How to implement data request display loading graph in vue2
This article mainly introduces vue2 to implement data request display loading diagram in detail, which has certain reference value. Interested friends can refer to it
In general projects, sometimes it is required, You display a gif image during data request, and then disappear after the data is loaded. For this, you generally only need to write js events in the encapsulated axios. Of course, we first need to add this image to app.vue. As follows:
<template> <p id="app"> <loading v-show="fetchLoading"></loading> <router-view></router-view> </p> </template> <script> import { mapGetters } from 'vuex'; import Loading from './components/common/loading'; export default { name: 'app', data() { return { } }, computed: { ...mapGetters([ 'fetchLoading', ]), }, components: { Loading, } } </script> <style> #app{ width: 100%; height: 100%; } </style>
The fetchLoading here is a variable stored in vuex. The following definition is required in store/modules/common.js:
/* 此js文件用于存储公用的vuex状态 */ import api from './../../fetch/api' import * as types from './../types.js' const state = { // 请求数据时加载状态loading fetchLoading: false } const getters = { // 请求数据时加载状态 fetchLoading: state => state.fetchLoading } const actions = { // 请求数据时状态loading FETCH_LOADING({ commit }, res) { commit(types.FETCH_LOADING, res) }, } const mutations = { // 请求数据时loading [types.FETCH_LOADING] (state, res) { state.fetchLoading = res } }
The loading component is as follows:
<template> <p class="loading"> <img src="./../../assets/main/running.gif" alt=""> </p> </template> <script> export default { name: 'loading', data () { return {} }, } </script> <!-- Add "scoped" attribute to limit CSS to this component only --> <style scoped> .loading{ position: fixed; top:0; left:0; z-index:121; width: 100%; height: 100%; background: rgba(0,0,0,0.3); display: table-cell; vertical-align: middle; text-align: center; } .loading img{ margin:5rem auto; } </style>
Finally, write the judgment loading event in the axios encapsulated in fetch/api.js : As follows
// axios的请求时间 let axiosDate = new Date() export function fetch (url, params) { return new Promise((resolve, reject) => { axios.post(url, params) .then(response => { // 关闭 loading图片消失 let oDate = new Date() let time = oDate.getTime() - axiosDate.getTime() if (time < 500) time = 500 setTimeout(() => { store.dispatch('FETCH_LOADING', false) }, time) resolve(response.data) }) .catch((error) => { // 关闭 loading图片消失 store.dispatch('FETCH_LOADING', false) axiosDate = new Date() reject(error) }) }) } export default { // 组件中公共页面请求函数 commonApi (url, params) { if(stringQuery(window.location.href)) { store.dispatch('FETCH_LOADING', true); } axiosDate = new Date(); return fetch(url, params); } }
This is achieved. When the data is loaded in the project, the gif image is displayed and disappears when the data is loaded.
Regarding vue.js learning tutorials, please click on the special vue.js component learning tutorials and Vue.js front-end component learning tutorials to learn.
For more vue learning tutorials, please read the special topic "Vue Practical Tutorial"
The above is what I compiled for everyone. I hope it will be helpful to everyone in the future.
Related articles:
Using Angular5 to implement server-side rendering practice
How to reset the idle state in vuex
Use jQuery to encapsulate animate.css (detailed tutorial)
vue-cli configuration file (detailed tutorial)
The above is the detailed content of How to implement data request display loading graph in vue2. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
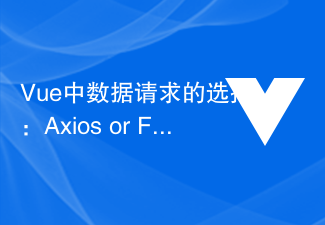
Choice of data request in Vue: AxiosorFetch? In Vue development, handling data requests is a very common task. Choosing which tool to use for data requests is a question that needs to be considered. In Vue, the two most common tools are Axios and Fetch. This article will compare the pros and cons of both tools and give some sample code to help you make your choice. Axios is a Promise-based HTTP client that works in browsers and Node.
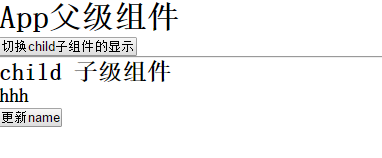
Difference in life cycle execution order between vue2 and vue3 Life cycle comparison The execution order in vue2 beforeCreate=>created=>beforeMount=>mounted=>beforeUpdate=>updated=>beforeDestroy=>destroyed The execution order in vue3 setup=>onBeforeMount=>onMounted=> onBeforeUpdate=>onUpdated=>onBeforeUnmount=&g
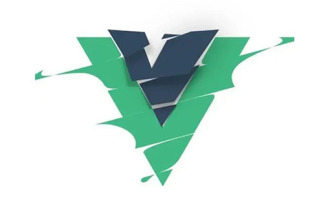
The diff algorithm is an efficient algorithm that compares tree nodes at the same level, avoiding the need to search and traverse the tree layer by layer. So how much do you know about the diff algorithm? The following article will give you an in-depth analysis of the diff algorithm of vue2. I hope it will be helpful to you!
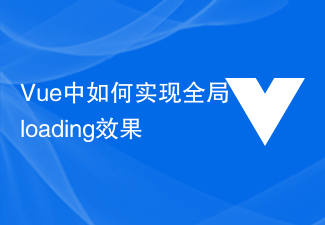
In front-end development, we often have a scenario where the user needs to wait for the data to be loaded during interaction with the web page. At this time, there is usually a loading effect displayed to remind the user to wait. In the Vue framework, it is not difficult to implement a global loading effect. Let’s introduce how to implement it. Step 1: Create a Vue plug-in We can create a Vue plug-in named loading, which can be referenced in all Vue instances. In the plug-in, we need to implement the following two methods: s
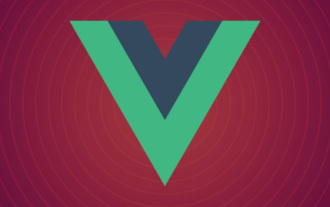
This article will take you through Vue learning and talk about how to set up the 404 interface in Vue2 and Vue3. I hope it will be helpful to you!
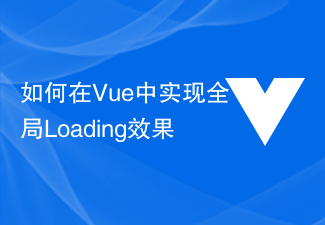
How to implement global Loading effects in Vue In Vue development, implementing global Loading effects is a common requirement. The global Loading effect can give users a good prompt to let them know that the page is loading, improving the user experience. This article will introduce how to implement global Loading effects in Vue and provide specific code examples. Create a global Loading component First, we need to create a global Loading component. This component can be a simple
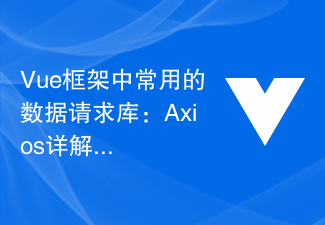
Commonly used data request libraries in the Vue framework: Axios detailed explanation Title: Commonly used data request libraries in the Vue framework: Axios detailed explanation Introduction: In Vue development, data request is an essential part. As a commonly used data request library in Vue, Axios has a simple and easy-to-use API and powerful functions, making it the preferred data request tool in front-end development. This article will introduce in detail how to use Axios and some common application scenarios, and provide corresponding code examples for readers' reference. Axios
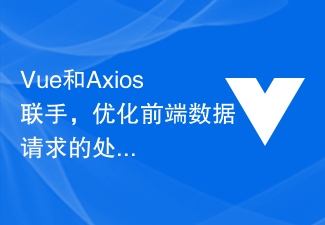
Vue and Axios join forces to optimize the processing process of front-end data requests. Front-end development often requires data interaction with the back-end. Data request and processing is one of the core tasks of front-end development. Vue.js is a popular front-end framework, and Axios is a Promise-based HTTP library. The combination of the two can greatly optimize the processing process of front-end data requests. This article will introduce how to use Vue and Axios together, and the examples are as follows. First, we need to introduce Vue and Axios into the project
