jQuery/Vue mouse move in and out effect
This article mainly introduces the jQuery/Vue mouse movement in and out effect, which has certain reference value. Now I share it with you. Friends in need can refer to it
Implementation ideas
1. Position the direction of the element according to the position of the mouse
2. Dynamically set the mask layer style according to the direction
3. Set animation to move the mask layer
jQuery version
jQuery plug-in can be extended through the $.fn.extend method.
html
<div class="container"> <div class="content" style="background:aqua"> <div class="shade"> <p>mouse hover</p> </div> </div> <div class="content" style="background:bisque"> <div class="shade"> <p>mouse hover</p> </div> </div> <div class="content" style="background:cadetblue"> <div class="shade"> <p>mouse hover</p> </div> </div> <div class="content" style="background:chocolate"> <div class="shade"> <p>mouse hover</p> </div> </div> <div class="content" style="background:cornflowerblue"> <div class="shade"> <p>mouse hover</p> </div> </div> <div class="content" style="background:darkkhaki"> <div class="shade"> <p>mouse hover</p> </div> </div> </div>
css
.container { width: 600px; margin: auto; margin-top: 100px; } .content { float: left; position: relative; height: 150px; width: 150px; margin: 20px; overflow: hidden; background: #ccc; } .content .shade { position: absolute; top: 0; display: none; width: 100%; height: 100%; line-height: 100px; color: #fff; background: rgba(0, 0, 0, 0.7); }
js
<script> (function ($) { $.fn.extend({ "mouseMove": function (child) { $(this).hover(function (e) { $this = $(this); var ele = $this.find(child); var clientX = e.clientX; var clientY = e.clientY; var top = parseInt($this.offset().top); var bottom = parseInt(top + $this.height()); var left = parseInt($this.offset().left); var right = parseInt(left + $this.width()); var absTop = Math.abs(clientY - top); var absBottom = Math.abs(clientY - bottom); var absLeft = Math.abs(clientX - left); var absRight = Math.abs(clientX - right); var min = Math.min(absTop, absBottom, absLeft, absRight); var eventType = e.type; switch (min) { case absTop: animate("top", eventType, ele); break; case absBottom: animate("bottom", eventType, ele); break; case absLeft: animate("left", eventType, ele); break; case absRight: animate("right", eventType, ele) } }) } }); function animate(direction, type, ele) { var timer = 200; var $target = $(ele); if (type == "mouseenter") { $target.stop(true, true); } if (direction == "top") { if (type == "mouseenter") { $target.css({ display: "block", top: "-100%", left: "0" }).animate({ top: 0, left: 0 }, timer) } else { $target.animate({ display: "block", top: "-100%", left: "0" }, timer) } } else if (direction == "left") { if (type == "mouseenter") { $target.css({ display: "block", top: "0", left: "-100%" }).animate({ left: 0, top: 0 }, timer) } else { $target.animate({ display: "block", left: "-100%" }, timer) } } else if (direction == "bottom") { if (type == "mouseenter") { $target.css({ display: "block", top: "100%", left: "0" }).animate({ top: 0, left: 0 }, timer) } else { $target.animate({ display: "block", top: "100%", left: "0" }, timer) } } else if (direction == "right") { if (type == "mouseenter") { $target.css({ display: "block", top: 0, left: "100%" }).animate({ left: "0%", top: 0 }, timer) } else { $target.animate({ display: "block", left: "100%" }, timer) } } } $('.content').mouseMove('.shade') })(window.jQuery); </script>
Vue version
General Vue component implementation determines the position of the mouse within an element. Use slots to display the contents of the mask layer.
html
<div id="app"> <mouse-hover style="background:aqua"> <div slot>mouse hover</div> </mouse-hover> <mouse-hover style="background:bisque"> <div slot>mouse hover</div> </mouse-hover> <mouse-hover style="background:cadetblue"> <div slot>mouse hover</div> </mouse-hover> <mouse-hover style="background:chocolate"> <div slot>mouse hover</div> </mouse-hover> <mouse-hover style="background:cornflowerblue"> <div slot>mouse hover</div> </mouse-hover> <mouse-hover style="background:darkkhaki"> <div slot>mouse hover</div> </mouse-hover> </div>
css
<style> html, body { text-align: center; color: #000; background-color: #353535; } * { box-sizing: border-box; } #app { width: 600px; margin: auto; margin-top: 100px; } .content { float: left; position: relative; height: 150px; width: 150px; margin: 20px; overflow: hidden; background: #ccc; } .content .shade { position: absolute; top: 0; left: -100%; width: 100%; height: 100%; line-height: 100px; color: #fff; background: rgba(0, 0, 0, 0.7); } </style>
js
<script src="https://cdn.bootcss.com/vue/2.5.17-beta.0/vue.min.js"></script> <script> (function () { const mouseHover = { name: 'mouseHover', template: ` <p class="content" @mouseenter="handleIn" @mouseleave="handleOut"> <p class="shade" ref="shade"> <slot></slot> </p> </p> `, data: () => { return {} }, methods: { handleIn: function (e) { const direction = this.direction(e); this.animate(direction, 'in'); }, handleOut: function (e) { const direction = this.direction(e); this.animate(direction, 'out'); }, direction: function (e, type) { const clientX = e.clientX; const clientY = e.clientY; const top = e.target.offsetTop; const bottom = parseInt(top + e.target.offsetHeight); const left = e.target.offsetLeft; const right = parseInt(left + e.target.offsetWidth); const absTop = Math.abs(clientY - top); const absBottom = Math.abs(clientY - bottom); const absLeft = Math.abs(clientX - left); const absRight = Math.abs(clientX - right); const min = Math.min(absTop, absBottom, absLeft, absRight); let direction; switch (min) { case absTop: direction = "top"; break; case absBottom: direction = "bottom"; break; case absLeft: direction = "left"; break; case absRight: direction = "right"; break; }; return direction; }, animate: function (direction, type) { let top = 0, left = 0; if (type == 'in') { this.$refs.shade.style.transition = 'none'; if (direction == 'top') { top = '-100%'; left = 0; } else if (direction == 'right') { top = 0; left = '100%'; } else if (direction == 'bottom') { top = '100%'; left = 0; } else if (direction == 'left') { top = 0; left = '-100%'; } this.$refs.shade.style.top = top; this.$refs.shade.style.left = left; setTimeout(() => { this.$refs.shade.style.transition = 'all .2s ease 0s'; this.$refs.shade.style.top = 0; this.$refs.shade.style.left = 0; }, 0) } else if (type == 'out') { if (direction == 'top') { top = '-100%'; left = 0; } else if (direction == 'right') { top = 0; left = '100%'; } else if (direction == 'bottom') { top = '100%'; left = 0; } else if (direction == 'left') { top = 0; left = '-100%'; } this.$refs.shade.style.top = top; this.$refs.shade.style.left = left; } } } } Vue.component(mouseHover.name, mouseHover) new Vue({ el: '#app' }) })(); </script>
The above is the entire content of this article. I hope it will be helpful to everyone’s study. More related content Please pay attention to PHP Chinese website!
Related recommendations:
Method of converting JS string to number
The above is the detailed content of jQuery/Vue mouse move in and out effect. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
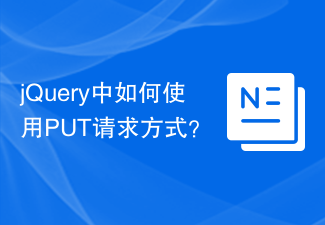
How to use PUT request method in jQuery? In jQuery, the method of sending a PUT request is similar to sending other types of requests, but you need to pay attention to some details and parameter settings. PUT requests are typically used to update resources, such as updating data in a database or updating files on the server. The following is a specific code example using the PUT request method in jQuery. First, make sure you include the jQuery library file, then you can send a PUT request via: $.ajax({u
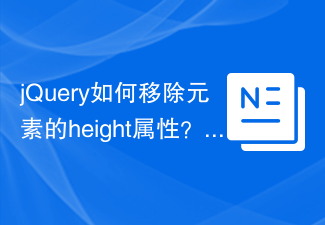
How to remove the height attribute of an element with jQuery? In front-end development, we often encounter the need to manipulate the height attributes of elements. Sometimes, we may need to dynamically change the height of an element, and sometimes we need to remove the height attribute of an element. This article will introduce how to use jQuery to remove the height attribute of an element and provide specific code examples. Before using jQuery to operate the height attribute, we first need to understand the height attribute in CSS. The height attribute is used to set the height of an element
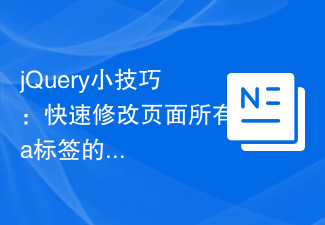
Title: jQuery Tips: Quickly modify the text of all a tags on the page In web development, we often need to modify and operate elements on the page. When using jQuery, sometimes you need to modify the text content of all a tags in the page at once, which can save time and energy. The following will introduce how to use jQuery to quickly modify the text of all a tags on the page, and give specific code examples. First, we need to introduce the jQuery library file and ensure that the following code is introduced into the page: <
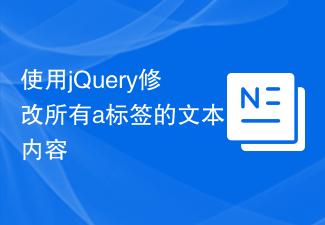
Title: Use jQuery to modify the text content of all a tags. jQuery is a popular JavaScript library that is widely used to handle DOM operations. In web development, we often encounter the need to modify the text content of the link tag (a tag) on the page. This article will explain how to use jQuery to achieve this goal, and provide specific code examples. First, we need to introduce the jQuery library into the page. Add the following code in the HTML file:
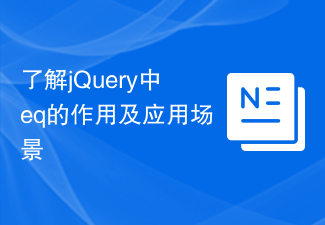
jQuery is a popular JavaScript library that is widely used to handle DOM manipulation and event handling in web pages. In jQuery, the eq() method is used to select elements at a specified index position. The specific usage and application scenarios are as follows. In jQuery, the eq() method selects the element at a specified index position. Index positions start counting from 0, i.e. the index of the first element is 0, the index of the second element is 1, and so on. The syntax of the eq() method is as follows: $("s
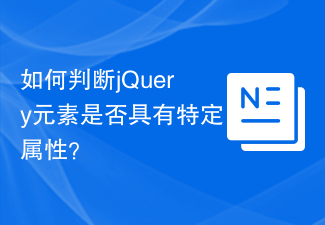
How to tell if a jQuery element has a specific attribute? When using jQuery to operate DOM elements, you often encounter situations where you need to determine whether an element has a specific attribute. In this case, we can easily implement this function with the help of the methods provided by jQuery. The following will introduce two commonly used methods to determine whether a jQuery element has specific attributes, and attach specific code examples. Method 1: Use the attr() method and typeof operator // to determine whether the element has a specific attribute
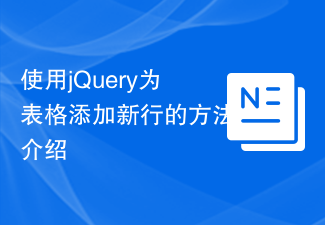
jQuery is a popular JavaScript library widely used in web development. During web development, it is often necessary to dynamically add new rows to tables through JavaScript. This article will introduce how to use jQuery to add new rows to a table, and provide specific code examples. First, we need to introduce the jQuery library into the HTML page. The jQuery library can be introduced in the tag through the following code:

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
