype.say = function(){ console.log( "我会说话" ); }; // 父类构造函数中的方法,使用借用构造函数的方式继承 function Coder(name,money) { Person.call(this); this.name = name; this.money = money; } // 父类构造函数原型上的方法,使用原型链继承 Coder.prototype = new Person(); Coder.prototype.constructor = Coder; // 重新修改 constructor Coder.prototype.say = function(){ console.log( "我是程序员,我叫" + this.name +",我一个月:"+ this.money ); } var p = new Person(); console.log( p.age ); console.log( p.arr ); console.log( p.kind ); p.say(); var c1 = new Coder("a",10000); console.log( c1.age ); c1.say(); c1.arr.push(4); console.log( c1.arr ); console.log( c1.name ); console.log( c1.money ); var c2 = new Coder("b",30000); c2.say(); console.log( c2.age ); console.log( c2.arr ); console.log( c2.name ); console.log( c2.money ); console.log(Coder.prototype.constructor); </script>
<script>
function Person(){
this.arr = [1,2,3];
this.age = 10;
}
Person.prototype.say = function(){
console.log( "我会说话" );
}
function Coder(){
Person.call(this);
}
Coder.prototype = cloneFn( Person.prototype );
Coder.prototype.constructor = Coder;
Coder.prototype.say = function(){
console.log( "我是程序员" );
}
var p = new Person();
console.log( p.age );
console.log( p.arr );
p.say();
console.log( "----------------" );
var c1 = new Coder();
console.log( c1.age );
c1.say();
c1.arr.push(4);
console.log( c1.arr );
console.log( "----------------" );
var c2 = new Coder();
console.log( c2.age );
console.log( c2.arr );
c2.say();
//------------------------------------------
function cloneFn( sourse ){
var o = Object.prototype.toString.call(sourse).toLowerCase().indexOf("array")!==-1 ? [] : {};
for( var attr in sourse ){
if( (typeof sourse[attr] === "object") && sourse[attr] !== null ){
o[attr] = cloneFn( sourse[attr] ) ;
}else{
o[attr] = sourse[attr];
}
}
return o;
}
</script>
Copy after login
11. class inheritance<script> function Person(){ this.arr = [1,2,3]; this.age = 10; } Person.prototype.say = function(){ console.log( "我会说话" ); } function Coder(){ Person.call(this); } Coder.prototype = cloneFn( Person.prototype ); Coder.prototype.constructor = Coder; Coder.prototype.say = function(){ console.log( "我是程序员" ); } var p = new Person(); console.log( p.age ); console.log( p.arr ); p.say(); console.log( "----------------" ); var c1 = new Coder(); console.log( c1.age ); c1.say(); c1.arr.push(4); console.log( c1.arr ); console.log( "----------------" ); var c2 = new Coder(); console.log( c2.age ); console.log( c2.arr ); c2.say(); //------------------------------------------ function cloneFn( sourse ){ var o = Object.prototype.toString.call(sourse).toLowerCase().indexOf("array")!==-1 ? [] : {}; for( var attr in sourse ){ if( (typeof sourse[attr] === "object") && sourse[attr] !== null ){ o[attr] = cloneFn( sourse[attr] ) ; }else{ o[attr] = sourse[attr]; } } return o; } </script>
<script>
class Person {
constructor(name){
this.name = name;
}
say(){
console.log( '我的名字叫 ${this.name}');
}
}
class Coder extends Person{
// constructor( name,money ){
// super(name); // 相当于 调用 父类的 构造函数,修改内部的this,
//// 一定要先调用 super 再使用 this
// this.money = money;
// }
say(){
console.log( `我的名字叫 ${this.name}` );
}
eat(){
console.log( "我会吃" );
}
}
var p = new Person("kimoo");
// console.log( p );
// p.say();
// p.eat(); // 报错: p.eat is not a function
var c = new Coder("zm");
console.log( c );
c.say();
c.eat();
</script>
Copy after login
12. Function scope, execution context, variable promotion, closure<script> class Person { constructor(name){ this.name = name; } say(){ console.log( '我的名字叫 ${this.name}'); } } class Coder extends Person{ // constructor( name,money ){ // super(name); // 相当于 调用 父类的 构造函数,修改内部的this, //// 一定要先调用 super 再使用 this // this.money = money; // } say(){ console.log( `我的名字叫 ${this.name}` ); } eat(){ console.log( "我会吃" ); } } var p = new Person("kimoo"); // console.log( p ); // p.say(); // p.eat(); // 报错: p.eat is not a function var c = new Coder("zm"); console.log( c ); c.say(); c.eat(); </script>
1. Scope
JS作用域分为全局作用域和函数作用域,函数作用域可以访问全局作用域中的变量,对象,函数等。但是函数作用域外部访问不到函数内部的变量,对象,函数。 但在ES6中新增了块级作用域。let,const在块中声明的变量,函数等,外部都访问不到。
{ var a=1; let b=2; console.log(b);//2 { console.log(b);//2 } } console.log(a);//1 console.log(b);//报错,b is not defined
2 .Execution contextHere is a very good article about js execution context, you can click the link to view
Summary:
1. 调用函数是会为其创建执行上下文,并压入执行环境栈的栈顶,执行完毕弹出,执行上下文被销毁,随之VO也被销毁 2. EC创建阶段分创建阶段和代码执行阶段 3. 创建阶段初始变量值为undefined,执行阶段才为变量赋值 4. 函数申明先于变量申明
3 .Variable promotion (domain resolution)
Key:During variable promotionThe function priority is higher than the variable priority
function foo() { console.log(f1); //f1() {} console.log(f2); //undefined var f1 = 'hosting'; var f2 = function() {} function f1() {} } foo();
4. Closure
Closure: Function A returns a function B, and function B uses the variables of function A, function B is called closure.
for ( var i=1; i<=5; i++) { setTimeout( function timer() { console.log( i ); }, i*1000 ); }
for (var i = 1; i <= 5; i++) { (function(j) { setTimeout(function timer() { console.log(j); }, j * 1000); })(i); }
for ( var i=1; i<=5; i++) { setTimeout( function timer(j) { console.log( j ); }, i*1000, i); }
for ( let i=1; i<=5; i++) { setTimeout( function timer() { console.log( i ); }, i*1000 ); }
1.Shallow copy
First, you can solve this problem through Object.assign.let a = { age: 1 } let b = Object.assign({}, a) a.age = 2 console.log(b.age) // 1
let a = { age: 1 } let b = {...a} a.age = 2 console.log(b.age) // 1
2. Deep copy
function cloneFn( sourse ){ var o = Object.prototype.toString.call(sourse).toLowerCase().indexOf("array")!==-1 ? [] : {}; for( var attr in sourse ){ if( (typeof sourse[attr] === "object") && sourse[attr] !== null ){ o[attr] = cloneFn( sourse[attr] ) ; }else{ o[attr] = sourse[attr]; } } return o; }
8 basic knowledge of JS that must be paid attention to
JS basic knowledge compilation
The above is the detailed content of Essential JS basic knowledge—Systematic compilation of common interview questions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
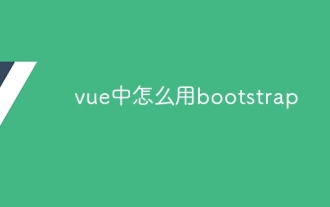
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.

HTML defines the web structure, CSS is responsible for style and layout, and JavaScript gives dynamic interaction. The three perform their duties in web development and jointly build a colorful website.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

WebdevelopmentreliesonHTML,CSS,andJavaScript:1)HTMLstructurescontent,2)CSSstylesit,and3)JavaScriptaddsinteractivity,formingthebasisofmodernwebexperiences.
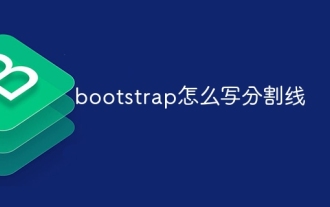
There are two ways to create a Bootstrap split line: using the tag, which creates a horizontal split line. Use the CSS border property to create custom style split lines.
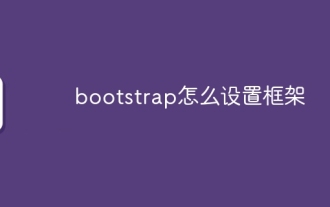
To set up the Bootstrap framework, you need to follow these steps: 1. Reference the Bootstrap file via CDN; 2. Download and host the file on your own server; 3. Include the Bootstrap file in HTML; 4. Compile Sass/Less as needed; 5. Import a custom file (optional). Once setup is complete, you can use Bootstrap's grid systems, components, and styles to create responsive websites and applications.
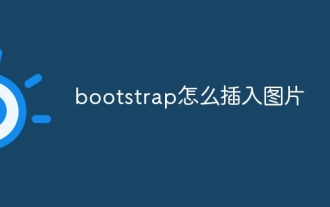
There are several ways to insert images in Bootstrap: insert images directly, using the HTML img tag. With the Bootstrap image component, you can provide responsive images and more styles. Set the image size, use the img-fluid class to make the image adaptable. Set the border, using the img-bordered class. Set the rounded corners and use the img-rounded class. Set the shadow, use the shadow class. Resize and position the image, using CSS style. Using the background image, use the background-image CSS property.
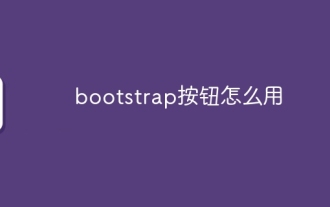
How to use the Bootstrap button? Introduce Bootstrap CSS to create button elements and add Bootstrap button class to add button text
