How to use JS to implement WeChat payment pop-up function
This article mainly introduces JS to implement the function of imitating WeChat payment pop-up window. The code is simple and easy to understand, very good, and has certain reference value. Friends who need it can refer to it.
I will provide the renderings first.
html code
<!DOCTYPE html> <html> <head> <title>仿手机微信支付输入密码界面效果</title> <meta charset="utf-8" /> <meta name="viewport" content="initial-scale=1.0, width=device-width, user-scalable=no" /> <link rel="stylesheet" type="text/css" href="css/rest.css" rel="external nofollow" /> </head> <body> <!-- 打开弹窗按钮 --> <button id="myBtn">去支付</button> <!-- 弹窗 --> <p id="myModal" class="modal"> <!-- 弹窗内容 --> <p class="modal-content"> <p class="paymentArea"> <p class="paymentArea-Entry"> <p class="paymentArea-Entry-Head"> <img src="images/xx_03.jpg" class="close" /> <img src="images/jftc_03.png" class="useImg"> <span class="tips-txt">请输入支付密码</span> </p> <p class="paymentArea-Entry-Content"> <p class="pay-name">测试商品</p> <p class="pay-price">¥88.88</p> </p> <ul class="paymentArea-Entry-Row"></ul> </p> <p class="paymentArea-Keyboard"> <h4> <img src="images/jftc_14.jpg" height="10" /> </h4> <ul class="target"> <li> <a>1</a> <a>2</a> <a>3</a> </li> <li> <a>4</a> <a>5</a> <a>6</a> </li> <li> <a>7</a> <a>8</a> <a>9</a> </li> <li> <a>清空</a> <a> 0 </a> <a>删除</a> </li> </ul> </p> </p> </p> </p> </body> </html>
css
body { margin: 0; padding: 0; font-size: 0.3rem; font-family: "微软雅黑", arial; } ul, li { margin: 0; padding: 0; list-style: none; } img { display: block; } #myBtn { display: block; width: 80%; height: auto; margin: 5rem auto; padding: 0.2rem; border-radius: 5px; border: 0; outline: none; font-family: "微软雅黑"; color: #fff; background-color: #5CB85C; } /* 弹窗 */ .modal { display: none; /* 默认隐藏 */ position: fixed; z-index: 1; left: 0; top: 0; width: 100%; height: 100%; overflow: auto; background-color: rgb(0, 0, 0); background-color: rgba(0, 0, 0, 0.4); -webkit-animation-name: fadeIn; -webkit-animation-duration: 0.4s; animation-name: fadeIn; animation-duration: 0.4s } /* 弹窗内容 */ .modal-content { position: fixed; bottom: 0; /*background-color: #fefefe;*/ width: 100%; -webkit-animation-name: slideIn; -webkit-animation-duration: 0.4s; animation-name: slideIn; animation-duration: 0.4s } /** * 支付弹窗样式 * **/ .paymentArea-Entry { width: 90%; margin: 0 auto; padding-bottom: 0.3rem; background-color: #fff; } .paymentArea-Entry-Head { display: flex; display: -webkit-flex; height: 0.8rem; line-height: 0.8rem; padding: 0.2rem; border-bottom: 1px solid #5CB85C; } /* 关闭按钮 */ .paymentArea-Entry-Head .close { width: 0.5rem; height: 0.5rem; padding: 0.15rem 0.15rem 0.15rem 0; } .paymentArea-Entry-Head .close:hover, .paymentArea-Entry-Head .close:focus { color: #000; text-decoration: none; cursor: pointer; } .paymentArea-Entry-Head .useImg { width: 0.8rem; height: 0.8rem; margin-right: 0.15rem; } .paymentArea-Entry-Head .tips-txt { font-size: 0.4rem; } .paymentArea-Entry-Content { position: relative; padding: 0.2rem 0; text-align: center; } .paymentArea-Entry-Content:after { content: ""; position: absolute; bottom: 0; left: 0.3rem; right: 0.3rem; height: 1px; background-color: #ddd; } .paymentArea-Entry-Content .pay-name { font-size: 0.3rem; } .paymentArea-Entry-Content .pay-price { font-size: 0.4rem; font-weight: bold; } ul.paymentArea-Entry-Row { display: flex; display: -webkit-flex; justify-content: space-between; margin: 0.2rem 0.3rem 0 0.3rem; padding: 0; border: 1px solid #a2a2a2; } ul.paymentArea-Entry-Row li { position: relative; flex-grow: 1; min-width: 1rem; height: 0.8rem; line-height: 0.8rem; text-align: center; border-right: 1px solid #ddd; } ul.paymentArea-Entry-Row li:last-child { border-right: 0; } ul.paymentArea-Entry-Row li img { position: absolute; top: 50%; left: 50%; width: 0.5rem; height: 0.5rem; margin: -0.25rem 0 0 -0.25rem; } .paymentArea-Keyboard { margin-top: 1.2rem; background-color: #fff; } .paymentArea-Keyboard h4 { height: 0.5rem; line-height: 0.5rem; margin: 0; text-align: center; } .paymentArea-Keyboard h4 img { width: 0.93rem; height: 0.32rem; margin: 0 auto; } .paymentArea-Keyboard h4:active { background-color: #e3e3e3; } .paymentArea-Keyboard ul { border-top: 1px solid #ddd; } .paymentArea-Keyboard li { display: flex; display: -webkit-flex; justify-content: space-between; border-bottom: 1px solid #ddd; } .paymentArea-Keyboard li a { flex-grow: 1; display: block; min-width: 1rem; line-height: 1rem; border-right: 1px solid #ddd; font-size: 0.3rem; text-align: center; text-decoration: none; color: #000; } .paymentArea-Keyboard li:last-child, .paymentArea-Keyboard li a:last-child { border: 0; } .paymentArea-Keyboard li a:active { outline: none; background-color: #ddd; } /* 添加动画 */ @-webkit-keyframes slideIn { from { bottom: -300px; opacity: 0 } to { bottom: 0; opacity: 1 } } @keyframes slideIn { from { bottom: -300px; opacity: 0 } to { bottom: 0; opacity: 1 } } @-webkit-keyframes fadeIn { from { opacity: 0 } to { opacity: 1 } } @keyframes fadeIn { from { opacity: 0 } to { opacity: 1 } }
js
//定义根目录字体大小,通过rem实现适配 document.addEventListener("DOMContentLoaded", function() { var html = document.documentElement; var windowWidth = html.clientWidth; //console.log(windowWidth) html.style.fontSize = windowWidth / 7.5 + "px"; }, false); // 获取弹窗 var modal = document.getElementById('myModal'); // 打开弹窗的按钮对象 var btn = document.getElementById("myBtn"); // 获取 <span> 元素,用于关闭弹窗 that closes the modal var span = document.getElementsByClassName("close")[0]; /*创建密码输入框*/ var entryArea = document.querySelector(".paymentArea-Entry-Row"); for(var i = 0; i < 6; i++) { var li = document.createElement("li"); entryArea.appendChild(li); } /*键盘操作*/ var doms = document.querySelectorAll('ul li a'); var entryLis = document.querySelectorAll(".paymentArea-Entry-Row li"); var pwds = ""; //存储密码 var count = 0; //记录点击次数 for(var i = 0; i < doms.length; i++) { ! function(dom, index) { dom.addEventListener('click', function() { var txt = this.innerHTML; switch(txt) { case "清空": if(count != 0) { for(var i = 0; i < entryLis.length; i++) { entryLis[i].innerHTML = ""; } pwds = ""; count = 0; console.log(pwds) console.log(count) } break; case "删除": if(count != 0) { console.log(pwds) entryLis[count - 1].innerHTML = ""; pwds = pwds.substring(0, pwds.length - 1); count--; console.log(pwds) console.log(count) } break; default: /*通过判断点击次数 向输入框填充内容*/ if(count < 6) { /*创建一个图片对象 插入到方框中*/ var img = new Image(); img.src = "images/dd_03.jpg"; entryLis[count].appendChild(img); /*为存储密码的对象赋值*/ if(pwds == "") { pwds = txt; } else { pwds += txt; } count++; if(pwds.length == 6) { location.href = "https://www.baidu.com"; } } else { return; alert("超出限制") } } }); }(doms[i], i); } // 点击按钮打开弹窗 btn.onclick = function() { modal.style.display = "block"; } // 点击 <span> (x), 关闭弹窗 span.onclick = function() { modal.style.display = "none"; if(count != 0) { for(var i = 0; i < entryLis.length; i++) { entryLis[i].innerHTML = ""; } pwds = ""; count = 0; } } // 在用户点击其他地方时,关闭弹窗 window.onclick = function(event) { if(event.target == modal) { modal.style.display = "none"; if(count != 0) { for(var i = 0; i < entryLis.length; i++) { entryLis[i].innerHTML = ""; } pwds = ""; count = 0; } } } /*开关键盘*/ var openKey = document.querySelector(".paymentArea-Entry-Row"); var switchOfKey = document.querySelector(".paymentArea-Keyboard h4"); switchOfKey.addEventListener('click', function() { var KeyboardH = document.querySelector(".paymentArea-Keyboard").clientHeight; document.querySelector(".paymentArea-Keyboard").style.height = KeyboardH + "px"; document.querySelector(".paymentArea-Keyboard").style.backgroundColor = "transparent"; document.querySelector(".paymentArea-Keyboard h4").style.display = "none"; document.querySelector(".paymentArea-Keyboard ul").style.display = "none"; }) openKey.addEventListener('click', function() { document.querySelector(".paymentArea-Keyboard").style.backgroundColor = "#fff"; document.querySelector(".paymentArea-Keyboard h4").style.display = "block"; document.querySelector(".paymentArea-Keyboard ul").style.display = "block"; })
The above is the entire content of this article. I hope it will be helpful to everyone’s learning. For more related content, please pay attention to PHP Chinese net!
Related recommendations:
js imitation QQ operation of sliding the contact to the left and sliding out the delete button
Use JS to display/hide window fixed position elements as the page scrolls
The above is the detailed content of How to use JS to implement WeChat payment pop-up function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
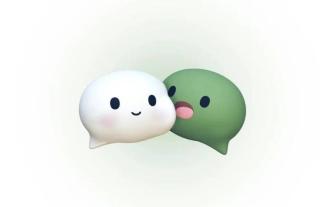
In WeChat, users can enter their payment password to make purchases, but how do they retrieve their payment password if they forget it? Users need to go to My-Services-Wallet-Payment Settings-to recover their payment password if they forget it. This introduction to how to retrieve your payment password if you forget it will tell you the specific operation method. The following is a detailed introduction, so take a look! WeChat usage tutorial. How to find the WeChat payment password if you forget it? Answer: My-Service-Wallet-Payment Settings-Forgot payment password. Specific method: 1. First, click My. 2. Click on the service inside. 3. Click on the wallet inside. 4. Find the payment settings. 5. Click Forgot payment password. 6. Enter your own information for verification. 7. Then enter the new payment password to change it.
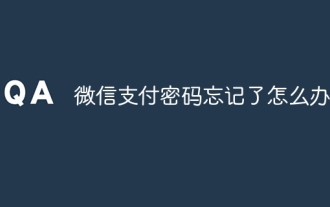
Solution for forgetting WeChat payment password: 1. Open WeChat APP, click "I" in the lower right corner to enter the personal center page; 2. In the personal center page, click "Pay" to enter the payment page; 3. On the payment page , click "..." in the upper right corner to enter the payment management page; 4. In the payment management page, find and click "Forgot payment password"; 5. Follow the page prompts and enter personal information for identity verification. After successful verification, you can Choose the method of "retrieve by swiping your face" or "retrieve by verifying bank card information" to retrieve your password, etc.
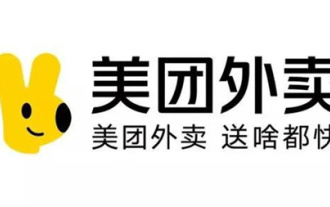
There are many food and snack shops provided in the Meituan takeout app, and all mobile phone users log in through their accounts. Add your personal delivery address and contact number to enjoy the most convenient takeout service. Open the homepage of the software, enter product keywords, and search online to find the corresponding product results. Just swipe up or down to purchase and place an order. The platform will also recommend dozens of nearby restaurants with high reviews based on the delivery address provided by the user. The store can also set up different payment methods. You can place an order with one click to complete the order. The rider can arrange the delivery immediately and the delivery speed is very fast. There are also takeout red envelopes of different amounts for use. Now the editor is online in detail for Meituan takeout users. We show you how to set up WeChat payment. 1. After selecting the product, submit the order and click Now
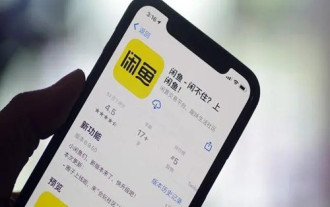
When everyone has nothing to do, they will choose to browse the Xianyu platform. Everyone can find that there are a large number of products on this platform, which can allow everyone to see various second-hand products. Although these products are second-hand products, there is absolutely no problem with the quality of these products, so everyone can buy them with confidence. The prices are very affordable, and they still allow everyone to face-to-face with these products. It is entirely possible for sellers to communicate and conduct some price bargaining operations. As long as everyone negotiates properly, then you can choose to conduct transactions, and when everyone pays here, they want to make WeChat payment, but it seems that the platform It's not allowed. Please follow the editor to find out what the specific situation is. Xianyu
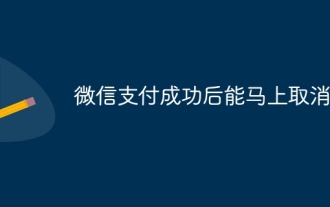
WeChat payment cannot be canceled immediately after successful payment. Refunds usually need to meet the following conditions: 1. The merchant's refund policy. The merchant will formulate its own refund policy, including the refund time window, refund amount and refund method; 2. Payment time, refunds usually require Apply within a certain time frame, and refunds may not be possible beyond this time frame; 3. Goods or service status. If the user has received the goods or enjoyed the service, the merchant may require the user to return the goods or provide corresponding proof; 4. Refund process, etc.
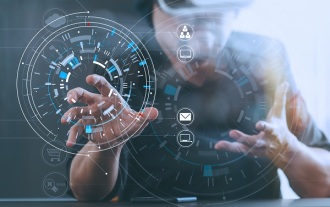
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
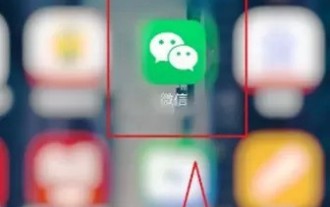
1. First, we need to open the WeChat APP on the mobile phone, and then click to log in to the WeChat account, so that we enter the WeChat homepage. 2. Click the [Me] button in the lower right corner of the WeChat homepage, then select the [Payment] option. We click to enter the payment page. 3. After entering the [Payment] page, click the [Wallet] option to enter, and click [Bill] in the upper right corner of the [Wallet] page.

Alibaba 1688 is a purchasing and wholesale website, and the items there are much cheaper than Taobao. So how does Alibaba use WeChat payment? The editor has compiled some relevant content to share with you. Friends in need can come and take a look. How does Alibaba use WeChat payment? Answer: WeChat payment cannot be used for the time being; 1. On the page where we purchase goods, we click [Change payment method] 2. Then in the pop-up page, we can only go to [Alipay, staged payment] , cashier] can be selected;
